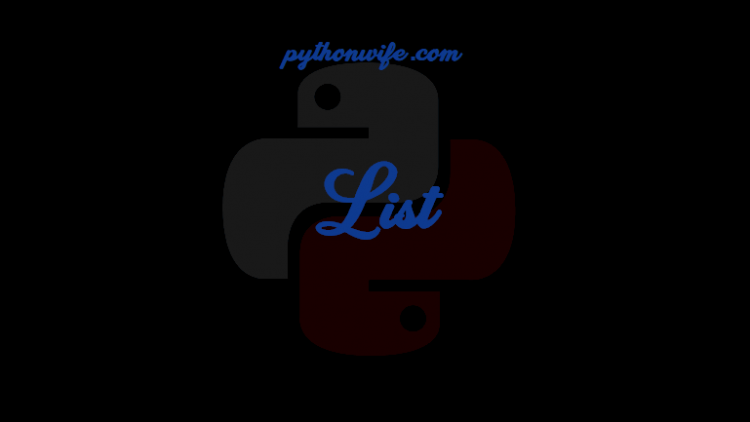
A list is an inbuilt data structure in python, that stores a sequence of data items. A list can hold elements with different data types.
Properties of the list:
- Ordered: The items in the list are indexed. We can access the element from the list using the index values.
- Mutable: The list is changeable, i.e. we can add or remove the items from a list.
- Allow duplicates: We can store duplicate elements in the list.
Creating a list
Python lists can be created by placing all the data items inside the square brackets separated by commas.
#creating an empty list
list_empty = []
print(list_empty)
#Output: []
#creating a list of integer
list_int = [1, 5, 4, 10, 4]
print(list_int)
#Output: [1, 5, 4, 10, 4]
#creating a list of mixed-data type
list_mixed = [1, 1.8, 'red']
print(list_mixed)
#Output: [1, 1.8, 'red']
#creating nested list
list_nested = [1, 2, [3,6]]
print(list_nested)
#Output: [1, 2, [3, 6]]
Time and space complexity of creating a list
The time complexity of creating a list is O(1) as we are just assigning a list of values to a variable, and the space complexity of creating a list is O(N), where “N” represents the length of the list.
Accessing an element in a List
We can access an element from a list using the index [] operator. The index value starts at 0. So, for a list having 5 data items, the index value ranges from 0 to 4.

The syntax to access an element from a list is as follows.
#syntax:
list_name[index_val]
The index_val must be an integer. If the index_val is greater than or equal to the length of the list, the code raises an IndexError exception.
Example,
#creating a list of mixed-data type
my_list = [1, 1.8, 'red']
#Accessing the 0th index element
print(my_list[0])
#Output: 1
#Accessing the 2nd index element
print(my_list[2])
#Output: 'red'
Python also allows negative indexing. The negative index values represent the data items from the end of the list. For example, -1 represents the last data item, -2 represents the second last data item, etc.

Example,
#creating a list of mixed-data type
my_list = [1, 1.8, 'red']
#Accessing the last element
print(my_list[-1])
#Output: 'red'
#Accessing the 2nd last element
print(my_list[-2])
#Output: 1.8
Time and space complexity of accessing an element
The time complexity to access an element in a list is constant, i.e. O(1) and the space complexity is also O(1) as we are not using any additional space to access an element.
Traversing a List
We can use a for loop to traverse through each element of the list. In the following example, a for loop iterates through each element of the list_1, and prints the element in each iteration.
#creating a list of mixed-data type
my_list = [1, 1.8, 'red', -6, -7.8]
#Traversing through list_1
for ele in my_list:
print(ele)
The above code returns the output as
1
1.8
red
-6
-7.8
Time and space complexity to traverse a list
The time complexity of the above code is linear, i.e O(N) as the for loop traverses each element of the list and the space complexity is O(1) because we are not using any extra space for traversing.
Updating the elements in a List
To update the element in a list first, we access the item using the index operator [], and then assign it with the new updated value.
Example,
#creating a list
my_list = [1, 1.8, 'red', -6, -7.8]
print("Original list: ", my_list)
#Changing the value of 1st index item to 'black'
my_list[1] = 'black'
#Changing the value of 3rd index item to 18
my_list[3] = 18
print("Updated list: ", my_list)
Output:
Original list: [1, 1.8, 'red', -6, -7.8]
Updated list: [1, 'black', 'red', 18, -7.8]
Time and space complexity to update element
The time complexity to update an element in the list is O(1) and the space complexity is also O(1) as extra memory space is not required.
Inserting an element in a List
There are different ways to insert an element in a list.
- Inserting an element at the beginning of the list.
- Inserting an element to any given place in the list.
- Inserting an element at the end of the list.
- Inserting elements of another iterable into a list.
In python, we have various inbuilt functions such as insert(), append(), and extend() to perform the insertion operation in a list.
insert()
The insert() method inserts an item at a specified position in the list. The syntax for the insert() function is as follows.
#syntax:
list_name.insert(index_val, element)
Parameters:
- index_val: The index or the position, where the item will be inserted.
- element: The new item that will be added to the list.
Example,
#creating a list
my_list = [1, 'red', 7.8, 4]
#Inserting an item at index 2
my_list.insert(2, 'green')
print(my_list)
#Output: [1, 'red', 'green', 7.8, 4]
Time and space complexity of insert()
The time complexity of the insert() function depends on the position in which we insert an element. For example, inserting an element at the beginning of a list i.e. at the 0th index will shift each element to the right by one index. Hence, the time complexity will be O(N) where N is the number of elements.
The space complexity for the insert() function is O(1) because we are allocating just one extra space for the insertion of an element.
append()
The append() adds an item at the end of the list. This method takes a single argument and the argument can be a number, list, string, etc.
The syntax for the append() function is as follows.
#syntax:
list_name.append(item)
Example,
#creating a list
my_list = [1, 'red', 7.8, 4]
#Adding an item at the end of the list
my_list.append('black')
print(my_list)
#Output: [1, 'red', 7.8, 4, 'black']
Time and space complexity of append()
The time complexity of the append() function is O(1) as it takes constant time to add an element at the end of the list and the space complexity is also O(1) as it takes constant space.
extend()
The extend() method adds all the items of an iterable(list, tuple, string, etc.) to the end of the list. The syntax for the extend() method is as follows.
#syntax:
list_name.extend(iterable)
Example,
#creating a list
my_list = [1, 'red', 7.8, 4]
#Adding the items of a list iterable to my_list
my_list.extend(['black', 9.76])
print(my_list)
#Output: [1, 'red', 7.8, 4, 'black', 9.76]
Time and space complexity of extend()
The time complexity of extend() method is O(N), where N is the length of the iterable. The space complexity is also O(N), as we are allocating ‘N’ extra spaces in the list.
Slicing a List
List slicing refers to accessing a subset or portion of the list. We can use the indexing syntax to slice a list. The syntax for slicing a list is given below.
#syntax:
list_name[start: end: step]
Parameters:
- start: The starting index value where the slicing of an object starts. By default, the value of start is 0.
- end: The ending index value until where the slicing takes place. The slicing stops at index end -1. By default, the value of the end parameter is the length of the object(list, string, tuple).
- step: The step value determines the increment between each index for slicing. By default, the value of the step is 1.
Example,
list_1 = [1, 5, "A", "red", 7, 8]
#start = 1, end = 4, step = 1
print(list_1[1:4])
#Output: [5, 'A', 'red']
list_2 = ['a', 4, 'abc', 4.56, 'A', 6]
#start = 1, end = 5, step = 2
print(list_2[1:5:2])
#Output: [4, 4.56]
Deleting an element from a List
We can use various inbuilt functions such as pop(), remove(), or a delete statement to remove an element from a list.
pop()
The pop() function removes the item at a specified index. The pop() function takes the index value of the list as a parameter and returns the value of the removed item. By default, the pop() function takes -1 index as an argument, i.e. the pop() function removes the last element of the list if the index value is not provided.
The syntax for the pop() function is as follows.
#syntax:
list_name.pop(index_val)
Example,
#creating a list
my_list = [1, 'red', 7.8, 4]
print("Original list: ", my_list)
#Removing a item at 1st index using pop()
val = my_list.pop(1)
print("The removed item is: ", val)
print("Updated list: ", my_list)
The above code returns the output as
Original list: [1, 'red', 7.8, 4]
The removed item is: red
Updated list: [1, 7.8, 4]
Time and space complexity of pop()
The time complexity of the pop() function depends on the item we are removing.
For example, deleting an element from the beginning of a list i.e. at the 0th index will shift each element to the left by one index. Hence, the time complexity will be O(N) where N is the number of elements.
But, deleting an element from the end of the list will just take constant time, i.e O(1) because we are not moving any element to the right or left.
The space complexity of the pop() function is O(1) because we are not using any extra memory to perform the deletion.
remove()
The remove() function deletes the first occurrence of the specified element from a list. The syntax for the remove() function is as follows.
#syntax:
list_name.remove(element)
Example,
#creating a list
my_list = [1, 'red', 7.8, 4, 3, 4]
#Removing the element '4' using remove()
my_list.remove(4)
print(my_list)
#Output: [1, 'red', 7.8, 3, 4]
Time and space complexity of remove()
The time complexity of the remove() function is O(N). For example, if we remove an item, and it is present at the 0th index, all the remaining elements will be shifted to the left by one position, which takes linear time complexity.
