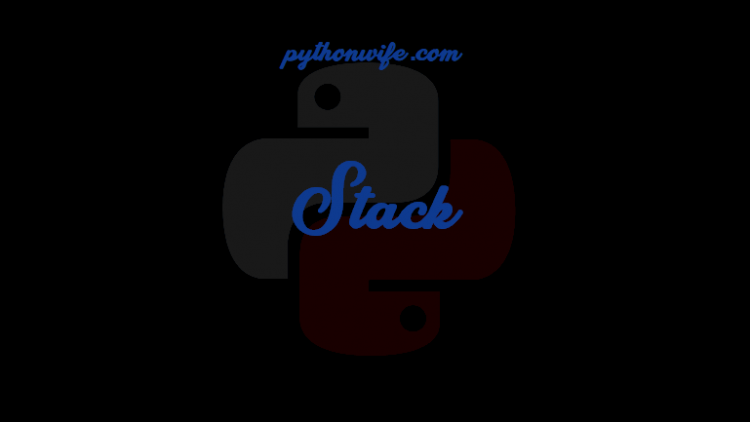
In python, the stack is an abstract data structure that stores elements linearly. The items in a stack follow the Last-In/First-Out (LIFO) order. This means that the last element to be inserted in a stack will be the first one to be removed.
We can illustrate the “stack” data structure with the real-life example of a stack of plates. The last plate will be conventionally placed on top of the stock. While taking a plate from the stack, a person will remove the plate kept on the top. This demonstrates how the LIFO manner works. The plate or the data item inserted last in the stack will be the first to be removed.
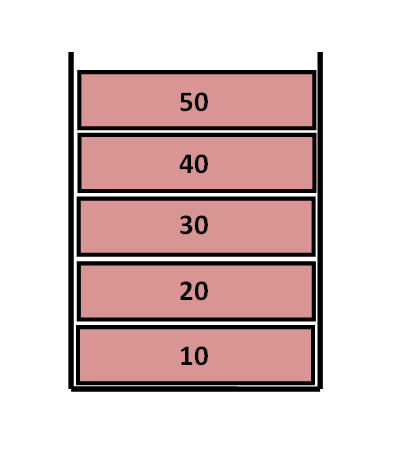
Stack Operations
Various operations can be performed on a stack in python.
- Create Stack – The creation of a stack is the most fundamental operation. Just like any other linear data structure, a stack can be implemented in python and used to store data elements.
- Push – The push operation is used to insert new elements into a stack. There is only one position where new elements can be entered , i.e., the top of a stack.
- Pop – The pop function is used for the removal of a data item from a stack data structure. Data items are also removed from the top of the stack following the LIFO approach.
- Peek – Peek operation is used to retrieve the element at the top of the stack without deleting it.
- isEmpty – The isEmpty method returns whether a stack is empty or not. This method returns a boolean value of True if the stack is empty, otherwise it returns False.
- isFull – The isFull method returns whether a stack is full or not. This method returns a boolean value of True if the stack is full, otherwise it returns False.
- deleteStack – Removes all the data elements and free up the allocated memory space.
Stack creation using List without size limit
The creation of Stack using the list data structure in python is a simple process. Create a stack class and initialize an “int” list inside the “Stack” class. We also create the “__str__” function of this class to return the string version of our stack in the desired order. When we create “__str__” function, it modifies the “__str__” function of python.
The advantage of using the list data structure to create Stack is that it is easy to implement and the list can be limitless. We can use the in-built functions, append and pop, associated with lists. However, its implementation may become slower as the size of the list increases.
#Creating Stack class using list without size limit
class Stack:
def __init__(self):
self.list = []
#Modifying the __str__ function to return the desired string version of Stack
def __str__(self):
values = self.list.reverse()
values = [str(x) for x in self.list]
return '\n'.join(values)
Time and Space Complexity
The time complexity of creating a Stack using a list is O(1) as it takes a constant amount of time to initialize a list. The space complexity is O(1) as well since no additional memory is required.
Operations on Stack using List without size limit
isEmpty
We define the isEmpty function to check whether the given stack is empty or not. If there are no elements in the list, we return a boolean value of True, otherwise False.
#isEmpty Function to check whether the stack is empty
def isEmpty(self):
if self.list == []:
return True
else:
return False
#Example
tempStack = Stack()
print(tempStack.isEmpty())
#Output
True
The time complexity is O(1) as it takes a constant time for initialization and space complexity is O(1) as no additional memory is required.
push
The push function is used to insert elements into the stack. We use the in-built function append which simply adds values to a pre-defined list.
#push Function to insert elements
def push(self, value):
self.list.append(value)
return "The element has been successfully added to the stack."
#Example
tempStack = Stack()
tempStack.push(1)
tempStack.push(2)
tempStack.push(3)
print(tempStack)
#Output
3
2
1
pop
The pop function is used to delete elements from a stack. We use the in-built pop function which deletes values from a predefined list.
