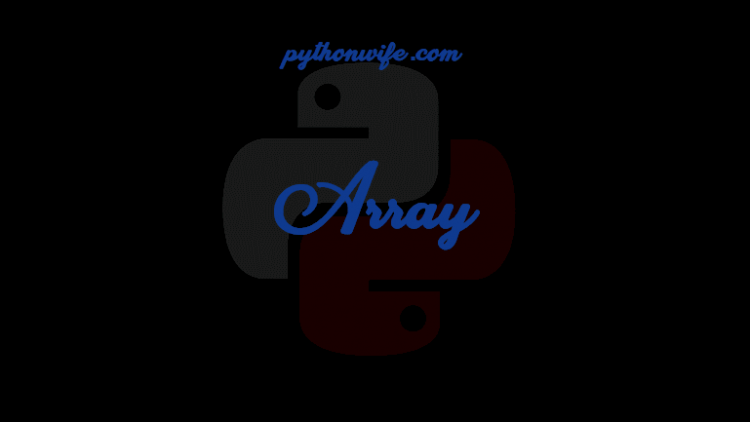
An array is a collection of objects of the same data type stored at the contiguous memory location. An array helps us to store multiple items of the same type together.
For example, if we want to store three numerical values, we can declare three variables and store the values. But, let us assume that we want to store 1000 numerical values, declaring 1000 variables and assigning them with their values will be difficult and time-consuming. In such cases, we can create an array of the specified size and store the values.
The below figure represents an array of integers of length 5. Each integer takes 4 bytes of memory.
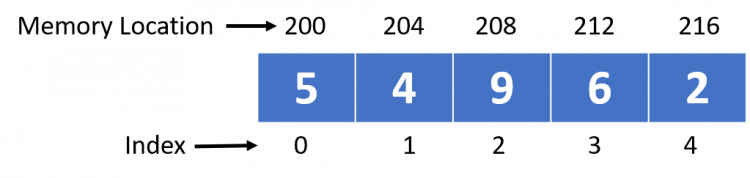
The properties of an array are described as follows.
- An array can store the elements of a specified data type.
- Each element of an array has a unique index. We can access the elements in an array by using the index value.
- The size or length of an array is predefined and cannot be modified.
- All the elements in an array are stored in the contiguous memory location.
Types of Array
An array can be classified based on the number of dimensions. The classification is given as follows.
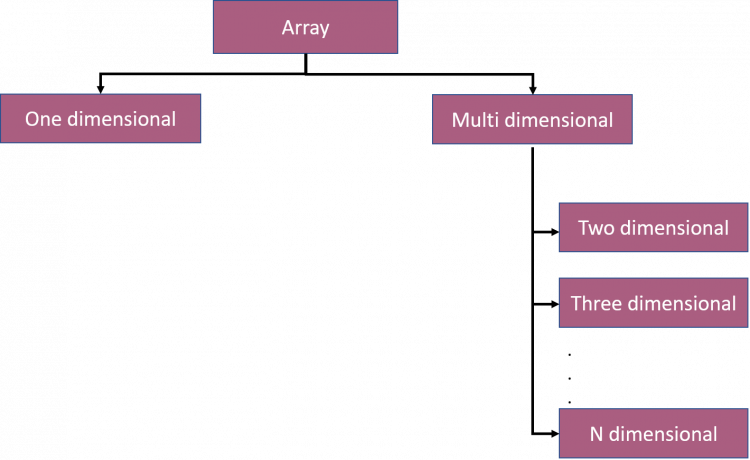
One-dimensional array
A one-dimensional array is also known as a linear array. In the one-dimensional array, each element is represented with a single index value. One dimensional array has only one row and multiple columns.
The following figure represents a one-dimensional array. We can access any element in the array by using the index values. For example, array[1] represents number 8, array[2] represents number 9, and so on.

All the elements in the one-dimensional array are stored contiguously. The below figure represents the memory allocation of the one-dimensional array. Since it is an array of integers, each element takes 4 bytes of memory.
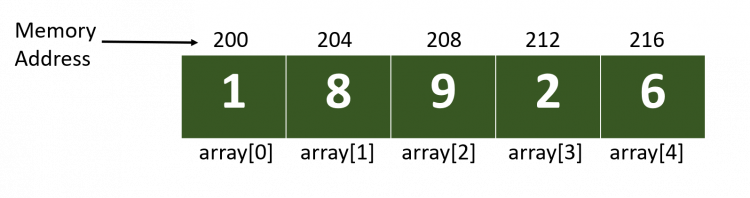
The total memory allocated to an array is the number of elements multiplied by the size of the individual element. In the above example, the total number of elements = 5 and the size of an integer element is 4 bytes. Hence, the total memory allocated is 5 * 4 = 20 bytes.
Two-dimensional array
A two-dimensional array is a combination of 1D arrays. In the two-dimensional array, the elements are stored in the form of a table and each element is represented with double index values. A two-dimensional array can have multiple rows and multiple columns.
The syntax for accessing an element in a two-dimensional array is,
#syntax:
array_name[i][j]
#i represents row number
#j represents column number
The following figure represents a two-dimensional array with three rows and three columns. The arr[0][0] represents number 3, arr[0][1] represents 6, and so on.