The space complexity for the remove() function is O(1) as we are not using additional space to remove an item from a list.
del statement
The del statement removes an item from a specific index. The syntax for using the del statement to remove an item is as follows.
#syntax:
del list_name[index_val]
Example,
#creating a list
my_list = [1, 'red', 7.8, 4, 3, 4]
#Removing the item at index 1 using del
del my_list [1]
print(my_list)
#Output: [1, 7.8, 4, 3, 4]
We can also delete more than one element from a list by using slicing with the delete statement.
Example,
#creating a list
my_list = [1, 'red', 7.8, 4, 3, 4]
#Removing the item from index 1 to index 3
del my_list [1: 4]
print(my_list)
#Output: [1, 3, 4]
Time and space complexity of del statement
The time complexity of using the del statement is O(N) because when we are removing an item or multiple items, the remaining items are shifted to the left by 1 index.
The space complexity is O(1) because we are not using extra space to remove the items from a list.
Searching an element in a list
We can check the existence of an element in a list by using the following method.
- in operator
- Linear search
“in” operator
The “in” operator checks if an element is present in a list and returns a boolean value. The “in” operator returns True if the item is found in the list. Otherwise, it returns False.
Consider a list my_list = [“bat”, 3, “apple”, “red”, 4.8]. In the following example, we check the existence of the element ‘red’ in my_list. If the item is found in the my_list, the program prints “Item Found”. Otherwise, it prints the statement “Not Found” as output.
my_list = ["bat", 3, "apple", "red", 4.8]
if 'red' in my_list:
print("Item Found")
else:
print("Not Found")
#Output: Item Found
Time and space complexity of the “in” operator
The time complexity of using the “in” operator is linear, i.e. O(N) because the in operator checks all the elements one by one in the list.
The space complexity of the “in” operator is O(1) because no extra space is required to check the existence of an element.
Linear search
In the following example, we use the linear search algorithm to search for an element in the list. We use a for loop to iterate over all the elements in the list. The program compares if the element at a specified index in the list is equal to the key element in each iteration.
If the element is found in the list, the index value is returned as output. Otherwise, the program returns the statement “Element not found” as output.
def search_ele(list_1, key):
for i in range(len(list_1)):
if list_1[i] == key:
return i # returning the index val
return "Element not found"
#creating a list
my_list = ["bat", 3, "apple", "red", 4.8]
#searching for element 'apple' in my_list
print(search_ele(my_list, 'apple'))
#Output: 2
#searching for an element 15 in my_list
print(search_ele(my_list, 15))
#Output: "Element not found"
Time and space complexity of linear search
The time complexity to search for an element in a list using the linear search algorithm is O(N) and the space complexity is O(1).
List Operations
We can use the ‘+’ operator to concatenate lists and the ‘*’ operator to repeat the elements in a list.
List concatenation using the ‘+’ operator
The ‘+’ operator concatenates or combines two or more lists.
For example, let us consider list_1 = [1, 2, ‘red’] and list_2 = [4, 5]. Concatenating list_1 and list_2 using ‘+’ operator returns the output as [1, 2, ‘red’, 4, 5].
#creating list_1
list_1 = [1, 2, 'red']
#creating list_2
list_2 = [4, 5]
#concatenating list_1 and list_2
list_3 = list_1 + list_2
print(list_3)
#Output: [1, 2, 'red', 4, 5]
Repeating list elements using the ‘*’ operator
The ‘*’ operator repeats the element in a list a specified number of times.
For example, consider a list my_list = [1, 2]. The statement my_list * 3 repeats the element in my_list three times and gives the output as [1, 2, 1, 2, 1, 2].
#creating a list
my_list = [1, 2]
#Multiplying my_list with 3
print(my_list * 3)
#Output: [1, 2, 1, 2, 1, 2]
List functions
len()
The len() function returns the total length of the list. The syntax for the len() function is as follows.
#syntax:
len(list_name)
Example,
#creating a list
my_list = [1, 6, 3, 7, 8]
#Getting the length of the list
print(len(my_list))
#Output: 5
max() and min()
The max() function returns the maximum value in the list, and the min() function returns the minimum value in the list. The syntax for max() and min() function is as follows.
#syntax:
max(list_name) #returns maximum value
min(list_name) #returns minimum value
Example,
#creating a list
my_list = [1, 6, 3, 7, 8]
#Getting the maximum value from the list
print(max(my_list))
#Output: 8
#Getting the minimum value from the list
print(min(my_list))
#Output: 1
sum()
The sum() function calculates the sum of all the elements in a list. The syntax for the sum() function is as follows.
#syntax
sum(list_name)
Example,
#creating a list
my_list = [1, 6, 3, 7, 8]
#Getting the sum of all elements
print(sum(my_list))
#Output: 25
clear()
The clear() function removes all the items and returns an empty list. The syntax for using the clear() function is as follows.
#syntax:
list_name.clear()
Example,
#creating a list
my_list = [1, 6, 3, 7, 8]
#clearing my_list
my_list.clear()
print(my_list)
#Output: []
count()
The count() function returns the number of occurrences of a specified element in the list. The syntax for the count() function is as follows.
#syntax:
list_name.count(element)
Example,
#creating a list
my_list = [1, 6, 3, 7, 6, 8, 6]
#Getting the count of element 6
val= my_list.count(6)
print(val)
#Output: 3
sort()
The sort() function sorts the original list in ascending order. The two optional parameters for the sort() function are key and reverse.
The syntax for the sort() function is as follows.
#syntax:
list_name.sort(key, reverse)
- The parameter reverse takes a boolean value. If the reverse is set to True, the sort() function sorts the list in descending order.
- The parameter key takes a function as a value for user-defined comparison.
Example,
#creating a list
my_list = [1, 6, 3, 7, 6, 8, 6]
#sorting my_list in ascending order
my_list.sort()
print(my_list)
#Output: [1, 3, 6, 6, 6, 7, 8]
reverse()
The reverse() returns the list in reverse order. The syntax for the reverse() function is as follows.
#syntax:
list_name.reverse()
Example,
#creating a list
my_list = [1, 6, 3, 7, 6, 8, 6]
#sorting my_list
my_list.reverse()
print(my_list)
#Output: [6, 8, 6, 7, 3, 6, 1]
list()
The list() constructor converts a sequence(tuple, set, strings, etc.) to the list data type.
In the following example, we converted a string and a tuple to the list data type.
#converting a string to list data type
string = "abcde"
my_list = list(string)
print(my_list)
#Output: ['a', 'b', 'c', 'd', 'e']
#converting a tuple to list data type
my_tuple = (4, 7, 8)
my_list = list(my_tuple)
print(my_list)
#Output: [4, 7, 8]
Lists vs Arrays
Similarities
- The items in both lists and arrays are indexed.
- List and array both are mutable.
- We can slice both.
- We can iterate through both data structures.
Differences
List | Array |
We don’t need to explicitly import a module to create a list. | We need to import a numpy or array module to create an array |
A list can contain elements of different data types. | An array contains elements of the same data type. |
We cannot perform arithmetic operations with a list. For example, consider list_1 = [2, 4, 8]. Performing the operation list_1/2 will raise an error. | An array can handle arithmetic operations. For example, consider an array arr_1 = numpy.array(‘2, 4, 8]). The statement arr_1/2 will give output as [1. 2. 4.]. |
A list consumes a large amount of memory as compared to an array. | An array is more efficient, as it stores the elements in a more compact way. |
A list is preferred for shorter sequences of data items. | An array is preferred for longer sequences of data items. |
List Interview Questions
Missing Number
Given a list of N-1 integers in the range of 1 to N with no duplicates write a program to find the missing number in the list.
Example,
Input = [1, 4, 3, 5]
Output: 2
The length of the list is N – 1. We know that, the sum of N natural numbers, i.e. from 1 to N = N*(N+1) // 2. Now, to get the missing number we subtract the sum of the first N natural numbers with the sum of the elements in the list.
def missing_num(my_list):
N = len(my_list) + 1
#sum of N natural numbers
sum_1 = N*(N+1)//2
#sum of elements in the list
sum_2 = sum(my_list)
#return missing number
return sum_1 - sum_2
list_1 = [1, 4, 3, 5]
print("Missing number in list_1: ", missing_num(list_1))
#Output: Missing number in list_1: 2
list_2 = [1, 7, 8, 2, 3 , 5, 6]
print("Missing number is list_2: ", missing_num(list_2))
#Output: Missing number in list_1: 4
Sum of two values Problem
Write a program to find all the distinct pairs of integers in a list whose, the sum is equal to a given target number.
Example,
Input:
list = [1, 3, 6, 2, 9, 4], target = 5
Output: (1, 4), (3, 2)
Explanation: The sum of 1 + 4 = 5 and 3 + 2 = 5
We use the two nested for loops. The first for loop traverse through all the elements of the list. The second nested for loop traverses through the remaining elements to check if the number can be added to get the given target number.
If both the elements are the same, we continue to the next iteration. Otherwise, if the sum of the elements is equal to the given target number, we print the numbers as output.
def sum(my_list, target):
for i in range(len(my_list)):
for j in range(i + 1, len(my_list)):
if my_list[i] == my_list[j]:
continue
elif my_list[i] + my_list[j] == target:
print(my_list[i], my_list[j])
list_1 = [1, 4, 6, 8, 3, 5, 7, 2]
target = 9
sum(list_1, target)
Output:
1 8
4 5
6 3
7 2
Maximum product of a pair in a list
Given a list of positive integers, write a program to find the pair with the maximum product.
