Data structures
Data Structure is a systematic way of organizing the data on the computer. Data is nothing but some useful information like documents, photos, videos, audio, or programs stored in computer memory.
We know that every software deals with some data and performs operations based on the given data. The performance of a software application depends on how data is stored and organized. We have to organize the data in a certain way that makes the processing of the data much more efficient.
Algorithms
Algorithms are a set of instructions for a computer program to accomplish a task. Let us take a daily life example. To buy a coffee, we go to the coffee shop, pay money and buy coffee.
Similarly, in computer science, an algorithm helps us to execute a set of instructions in a program and get the expected output. For example, Google map uses a graph algorithm(Djikstra’s algorithm) to find the shortest path between the source and the destination.
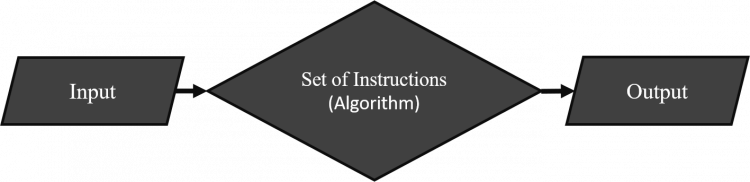
Types of Data Structures
The data structures can be broadly classified into two types based on if it is predefined in the programming language or is defined by the user.
- Primitive data structure: The data structures that are inbuilt in the programming language and are available to the user is called Primitive data structure. Some of the most commonly used predefined data structures are list, tuple, dictionary, and set.
- Non-Primitive data structure: The user-defined data structures are called Non-primitive data structures. The Non-Primitive data structures are subdivided into Linear and Non-linear data structures.
- Linear data structures: In linear data structures, the data items are arranged in the memory in a linear sequential manner. Linear data structures are classified as a linked list, stack, and queue.
- Non-linear data structures: In Non-linear data structures, the data items are not organized sequentially. Graphs and trees are non-linear data structures.
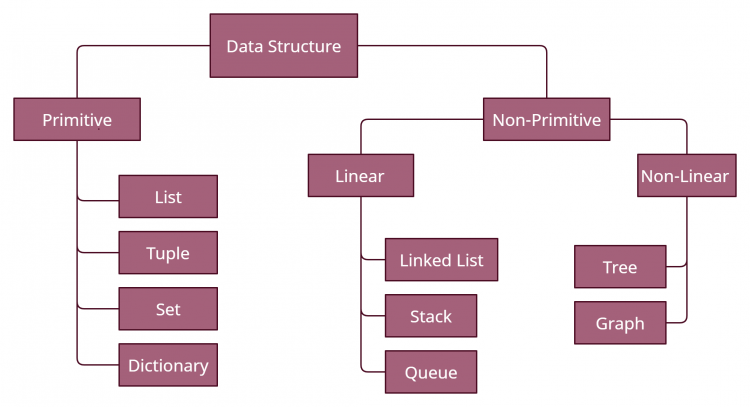
Types of Algorithms
Algorithms can be classified based on the problem-solving approach.
- Simple recursive algorithms: A recursive algorithm is an algorithm that calls itself recursively until a specified condition is met.
- Divide and conquer algorithms: In the divide and conquer algorithm, we divide a problem into sub-problems and solve the problem recursively. The solution of the sub-problems is combined to obtain the solution of the original problem. Quick sort and merge sort are examples of the divide and conquer algorithm.
- Dynamic Programming algorithms: The dynamic programming approach is based on memorization. This algorithm remembers the past results, which can be reused to get the new results.
- Greedy algorithms: A greedy algorithm is an algorithmic strategy that chooses an optimum solution at each step, which eventually leads to a global optimum solution.
- Brute force algorithms: A brute force algorithm is the simplest and straightforward algorithm to solve a problem.
- Randomized algorithms: The randomized algorithm used a random number at least once during evaluation, to make a decision. The quick sort algorithm is an example of a randomized algorithm. The quick sort algorithm picks a random pivot element and decisions are made based on these elements.