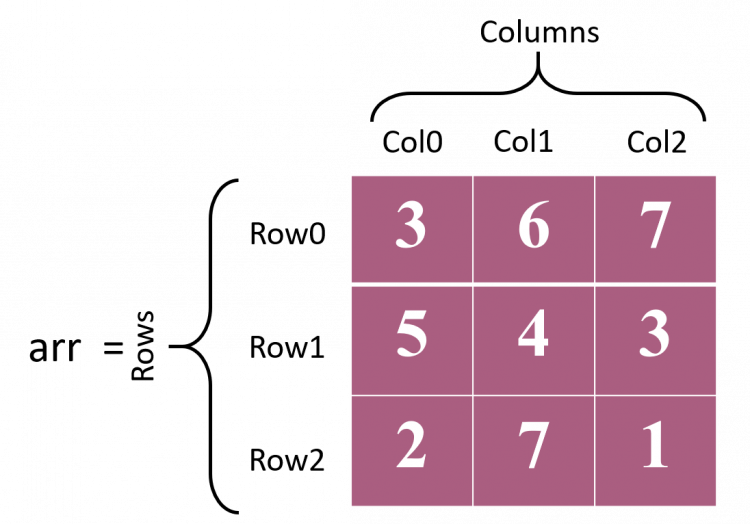
The multi-dimensional arrays are stored in the memory in a row-wise order or column-wise order. In the row-wise order, all the elements of the 0th row will be followed by the elements of the 1st row and so on, and in the column-wise order, the elements of the 0th column will be followed by the 1st column and so on.
The below figure represents a 2D array stored in a row-wise manner in the memory. The total number of elements = 9 and since it is an array of integers, the size of the element = 4 bytes. The total memory space consumed = 9 * 4 = 36 bytes.
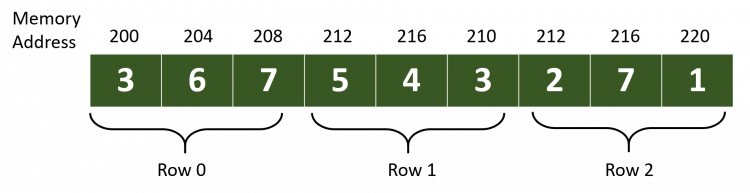
Three-dimensional array
A three-dimensional array is a combination of two-dimensional arrays. The elements in the three-dimensional array are represented with three index values.
The syntax for accessing an element in a three-dimensional array is,
#syntax:
array_name[i][j][k]
#i represents array number
#j represents row number
#k represents the column number
The following figure represents a three-dimensional array. The arr[1][1][2] represents number 8, arr[2][0][0] represents number 3 in the three-dimensional array given below.
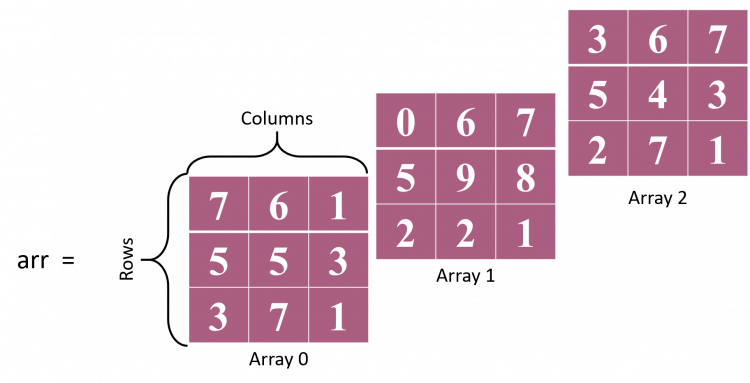
The below figure represents a 3D array stored in a row-wise manner in the memory, i.e. all the rows of the first array will be followed by the rows of the second array and so on.
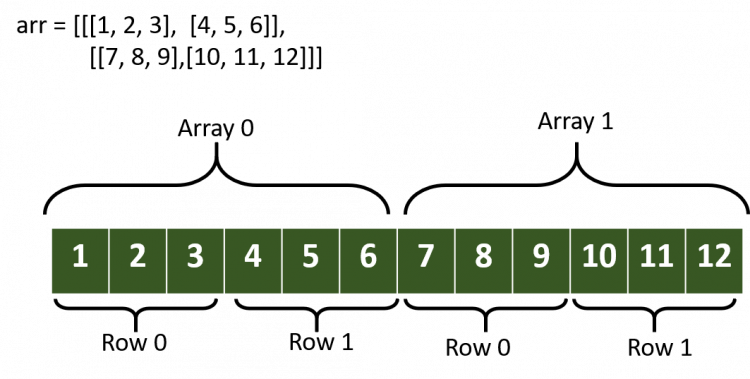
Creating an Array
In python, we don’t have an inbuilt array data structure. Instead of an array, we have another inbuilt data structure called a list, which can store a collection of objects of different data types. A user can treat a list as an array, but the main drawback of using a list is the user cannot constrain the type of elements stored in the list.
We can create an array in python, by using the array module. An array module creates an array of specified data types.
The syntax for creating an array is given below.
#syntax:
from array import *
array_name = array(type_code, [initializers])
The type of array is specified by using a type code, which is a single character. The different type codes are listed as follows.
