Functions are a group of statements that can be executed when we call them. We need to define these statements inside a single block such that whenever there is a need for these statements we can call the block.
Thus we can call this block any number of times without re-writing it. These kinds of blocks are called functions and are declared using a keyword def
.
In the below example, we are performing addition between two numbers by repeatedly writing the same syntax as many times as required.
a = 5
b = 6
print(a + b)
a = 7
b = 2
print(a + b)
a = 12
b = 5
print(a + b)
# Output
11
9
17
This makes the program look lengthy, but using Functions, performing of addition can be placed in a block and that block can be called as many times as required with variable values.
# Function defining
def addition(a, b):
print(a + b)
# Function calling
addition(5, 6)
addition(7, 2)
addition(12, 5)
# Output
11
9
17
From the above two examples, we can notice that the functions make code reusable.
There are two types of functions in Python:-
- In-Built Functions
- User defined Functions
In-Built Functions
The Functions that come along when installing Python software are known as In-Built Functions. These in-built functions can be called from any part of the code based on the requirement. A few examples of In-Built Functions are:-
type()
– returns the datatype of the variable that we pass into itid()
– returns the memory address of the value stored inside memorylen()
– returns the length of the sequence that we pass into itinput()
,eval()
,print()
,str()
,max()
,min()
, etc.,
User-Defined Functions
The Functions that are explicitly defined by the user based on requirements are called User-Defined or Customized Functions.
To define a function we use def
keyword and then followed by the function name. After the function name parenthesis[ () ] are used which are followed by a colon[ : ]. This colon is used to provide indentation for the function body.
Function Naming Rules:-
- Must start with a letter or a underscore and may contain numbers
- Function name should not contain special characters apart from underscore
- Function names are case sensitive. eg:- function1() and Function1() are different
- Function name cannot be a reserved word
Code written inside this indent belongs to the corresponding Function. In the end, based on requirements we might or might not use a return statement. The return statement is used to return a value that is computed by the function.
# Syntax of function
def func():
---
body of function
---
return <some_value>
The def keyword is mandatory to define a function and the return keyword is optional to use.
Let’s write a function to calculate the sum of the values.
def sum(a, b, c, d):
sum_value = a + b + c + d
return sum_value
print(sum(1, 3, 2, 5))
print(sum(3, 75, 12, 234))
# Output
11
324
Function Calling
The function can be called using the function name and a pair of parenthesis. The function can be called only after defining the function. If we try to call the function before defining it, the Python interpreter returns the name not defined error.
# Function Definition
def func1():
return "hi"
# Functon Call
func1()
Function Aliasing
Function aliasing means giving the function another name. Function aliasing is not a function call, it’s rather assigning a new name to the function.
We can assign a new name to the function by assigning the function name to a new name. Thus the function can be called using both the names.
def func():
print("function body")
new_func = func
new_func()
func()
# Output
function body
function body
We can check the memory address of both new_func
and func
using id()
function, it will be the same since one is an alias of the other, and both names point to the same memory address.
print(id(new_func))
print(id(func))
# Output
2408500089040
2408500089040
return statement
The return keyword in python exits the function execution and tells the python interpreter to run the rest of the program.
The return statement is optional but if the user wants to return the value to the store and use it somewhere we can use it. It’s completely based on the user requirement only.
The return statement does not print the value it rather can be used to return the result to the caller.
def mul(x1, x2):
value = x1 * x2
return value
mul_result = mul(2, 4)
print(mul_result)
# Output
8
If we don’t use a return statement then by default the value returned is None.
def mul(x1, x2):
value = x1 * x2
mul_result = mul(2, 4)
print(mul_result)
# Output
None
We can also return multiple values using the return statement. These multiple returned values can be assigned to multiple variables.
def calc(a, b):
sum_value = a + b
sub_value = a - b
mul_value = a * b
return sum_value, sub_value, mul_value
result_sum, result_sub, result_mul = calc(3, 2)
But let’s see internally how the returned values are being assigned to the variables. This happens because of the values being returned in the return statement.