In the following program, we declare a user-defined function maxProduct to get the pair with maximum product in a list. A variable maxim is initialized to 0, to store the maximum product value.
We use the two nested for loops. The first loop traverses through all the elements of the list. The second nested for loop traverses through the remaining elements to check if the product of the two pairs is greater than maxim.
def maxProduct(my_list):
maxim = 0
for i in range(len(my_list)):
for j in range(i + 1, len(my_list)):
if my_list[i]*my_list[j] > maxim:
maxim = my_list[i]*my_list[j]
pairs = str(my_list[i]) + ", " + str(my_list[j])
print("Pairs: ", pairs)
print("Maximum Product: ", maxim)
list_1 = [1, 4, 6, 8, 3, 5, 7, 2]
maxProduct(list_1)
Output:
Pairs: 8, 7
Maximum Product: 56
Is Unique
Given a list of numbers, write a program to check if the list contains all the unique elements or not.
Example,
Input 1: [1, 7, 5, 6, 8]
Output: Unique
Input 2: [1, 7, 7, 6, 5, 8]
Output: Not Unique
In the following code, we declare a user-defined function isUnique to check if the list is unique. We initialize the variable visited = [].
A for loop iterates through the my_list. In each iteration, we check if the element in the my_list is present in the visited list. If the condition is True, the element is a duplicate element. Hence the list is not unique. If the element is not present in the visited list, we add the element to the visited list by using the append() method.
def isUnique(my_list):
visited = []
for i in my_list:
if i in visited:
return "Not Unique"
else:
visited.append(i)
return "Unique"
list_1 = [1, 1, 2, 8, 9, 4, 4]
print(isUnique(list_1))
#Output: Not Unique
list_2 = [1, 4, 6, 8, 3]
print(isUnique(list_2))
#Output: Unique
Permutation
Write a program to check if two lists are permutations of each other.
Example,
Input:
list_1 = [1, 4, 5, 7]
list_2 = [4, 1, 7, 5]
Output : True
We declare a user-defined function isPermutation to check if the two lists are a permutation of each other, i.e. the list must have the same elements, but the elements can be in a different order.
If the length of both lists is not equal, then we return False. If the length of the lists is equal, we sort both the list using the sort() function. Now, we compare both the list using the ‘==’ operator to check if both the list contain the same elements. If the condition is True, we return True. Otherwise, we return False as output.
def isPermutation(list_1, list_2):
if len(list_1) != len(list_2):
return False
list_1.sort()
list_2.sort()
if list_1 == list_2:
return True
else:
return False
list_1 = [1, 8, 3, 4, 6]
list_2 = [1, 4, 6, 8, 3]
print(isPermutation(list_1, list_2))
#Output: True
Average Temperature
Write a program to find the number of days having a temperature greater than the average temperature.
In the following program, we take the user input for the number of days we want to calculate the average temperature and store it in the variable num_days.
We initialize the variable list_temp with an empty list to store the temperature of each day and initialize another variable count with 0 to store the count of the days having temperatures greater than average temperature.
A for loop iterates for the range of 0 to num_days and takes the temperature of each day as input and store it in the variable temp. In each iteration, the temperature temp is appended to the list list_temp. The sum(list_temp)/ num_days gives the average temperature. The average temperature is stored in the variable avg_temp.
Now, to calculate the number of days having a temperature greater than the average temperature, we iterate through the list_temp using a for loop. In each iteration, we check if the temperature is greater than avg_temp. If the condition is True, we increment the variable count by 1.
num_days = int(input("How many day's temperature? "))
#To store temperature of each day
list_temp = []
#To get the count of days having temperature > avg temp
count = 0
for i in range(num_days):
temp = int(input("Day" +str(i+1) + " temperature: "))
list_temp.append(temp)
avg_temp = sum(list_temp) / num_days
print("Average temperature: ", avg_temp)
for i in list_temp:
if i > avg_temp:
count += 1
print("Days with temperature above the average temperature: ", count)
Running the above code returns the output as
How many day's temperature? 4
Day1 temperature: 19
Day2 temperature: 20
Day3 temperature: 21
Day4 temperature: 22
Average temperature: 20.5
Days with temperature above the average temperature: 2
Middle function
Write a program that takes a list and returns a new list that contains all the elements except the first and last elements.
Example,
Input: [1, 6, 7, 8, 4]
Output: [6, 7, 8]
We use the slice operation to get a portion of the list. The list[1: len(list) -1] returns a new list without the first and last elements.
def middle(my_list):
return my_list[1: len(my_list) - 1]
list_1 = [1, 5, 3, 8, 2]
print(middle(list_1))
#Output: [5, 3, 8]
list_2 = [1, 3, 9, 0, 1, 2, 3]
print(middle(list_2))
#Output: [3, 9, 0, 1, 2]
2D list
Given a square matrix, write a program to calculate the sum of diagonal elements.
Example,
Input:
[[1, 4, 6],
[7, 8, 9],
[10, 4, 6]]
Output: 13
Explanation: The sum of diagonal elements is 1 + 8 + 6 = 15
We declare a user-defined function sumDiagonal that takes a list my_list as a parameter and calculates the sum of diagonal elements. We initialize another variable total with 0, to store the sum of diagonal elements.
The len(my_list) gives the number of rows. We use a for loop to iterate over each row. In each iteration, we added the diagonal element my_list[i][i] to the variable total.
def sumDiagonal(my_list):
total = 0
for i in range(len(my_list)):
total += my_list[i][i]
return total
list_1 = [[1, 4, 6], [7, 8, 9], [10, 4, 6]]
print(sumDiagonal(list_1))
#Output: 15
Best Score
Given a list of scores, write a program to get the first and second-best scores.
Example,
Input: [60, 78, 90, 87]
Output: First = 90
Second = 87
To get the first and second-best scores, we sort the list in descending order using the sort(reverse = True) function and return the first two maximum elements.
def BestScore(my_list):
my_list.sort(reverse = True)
print("First : ", my_list[0])
print("Second: ", my_list[1])
list_1 = [60, 78, 90, 87]
BestScore(list_1)
Output:
First: 90
Second: 87
Remove duplicates
Given a list, write a program to remove the duplicate elements from a list.
Example,
Input: [1, 5, 6, 5, 1, 2, 3]
Output: [1, 5, 6, 2, 3]
In the following example, We initialize a variable unique_list with an empty list. A for loop iterates over the my_list. In each iteration, the program checks if the element is not present in the unique_list. If the condition is True, the element is added to the unique_list using the append() function.
The unique_list contains the elements without any duplicate values.
def removeDuplicates(my_list):
unique_list =[]
for i in my_list:
if i not in unique_list:
unique_list.append(i)
return unique_list
list_1 = [1, 5, 6, 5, 1, 2, 3]
print(removeDuplicates(list_1))
#Output: [1, 5, 6, 2, 3]
list_2 = [1, 1, 4, 6, 4]
print(removeDuplicates(list_2))
#Output: [1, 4, 6]
Rotate a Matrix by 90
Write a program to rotate a matrix by 90 degrees in the clockwise direction.
Example,
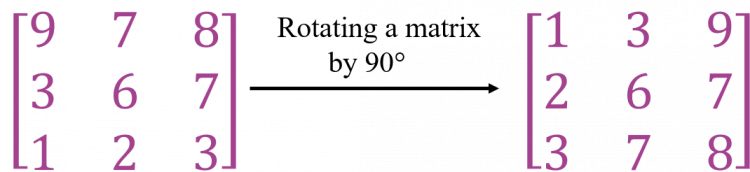
Approach:
- Step 1: Transpose the matrix
- Step 2: Reverse each row of the matrix

We can obtain the transpose of a matrix by interchanging the rows and columns of the matrix. If the order of the matrix is r x c, the transpose operation of the matrix changes the order to c x r. Here, ‘c’ represents the number of columns and ‘r’ represents the number of rows.
In the following program, for a matrix_A of order r x c, we declare another matrix_B of order c x r initialized with 0. To obtain the transpose of the matrix, we use nested for loop to traverse through each row and each column. In each iteration, the element at the ith row and jth column of matrix_A are placed at jth row and ith column of matrix_B.
In the next step, we again use the two nested for loop to reverse each row of the matrix.
def rotated(matrix_A):
print("matrix_A: ", matrix_A)
#Initializing matrix_B with 0
matrix_B = [[0 for i in range(len(matrix_A))]
for j in range(len(matrix_A[0]))]
#Transpose of matrix
for i in range(len(matrix_A)):
for j in range(len(matrix_A[0])):
matrix_B[j][i] = matrix_A[i][j]
print("Transpose of matrix_A: ", matrix_B)
#Reversing each row of matrix_B
M = len(matrix_B)#Number of rows
N = len(matrix_B)#Number of columns
for i in range(M):
for j in range(N//2):
#reversing each row
matrix_B[i][j], matrix_B[i][N-j-1] = matrix_B[i][N-j-1], matrix_B[i][j]
print("Rotating matrix_A by 90 degree: ", matrix_B)
matrix_A =[[9, 7, 8],
[3, 6, 7],
[1, 2, 3]]
rotated(matrix_A)
The above code returns the output as
matrix_A: [[9, 7, 8], [3, 6, 7], [1, 2, 3]]
Transpose of matrix_A: [[9, 3, 1], [7, 6, 2], [8, 7, 3]]
Rotating matrix_A by 90 degree: [[1, 3, 9], [2, 6, 7], [3, 7, 8]]
FAQ Question
How to Reverse a List in Python?