#pop Function to remove elements
def pop(self):
if self.isEmpty():
return "The stack is empty."
else:
return self.list.pop()
#Example
tempStack = Stack()
tempStack.push(1)
tempStack.push(2)
tempStack.push(3)
tempStack.pop()
print(tempStack)
#Output
2
1
peek
The peek function is used to return the topmost value from the stack without actually deleting that element. We can access this element from our stack by using indexing on the list data structure.
#peek Function to return the topmost element
def peek(self):
if self.isEmpty():
return "The stack is empty."
else:
return self.list[len(self.list)-1]
#Example
tempStack = Stack()
tempStack.push(1)
tempStack.push(2)
tempStack.push(3)
print(tempStack.peek())
#Output
3
delete
The delete function is used to delete the entire list and thereby the stack. We achieve this by setting the list to None and deallocating the occupied space in memory.
#delete Function to the entire stack
def delete(self):
self.list = None
#Example
tempStack = Stack()
tempStack.push(1)
tempStack.push(2)
tempStack.push(3)
tempStack.delete()
Stack creation using List with size limit
Previously, we had created a stack where there was no upper bound as to how many elements could be inserted. Now, we will create a stack where the size will be predefined.
Stack creation using a list having a size limit has the same isEmpty(), pop(), peek(), and delete() methods like the one without size limit. However, the methods isFull() and push() are different since they require an upper bound condition when the stack gets fully filled.
#Creating Stack class using list with size limit
class Stack:
def __init__(self, maxSize):
self.maxSize = maxSize
self.list = []
#Modifying the __str__ function to return the desired string version of Stack
def __str__(self):
values = self.list.reverse()
values = [str(x) for x in self.list]
return '\n'.join(values)
Time and Space Complexity
The time complexity of creating a Stack using a list is O(1) as it takes a constant amount of time to initialize a list. The space complexity is O(1) as well since no additional memory is required.
Operations on Stack using List with size limit
isFull
The isFull function checks whether a given stack is fully filled or not. If the max occupancy of the list is reached, we return a boolean value of Tree, otherwise False.
#isFull Function to check whether the stack is full or not
def isFull(self):
if len(self.list) == self.maxSize:
return True
else:
return False
#Example
tempStack = Stack(3)
tempStack.push(1)
tempStack.push(2)
tempStack.push(3)
print(tempStack.isFull())
#Output
True
The time complexity is O(1) as it takes a constant time for the comparison of two values. The space complexity is O(1) as no additional memory is required.
push
The push function is used to insert elements into a stack. We use the in-built function “append” to enter elements in the list. However, in the case of limited size, we stop the process of adding elements when the max occupancy is reached.
#push Function to insert elements
def push(self, value):
if self.isFull():
return "The stack is full."
else:
self.list.append(value)
return "The element has been successfully added to the stack."
#Example
tempStack = Stack(3)
tempStack.push(1)
tempStack.push(2)
tempStack.push(3)
print(tempStack)
#Output
3
2
1
Time and Space complexity of Operations on Stack using List
Time Complexity SpaceComplexity Create Stack O(1) O(1) Push O(1)/O(n^2) O(1) Pop O(1) O(1) Peek O(1) O(1) isEmpty/isFull O(1) O(1) Delete Entire Stack O(1) O(1)
Creation of Stack using Linked List
Time Complexity | SpaceComplexity | |
Create Stack | O(1) | O(1) |
Push | O(1)/O(n^2) | O(1) |
Pop | O(1) | O(1) |
Peek | O(1) | O(1) |
isEmpty/isFull | O(1) | O(1) |
Delete Entire Stack | O(1) | O(1) |
In python, we can implement a stack by using a linked list as its inclusive data structure. The complexity of the data structure increases but operations such as insertion, deletion, traversal, etc. take significantly less amount of time to execute.
To create a stack using the linked list data structure, firstly we create the node and linked list class for our fundamental data structure. Then we initialize the “Stack” class which includes an instance of the linked list data structure.
#Creating the Node class
class Node:
def __init__(self, value = None):
self.value = value
self.next = None
#Create the Linked List class
class LinkedList:
def __init__(self):
self.head = None
#Iterator Function
def __iter__(self):
node = self.head
while node:
yield node
node = node.next
#Creating Stack class using list linked list
class Stack:
def __init__(self):
self.LinkedList = LinkedList()
#Modifying the __str__ function to return the desired string version of Stack
def __str__(self):
values = [str(x.value) for x in self.LinkedList]
return '\n'.join(values)
Operations on Stack using Linked List
isEmpty
If the head node of the linked list is none, this indicates that the stack is empty.