Type Code | C type | Python Type | Minimum size(in bytes) |
‘b’ | signed char | int | 1 |
‘B’ | unsigned char | int | 1 |
‘u’ | wchar_t | unicode character | 2 |
‘h’ | signed short | int | 2 |
‘H’ | unsigned short | int | 2 |
‘i’ | signed int | int | 2 |
‘I’ | unsigned int | int | 2 |
‘l’ | signed long | int | 4 |
‘L’ | unsigned long | int | 4 |
‘q’ | signed long long | int | 8 |
‘Q’ | unsigned long long | int | 8 |
‘f’ | float | float | 4 |
‘d’ | double | float | 8 |
In the following example, we create an array arr_1 of integer data type using ‘i’ type code and another array arr_2 of double data type using ‘d’ type code.
from array import *
#creating an array of integer data type
arr_1 = array('i', [3, 2, 7, 6, 9])
print(arr_1)
#Output: array('i', [3, 2, 7, 6, 9])
#creating an array of double data type
arr_2 = array('d', [1.4, 7.8, 9.6, 8.2])
print(arr_2)
#Output: array('d', [1.4, 7.8, 9.6, 8.2])
Time and space complexity of creating an array
The time complexity of creating an array is O(1) as we are just assigning a list of values to a variable, and the space complexity of creating an array is O(n), “n” represents the length of the array.
Getting the user input for an Array
Python has an inbuilt input() function which allows the programmer to ask users for input text. The control of the program pauses executing when it encounters an input() function and resumes the execution again when the user has given the input.
In the following example, we create an empty array arr_1 of integer data type using the array module. In the next step, we ask the user for the length of the array.
Then, we use a for loop that iterates for a range between 0 to the length of the array. In each iteration, we ask the user for the value and add it to the arr_1 using the append() function.
from array import *
#creating an empty array
arr_1 = array('i', [])
#Asking the user for the length of the array
n = int(input("Enter the length of the array: "))
#Adding the elements to the array using for loop
for i in range(n):
val = int(input("Enter value: "))
arr_1.append(val)
print("Array: ", arr_1)
The above code returns the output as
Enter the length of the array: 4
Enter value: 5
Enter value: 7
Enter value: 14
Enter value: 12
Array: array('i', [5, 7, 14, 12])
Inserting an element in an Array
We can insert an element in an array using the insert() function. The insert() function inserts an element at a specific index in the array.
The syntax for the insert() function is as follows.
#syntax:
array_name.insert(index, element)
Parameters:
- index: The index represents the position, where the element is to be inserted.
- element: The element represents the new item that will be inserted into the array.
In the following example, we create an array arr_1 and insert a new element at different positions.
from array import *
#creating an array of integer data type
arr_1 = array('i', [5, 3, 4, 8, 9])
print(arr_1)
#Output: array('i', [5, 3, 4, 8, 9])
#inserting the element 1 at the 0th index
arr_1.insert(0, 1)
print(arr_1)
#Output: array('i', [1, 5, 3, 4, 8, 9])
#inserting the element 7 at the 2nd index
arr_1.insert(2, 7)
print(arr_1)
#Output: array('i', [1, 5, 7, 3, 4, 8, 9])
Time and space complexity of the insert()
The time complexity of the insert() function depends on the position in which we insert an element.
For example, inserting an element at the beginning of an array i.e. at the 0th index will shift each element to the right by one index. Hence, the time complexity will be O(N) where N is the number of elements.
But, inserting an element at the end of an array will just take constant time, i.e O(1) because we are not moving any element to the right or left.
The space complexity for insert() operation is O(1) because we are allocating just one extra space for the insertion of an element.
Traversing an Array
Traversing means going through all the elements one by one. We can traverse an array using a for loop. In the following example, a for loop traverses through all the elements of arr_1 and prints the element in each iteration.
from array import *
#creating an array of integer data type
arr_1 = array('i', [5, 3, 4, 8, 9])
#Traversing the array arr_1
for ele in arr_1:
print(ele)
The above program returns the output as
5
3
4
8
9
Time and space complexity to traverse an array
The time complexity of the above code is linear, i.e O(N) as the for loop traverses each element of the array and the space complexity is O(1) because we are not using any extra space for traversing.
Accessing an element of an Array
We can access an element at a specific index using the index [] operator. The syntax to access an element in an array is as follows.
#syntax:
array_name[index_val]
If the index_val is greater than or equal to the length of the array, the program raises an IndexError exception.
To overcome this error, we use a conditional statement to check if the index_val is greater than or equal to the length of the array. If the condition is True, the program gives output as “No element found”. Otherwise, the program prints the element as output.
from array import *
def access_ele(arr, index_val):
#checking if index_val greater than len(arr)
if index_val >= len(arr):
return "No element Found"
else:
return arr[index_val]
#creating an array of integer data type
my_arr = array('i', [5, 3, 4, 8, 9])
#Accessing an element at the 2nd index
print(access_ele(my_arr, 2))
#Output: 4
#Accessing an element at the 5th index
print(access_ele(my_arr, 5))
#Output: "No element Found"
Time and space complexity of accessing an element
The time complexity to access an element in an array is constant, i.e. O(1) and the space complexity is also O(1) as we are not using any additional space to access an element.
Searching for an element in an Array
In the following example, we use the linear search algorithm to search for an element in the array. We use a for loop to iterate over all the elements in the array. The program compares if the element at a specific index is equal to the key element in each iteration.
If the element is found in the array, the index value is returned as output. Otherwise, the program returns the statement “Element not found” as output.
from array import *
def search_ele(arr, key):
for i in range(len(arr)):
if arr[i] == key:
return i # returning the index val
return "Element not found"
#creating an array of integer data type
my_arr = array('i', [5, 3, 4, 8, 9])
#searching for element 4
print(search_ele(my_arr, 4))
#Output: 2
#searching for an element 15
print(search_ele(my_arr, 15))
#Output: "Element not found"
Time and space complexity to search an element
The time complexity to search for an element in an array using the linear search algorithm is O(N) and the space complexity is O(1).