The reverse()
method is used to reverse the elements in a list. This method does not return any value, instead, it updates the existing list. In the below code, the reverse()
method reverses the list of string elements.
string = ['Technology', 'Knowledge', 'Advancement']
print('Original List:', string)
string.reverse()
print('Updated List:', string)
Output
Original List: ['Technology', 'Knowledge', 'Advancement']
Updated List: ['Advancement', 'Knowledge', 'Technology']
How to Make a List in Python?
A list is generated in Python programming by putting all the items (elements) inside square brackets [] and separating them with commas. It can include an unlimited number of elements of various types (integer, float, string, etc…).
The below code shows various types of lists.
# empty list
emp_list = []
# list of integers
int_list = [1, 2, 3]
# list of strings
str_list = ["Hello","world","welcome"]
#list with mixed data types
mix_list = [1, "Hello", 3.4]
#nested list
nest_list = ["Programming", [8, 4, 6], ['a']]
print(emp_list)
print(int_list)
print(str_list)
print(mix_list)
print(nest_list)
Output
[]
[1, 2, 3]
['Hello', 'world', 'welcome']
[1, 'Hello', 3.4]
['Programming', [8, 4, 6], ['a']]
How to Append to a List in Python?
The append()
method is used to add elements to the end of the list. This method takes an item to be added at the end of the list as a parameter. In the below code, an integer element is appended to the end of the list of strings.
string = ['cat', 'dog', 'rabbit']
string.append(456)
print('List after appending: ', string)
Output
List after appending: ['cat', 'dog', 'rabbit', 456]
How to Sort a List in Python?
The sort()
method can be used to sort the elements in a list. In the below code, the reverse parameter is passed to the sort()
method, which determines to sort the list in either ascending or descending order. This method will sort and modify the existing list.
my_list = [9,8,9,4,6,6,90,98]
#sorts the list in descending order
my_list.sort(reverse=True)
print('Sorted list in Descending order:', my_list)
my_list.sort(reverse=False)
print('Sorted list in Ascending order:', my_list)
Output
Sorted list in Descending order: [98, 90, 9, 9, 8, 6, 6, 4]
Sorted list in Ascending order: [4, 6, 6, 8, 9, 9, 90, 98]
How to Add to a List in Python?
The insert()
method can be used to add elements to the list at the specified index. The extend()
method is used to add another list to the end of the existing list.
fruits = ["apple", "banana", "cherry"]
fruits.insert(1, "orange")
print("The list after inserting at specified index")
print(fruits)
veggie = ["Tomato","potato","carrot"]
fruits.extend(veggie)
print("The list after extending it with another list")
print(fruits)
Output
The list after inserting at specified index
['apple', 'orange', 'banana', 'cherry']
The list after extending it with another list
['apple', 'orange', 'banana', 'cherry', 'Tomato', 'potato', 'carrot']
How to Remove an Item from a List in Python?
The del statement is used to delete the item ‘banana’ from the list, by specifying the index of the item to be deleted. And remove()
method removes the item ‘cherry’ from the list by passing the value of the item to be deleted as the parameter.
lst = ['apple', 'orange', 'banana', 'cherry', 'Tomato', 'potato', 'carrot']
print("Original List is :", lst)
lst.remove('cherry')
print("After deleting the item using remove :", lst)
del lst[2]
print("After deleting the item using del :", lst)
Output
Original List is : ['apple', 'orange', 'banana', 'cherry', 'Tomato', 'potato', 'carrot']
After deleting the item using remove : ['apple', 'orange', 'banana', 'Tomato', 'potato', 'carrot']
After deleting the item using del : ['apple', 'orange', 'Tomato', 'potato', 'carrot']
How to Copy a List in Python?
The copy()
method copies a list to another list.
old_list = ['cat', 0, 6.7]
new_list = old_list.copy()
print('Copied List is:', new_list)
Output
Copied List is: ['cat', 0, 6.7]
How to Add Items to a List in Python?
insert()
, append()
, extend()
and list concatenation are used to add items to the list. The insert()
method adds elements at the specified index. The append()
method adds elements at the end of the list.
The extend()
method and list concatenation (done using + operator) adds another list to the end of the existing list.
fruits = ["apple", "banana", "cherry"]
fruits.insert(1, "orange")
print("The list after inserting at specified index")
print(fruits)
veggie = ["Tomato","potato","carrot"]
fruits.extend(veggie)
print("The list after extending it with another list")
print(fruits)
fruits.append("strawberry")
print("The list after appending it with another item")
print(fruits)
new_list = ["hello","world"]
result = fruits + new_list
print("The list after performing list concatenation")
print(result)
Output
The list after inserting at specified index
['apple', 'orange', 'banana', 'cherry']
The list after extending it with another list
['apple', 'orange', 'banana', 'cherry', 'Tomato', 'potato', 'carrot']
The list after appending it with another item
['apple', 'orange', 'banana', 'cherry', 'Tomato', 'potato', 'carrot', 'strawberry']
The list after performing list concatenation
['apple', 'orange', 'banana', 'cherry', 'Tomato', 'potato', 'carrot', 'strawberry', 'hello', 'world']
How to Turn a List into a String Python?
- We iterate through the list and add each element at every index in some empty string.
- The
join()
method is also used to convert a list to a string.
def listToString(s):
string = ""
for ele in s:
string += ele
return string
s = ['Python', 'wife', 'website']
print(listToString(s))
def listToStringusingjoin(s):
str1 = " "
return (str1.join(s))
s = ['Hello', 'all', 'Welcome']
print(listToStringusingjoin(s))
Output
Python wife website
Hello all Welcome
How to Iterate through a List in Python?
There are different ways to iterate through lists. In the below code, we use for loop, while loop, list comprehension, and enumerate to iterate through List.
list = [1, 3, 5, 7, 9]
print("Iterating through for loop")
for i in list:
print(i,end='\t')
list = [1, 3, 5, 7, 9]
print("\nIterating through while loop")
length = len(list)
i = 0
while i < length:
print(list[i],end='\t')
i += 1
list = [1, 3, 5, 7, 9]
print("\nIterating using list comprehend")
[print(i,end='\t') for i in list]
list = [1, 3, 5, 7, 9]
print("\nIterating using enumerate")
for i, val in enumerate(list):
print (val,end='\t')
Output
Iterating through for loop
1 3 5 7 9
Iterating through while loop
1 3 5 7 9
Iterating using list comprehend
1 3 5 7 9
Iterating using enumerate
1 3 5 7 9
How to Flatten a List in Python?
In the below code, the list is flattened using list comprehend, and nested for loop.
This list comprehension loops through all of the lists in the Python variable “foods.” After that, each value is added to the main list. We print our list to the console once it has been generated.
To iterate over each list item in each list in the original list of lists, our code uses two for loops.
foods = [
["Tomato and Cucumber", "Hummus, Beetroot, and Lettuce"],
["Cheese", "Egg"],
["Ham", "Bacon", "Chicken Club", "Tuna"]
]
print("using list comprehension\n")
new_foods = [food for sublist in foods for food in sublist]
print(new_foods)
print("\nusing nested for loop\n")
new_foods = []
for sublist in foods:
for food in sublist:
new_foods.append(food)
print(new_foods)
Output
using list comprehension
['Tomato and Cucumber', 'Hummus, Beetroot, and Lettuce', 'Cheese', 'Egg', 'Ham', 'Bacon', 'Chicken Club', 'Tuna']
using nested for loop
['Tomato and Cucumber', 'Hummus, Beetroot, and Lettuce', 'Cheese', 'Egg', 'Ham', 'Bacon', 'Chicken Club', 'Tuna']
How to get Length of the List in Python?
The len()
method gets the length of the list. The len()
is an in-built function in python, that returns the length of a given object.
sample_list=[12,87,"hello",4,"tech",90,4]
print("The length of given list is ",len(sample_list))
Output
The length of given list is 7
How to Print a List in Python?
The list can be printed in two ways.
- Just passing the list name in the print() method prints the list.
- Using for loop the list iterated through and printed.
print("using print statement\n")
sample_list=[12,87,"hello",4,"tech",90,4]
print(sample_list)
print("\nusing for loop\n")
for x in range(len(sample_list)):
print(sample_list[x], end='\t')
Output
using print statement
[12, 87, 'hello', 4, 'tech', 90, 4]
using for loop
12 87 hello 4 tech 90 4
How to Check if an Element is in a List Python?
The for loop is used to iterate through the list. If the element is found in the list, the counter variable is set to 1.
MyList = ['hello','Pythonwife',78,'d',345]
Counter=0
word=input("enter the word to be found\n")
for i in MyList:
if(i == word) :
Counter=1
if(Counter== 1) :
print("is present in the List")
else:
print("is not present in the List")
Output
enter the word to be found
Pythonwife
is present in the List
How to Make an Empty List in Python?