#isEmpty Function to check whether the stack is empty
def isEmpty(self):
if self.LinkedList.head == None:
return True
else:
return False
#Example
tempStack = Stack()
print(tempStack.isEmpty())
#Output
True
push
New elements are inserted at the top of the linked list. Every time we push a data item to the stack, the head node points to the newly added node.
#push Function to insert elements
def push(self, value):
new_node = Node(value)
new_node.next = self.LinkedList.head
self.LinkedList.head = new_node
#Example
tempStack = Stack()
tempStack.push(1)
tempStack.push(2)
tempStack.push(3)
print(tempStack)
#Output
3
2
1
pop
The elements are removed from the stack with the help of the head reference. The head node always points to the element at the top of the stack, which needs to be popped.
#pop Function to remove elements
def pop(self):
if self.isEmpty():
return "The stack is empty."
else:
temp_node = self.LinkedList.head.value
self.LinkedList.head = self.LinkedList.head.next
return temp_node
#Example
tempStack = Stack()
tempStack.push(1)
tempStack.push(2)
tempStack.push(3)
tempStack.pop()
print(tempStack)
#Output
2
1
peek
The peek function returns the topmost value from the stack. While using linked list, head node is the topmost element. Hence, we return the value of the head node without removing it from the stack.
#peek Function to return the topmost element
def peek(self):
if self.isEmpty():
return "The stack is empty."
else:
temp_node = self.LinkedList.head.value
return temp_node
#Example
tempStack = Stack()
tempStack.push(1)
tempStack.push(2)
tempStack.push(3)
print(tempStack.peek())
#Output
3
delete
To delete an entire stack built using linked list, we simple set the head node of the linked list to None, thereby deallocating the used up memory space.
#delete Function to the entire stack
def delete(self):
self.LinkedList.head = None
#Example
tempStack = Stack()
tempStack.push(1)
tempStack.push(2)
tempStack.push(3)
tempStack.delete()
Time and Space complexity of Operations on Stack built using Linked List
Time Complexity SpaceComplexity Create Stack O(1) O(1) Push O(1) O(1) Pop O(1) O(1) Peek O(1) O(1) isEmpty O(1) O(1) Delete Entire Stack O(1) O(1)
Interview Questions on Stacks
Design a data structure to implement three stacks using a single list.
#Implementing three stacks using a single list
class MultiStack:
def __init__(self, stackSize):
self.numberOfStacks = 3
self.tempList = [0] * (stackSize * self.numberOfStacks)
self.sizes = [0] * self.numberOfStacks
self.stackSize = stackSize
def isFull(self, numStack):
if self.sizes[numStack] == self.stackSize:
return True
else:
return False
def isEmpty(self, numStack):
if self.sizes[numStack] == 0:
return True
else:
return False
def topIndex(self, numStack):
offset = numStack * self.stackSize
return offset + self.sizes[numStack] - 1
def push(self, item, numStack):
if self.isFull(numStack):
return "The stack is full"
else:
self.sizes[numStack] += 1
self.tempList[self.topIndex(numStack)] = item
def pop(self, numStack):
if self.isEmpty(numStack):
return "The stack is empty"
else:
value = self.tempList[self.topIndex(numStack)]
self.tempList[self.topIndex(numStack)] = 0
self.sizes[numStack] -= 1
return value
def peek(self, numStack):
if self.isEmpty(numStack):
return "The stack is empty"
else:
value = self.tempList[self.topIndex(numStack)]
return value
tempStack = MultiStack(6)
print(tempStack.isFull(0))
print(tempStack.isEmpty(1))
tempStack.push(1, 0)
tempStack.push(2, 0)
tempStack.push(3, 2)
print(tempStack.pop(0))
#Output
False
True
2
Create a stack data structure with a custom min function which returns the element with the minimum value. The push, pop and min function should execute in O(1) complexity.
#Creating stack data structure with min function
class Node():
def __init__(self, value=None, next=None):
self.value = value
self.next = next
def __str__(self):
string = str(self.value)
if self.next:
string += ' ' + str(self.next)
return string
class Stack():
def __init__(self):
self.top = None
self.min_node = None
def min(self):
if not self.min_node:
return None
return self.min_node.value
def push(self, item):
if self.min_node and (self.min_node.value < item):
self.min_node = Node(value=self.min_node.value, next=self.min_node)
else:
self.min_node = Node(value=item, next=self.min_node)
self.top = Node(value=item, next=self.top)
def pop(self):
if not self.top:
return None
self.min_node = self.min_node.next
item = self.top.value
self.top = self.top.next
return item
tempStack = Stack()
tempStack.push(5)
print(tempStack.min())
tempStack.push(6)
print(tempStack.min())
tempStack.push(3)
print(tempStack.min())
tempStack.pop()
print(tempStack.min())
#Output
5
5
3
5
Consider a stack of plates. Each stack has a certain threshold, above which it would topple. Create a stack data structure which initializes new stacks every time the previous stack reaches its threshold. The push and pop functions should execute similar to a normal stack.