Deleting an element from an Array
The remove() function deletes the first occurrence of the specified element from an array. The syntax for the remove() function is as follows.
#syntax:
array_name.remove(ele)
If the element to be removed is not found in the array, then the program raises a ValueError exception.
Example,
from array import *
#creating an array of integer data type
my_arr = array('i', [5, 3, 4, 8, 9])
#Deleting element 4 from my_arr
my_arr.remove(4)
print(my_arr)
#Output: array('i', [5, 3, 8, 9])
#Deleting element 10 form my_arr
my_arr.remove(10)
print(my_arr)
#Output: ValueError: array.remove(x): x not in array
Time and space complexity of remove()
The time complexity of the remove() function depends on the item we are removing.
For example, deleting an element at the beginning of an array i.e. at the 0th index will shift each element to the left by one index. Hence, the time complexity will be O(N) where N is the number of elements.
But, deleting an element from the end of the array will just take constant time, i.e O(1) because we are not moving any element to the right or left.
The space complexity of the remove() function is O(1) because we are not using any additional space.
Operations on a One-Dimensional Array
The array module comes with several other inbuilt functions. Some of them are described as follows.
append()
The append() functions add a new item at the end of an array. The syntax for using the append() function is as follows.
#syntax:
array_name.append(item)
Example,
from array import *
#creating an array of integer data type
my_arr = array('i', [5, 3, 4])
#Appending the element 15 using append()
my_arr.append(15)
print(my_arr)
#Output: array('i', [5, 3, 4, 15])
extend()
The extend() function takes an iterable as a parameter and adds all the items in the iterable at the end of the array. The element in the iterable must be of the same data type. The iterable can be an array, list, tuple, etc.
The syntax for the extend() function is given below.
#syntax:
array_name.extend(iterable)
Example,
from array import *
#creating an array of integer data type
my_arr = array('i', [5, 3, 4])
#Adding the items of arr_2 using extend()
arr_2 = array('i', [1, 2])
my_arr.extend(arr_2)
print(my_arr)
#Output: array('i', [5, 3, 4, 1, 2])
fromlist()
The fromlist() takes a list as a parameter and adds all the items of the list at the end of the array. The syntax for fromlist() method is given below.
#syntax:
array_name.fromlist(list)
Example,
from array import *
#creating an array of integer data type
my_arr = array('i', [5, 3, 4])
#Adding the item of a list using fromlist()
list_1 = [15, 13]
my_arr.fromlist(list_1)
print(my_arr)
#Output: array('i', [5, 3, 4, 15, 13])
pop()
The pop() function takes an index value as a parameter and removes the item at the specified index. By default, the index value = -1 i.e. if the index value is not provided the pop() function removes the last element of the array.
The syntax for the pop() function is as follows.
#syntax:
array_name.pop(index)
Example,
from array import *
#creating an array of integer data type
my_arr = array('i', [5, 3, 4])
#Popping an item using pop()
my_arr.pop() #removes last item
print(my_arr)
#Output: array('i', [5, 3])
index()
The index() function returns the index of the first occurrence of the specified item. The syntax for the index() function is as follows.
#syntax:
array_name.index(element)
Example,
from array import *
#creating an array of integer data type
my_arr = array('i', [5, 3, 4, 15])
#Getting the index of item 15
ind = my_arr.index(15)
print("Index val of element 15: ", ind)
reverse()
The reverse() method reverses the order of elements in an array. The syntax for the reverse() method is as follows.
#syntax:
array_name.reverse()
Example,
from array import *
#creating an array of integer data type
my_arr = array('i', [5, 3, 4, 15])
my_arr.reverse() #reverses my_arr
print(my_arr)
#Output: array('i', [15, 4, 3, 5])
buffer_info()
The buffer_info() returns the current memory address and the length of the array in a tuple format. The syntax for using buffer_info() is as follows.
#syntax:
array_name.buffer_info()
In the following example, we initialize my_arr = array(‘i’, [5, 3, 4, 15]). The statement my_arr.buffer_info() returns the output as (2324029538416, 4). The number 2324029538416 represents the current memory address of my_arr and the number 4 represents the length of the my_arr.
from array import *
#creating an array of integer data type
my_arr = array('i', [5, 3, 4, 15])
print(my_arr.buffer_info())
#Output: (2324029538416, 4)
count()
The count() function returns the number of occurrences of a specific element in an array. The syntax for using the count() method is given below.
#syntax:
array_name.count(val)
Example,
from array import *
#creating an array of integer data type
my_arr = array('i', [5, 3, 4, 15, 5, 5])
#Counting the occurrences of element 5
print(my_arr.count(5))
#Output: 3
tolist()
The tolist() method converts an array to a list. The syntax for tolist() is as follows.
#syntax:
array_name.tolist()
Example,
from array import *
#creating an array of integer data type
my_arr = array('i', [5, 3, 4, 15])
#Converting an array to a list
my_list = my_arr.tolist()
print(my_list)
#Output: [5, 3, 4, 15]
Slicing
We can slice an array by using the indexing syntax. For example, consider an array my_arr = array(‘i’, [7, 9, 4, 5, 6, 10, 15, 12]). Slicing the array form index 1 to index 5 will give output as array(i, [9, 4, 5, 6, 10]).
The syntax to slice in an array is given below.
#syntax:
array_name[start: stop]
Parameters:
- start: The starting index value where the slicing of an array starts. By default, the value of start is 0 if not provided.
- stop: The ending index value until where the slicing takes place. The slicing stops at index end -1. By default, the value of the end parameter is the length of the array, if not provided.