An empty list can be created using an empty pair of square brackets or using the list()
constructor.
sample_list=[]
new_list=list()
print(sample_list)
print(new_list)
Output
[]
[]
How to Convert String to List in Python?
The spilt()
method is used to split the string and store it in a list. The split()
method uses the delimiter string “delimiter” to return a list of the words in the string.
def ConvertStringToList(string):
li = string.split(" ")
return li
str1 = "Knowledge is power"
print(ConvertStringToList(str1))
Output
['Knowledge', 'is', 'power']
How to Loop through a List in Python?
In the below code, for loop, and while loop is used iterate and loop through the list.
list = [1, 3, 5, 7, 9]
print("Iterating through for loop")
for i in list:
print(i,end='\t')
list = [1, 3, 5, 7, 9]
print("\nIterating through while loop")
length = len(list)
i = 0
while i < length:
print(list[i],end='\t')
i += 1
Output
Iterating through for loop
1 3 5 7 9
Iterating through while loop
1 3 5 7 9
How to Check if a List is Empty Python?
The len()
method is used to return the length of the list passed as an argument. If the length is returned as 0, then it indicates that the list is empty.
my_list=[]
length=len(my_list)
if(length == 0):
print("The list is empty")
else:
print("The list is not empty")
Output
The list is empty
How to Append a List in Python?
In the following code, the list newlist is appended to mylist using the append()
method.
mylist=["apple","orange","banana"]
newlist=["tomato,potato"]
newlist.append(mylist)
print("The appended list is")
print(newlist)
Output
The appended list is
['tomato,potato', ['apple', 'orange', 'banana']]
How to Index a List in Python?
The index()
method is used to return the index of the specified element in the list. The index()
method takes 3 arguments: the element to be searched, start index to indicate the starting index to search and, end index to indicate the ending index to search.
vowels = ['a', 'e', 'i', 'o', 'i', 'u']
result = vowels.index('e')
print('The index of e:', result)
result = vowels.index('i')
print('The index of i:', result)
Output
The index of e: 1
The index of i: 2
How to Remove an Element from a List Python?
The clear()
method is used to remove all the elements in the list. The pop()
method is used to remove an element by index and get its value. The remove()
method will remove an element by its value.
The del()
method is used to remove items by index or slice. To remove an element that meets certain condition list comprehension is used
l=[1,2,3,4,5,6]
print(l)
l.clear()
print("Using clear method",l)
l=[1,2,3,4,5,6,45,89,78,76,77,90,34,22]
print("The element to be removed is",l.pop(3))
print("Using pop method",l)
l.remove(45)
print("Using remove method",l)
del l[6]
print("Usind del method",l)
del l[2:5]
print("Removing multiple elements with del",l)
print("Removing the elements that are odd numbers using list comprehension")
print([i for i in l if i % 2 == 0])
Output
[1, 2, 3, 4, 5, 6]
Using clear method []
The element to be removed is 4
Using pop method [1, 2, 3, 5, 6, 45, 89, 78, 76, 77, 90, 34, 22]
Using remove method [1, 2, 3, 5, 6, 89, 78, 76, 77, 90, 34, 22]
Usind del method [1, 2, 3, 5, 6, 89, 76, 77, 90, 34, 22]
Removing multiple elements with del [1, 2, 89, 76, 77, 90, 34, 22]
Removing the elements that are odd numbers using list comprehension
[2, 76, 90, 34, 22]
How to get the Size of a List in Python?
The len()
function is in-built in python to find the size of the list.
my_list=["alice",90,"wonderland",98,78.9,"strength"]
print("size of the list is ",len(my_list))
Output
size of the list is 6
How to Declare a List in Python?
In Python, you can declare a list with square brackets, use the list()
method to start it, create an empty list, or use a list comprehension. In Python, square brackets are the most popular way to declare a list.
l=[]
my_list=list()
new_list=[1,2,3,4,5,6]
print(l,mylist,new_list)
Output
[] [] [1, 2, 3, 4, 5, 6]
How to Randomize a List in Python?
The shuffle()
method is used to randomize the elements in the list. The shuffle()
method will take the list and reorganize the elements in the list. This function is available in the random library.
import random
fruits = ["apple", "banana", "cherry","strawberry","pineapple"]
random.shuffle(fruits)
print(fruits)
Output
['pineapple', 'apple', 'cherry', 'banana', 'strawberry']
How to Find the Sum of Elements in a List in Python?
In the below code for loop is used to iterate through the elements and the value of each element is added to the sum.
The sum()
method also be used to find the sum of elements in the list.
total = 0
list1 = [11, 5, 17, 18, 23]
for ele in range(0, len(list1)):
total = total + list1[ele]
print("Sum for loop: ", total)
Sum=sum(list1)
print("Sum using sum method: ", Sum)
Output
Sum for loop: 74
Sum using sum method: 74
How to Add Numbers in a List Python?
In the following code, the user is asked to enter the length of the list. An empty list is initialized. Using for loop elements for the list are read from the user. The pre-defined function sum()
is performs addition of all the numbers in the list. The list should contain the only number-related data types or string numbers, otherwise, Python will throw an error because of the int conversion.
lst = []
num = int(input('How many numbers: '))
for n in range(num):
numbers = int(input('Enter number '))
lst.append(numbers)
print("Sum of elements in given list is :", sum(lst))
Output
How many numbers: 6
Enter number 1
Enter number 2
Enter number 34
Enter number 56
Enter number 8
Enter number 3
Sum of elements in given list is : 104
How to Split a List in Python?
The split()
method is used to elements in the list, by passing the delimiter as an argument. ‘\t’ is used as a delimiter here. So after delimiter, it is split into another element.
l = ['element1\t0238.94', 'element2\t2.3904', 'element3\t0139847']
[i.split('\t') for i in l]
Output
[['element1', '0238.94'], ['element2', '2.3904'], ['element3', '0139847']]
How to Slice a List in Python?
The slice()
method takes start index and end index as parameters. If the start index is alone mentioned, then from the start all the elements will be considered. If the end index is alone mentioned, then from the beginning all the elements till the end index will be considered.
Lst = ["hello","world","welcome","Tech",67,90,"hi"]
print(Lst[::])
print(Lst[3:])
print(Lst[:4])
print(Lst[2:5])
Output
['hello', 'world', 'welcome', 'Tech', 67, 90, 'hi']
['Tech', 67, 90, 'hi']
['hello', 'world', 'welcome', 'Tech']
['welcome', 'Tech', 67]
How to Convert a List of Strings to Integers in Python?
In the below code for loop is used to traverse through the list elements. The data type conversion is done for each element from string to an integer.
test_list = ['1', '4', '3', '6', '7','9']
print ("Original list is : " + str(test_list))
for i in range(0, len(test_list)):
test_list[i] = int(test_list[i])
print ("After conversion : " + str(test_list))
Output
Original list is : ['1', '4', '3', '6', '7', '9']
After conversion : [1, 4, 3, 6, 7, 9]
How to Delete an Element from a List in Python?
The clear()
method is used to delete all the elements in the list. The pop()
method is used to delete an element by index and get its value. The remove()
method will delete an element by its value.
The del()
method is used to delete items by index or slice. To delete an element that meets certain condition list comprehension is used.
l=[1,2,3,4,5,6]
print(l)
l.clear()
print("Using clear method",l)
l=[1,2,3,4,5,6,45,89,78,76,77,90,34,22]
print("The element to be removed is",l.pop(3))
print("Using pop method",l)
l.remove(45)
print("Using remove method",l)
del l[6]
print("Usind del method",l)
del l[2:5]
print("Removing multiple elements with del",l)
print("Removing the elements that are odd numbers using list comprehension")
print([i for i in l if i % 2 == 0])
Output
[1, 2, 3, 4, 5, 6]
Using clear method []
The element to be removed is 4
Using pop method [1, 2, 3, 5, 6, 45, 89, 78, 76, 77, 90, 34, 22]
Using remove method [1, 2, 3, 5, 6, 89, 78, 76, 77, 90, 34, 22]
Usind del method [1, 2, 3, 5, 6, 89, 76, 77, 90, 34, 22]
Removing multiple elements with del [1, 2, 89, 76, 77, 90, 34, 22]
Removing the elements that are odd numbers using list comprehension
[2, 76, 90, 34, 22]
How to Check if a String is in a List Python?
Using in method, we can check if the list has a string or not
test_list = [ 1,2,3,"hello",9,"all",10,"tech"]
if ("hello" in test_list):
print ("Element Exists")
else:
print("Element doesn't exist")
Output
Element Exists
How to Replace an Item in a List in Python?
In the below code, list indexing and for loop are used to replace an item in the list. With list indexing, we could also add or subtract the values.
prices = [99.95, 72.50, 30.00, 29.95, 55.00]
print("Using indexing\n")
prices[2] = 104.95
print(prices)
prices[0] = prices[0] + 5
print(prices)
print("\n")
for index, item in enumerate(prices):
if item > 50:
prices[index] = round(prices[index] - (prices[index] * 10 / 100), 2)
print("Using for loop\n", prices)
Output
Using indexing
[99.95, 72.5, 104.95, 29.95, 55.0]
[104.95, 72.5, 104.95, 29.95, 55.0]
Using for loop
[94.45, 65.25, 94.45, 29.95, 49.5]
How to Create a List of Tuples in Python?