#Creating SetOfStacks class to resolve the threshold problem.
class SetOfStacks():
def __init__(self, capacity):
self.capacity = capacity
self.stacks = []
def __str__(self):
return self.stacks
def push(self, item):
if len(self.stacks) > 0 and (len(self.stacks[-1])) < self.capacity:
self.stacks[-1].append(item)
else:
self.stacks.append([item])
def pop(self):
while len(self.stacks) and len(self.stacks[-1]) == 0:
self.stacks.pop()
if len(self.stacks) == 0:
return None
else:
return self.stacks[-1].pop()
def pop_at(self, stack_number):
if len(self.stacks[stack_number]) > 0:
return self.stacks[stack_number].pop()
else:
return None
tempStack = SetOfStacks(2)
tempStack.push(1)
tempStack.push(2)
tempStack.push(3)
tempStack.push(4)
print(tempStack.pop_at(1))
#Output
4
FAQ Questions:
How to Create a Stack in Python?
Time Complexity | SpaceComplexity | |
Create Stack | O(1) | O(1) |
Push | O(1) | O(1) |
Pop | O(1) | O(1) |
Peek | O(1) | O(1) |
isEmpty | O(1) | O(1) |
Delete Entire Stack | O(1) | O(1) |
Design a data structure to implement three stacks using a single list.
#Implementing three stacks using a single list
class MultiStack:
def __init__(self, stackSize):
self.numberOfStacks = 3
self.tempList = [0] * (stackSize * self.numberOfStacks)
self.sizes = [0] * self.numberOfStacks
self.stackSize = stackSize
def isFull(self, numStack):
if self.sizes[numStack] == self.stackSize:
return True
else:
return False
def isEmpty(self, numStack):
if self.sizes[numStack] == 0:
return True
else:
return False
def topIndex(self, numStack):
offset = numStack * self.stackSize
return offset + self.sizes[numStack] - 1
def push(self, item, numStack):
if self.isFull(numStack):
return "The stack is full"
else:
self.sizes[numStack] += 1
self.tempList[self.topIndex(numStack)] = item
def pop(self, numStack):
if self.isEmpty(numStack):
return "The stack is empty"
else:
value = self.tempList[self.topIndex(numStack)]
self.tempList[self.topIndex(numStack)] = 0
self.sizes[numStack] -= 1
return value
def peek(self, numStack):
if self.isEmpty(numStack):
return "The stack is empty"
else:
value = self.tempList[self.topIndex(numStack)]
return value
tempStack = MultiStack(6)
print(tempStack.isFull(0))
print(tempStack.isEmpty(1))
tempStack.push(1, 0)
tempStack.push(2, 0)
tempStack.push(3, 2)
print(tempStack.pop(0))
#Output
False
True
2
Create a stack data structure with a custom min function which returns the element with the minimum value. The push, pop and min function should execute in O(1) complexity.
#Creating stack data structure with min function
class Node():
def __init__(self, value=None, next=None):
self.value = value
self.next = next
def __str__(self):
string = str(self.value)
if self.next:
string += ' ' + str(self.next)
return string
class Stack():
def __init__(self):
self.top = None
self.min_node = None
def min(self):
if not self.min_node:
return None
return self.min_node.value
def push(self, item):
if self.min_node and (self.min_node.value < item):
self.min_node = Node(value=self.min_node.value, next=self.min_node)
else:
self.min_node = Node(value=item, next=self.min_node)
self.top = Node(value=item, next=self.top)
def pop(self):
if not self.top:
return None
self.min_node = self.min_node.next
item = self.top.value
self.top = self.top.next
return item
tempStack = Stack()
tempStack.push(5)
print(tempStack.min())
tempStack.push(6)
print(tempStack.min())
tempStack.push(3)
print(tempStack.min())
tempStack.pop()
print(tempStack.min())
#Output
5
5
3
5
Consider a stack of plates. Each stack has a certain threshold, above which it would topple. Create a stack data structure which initializes new stacks every time the previous stack reaches its threshold. The push and pop functions should execute similar to a normal stack.
#Creating SetOfStacks class to resolve the threshold problem.
class SetOfStacks():
def __init__(self, capacity):
self.capacity = capacity
self.stacks = []
def __str__(self):
return self.stacks
def push(self, item):
if len(self.stacks) > 0 and (len(self.stacks[-1])) < self.capacity:
self.stacks[-1].append(item)
else:
self.stacks.append([item])
def pop(self):
while len(self.stacks) and len(self.stacks[-1]) == 0:
self.stacks.pop()
if len(self.stacks) == 0:
return None
else:
return self.stacks[-1].pop()
def pop_at(self, stack_number):
if len(self.stacks[stack_number]) > 0:
return self.stacks[stack_number].pop()
else:
return None
tempStack = SetOfStacks(2)
tempStack.push(1)
tempStack.push(2)
tempStack.push(3)
tempStack.push(4)
print(tempStack.pop_at(1))
#Output
4
FAQ Questions:
How to Create a Stack in Python?