Example,
from array import *
#creating an array of integer data type
my_arr = array('i', [7, 9, 4, 5, 6, 10, 15, 12])
print(my_arr[1:6]) #start = 1, end = 6
#Output: array('i', [9, 4, 5, 6, 10])
print(my_arr[: 5]) #start = 0, end = 6
#Output: array('i', [7, 9, 4, 5, 6])
print(my_arr[2: ]) #start = 6, end = 8
#Output: array('i', [4, 5, 6, 10, 15, 12])
Creating a Two-Dimensional Array
We know that two-dimensional arrays are a combination of 1D arrays. For example, [[4, 2, 3], [8, 9, 10], [6, 7, 8]] represents a two-dimensional array.
We will use the NumPy module instead of the array module to create a 2D array, as the NumPy module provides high-performance multidimensional arrays and different tools to work with these arrays.
The syntax for creating a 2D array using the NumPy module is as follows.
#syntax:
from numpy import *
arr_name = array(list or tuple)
In the following example, we create a 2D array by passing a list of lists as a parameter to the array() function.
from numpy import *
my_arr = array([[4, 5, 6], [5, 3, 2]])
print(my_arr)
#Output: [[4 5 6]
[5 3 2]]
Time complexity and space complexity of creating a two-dimensional array
The time complexity of creating a 2D array is O(1) as we are just assigning the values of a 2D array to a variable, and the space complexity of creating a 2D array is O(m*n), where “m” represents the number of rows, and “n” represents the number of columns.
Insertion in a Two-Dimensional Array
We can use the insert() function to add a row or column to a 2D array. There are two ways to add elements in a 2D array
- Adding a column
- Adding a row
The syntax for the insert() function is as follows.
#syntax:
insert(array, index, values, axis =None)
Parameters:
- array: The input array, where rows and columns will be inserted.
- index: The index values before which the values are to be inserted.
- values: The values to be added to the input array.
- axis: Axis along which we want to insert the values. If axis = 1, the elements will be added in a column-wise manner, and if axis = 0, the elements will be added in a row-wise manner. By default, the value of axis = None, i.e the values will be added to the flattened input array.
Adding a column to the two-dimensional array using insert()
In the following example, we create a 2D array arr_1 using the numpy module. The statement insert(arr_1, 1, [11, 12, 13], axis = 1) inserts a column with values [11, 12, 13] in the 1st column of the array arr_1. Here, axis = 1 represents that the values will be added in a column-axis.
from numpy import *
arr_1 = array([[4, 5, 6], [5, 3, 2], [7, 0, 4]])
print(arr_1)
#Output: [[4 5 6]
[5 3 2]
[7 0 4]]
#Insering a column before column 1
arr_2 = insert(arr_1,1, [11, 12, 13], axis = 1)
print(arr_2)
#Output: [[ 4 11 5 6]
[ 5 12 3 2]
[ 7 13 0 4]]
Adding a row to the two-dimensional array using insert()
In the following example, we create a 2D array arr_1 using the numpy module. The statement insert(arr_1, 1, [11, 12, 13], axis = 1) inserts a row with values [11, 12, 13] in the1st row of the array arr_1. Here, axis = 0 represents the values will be added in a row-axis.
from numpy import *
arr_1 = array([[4, 5, 6], [5, 3, 2], [7, 0, 4]])
print(arr_1)
#Output: [[4 5 6]
[5 3 2]
[7 0 4]]
arr_2 = insert(arr_1,1, [11, 12, 13], axis = 0)
print(arr_2)
#Output: [[ 4 5 6]
[11 12 13]
[ 5 3 2]
[ 7 0 4]]
Time and space complexity of insert()
The time complexity of the insert() function depends on the position in which we insert an element.
Consider a 2D array of size m x n, inserting a column at the 0th column will shift each element of the 2D array to the right by one step. Hence, the time complexity will be O(m*n).
But, inserting a column at the end of a 2D array will just take constant time, i.e O(1) because we are not moving any element to the right or left.
The space complexity to insert a row or column using insert() function is O(1).
Adding a row or column at the end of the 2D array using append()
We can add a row or column at the end of the 2D array using the append() function. The syntax for the append() function is as follows.
#syntax:
append(array, values, axis =None)
Parameters:
- array: The input array, where rows and columns will be inserted.
- values: The values to be added to the input array.
- axis: Axis along which we want to insert the values. If axis = 1, the elements will be added after the last column, and if axis = 0, the elements will be added after the last row. By default, the value of axis = None, i.e. the values will be added to the flattened input array.
Example,
from numpy import *
arr_1 = array([[4, 5, 6], [5, 3, 2], [7, 0, 4]])
print(arr_1)
#Output: [[4 5 6]
[5 3 2]
[7 0 4]]
#Adding a row to the arr_1
arr_2 = append(arr_1, [[11, 12, 13]], axis = 0)
print(arr_2)
#Output: [[ 4 5 6]
[ 5 3 2]
[ 7 0 4]
[11 12 13]]
#Adding a column to the arr_1
arr_3 = append(arr_1, [[11],[12], [13]], axis = 1)
print(arr_3)
#Output: [[ 4 5 6 11]
[ 5 3 2 12]
[ 7 0 4 13]]
The time complexity of the append() function is O(1) and the space complexity of the append() function is also O(1).
Accessing element from a 2D Array
We can access the element from a 2D array using double index values. The syntax for accessing an element in a two-dimensional array is,
#syntax:
array_name[i][j]
#i represents row number
#j represents column number_
The len(arr_name) gives the length of the row and len(arr_name[0]) gives the length of the column. If the index value of the row or column is greater than the last index value of the row or column, the program raises an IndexError exception.