The below code is used to create a list of tuples using list comprehension. The first element will be the same, while the second element will be the square of the first element.
sample_list = [1, 2, 5, 6]
result = [(val, pow(val, 2)) for val in sample_list]
print("List of tuples")
print(result)
Output
List of tuples
[(1, 1), (2, 4), (5, 25), (6, 36)]
How to Make a Copy of a List in Python?
To make a copy of a list the in-built function copy()
in python is made use of in the following code.
my_list=[23,1,4,5,6,7,8]
new_list=[]
new_list=my_list.copy()
print("The copied list is\n",new_list)
Output
The copied list is
[23, 1, 4, 5, 6, 7, 8]
How to find Next Element in List without using Iterator in Python?
The next()
method is used to find the next item in the list without using an iterator.
mylist = iter(["apple", "banana", "cherry","strawberry","orange"])
x = next(mylist)
print(x)
x = next(mylist)
print(x)
x = next(mylist)
print(x)
Output
apple
banana
cherry
How to Take the First Item from list using Python?
The first integer from the list can be obtained using list index and list slicing.
test_list = [1, 5, 6, 7, 4]
print ("Using list index")
res1 = test_list[0]
print ("The first element of list is : " , res1)
res2 = test_list[0:1]
print ("The first element of list is : " , res2)
Output
Using list index
The first element of list is : 1
The first element of list is : [1]
The first element of list is : [1, 1]
How to Get Last Item from the List using Python?
The last item can be retrieved using list index and list slicing. In the list index, either -1 can be used to get the last index element, or len(list)-1 can be used to get the last index element.
test_list = [1, 5, 6, 7, 4]
print ("Using list index")
res1 = test_list[len(test_list)-1]
print ("The first element of list is : " , res1)
res2 = test_list[len(test_list)-1:]
print ("The first element of list is : " , res2)
Output
Using list index
The first element of list is : 4
The first element of list is : [4]
How to Loop over a List of Dictionary in Python?
A list of dictionary of ages is created. And to loop over a list of dictionaries, for loop is used.
myList = [{'age':x} for x in range(1,10)]
for i, age in enumerate(d['age'] for d in myList):
print(age,end="\t")
Output
1 2 3 4 5 6 7 8 9
How to Check How Many Duplicates are Present Inside a List in Python?
The given list is iterated through, and the duplicate elements are stored in a separate list. To find the number of duplicates, len() function is used on the list of duplicate items.
def duplicate(x):
size = len(x)
repeated = []
for i in range(size):
k = i + 1
for j in range(k, size):
if x[i] == x[j] and x[i] not in repeated:
repeated.append(x[i])
return repeated
list1 = [10, 20, 30, 20, 20, 30, 40,
50, -20, 60, 60, -20, -20]
print("The duplicated elements are:")
print (duplicate(list1))
print("No.Of.Duplicates",len(duplicate(list1)))
Output
The duplicated elements are:
[20, 30, -20, 60]
No.Of.Duplicates 4
How to Print List Items Without Bracket With Comma?
The symbol * is used to print a list without brackets. The argument sep is used in print()
function, to print list items with a comma.
my_list = ["Welcome", "Home", "people",1,3,6]
print(*my_list, sep = ", ")
Output
Welcome, Home, people, 1, 3, 6
How to Store a List of Objects in an Array using Python?
The Python NumPy package has built-in functions for converting lists to arrays.
import numpy as np
my_list = [2,4,6,8,10,"hello"]
my_array = np.array(my_list)
# printing my_array
print(my_array)
# printing the type of my_array
print(type(my_array))
Output
['2' '4' '6' '8' '10' 'hello']
<class 'numpy.ndarray'>
How to Loop Over a List of Dictionary to Print Key-Value Pairs in Python?
To print key-value pairs in the list of dictionaries for loop is used to iterate and print()
function is used to print.
list=[{'a': 3}, {'b': 5},{'c':6}]
for i in list:
print(i)
Output
{'a': 3}
{'b': 5}
{'c': 6}
How Access List of Dictionaries in Python?
Just loop over all the items in your list, and then for each of the dictionaries, we can access whatever values we want. The following code just the keys in the list of dictionaries
my_list=[{'a': 3}, {'b': 5},{'c':6}]
for d in my_list:
for key in d:
print(d[key])
Output
3
5
6
How to Update a List in Python by Index?
A single item or multiple elements can be updated using an index in Python.
list_new = ['physics', 'chemistry', 'maths', 2000,1965];
print("Value at index 2 : ")
print(list_new)
list_new[2] = 2001;
print("updated at index 2 : ")
print(list_new)
list_new[0]="Biology"
list_new[4]=1678
print("Updated multiple index :")
print(list_new)
Output
Value at index 2 :
['physics', 'chemistry', 'maths', 2000, 1965]
updated at index 2 :
['physics', 'chemistry', 2001, 2000, 1965]
Updated multiple index :
['Biology', 'chemistry', 2001, 2000, 1678]
How to Force Write Python List to a File?
To write a list into the file, we open a file in write mode using open()
method. The write()
method takes the list as the parameter, to write the list content into the file.
new_list = ["abc", "def", "ghi","wdf"]
textfile = open("sample.txt", "w")
for element in a_list:
textfile.write(element + "\n")
textfile.close()
Output
#sample.txt
abc
def
ghi
wdf
How to Store a Random Integer in List Python?
The random.randint()
function is used to generate a random integer number, which is then stored in the existing list using the append()
function.
import random
randomlist = [1,2,3,4]
n = random.randint(1,30)
randomlist.append(n)
print(randomlist)
Output
[1, 2, 3, 4, 11]
How to Make a List of Variables in Python?
To make a list of variables place the variables of any data type inside the square brackets.
my_list=[1,2,"hello",9.6]
print(my_list)
Output
[1, 2, 'hello', 9.6]
How do you Read a Text File into a List using Python?
The read()
function is used to read the content of the file. The split()
function is used to return the list of values of the file split by the parameter sep.
my_file = open("sample.txt", "r")
content = my_file.read()
content_list = content.split(",")
my_file.close()
print("The file content as list:")
print(content_list)
Output
#sample.txt
1,2,3,hello,home
The file content as list:
['1','2','3','hello','home']
How to Build Reverse a List Function using Python?
The following user-defined function reverses the list by traversing using for loop and insert() function.
def reverse(a):
rev_str = []
for x in a:
rev_str.insert(0, x)
return rev_str
print(reverse([1,2,3,4,5]))
Output
[5, 4, 3, 2, 1]
How to Limit a List to 8 in Python?
The slicing method can be used to limit the size of the list to 8.
my_list=[1,2,3,4,5,6,7,8,9,10,11,12]
if len(my_list) > 8:
my_list = my_list[:8]
print(my_list)
Output
[1, 2, 3, 4, 5, 6, 7, 8]
How to Identify Whether a Variable is a List in Python?
The isinstance()
method can be used to identify whether a variable is a list or not. This method takes object and classinfo as parameters. The parameter classinfo is set to list here.
a_list = ["a", "b", "c"]
list_check = isinstance(a_list, list)
print(list_check," It is a list")
Output
True It is a list
How to Make a List of 20 Unique Random Integers in Python?
The random.sample()
method is used to generate 20 unique random integers.
import random
var = random.sample(range(1, 30), 20)
var
Output
[22, 7, 10, 11, 1, 12, 2, 5, 18, 28, 15, 29, 4, 21, 20, 14, 13, 8, 17, 23]
How to Encode when Appending to List in Python?
The encode()
method is used to encode the word. The append()
method is used to append the encoded-word at the end of the list.
str = ["hello","hi"]
word="PhythonwifeWebsite"
print ("The encoded string in utf8 format is : ",)
encodedword = word.encode('utf8', 'ignore')
print(encodedword)
str.append(encodedword)
print(str)
Output
The encoded string in utf8 format is :
b'PhythonwifeWebsite'
['hello', 'hi', b'Phythonwife']
How to Use a List in Python as a Stack?
The append()
method is used to add items and the pop()
method is used to remove the items.
stack = [10, 20, 30,"hello","hi"]
stack.append(40)
stack.append(50)
stack.append("welcome")
print ("Stack elements after append opration...");
print (stack)
print (stack.pop (), " is removed")
print (stack.pop (), " is removed")
print (stack.pop (), " is removed")
print ("Stack elements after pop operation...");
print (stack)
Output
Stack elements after append opration...
[10, 20, 30, 'hello', 'hi', 40, 50, 'welcome']
welcome is removed
50 is removed
40 is removed
Stack elements after pop operation...
[10, 20, 30, 'hello', 'hi']
How to Split List into One Word per Line Python?
The for loop is used to print one word per line from the list of words.
items=["hello","hi","welcome","to","Pythonwife","website"]
for item in items:
print (item)
Output
hello
hi
welcome
to
Pythonwife
website
How to Print the Index of 2d List in Python?
The where(condition)
method in Numpy can be used to find the index of 2d list.
import numpy as np
twod_list=[[1, 2, 3],
[4, 5, 6],
[7, 8, 9]]
print(twod_list)
index_y,index_x = np.where(twod_list == 6)
print(index_y,index_x)
Output
[1][2]
How to Pull a Random Variable from List using Python?