The stack class can be used to create a stack with functions like pop()
and push()
to insert and remove elements from the stack.
class Stack:
def __init__(self):
self.items = []
def push(self, item):
self.items.append(item)
def pop(self):
return self.items.pop()
def size(self):
return len(self.items)
def printstack(self):
x = self.size()
if(x>0):
print(self.items)
else:
print("stack is empty")
sample = Stack()
sample.push('Welcome')
sample.push('To')
sample.push('Pythonwife')
sample.push('Website')
sample.printstack()
sample.pop()
sample.pop()
print("After poping 2 elements")
sample.printstack()
Output
['Welcome', 'To', 'Pythonwife', 'Website']
After poping 2 elements
['Welcome', 'To']
What is a Stack in Python?
A stack is a linear data structure that uses the Last-In/First-Out (LIFO) or First-In/Last-Out (FILO) method to store objects. A new element is added to one end of the stack, and an element is deleted from the other end.
Push and Pop are terms that describe the operations of inserting and deleting data.
sample = Stack()
sample.push(1)
sample.push(2)
sample.push(3)
sample.push(4)
size = sample.size()
print(size)
sample.printstack()
Output
4
[1, 2, 3, 4]
How to Use Stack in Python?
In a stack, the element inserted last in sequence will come out first as we can remove only from the top of the stack.
AStack = Stack()
AStack.push("Monday")
AStack.push("Tuesday")
AStack.peek()
print(AStack.peek())
AStack.push("Wednesday")
AStack.push("Thursday")
print(AStack.peek())
Output
Tuesday
Thursday
How to Use a List as a Stack in Python?
The list data structure in Python can be used as a stack.
MyStack = []
def DisplayStack():
print("Stack currently contains:")
for Item in MyStack:
print(Item)
def Push(Value):
MyStack.append(Value)
def Pop():
if len(MyStack) > 0:
MyStack.pop()
else:
print("Stack is empty.")
Push(1)
Push(2)
Push(3)
DisplayStack()
print("4 is inserted")
Push(4)
DisplayStack()
print("One Element is poped")
Pop()
DisplayStack()
print("Three Elements are poped")
Pop()
Pop()
Pop()
DisplayStack()
Output
Stack currently contains:
1
2
3
Stack currently contains:
1
2
3
4
One Element is poped
1
2
3
Three Elements are poped
Stack is empty.
Stack currently contains:
How to put a List into a Stack using Python?
Like any other variable, the List can be passed to the push()
function, to put a list into a stack.
my_stack=Stack()
my_stack.push([1,2,3])
my_stack.push(["hi","hello","welcome"])
my_stack.printstack()
Output
[[1, 2, 3], ['hi', 'hello', 'welcome']]
How to Check if a Stack is Empty or Not using Python?
The isEmpty()
function in stack class can be used to check if a stack is empty or not.
my_stack=Stack()
my_stack.printstack()
my_stack.isEmpty()
Output
stack is empty
True
Which Data Structure in Python is Used to Represent a Stack?
The stack data structure in Python can be used as a stack. The push()
is used to add elements to the top of the stack, whereas pop()
is used to remove elements in LIFO order. The size()
is used to return the number of elements in the stack.
class Stack:
def __init__(self):
self.items = []
def push(self, item):
self.items.append(item)
def pop(self):
return self.items.pop()
def size(self):
return len(self.items)
def printstack(self):
x = self.size()
if(x>0):
print(self.items)
else:
print("stack is empty")
sample = Stack()
sample.push(1)
sample.push(2)
sample.push(3)
sample.push(4)
sample.printstack()
print("size od stack is ",sample.size())
sample.pop()
sample.pop()
print("After poping 2 elements")
sample.printstack()
Output
[1, 2, 3, 4]
size od stack is 4
After poping 2 elements
[1, 2]
How to Increase your Call Stack Depth in Python?
The setrecursionlimit()
function can be used to increase the stack depth allowed.
def rec_func(i):
if (i==0):
return 0
else:
return rec_func(i-1)
def example():
try:
rec_func(10000)
except:
sys.setrecursionlimit(11000)
rec_func(10000)
print("in exception")
example()
Output
in exception
How to Define a Stack in Python?
A stack is a linear data structure that uses the Last-In/First-Out (LIFO) or First-In/Last-Out (FILO) method to store objects. A new element is added to one end of the stack, and an element is deleted from the other end.