To overcome this error, we use a conditional statement to check if the row_ind or col_ind is greater than the last index value of row and column. If the condition is True, the program gives output as “Incorrect index”. Otherwise, the program returns the element as output.
from numpy import *
def access_ele(arr, row_ind, col_ind):
#checking if row_ind and col_ind greater than ending index
if row_ind >= len(arr) or col_ind >= len(arr[0]):
return "Incorrect index"
else:
return arr[row_ind][col_ind]
my_arr = array([[4, 5, 6], [5, 3, 2], [7, 0, 4]])
#Accessing an element at the row_ind = 2, col_ind = 1
print(access_ele(my_arr, 2, 2))
#Output: 4
#Accessing an element at the row_ind = 3,col_ind = 2
print(access_ele(my_arr, 3, 2))
#Output: Incorrect index
Time and space complexity of accessing an element in 2D array
The time complexity to access an element in a 2D array is constant, i.e. O(1) and the space complexity is also O(1) as we are not using any additional space to access an element.
Traversing a 2D Array
We can iterate through all the elements of a 2D array using nested for loop. The first for loop iterates through each row of the 2D array, and the second nested for loop iterates through each column of the 2D array.
In the following example, we use the nested for loop that traverses each element of the 2D array and prints the data item in the 2D array.
from numpy import *
my_arr = array([[4, 5, 6], [5, 3, 2], [7, 0, 4]])
for i in range(len(my_arr)):
for j in range(len(my_arr[0])):
print(my_arr[i][j], end = " ")
#Output: 4 5 6 5 3 2 7 0 4
Time and space complexity of traversing a 2D array
The time complexity of the above code is O(m*n), as the for loop traverses each element of the array. Here, m is the number of rows and n is the number of columns. The space complexity is O(1) because we are not using any extra space for traversing.
Searching for an element in a 2D Array
In the following example, we use a for loop to traverse the 2D array. In each iteration, the program compares the element of the 2D array with the key element. If the element is found, the row index and column index are returned as output. Otherwise, the program returns the statement “Element not found” as output.
from numpy import *
def search_ele(arr, key):
for i in range(len(arr)):
for j in range(len(arr[0])):
if arr[i][j] == key:
return "row = " + str(i) +" col = " + str(j) # returning the index val
return "Element not found"
my_arr = array([[4, 5, 6], [5, 3, 2], [7, 0, 4]])
#searching for an element 7
print(search_ele(my_arr, 7))
#Output:row = 2 col = 0
#searching for an element 15
print(search_ele(my_arr, 15))
#Output: "Element not found"
Time and space complexity to search an element in a 2D array
The time complexity to search an element in a two-dimensional array is O(m*n) as we traverse through each element of the 2D array. The space complexity is O(1) because we are not using any additional space for searching an element.
Deleting a row or column from a 2D Array
There are two ways to delete elements from a 2D array
- Deleting a row
- Deleting a column
We can use the delete() function in the NumPy module to delete a row or column from a 2D array. The syntax for the delete() function is as follows.
#syntax:
delete(array, index, axis =None)
Parameters:
- array: The input array, from which rows and columns will be deleted.
- index: The index values of rows or columns to delete.
- axis: Axis along which we want to delete the values. If axis = 1, the elements fromthe column will be deleted, and if axis = 0, the elements from the row will be deleted. By default, the value of axis = None, i.e the values will be deleted from the flattened input array.
Deleting a column from a 2D array
In the following example, we create a 2D array arr_1 using the NumPy module. The statement delete(arr_1, 1, axis = 1) deletes the 1st column of the array arr_1.
from numpy import *
arr_1 = array([[4, 5, 6], [5, 3, 2], [7, 0, 4]])
print(arr_1)
#Output: [[4 5 6]
[5 3 2]
[7 0 4]]
#Deleting column 1
arr_2 = delete(arr_1, 1, axis = 1)
print(arr_2)
#Output: [[4 6]
[5 2]
[7 4]]
Deleting a row from a 2D array
In the following example, we create a 2D array arr_1 using the NumPy module. The statement delete(arr_1, 1, axis = 0) deletes the 1st row of the array arr_1.
from numpy import *
arr_1 = array([[4, 5, 6], [5, 3, 2], [7, 0, 4]])
print(arr_1)
#Output: [[4 5 6]
[5 3 2]
[7 0 4]]
#Deleting row 1
arr_2 = delete(arr_1, 1, axis = 0)
print(arr_2)
#Output: [[4 5 6]
[7 0 4]]
Time and space complexity of deleting a row or column in a 2D array
The time complexity of the delete() function depends on the position from which we delete an element.
Consider a 2D array of size m x n, deleting the 0th column will shift each element of the 2D array to the left by one step. Hence, the time complexity will be O(m*n).
But, deleting the last column of a 2D array will just take constant time, i.e O(1) because we are not moving any element to the right or left.
The space complexity to delete a row or column is constant, i.e. O(1).
Advantages and disadvantages of using Arrays
Advantages
- We can store multiple elements items of same data type.
- Random access of the element. Accessing an element from an array takes O(1) time.
Disadvantages
- We cannot use an array to store the elements of different data types.
- The size of the array is fixed to hold its content. The memory allocated cannot be increased or decreased.