These multiple comma-separated values are represented as a tuple. We can also check this using type() function.
result = calc(5, 6)
print(type(result))
# Output
<class 'tuple'>
Thus these multiple values returned are unpacked based on the number of values that are being assigned to them.
Parameters in Functions
The Parameters in a function are the values we pass into the function. In the function definition if there are any parameters during function definition then definitely we need to pass an equal number of parameters during a function call.
- The parameters that are passed into the function during function definition are called formal parameters
- The parameters that are passed into the function during function call are called actual arguments
- In the below example, –
x1, x2, x3
are called formal parameters anda1, a2, a3
are called actual arguments
def func(x1, x2, x3):
---
body of function
---
return <some_value>
func(a1, a2, a3)
If we fail to pass the parameters that are required by the function then python returns a Type error.
def func(x1, x2, x3):
---
body of function
---
return <some_value>
func(x1)
TypeError: func() missing 2 required positional argument: 'x2' and 'x3'
Positional Arguments
The Positional Arguments in Python are those that are the same in the way of the positional order. Each argument in this positional argument is mapped to the parameter in the function definition in the same order they are declared.
In the below example, the arguments that we pass during function calling are positional arguments and these are mapped to the formal parameters of the function. If we change the order of these arguments the result is going to be different.
def func(f1, f2, f3):
print(f1 + f2 - f3)
func(1, 4, 2)
func(4, 2, 1)
func(2, 3, 4)
# Output
3
5
1
The number of positional arguments that we pass has to be equal to the number of parameters that the function definition has.
keyword Arguments
If we pass the values using keyword/parameter assigned to them in the function calling itself then they are called keyword arguments.
For the keyword arguments, the position need not be in order. Since we map values to a parameter in the function call itself.
In the below example, we are passing the values into the function using keyword arguments. Even if we change the order of passing these values since the values are already mapped it returns the correct result
def func(name, id):
print(name, id)
func(name = "John", id = "234-471")
func(id = "234-967", name = "Walden")
# Output
John 234-471
Walden 234-967
We can also pass both positional and keyword arguments combined, but the order has to be first the positional arguments, and then keyword arguments have to be passed.
def func(company_name, employee_name, employee_id):
print(f"{company_name}: {employee_name}, {employee_id}")
func("comp1", employee_id = "r985", employee_name = "John")
func("comp2", employee_name = "mike", employee_id = "j9834")
# Output
comp1: John, r985
comp2: mike, j9834
A syntax error will be raised if we pass first keyword arguments and then positional arguments.
def func(company_name, employee_name, employee_id):
print(f"{company_name}: {employee_name}, {employee_id}")
# Invalid function call
func(employee_id = "r985", comp1, "John")
# SyntaxError
positional argument follows keyword argument
Default Arguments
We can assign default values to the parameters in the function which are known as default arguments. If the function call is made without passing any arguments then these default arguments are used.
If we pass positional arguments then instead of default arguments these positional arguments are used as the parameters.
In the below example, we are assigning a default value to the formal parameters of the function.
def func(value = "default_user"):
print(f"{value} logged in")
func()
# Output
default_user logged in
If we pass any value to the function call then instead of the default value that is assigned previously, the values passed in the function call will be used.
def func(value = "default_user"):
print(f"{value} logged in")
func("John")
# Output
John logged in
If we have default and non-default arguments then the order has to be is non-default arguments follow default arguments.
def func(msg, value = "default_user"):
print(f"{value} logged in {msg}")
func("welcome")
func("welcome", "John")
# Output
default_user logged in welcome
John logged in welcome
We should not accept non-default arguments that follow default arguments which might result in a syntax error.
Variable Length Arguments
In Python, we can pass any number of values to the function using variable-length arguments. We can declare a variable-length argument using the symbol( * ).