The Push and pop are words used to describe the operations of inserting and deleting data.
class Stack:
def __init__(self):
self.items = []
def isEmpty(self):
return self.items == []
def push(self, item):
self.items.append(item)
def pop(self):
return self.items.pop()
def peek(self):
return self.items[len(self.items)-1]
def size(self):
return len(self.items)
def printstack(self):
x = self.size()
if(x>0):
print(self.items)
else:
print("stack is empty")
sample = Stack()
sample.push(1)
sample.push(2)
sample.push(3)
sample.push(4)
sample.printstack()
Output
[1, 2, 3, 4]
How to Pop a Stack in Python?
The pop()
function in the Stack class is used to remove the last inserted element in a stack.
sample = Stack()
sample.push(1)
sample.push(2)
sample.push(3)
sample.push(4)
sample.printstack()
sample.pop()
print("After poping an element")
sample.printstack()
Output
[1, 2, 3, 4]
After poping an element
[1, 2, 3]
How to Print a Stack in Python?
The elements are poped using the pop()
function and printStack()
function is recursively called to print the stack elements.
import sys
class Stack:
def __init__(self):
self.s = []
def push(self, data):
self.s.append(data)
def pop(self):
return self.s.pop()
def peek(self):
return self.s[-1]
def count(self):
return len(self.s)
def printStack(s):
if s.count() == 0:
return
x = s.peek()
s.pop()
printStack(s)
print("{} ".format(x), end = "")
s.push(x)
if __name__=='__main__':
s=Stack()
s.push(10)
s.push(20)
s.push(30)
s.push(40)
printStack(s)
Output
10 20 30 40
How to Delete All Elements of Stack using Python?
Using the while loop and size()
function we can delete all the elements of the stack.
sample = Stack()
sample.push("hi")
sample.push("hello")
sample.push(10)
sample.push(100)
print("Initial stack")
sample.printstack()
size = sample.size()
while(size>0):
sample.pop()
size = size-1
sample.printstack()
Output
Initial stack
['hi', 'hello', 10, 100]
stack is empty
How to Horizontally Stack Two Arrays using Python?
The hstack()
function is used to stack the sequence of input arrays horizontally (i.e. column wise) to create a single array.
import numpy as np
in_arr1 = np.array([ 10, 20, 30] )
print ("1st array : \n", in_arr1)
in_arr2 = np.array([ 'Pythonwife', 'Website', 'Python'] )
print ("2nd array : \n", in_arr2)
out_arr = np.hstack((in_arr1, in_arr2))
print ("Horizontally stacked array:\n ", out_arr)
Output
1st array :
[10 20 30]
2nd array :
['Pythonwife' 'Website' 'Python']
Horizontally stacked array:
['10' '20' '30' 'Pythonwife' 'Website' 'Python']
How to Check the Matching Paranthesis in Python using Stack?
The checkParanthesis()
function is used to check if the parenthesis is balanced or not.
open_list = ["[","{","("]
close_list = ["]","}",")"]
# Function to check parentheses
def checkParanthesis(myStr):
stack = []
for i in myStr:
if i in open_list:
stack.append(i)
elif i in close_list:
pos = close_list.index(i)
if ((len(stack) > 0) and
(open_list[pos] == stack[len(stack)-1])):
stack.pop()
else:
return "Unbalanced"
if len(stack) == 0:
return "Balanced"
else:
return "Unbalanced"
string = "{[]{()}}"
print(string,"-", checkParanthesis(string))
string = "[{}{})(]"
print(string,"-", checkParanthesis(string))
string = "((()))"
print(string,"-",checkParanthesis(string))
Output
{[]{()}} - Balanced
[{}{})(] - Unbalanced
((())) - Balanced
How to implement stack class with push() and pop() function in python?
The following code has the class stack with push()
and pop()
function.
class Stack:
def __init__(self):
self.items = []
def push(self, item):
self.items.append(item)
def pop(self):
return self.items.pop()
def size(self):
return len(self.items)
def printstack(self):
x = self.size()
if(x>0):
print(self.items)
else:
print("stack is empty")
sample = Stack()
sample.push("hi")
sample.push("hello")
sample.push(10)
sample.push(100)
print("Initial stack")
sample.printstack()
Output
Initial stack
['hi', 'hello', 10, 100]
How To Stack Vectors in Python?
The numpy.stack() can be used to stack the vectors together in python.
import numpy as np
in_arr1 = np.array([ 10, 42, 53] )
print ("1st Input array : \n", in_arr1)
in_arr2 = np.array([ 24, 35, 60] )
print ("2nd Input array : \n", in_arr2)
out_arr1 = np.stack((in_arr1, in_arr2), axis = 0)
print ("Output stacked array along axis 0:\n ", out_arr1)
out_arr2 = np.stack((in_arr1, in_arr2), axis = 1)
print ("Output stacked array along axis 1:\n ", out_arr2)
Output
1st Input array :
[10 42 53]
2nd Input array :
[24 35 60]
Output stacked array along axis 0:
[[10 42 53]
[24 35 60]]
Output stacked array along axis 1:
[[10 24]
[42 35]
[53 60]]
How to Get the Last Element in a Stack using Python?