FAQ on Arrays
How to add, subtract and multiply two arrays in python
To add, subtract or multiply elements in arrays, the length of the arrays should be the same. In the below code, we use a, d and p to store the sum, difference, and product of arr1 and arr2.
a = [1,2,3]
b = [4,5,6]
s = []
d = []
p = []
for i in range(len(a)):
s.append(a[i]+b[i])
d.append(a[i]-b[i])
p.append(a[i]*b[i])
print(s)
print(d)
print(p)
Output –
[5, 7, 9]
[-3, -3, -3]
[4, 10, 18]
How to check if two arrays are equal in python
We can use ‘==’ operator to check the equality of arrays.
a = [1,2,3]
b = [1,2,3]
c = [1,2,4]
print(a==b)
print(a==c)
Output –
True
False
How to create a list of arrays in python
Elements of a list can be heterogeneous. So, we can use the following method to create a list of arrays.
list1 = [[1,2],[3,4],[5,6,7,8]]
How to slice arrays in python
Slice operator[ : ] can be used to slice arrays/lists in python.
a = [1,2,3,4,5]
print(a[0:3])
print(a[1:])
print(a[:2])
Output–
[1, 2, 3]
[2, 3, 4, 5]
[1, 2]
How to access lists of arrays in python
Lists of an array can be accessed using their indices.
list1 = [[1,2],[3,4],[5,6,7,8]]
print(list1[0], list1[1], list1[2])
Output–
[1, 2] [3, 4] [5, 6, 7, 8]
How to map two arrays in python
In the below code, we zip the lists together, and create a dictionary for zipped lists.
arr1 = [0,0,1,2,3]
arr2 = [4,4,6,8,7]
map12 = dict(zip(array_1, array_2))
print(map12)
Output–
{1: 4, 2: 5, 3: 6}
How to put multiple arrays in a list in python
We can use the append keyword to add a list to another list.
a = []
a.append([1,2,3])
a.append([4,5])
print(a)
Output–
[[1, 2, 3], [4, 5]]
How to shuffle two arrays in same fashion python
You cannot shuffle multiple arrays in a similar fashion. But you can reassign elements of your choice to similar indices.
Why python arrays are called list
There’s no definite data type for arrays in python, so we use lists for the same which serves its purpose as an array. For small data, lists can be used as arrays. But for large data, to make the manipulation of the arrays easier, NumPy is preferred over lists.
How to create an array of empty arrays python
append() keyword with empty entities can be used to create an element of an empty list/array.
a = [1,2,3]
a,append([])
print(a)
Output–
[1, 2, 3, []]
Python indices where 2 arrays match
Indices are independent of whether the arrays match or not
a = [1,2,3,4]
b=[5,6,7,4]
for i,j in a,b:
if i==j:
print('Similar')
How to write to 2d arrays in python
The 2D array is nothing but an array of several arrays. To access the elements in a 2D array, we use 2 variables, one for the row and another for the column.
a = [[1,2,3],[4,5,6],[5,6,7]]
for i in a:
for j in i:
print(j,end='')
print()
Output–
123
456
567
How to construct large arrays python
To construct large arrays, lists can be used but NumPy is more efficient and easy to work with.
from numpy import np
a = [0,1,2,3,4,5,6,7,8,9,10,11,12]
b = np.arange(13)
print(a,b)
Output–
[0, 1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11, 12] [ 0 1 2 3 4 5 6 7 8 9 10 11 12]
Python how to combine two truth arrays
Truth arrays cannot be combined as it just indicates the equivalence of arrays.
Python how to check the intersection of two arrays
In the following code, elements of a are compared with each element in b and if the element exists in both the arrays, it is stored in an array.
a = [1,2,3,4,5]
b = [4,5,6,7,8]
c = [i for i in a if i in b]
print(c)
Output–
[4, 5]
How to create arrays from user input
Elements of an array can be given as input by setting the number of elements and iterating through the range of the number as below.
a = []
n = int(input())
for i in range(n):
a.append(int(input()))
print(a)
What is the difference between arrays and lists python
In the classical sense, lists can be used as arrays, as lists can assume both homogeneous and heterogeneous elements. The difference is, arrays consist of homogeneous elements and lists can consist of heterogeneous elements.
How to know the number of sub arrays in python
The keyword len() can be used to find the number of sub-arrays in an array.
a = [[1,2], [3,4,5]]
print(len(a))
Output–
2
How to clear lists and arrays python 3
The clear() keyword can be used to clear a list/array
a = [[1,2], [3,4,5]]
print(a)
a.clear()
print(a)
Output–
[[1, 2], [3, 4, 5]]
[]
How to read text files in python and convert into int array
If the text file contains strings that purely consist of integers, then the following method can be used.
h = open('integer.txt', 'r')
a = []
for line in h:
a.append(line)
print(a)
How to shuffle arrays in python
shuffle() keyword can be used to shuffle an array.
import random
mylist = [1,2,3,4,5]
random.shuffle(mylist)
print(mylist)
Output–
[2,5,1,4,3]
What is sliding window in arrays in python
The window sliding algorithm is used to find the maximum sum of elements in the array taking a given number of elements as fixed in one iteration. The length of the array should be greater than the fixed length. In the following code, in each iteration, the sum can be calculated by removing the leftmost element and adding the rightmost element to w_sum.