We can use random.choice()
method to pull a random variable from the list. The list variable is passed as an argument to the method.
my_list=[1,2,3,4,5,6,"hello","tech"]
print("The randomly chosen variable:")
print(random.choice(my_list))
Output
The randomly chosen variable:
hello
How to Pop from the Beginning of the List using Python?
The list.pop()
method can be used to pop the list items from the list. The index 0 is passed as an argument to the pop()
method, to pop from the beginning of the list.
a_list = [1, 2, 3,"hello","sample","tree",9,23]
a_list.pop(0)
a_list.pop(0)
print(a_list)
Output
[3, 'hello', 'sample', 'tree', 9, 23]
How to Take a Line from a Text File and Make it into a List in Python?
The strip()
method is used to remove the line breaks. The split()
method is used to create a list of words in a line. The append()
method is used to append the word to the list.
#sample.txt
This file
will
be converted to a list
a_file = open("sample.txt", "r")
list_of_lists = []
for line in a_file:
stripped_line = line.strip()
line_list = stripped_line.split()
list_of_lists.append(line_list)
a_file.close()
print(list_of_lists)
Output
[['This', 'file'],
['will'],
['be', 'converted', 'to', 'a', 'list']]
How to Print the Second Item in Nested List using Python?
By using the index of the nested list in the print()
function, we can print the second item in the nested list.
listsample=[[1,2,3],[1,"hi",9.8,[3,4,5]],["Welcome","all","Home"]]
print(listsample[1])
Output
[1, 'hi', 9.8, [3, 4, 5]]
How to Find and Replace Element in List using Python?
The for loop is used to iterate through the list to find the item. It is replaced with another item using the list index. The below code replaces item 1 with item 10
a= [1, 2, 3, 4, 5, 1, 2, 3, 4, 5, 1]
for n, i in enumerate(a):
if i == 1:
a[n] = 10
print("After replacing 1 with 10")
print(a)
Output
After replacing 1 with 10
[10, 2, 3, 4, 5, 10, 2, 3, 4, 5, 10]
How to Add Period After Numbers in List using Python?
The join()
method is used to add a period after every number in the list.
flavors = ['1','2','3','5','6','7']
". ".join(flavors)
Output
'1. 2. 3. 5. 6. 7'
How to Remove Columns from a List in Python?
The columns can be removed from the list using 2 methods:
- The del operator and for loop can be used.
- The pop() method and List comprehension can be used.
test_list = [[4, 5, 6, 8],
[2, 7, 10, 9],
[12, 16, 18, 20]]
print ("The original list is : " + str(test_list))
for j in test_list:
del j[1]
print ("column deletion-for loop and del : " + str(test_list))
[j.pop(1) for j in test_list]
print ("column deletion-pop and list comprehension : " + str(test_list))
Output
The original list is : [[4, 5, 6, 8], [2, 7, 10, 9], [12, 16, 18, 20]]
column deletion-for loop and del : [[4, 6, 8], [2, 10, 9], [12, 18, 20]]
column deletion-pop and list comprehension : [[4, 8], [2, 9], [12, 20]]
How to Return n Items from a List using Function in Python?
The slice method can be used in a user-defined function to return n items from the list.
def returnitems(lst,n):
newlist=lst[:n]
return newlist
lst=[1,2,3,4,5,6]
result=returnitems(lst,3)
print(result)
Output
[1, 2, 3]
How to List Every File in a directory using Python?
The listdir()
method in OS returns a list of all files and directories in a given directory. It’s the current directory by default.
import os
path = "C:/sample"
dir_list = os.listdir(path)
print("Files and directories in '", path, "' :")
print(dir_list)
Output
Files and directories in ' C:/sample ' :
['Pythonwife']
How to Check the Dimension of a List in Python?
The ndim parameter is used with the print statement to find the dimension of the list. The arange method in the NumPy module is used along with reshape to form lists.
import numpy as np
d1_list = np.arange(3)
d2_list = np.arange(12).reshape((3, 4))
d3_list = np.arange(24).reshape((2, 3, 4))
print(d1_list.ndim)
print(d2_list.ndim)
print(d3_list.ndim)
Output
1
2
3
How to Print a List on a New Line in Python?
The sep parameter can be used in the print()
method to print the value in a new line. The value of the sep attribute is set as “\n”.
print("printing lists in new line")
a=[1,2,3,4,5,6]
print(*a, sep = "\n")
Output
printing lists in new line
1
2
3
4
5
6
How to Return the First Number in a List using Python?
The first number in the list can be returned using 3 methods:
- Using list index.
- Using list slicing.
- Using list comprehension.
test_list = [1, 5, 6, 7, 4]
print ("The original list is : " + str(test_list))
res=test_list[0]
print ("first element using index : " + str(res))
res = test_list[0:1]
print ("first element using slicing : " + str(res))
res = [ test_list[i] for i in (0,0) ]
print ("first element using list comprehension : " + str(res))
Output
The original list is : [1, 5, 6, 7, 4]
first element using index : 1
first element using slicing : [1]
first element using list comprehension : [1, 1]
How to Exclude an Element from a List using Python?
The element can be excluded from the list in 3 ways:
- Using
remove()
method - Using
pop ()
method - Using del operator
myList = ["Bran",11,22,33,"Stark",22,33,11]
myList.remove("Bran")
print("Using remove method")
print(myList)
myList.pop(1)
print("Using pop method")
print(myList)
del myList[2]
print("Using del method")
print(myList)
Output
Using remove method
[11, 22, 33, 'Stark', 22, 33, 11]
Using pop method
[11, 33, 'Stark', 22, 33, 11]
Using del method
[11, 33, 22, 33, 11]
How to Copy an Array into a List in Python?
The tolist()
method in the Numpy library is used to copy an array into a list.
import numpy as np
arr = np.array([1, 2, 3])
print("Array: ",arr)
list1 = arr.tolist()
print("List: ",list1)
Output
Array: [1 2 3]
List: [1, 2, 3]
How to Apply a Function to Elements in a List in Python?
The map()
function is used to apply functions to elements in the list. In the below code, the list elements are converted to uppercase using the upper method.
a_list = ["Hello", "Bird", "kid"]
map_object = map(str.upper, a_list)
new_list = list(map_object)
print(new_list)
Output
['HELLO', 'BIRD', 'KID']
How to Combine Strings using While loop in List using Python?
The while loop is used to iterate through list items and store them in variable elements. The parameter end=""
is used to combine the strings.
myList = ['pineapple', 'banana', 'watermelon', 'mango']
index = 0
while index < len(myList):
element = myList[index]
print(element,end="")
index += 1
Output
pineapplebananawatermelonmango
Which Python Keyword is Used to Iterate through a List?
The iter()
and next()
is used to iterate through the list.
mylist = iter(["apple", "banana", "cherry"])
x = next(mylist, "orange")
print(x)
Output
apple
How to not include Hyphenated Words in List using Python?
The for loop is used to iterate through the list. The range()
function iterates through each character in each word of the list. The replace()
function replaces hyphen(-) with empty character(“”).
mylist=["hello-w-orld","welco-me-home-","a-ll"]
newlist=[]
for word in mylist:
size=len(word)
for i in range(0,size):
newword=word.replace("-","")
newlist.append(newword)
print(newlist)
Output
['helloworld', 'welcomehome', 'all']
How to Access the First Letter of the First Element in a List using python?
The list index can be used to print the first letter of the first element in a list. The index [0][0] indicates the first letter of a first element.
mylist=["hello"]
print(mylist[0][0])
Output
h
How to find Overlap between Two Lists Using Python?
The user-defined function intersection traverse through two lists. If similar elements are found, those similar elements are stored in a separate list.
def intersection(lst1, lst2):
lst3 = [value for value in lst1 if value in lst2]
return lst3
lst1 = [4, 9, 1, 17, 11, 26, 28, 54, 69]
lst2 = [9, 9, 74, 21, 45, 11, 63, 28, 26]
print(intersection(lst1, lst2))
Output
[9, 11, 26, 28]
How to Move Items from one List to another List in Python?
Using pop()
, insert()
and index()
methods the list elements can be moved from one list to another. In the following code, list element 10 is moved from list2 to list1.
test_list1 = [4, 5, 6, 7, 3, 8]
test_list2 = [7, 6, 3, 8, 10, 12]
res = test_list1.insert(4, test_list2.pop(test_list2.index(10)))
print("The list 1 : " + str(test_list1))
print("The list 2 : " + str(test_list2))
Output
The list 1 : [4, 5, 6, 7, 10, 3, 8]
The list 2 : [7, 6, 3, 8, 12]
How to Convert All Tuples in a List into a List using Python?
The List Comprehension method can be used to convert a list of tuples into a list.
inputs = [('Welcome', 'To'), ('Python', 'Wife'), ('Website', 'all')]
out = [item for t in inputs for item in t]
print(out)
Output
['Welcome', 'To', 'Python', 'Wife', 'Website', 'all']
How to Plot the Data in a List using Python?