The stack follows the Last in First Out procedure. So, the pop() function can be used to get the last element in a stack.
my_stack = Stack()
my_stack.push("Welcome")
my_stack.push("To")
my_stack.push("Pythonwife")
my_stack.push("Website")
print("The last element in the given stack is : ",my_stack.pop())
Output
The last element in the given stack is : Website
How to Stack Arrays of Different Lengths using Python?
The itertools library and user-defined stack_padding()
function can be used to stack the arrays of different lengths.
import itertools
a = np.array([1,2,3])
b = np.array([4,5])
l = [a,b]
def stack_padding(l):
return np.column_stack((itertools.zip_longest(*l, fillvalue=0)))
stack_padding(l)
Output
array([[1, 2, 3],
[4, 5, 0]])
How to Find Solution to Maze using Stack in Python?
The following code can be used to find a solution to the maze using a stack.
#maze.txt
#############
S # # #
# # ## # #
# # ## ### #
# # #
######### # #
# # E
#############
def load_maze(file_name):
f = open(file_name)
maze = f.read()
f.close()
return maze
def convert_maze(maze):
converted_maze = []
lines = maze.splitlines()
for line in lines:
converted_maze.append(list(line))
return converted_maze
def print_maze(maze):
for row in maze:
for item in row:
print(item, end='')
print()
def find_start(maze):
for row in range(len(maze)):
for col in range(len(maze[0])):
if maze[row][col] == 'S':
return row, col
from time import sleep
def is_valid_position(maze, pos_r, pos_c):
if pos_r < 0 or pos_c < 0:
return False
if pos_r >= len(maze) or pos_c >= len(maze[0]):
return False
if maze[pos_r][pos_c] in ' E':
return True
return False
def solve_maze(maze, start):
stack = []
stack.append(start)
while len(stack) > 0:
pos_r, pos_c = stack.pop()
print("Current position", pos_r, pos_c)
if maze[pos_r][pos_c] == 'E':
print("GOAL")
return True
if maze[pos_r][pos_c] == 'X':
continue
maze[pos_r][pos_c] = 'X'
if is_valid_position(maze, pos_r - 1, pos_c):
stack.append((pos_r - 1, pos_c))
if is_valid_position(maze, pos_r + 1, pos_c):
stack.append((pos_r + 1, pos_c))
if is_valid_position(maze, pos_r, pos_c - 1):
stack.append((pos_r, pos_c - 1))
if is_valid_position(maze, pos_r, pos_c + 1):
stack.append((pos_r, pos_c + 1))
print('Stack:' , stack)
print_maze(maze)
return False
maze = load_maze("maze.txt")
maze = convert_maze(maze)
print_maze(maze)
start = find_start(maze)
print(start)
print(solve_maze(maze, start))
Output
(1, 0)
Current position 1 0
Stack: [(1, 1)]
Current position 1 1
Stack: [(2, 1)]
Current position 2 1
Stack: [(3, 1)]
...
Current position 6 11
Stack: [(3, 3), (3, 4), (1, 7), (6, 12)]
Current position 6 12
GOAL
True
How to Make a Stack Empty using Deque in Python?
The deque()
from the collections and pop()
can be used to make the stack empty.
from collections import deque
stack = deque()
stack.append('12a')
stack.append('b23')
stack.append('c')
print('Initial stack:')
print(stack)
print('\nElements poped from stack:')
print(stack.pop())
print(stack.pop())
print(stack.pop())
print('\nStack after elements are poped:')
print(stack)
Output
Initial stack:
deque(['12a', 'b23', 'c'])
Elements poped from stack:
c
b23
12a
Stack after elements are poped:
deque([])
How to Check if Type is Class Stack in Python?
The type()
function can be used to check if the type of class is a stack.
class Stack:
def __init__(self):
self.list = []
def push(self, value):
self.list.append(value)
return "The element has been successfully added to the stack."
#Example
tempStack = Stack()
tempStack.push(1)
tempStack.push(2)
tempStack.push(3)
print("The type of is ", type(tempStack))
Output
The type of is <class '__main__.Stack'>
How to Stack Arrays of Different Dimension using Python?
The shape
and reshape
in NumPy library can be used to stack arrays of different dimensions.
arr = np.array(range(10)).reshape((5,2))
print(arr)
arr_p1 = np.zeros(arr[0:3].shape)
arr_p1_src = arr[0:2]
arr_p1[:arr_p1_src.shape[0],:arr_p1_src.shape[1]] = arr_p1_src
t2 = np.array([arr_p1, arr[0:3]])
print(t2)
Output
[[0 1]
[2 3]
[4 5]
[6 7]
[8 9]]
[[[0. 1.]
[2. 3.]
[0. 0.]]
[[0. 1.]
[2. 3.]
[4. 5.]]]