These variable-length arguments are represented using *variable_name
in the function definition.
def func(*var_args):
Even if we pass zero arguments also function does not throw any error, since the arguments are variable-length arguments.
def func(*var_args):
if len(var_args) == 0:
print("zero arguments passed")
else:
print(var_args)
func()
func(2, 3, 4, "hii")
# Output
zero arguments passed
(2, 3, 4, 'hii')
We can pass any number of arguments to the function in the function call and all these values will be stored as tuples.
To access all these values we can iterate through this tuple using a for-loop.
def addition(*var_args):
if len(var_args) == 0:
print("zero arguments passed")
else:
result = 0
for i in var_args:
result = result + i
print(result)
addition()
addition(2, 3, 6)
addition(-3, -65, -2)
# Output
zero arguments passed
11
-70
We can also pass variable length arguments with positional arguments. The order has to be positional arguments followed by variable-length arguments.
def func(pos_args, *var_args):
print(pos_args)
print(var_args, type(var_args))
func(1, 23, 4)
# Output
1
(23, 4) <class 'tuple'>
If we pass any values after variable-length arguments then we should pass those values as keyword arguments. The order has to be keyword arguments follows variable length arguments.
def func(*var_args, kw_args):
print(var_args)
print(kw_args)
func(1, 23, a = 4)
# Output
4
(1, 23)
*args and **kwargs
To pass variable length arguments into the function *args
and **kwargs
are used. *args
is used to variable-length values into the function and **kwargs
is used to send variable-length keyword arguments.
In the **kwargs
values are sent in the form of key-value pairs. These key-value pairs are stored in a dictionary.
def func(**kwargs):
print(kwargs, type(kwargs))
func(company = "com1", emplyee_name = "john")
# Output
{'company': 'com1', 'emplyee_name': 'john'} <class 'dict'>
If we don’t send any key-value pairs then an empty dictionary is returned.
def func(**kwargs):
print(kwargs, type(kwargs))
func()
# Output
{} <class 'dict'>
We can also send combined *args
and **kwargs
in the function call. The *args
are basically positional arguments and are stored in the tuple.
The **kwargs
are keyword arguments and are stored in a dictionary. While passing both *args
and **kwargs
the positional arguments are followed by keyword arguments.
def func(*args, **kwargs):
print(args, kwargs)
func("john", "male", age = 60)
func("smiley", gender = "female", age = 45)
# Output
('john', 'male') {'age': 60}
('smiley',) {'gender': 'female', 'age': 45}
Global and Local Variables in Python
Local Variables in Python
Local variables are those that are bound to only a specific part of the program. The scope of the local variable is limited. A local variable declared inside a function cannot be used in other functions or outside of that function. If we try to use the local variable outside its scope it throws an error.
def func1():
a = 'hi'
print('local variable:', a)
func1()
print('local variable:', a)
def func2():
print('local variable:', a)
func2()
# Output
local variable in func1: hi
File "C:\Users\file.py", line 8, in func2
NameError: name 'a' is not defined
Global Variables in Python
Global variables are those which can be accessed throughout the entire program. These variables can be used even inside any function that has been declared after the variable is created because they are accessible to any part of the program.
glob_var = 5
def func():
print('global variable:', glob_var)
func()
# Output
global variable: 5
Keyword global
Generally, a global variable is available to all the functions in the program, and any change in the value of the global variable inside a function does not affect the value outside the function.
In the below example, test_var
is a global variable, and changes made to the test_var
in func1 do not reflect in func2. Because the test_var
inside func1 is local and will be limited to that particular function itself.
test_var = 5
def func1():
test_var = 'hi'
print("value in func1 :", test_var)
func1()
def func2():
print("value in func2 :", test_var)
func2()
# Output
value in func1 : hi
value in func2 : 5
To change the scope of the test_var
in func1 into a global we can define the variable as a global variable using the global
keyword. Once it is defined as a global variable we can use it across other parts of the program.
test_var = 5
def func1():
global test_var
test_var = 'hi'
print("value in func1 :", test_var)
func1()
def func2():
print("value in func2 :", test_var)
func2()
# Output
value in func1 : hi
value in func2 : hi
Note:- When a variable is accessed before declaring it as global then, the python interpreter throws a syntax error.
test_var = 5
def func1():
print(test_var)
global test_var
test_var = 'hi'
print(test_var)
func1()
# SyntaxError
name "test_var" is used prior to global declaration
We can check the scope of the variable using inbuilt methods in python. globals()
and locals()
return whether a variable is defined globally or locally. globals()
and locals()
are dictionaries that contain all their respective global and local variables.