The two lines x1, y1, and x2, y2 are taken as Lists. The data in the lists are plotted using the plot()
function.
import matplotlib.pyplot as plt
x1 = [1,4,3]
y1 = [2,6,1]
plt.plot(x1, y1, label = "line 1")
x2 = [3,2,9]
y2 = [4,7,3]
plt.plot(x2, y2, label = "line 2")
plt.xlabel('x - axis')
plt.ylabel('y - axis')
plt.title('Two lines on same graph!')
plt.legend()
plt.show()
Output
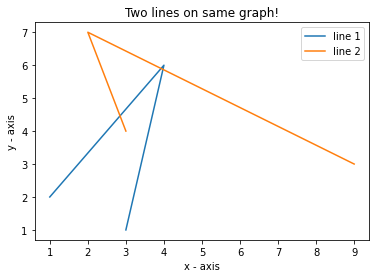
How to Check if All the Elements in a List is a String in Python?
To find if all the elements in the list are strings, the isinstance()
method is passed to the all()
method. The list item and the datatype are passed as arguments to the isinstance()
method.
l = ["one", "two", 3]
print(all(isinstance(item, str) for item in l))
l = ["one", "two", '3']
print(all(isinstance(item, str) for item in l))
Output
False
True
How to Divide a List into Equal Parts in Python?
Using the slice operator and moving the initial and final positions by a fixed integer, we can break a list into equal-sized portions.
my_list=[10,20,30,40,50,60,70,80,90,100,110,120]
x=0
y=12
for i in range(x,y,4):
x=i
print (my_list[x:x+4])
Output
[10, 20, 30, 40]
[50, 60, 70, 80]
[90, 100, 110, 120]
How to Have a Function Accept a List as Argument in Python?
The list can be passed as an argument to a function, by simply passing the name of the list to the function.
def my_function(names):
for x in names:
print(x)
names = ["Knowledge","tech", "Pythonwife", "Website"]
my_function(names)
Output
Knowledge
tech
Pythonwife
Website
How to Sort a List Alphabetically in Python?
The sorted()
method is used to sort the list alphabetically. It takes the list to be sorted as a parameter.
my_list = ["Knowledge","CherCher","tech", "Pythonwife", "Website"]
sorted_list = sorted(my_list)
print(sorted_list)
Output
['CherCher', 'Knowledge', 'Pythonwife', 'Website', 'tech']
How to Remove Duplicates from a List in Python?
The for loop is used to traverse through the list. If any element is duplicated, it is stored in a separate list and is returned.
def duplicate(x):
size = len(x)
repeated = []
for i in range(size):
k = i + 1
for j in range(k, size):
if x[i] == x[j] and x[i] not in repeated:
repeated.append(x[i])
return repeated
list1 = [10, 20, 30, 20, 20, 30, 40,
50, -20, 60, 60, -20, -20]
print("The duplicated elements are:")
print (duplicate(list1))
Output
The duplicated elements are:
[20, 30, -20, 60]
How to Find the Index of an Element in a List in using Python?
The index() method can be used to find the index of an element.
fruits=["apple","cherry","strawberry","banana","orange","pineapple"]
print("Index of orange is : ",fruits.index('orange'))
Output
Index of orange is : 4
How to Search an Element in List using Python?
The for loop is used to traverse through the list and the list index can be used to search the element in the list.
def search_ele(list_1, key):
for i in range(len(list_1)):
if list_1[i] == key:
return i
return "Element not found"
my_list = ["bat", 3, "apple", "red", 4.8]
print("The apple is found at index:",search_ele(my_list, 'apple'))
print(search_ele(my_list, 15))
Output
The apple is found at index: 2
Element not found
How to Alphabetize a List in Python?
The sort()
method can be used to alphabetize a list in python.
vowels = ['e', 'a', 'u', 'o', 'i']
vowels.sort()
print('Sorted list:', vowels)
Output
Sorted list: ['a', 'e', 'i', 'o', 'u']
How to Make a 2d List in Python?
By using List Comprehension we can make a 2d list in python.
x = 2
y = 3
a_2d_list = [[1 for j in range(y)] for i in range(x)]
print(a_2d_list)
Output
[[1, 1, 1], [1, 1, 1]]
How to sort a list using the Quick Sort method with start as the pivot in Python?
The Quicksort picks up a pivot and partitions the list around the pivot element.
def partition(start, end, array):
pivot_index = start
pivot = array[pivot_index]
while start < end:
while start < len(array) and array[start] <= pivot:
start += 1
while array[end] > pivot:
end -= 1
if(start < end):
array[start], array[end] = array[end], array[start]
array[end], array[pivot_index] = array[pivot_index], array[end]
return end
def quick_sort(start, end, array):
if (start < end):
p = partition(start, end, array)
quick_sort(start, p - 1, array)
quick_sort(p + 1, end, array)
array = [ 10, 7, 8, 9, 1, 5 ]
quick_sort(0, len(array) - 1, array)
print(f'Sorted array: {array}')
Output
Sorted array: [1, 5, 7, 8, 9, 10]
How to take off 2 Characters in an Item from List using Python?
The list slicing can be used to remove or take off any 2 characters from an element in the list.
keys = ['20134', '11013', '12014', '13015', '14016', '14027', '14031', '21004', '21505']
keys = [k[:-2] for k in keys]
keys
Output
['201', '110', '120', '130', '140', '140', '140', '210', '215']
How to Enumerate a List in Python?
The enumerate()
method can be used to add counter value to an iterable and returns an enumerable object. The list()
method can then be used to convert into a list of tuples.
l1 = ["Python","wife","website"]
obj1 = enumerate(l1)
print (list(enumerate(l1)))
Output
[(0, 'Python'), (1, 'wife'), (2, 'website')]
How to make a Deep Copy of a List in Python?
The deepcopy()
method in the copy library is used to make a deep copy of the list. In the case of deep copy, any changes made to the copied list do not reflect in the original list.
import copy
print("The original list :")
print(li1)
li1 = [1, 2, [3,5], 4]
print("The list after deep copy :")
li3 = copy.deepcopy(li1)
print(li3)
li3[1]="tech"
print("The copied list after modifying ")
print(li3)
print("The original list after modifying ")
print(li1)
Output
The original list :
[1, 'tech', [3, 5], 4]
The list after deep copy :
[1, 2, [3, 5], 4]
The copied list after modifying
[1, 'tech', [3, 5], 4]
The original list after modifying
[1, 2, [3, 5], 4]
What does List Index Out of Range Mean in Python?
The list out of index error arises when we try to access a list element that does not exist.
list_element=["python","wife","website"]
print(list_element[4])
Output
IndexError: list index out of range
How to Turn a List into a Matrix in Python?
The list slicing and for loop can be used to turn the list into a matrix.
# initializing list
test_list = [4, 6, 8, 1, 2, 9, 0, 10, 12]
print("The original list is : " + str(test_list))
N = 2
res = []
for idx in range(0, len(test_list) // N):
res.append(test_list[0: (idx + 1) * N])
print("Constructed Chunk Matrix : " + str(res))
Output
The original list is : [4, 6, 8, 1, 2, 9, 0, 10, 12]
Constructed Chunk Matrix : [[4, 6], [4, 6, 8, 1], [4, 6, 8, 1, 2, 9], [4, 6, 8, 1, 2, 9, 0, 10]]
How to Find the Largest Number in a List using Python?
The sort()
function can be used to sort the list. The last element from the sorted list is obtained using list slicing.
list1 = [10, 20, 4, 45, 99]
list1.sort()
print("Largest element is:", list1[-1])
Output
Largest element is: 99
How to Convert Array to List in Python?
The tolist()
method is used to convert an array to a list.
from array import *
def array_to_list(array_sample):
num_list = array_sample.tolist()
print(num_list)
array_sample = array('i', [45,34,67])
array_to_list(array_num)
Output
[45, 34, 67]
How to Check for Empty List in Python?
The len()
method is used to check if the list is empty or not. If the len()
method returns 0, the list is empty.
def checkempty(sample):
if(len(sample)==0):
print("The list is empty")
else:
print("The list is not empty")
list1=[]
list2=[1,2,3]
checkempty(list1)
checkempty(list2)
Output
The list is empty
The list is not empty
How to Find the Maximum Value in a List using Python?
The max()
function is used to find the maximum value in a list.
my_list=[23,9,34,56,0]
largest=max(my_list)
print("The maximum value :",largest)
Output
The maximum value : 56
How to Shuffle Elements in a List using Python?
The shuffle()
method in random library is used to shuffle the elements in a list.
import random
listnew=[1,2,3,4,5,6]
print("The original list is\n",listnew)
print("The shuffled list is ")
random.shuffle(listnew)
print(listnew)
Output
The original list is
[1, 2, 3, 4, 5, 6]
The shuffled list is
[3, 6, 4, 2, 5, 1]
How to Multiply All the Elements in a List using Python?
The prod()
method in the Numpy library is used to get the multiplication of all the elements in the list.
import numpy
my_list1 = [1, 2, 0]
my_list2 = [3, 3, 5]
result1 = numpy.prod(my_list1)
result2 = numpy.prod(my_list2)
print("Product of elements in list1: ", result1)
print("Product of elements in list2: ", result2)
Output
Product of elements in list1: 0
Product of elements in list2: 45
What does list() Function do in Python?
The list()
accepts an iterable object as input and creates a new list with its elements. Elements can take many forms. It might also be a different list or an iterable object, and it will be added to the new list in the same way.
print(list())
vowel_string = 'aeiou'
print(list(vowel_string))
vowel_tuple = (1,2,3,4,5,6)
print(list(vowel_tuple))
Output
[]
['a', 'e', 'i', 'o', 'u']
[1, 2, 3, 4, 5, 6]