How to Check for Maximum Element in a Stack using Python?
The user-defined getmax()
function in the StackWithMax class can be used to find the maximum element in a stack.
class StackWithMax:
def __init__(self):
self.mainStack = []
self.trackStack = []
def push(self, x):
self.mainStack.append(x)
if (len(self.mainStack) == 1):
self.trackStack.append(x)
return
if (x > self.trackStack[-1]):
self.trackStack.append(x)
else:
self.trackStack.append(self.trackStack[-1])
def getMax(self):
return self.trackStack[-1]
if __name__ == '__main__':
s = StackWithMax()
s.push(20)
s.push(200)
s.push(10)
s.push(290)
s.push(50)
print("The maximum element in the stack is",s.getMax())
Output
The maximum element in the stack is 290
How to Pop All Elements of the Stack in Python?
The pop()
function can be used to pop all the elements until the stack becomes empty.
sample = Stack()
sample.push("hi")
sample.push("hello")
sample.push(10)
sample.push(100)
print("Initial stack")
sample.printstack()
size = sample.size()
while(size>0):
sample.pop()
size = size-1
sample.printstack()
Output
Initial stack
['hi', 'hello', 10, 100]
stack is empty
How to Implement a Stack and Queue in Python?
The Queue class contains add_element()
and remove_element()
functions to add and remove elements from the queue. The Stack class contains push()
and pop()
functions to add and remove elements from the queue.
print("Queue implementation")
class Queue:
def __init__(self):
self.queue = list()
def add_element(self,val):
if val not in self.queue:
self.queue.insert(0,val)
return True
return False
def size(self):
return len(self.queue)
def printqueue(self):
x = self.size()
print(x)
while(x>0):
print(self.queue.pop())
x=x-1
def remove_element(self):
if len(self.queue)>0:
self.queue.pop()
return ("Queue is Empty")
TheQueue = Queue()
TheQueue.add_element("Apple")
TheQueue.add_element("Mango")
TheQueue.add_element("Guava")
TheQueue.add_element("Papaya")
TheQueue.remove_element()
TheQueue.remove_element()
print("The length of Queue: ",TheQueue.size())
TheQueue.printqueue()
print("Stack implementation")
class Stack:
def __init__(self):
self.items = []
def isEmpty(self):
return self.items == []
def push(self, item):
self.items.append(item)
def pop(self):
return self.items.pop()
def peek(self):
return self.items[len(self.items)-1]
def size(self):
return len(self.items)
def printstack(self):
x = self.size()
if(x>0):
print(self.items)
else:
print("stack is empty")
sample = Stack()
sample.push(1)
sample.push(2)
sample.push(3)
sample.push(4)
size = sample.size()
print("The length of stack : ",size)
sample.printstack()
Output
Queue implementation
The length of Queue: 2
2
Guava
Papaya
Stack implementation
The length of stack : 4
[1, 2, 3, 4]
What Happens If we Pop a Empty Stack in Python?
Poping an empty stack will raise an index error.
sample = Stack()
sample.push(1)
sample.push(2)
sample.push(3)
size = sample.size()
print(size)
sample.pop()
sample.pop()
sample.pop()
sample.pop()
sample.printstack()
Output
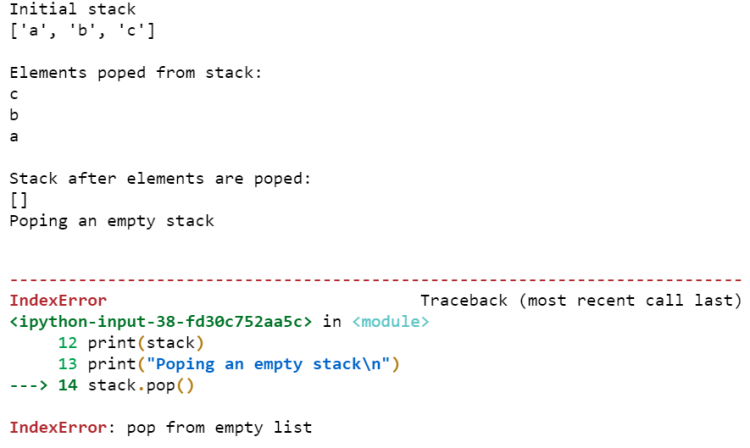
how to get top element of stack in python
The peek()
function can be used to get the top element in a stack.
def peek(self):
if self.isEmpty():
raise Exception("Peeking from an empty stack")
return self.head.next.value
if __name__ == "__main__":
stack = Stack()
for i in range(1, 11):
stack.push(i)
print(f"Stack: {stack}")
print(f"Stack: {stack}")
print(f"Stack top: ",stack.peek())
Output
Stack: 10 -> 9 -> 8 -> 7 -> 6 -> 5 -> 4 -> 3 -> 2 -> 1
Stack top : 1