If w_sum is greater than the previously calculated max_sum, it is updated. The process is continued until the end of the array.
def maxSum(arr, k):
# length of the array
n = len(arr)
if n < k:
print("Invalid")
return -1
# sum of first window of size k
w_sum = sum(arr[:k])
# first sum computed
max_sum = w_sum
for i in range(n - k):
w_sum = w_sum - arr[i] + arr[i + k]
max_sum = max(w_sum, max_sum)
return max_sum
# main code
arr = [1, 4, 2, 10, 2, 3, 1, 0, 20]
k = 4
print(maxSum(arr, k))
Output–
24
Indices where arrays are not equal python
!= operator can be used to find the index where the array elements are not equal.
a = [1,2,3,4]
b = [5,2,7,4]
for i in range(len(a)):
if a[i] != b[i]:
print(i)
Output-
1
3
Python how to use a while loop to create arrays
In the following code, we use a while loop to append elements in an array.
a = []
n = int(input())
i = 0
while i < n:
a.append(int(input())) #input elements
print(a) #returns the array of size n
Output-
1
2
3
[1, 2, 3]
How to iterate through two arrays simultaneously python
A single variable can be used for both the arrays to iterate through them simultaneously
a = [1,2,3,4]
b = [5,6,7,8]
for i in range(len(a)):
print(a[i], b[i])
Output–
1 5
2 6
3 7
4 8
Python what structures are faster, lists or arrays
Arrays using NumPy are faster than arrays using lists.
Multiprocessing python why does map return 2d arrays as lists of columns
In multiprocessing python, map returns 2d arrays as lists of columns to picture 2d arrays as matrices.
How to stack two arrays in python
+ operator can be used to combine 2 arrays.
a = [1,2,3,4]
b = [5,6,7,8]
c = a+b
print(c)
Output-
[1,2,3,4,5,6,7,8]
Three arrays and want to see where values are equal python
== operator can be used to index where the elements are equal in 3 arrays.
a = [1,2,3]
b = [4,5,3]
c = [2,4,3]
for i in range(len(a)):
if a[i] == b[i] and a[i] == c[i]:
print(i)
Output–
2
How to build parallel arrays in python
To build parallel arrays, the length of the arrays should be equal. A single variable can be used to iterate through all the arrays simultaneously.
a = ['a','b','c']
b = [1,2,3]
for i in range(len(a)):
print(a[i],':',b[i])
Output–
a : 1
b : 2
c : 3
How to combine two arrays and remove overlapping values python
not in keywords can be used as follows.
a = [1,2,3,4]
b = [1,2,5,6]
for i in b:
if i not in a:
a.append(i)
print(a)
Output–
[1, 2, 3, 4, 5, 6]
How to find the covariance of two arrays in python
Covariance of 2 arrays can be found by covar()
function as follows.
def covar(x, y):
# Finding the mean of the series x and y
mean_x = sum(x)/float(len(x))
mean_y = sum(y)/float(len(y))
# Subtracting mean from the individual elements
sub_x = [i - mean_x for i in x]
sub_y = [i - mean_y for i in y]
numerator = sum([sub_x[i]*sub_y[i] for i in range(len(sub_x))])
denominator = len(x)-1
cov = numerator/denominator
return cov
x = [12,45,23,58]
y = [52,1,35,89]
cov = covar(x, y)
print("Covariance of x and y :", cov)
Output–
Covariance of x and y : 176.5
How to count intersection between two arrays in python
Common elements can be found by using the lambda
function and length can be found by using len()
.
a = [1,2,3,4,5]
b = [4,5,6,7,8]
c = list(filter(lambda x: x in a, b))
print(len(c))
Output–
2
How to access a dictionary of arrays in python
Dictionary of arrays can be accessed by considering values as arrays and keys as key elements.
dict_ab = {'a':[1,2,3],'b':[4,5,6]}
print(dict_ab.keys())
print(dict_ab.values())
Output–
dict_keys(['a', 'b'])
dict_values([[1, 2, 3], [4, 5, 6]])
How to split an array into k number of arrays in python
split() keyword can be used in NumPy library to split an array
import numpy as np
arr = np.array([1, 2, 3, 4, 5, 6])
k =int(input())
newarr = np.array_split(arr, k)
print(newarr)
Output-
4
[array([1, 2]), array([3, 4]), array([5]), array([6])]
How to turn an array of dictionaries into dictionary of arrays in python
It cannot be done as lists are unhashable.
How to split strings to arrays in python
Strings can be split into an array by appending each element into it
a = 'Exampleofastring'
b=[]
for i in a:
b.append(i)
Output–
['E', 'x', 'a', 'm', 'p', 'l', 'e', 'o', 'f', 'a', 's', 't', 'r', 'i', 'n', 'g']
How to apply functions in arrays python
Arrays can be sent as parameters to a function
def fun(x):
print(x)
arr = [1,2,3,4,5]
fun(arr)
Output–
[1, 2, 3, 4, 5]
How to delete parts of arrays in python
An element in an array can be deleted by using pop(_index_)
a = ["a", "b", "c"]
a.pop(1)
print(a)
Output–
['a', 'c']
Convert all the floats in array to integers
int() can be used for each element in the float array as follows
a = [1.2,2.3,3.4,4.5]
b=[]
for i in a:
b.append(int(a))
print(b)
Output–
[1, 2, 3, 4]
Convert all the strings in array to integers
Strings in an array cannot be converted to an integer as both are different data types
Convert all ints in array to floats
float() can be used for each element in the integer array as follows
a = [1,2,3,4]
b=[]
for i in a:
b.append(float(a))
print(b)
Output–
[1.0, 2.0, 3.0, 4.0]
Convert all array of integers to strings
append() keyword can be used to convert an integer array into a string
a = [1,2,3,4,5]
b=''
for i in a:
b.append(i)
Output–
'12345'