- All the variables and their values are stored as a dictionary key and value pairs in
globals()
if it’s a global variable. - local variables and their values are stored in
locals()
dictionary as key-value pairs.
Basically, if we try to get a global value of a local variable we can use globals()
dictionary using globals().get()
.
test_var = 5
def func1():
test_var = 'hi'
print(globals().get("test_var"), test_var)
func1()
# Output
5 hi
In the below code, we have defined x as a global variable and y as a local variable. Since x is globally defined we can use it in different functions and check its scope, whereas variable y is a local variable thus we have to check its scope within the function.
def func1():
global x
y = 5
x = 'hi'
print(x)
if "y" in locals():
print("y is local")
func1()
def func2():
if "x" in globals():
print("x is global")
func2()
# Output
hi
y is local
x is global
Nested Functions
A function inside another function is called a nested function. The control flow will be first the outer function has to be called and once the execution of outer function has started at some point the inner function is called.
Thus both the functions are executed based on the requirement.
In the below example we can directly call the outer function and since the inner function is local to the outer function it cannot be called from outside of the outer function.
The interpreter throws an error if we call an inner function from outside of the outer function.
def outer():
print("outer 1")
def inner():
print("inner 1")
print("outer 2")
outer()
inner()
# Output
outer 1
outer 2
NameError: name 'inner' is not defined
Thus the inner function has to be called from inside of the outer function. Below are two examples for calling the inner function.
def outer():
print("outer 1")
def inner():
print("inner 1")
print("outer 2")
inner()
outer()
# Output
outer 1
outer 2
inner 1
def outer():
print("outer 1")
def inner():
print("inner 1")
print("outer 2")
return inner
func = outer()
func()
func()
# Output
outer 1
outer 2
inner 1
inner 1
Recursive Functions
A Recursive function is a function that calls itself during its execution. The process may repeat several times such that the result is returned at the end of each iteration. The recursive function is always made up of two blocks, the base case and the recursive case.
Recursive functions reduce the length of the code and make look it simpler. Recursion also solves many complex problems in a simple way.
Please click the link for a complete tutorial on Recursions in Python
In the below example, we are calculating the factorial of a number using the function recursion. The function is calling itself until a certain condition is achieved.
def recursion(n):
if n == 0:
result = 1
else:
result = n * recursion(n-1)
return result
print(recursion(6))
# Output
720
filter() in Python
filter()
function is used to filter the values of a sequence based on the condition. If the items of the sequence pass the condition then they are stored in a filter object.
filter()
takes a function and a sequence as parameters, these items of the sequence are passed to the function and filtered.
filter(function, iterable_sequence)
For example, we can pass a list of numbers to a function that returns True if the number is even and returns False if the number is odd. When the function returns True that particular item is considered as even and stored in the filter object. Thus the values are filtered.
def even_or_odd(n):
if n % 2 == 0:
return True
else:
return False
result = filter(even_or_odd, [1, 2, 3, 98, 123])
print(result)
# Output
<filter object at 0x0000028D39FFF610>
If we like to store the result in a list or a tuple then we can type-cast the filter object using list()
, tuple()
. But if the items can be updated if it’s a list and cannot be modified if it’s a tuple.
print(list(result))
# Output
[2, 98]
If we want to remove the duplicates in the result then we can type-cast into the set using set()
.
result = filter(even_or_odd, [46, 122, 3, 98, 122, 47, 46, 98, 98])
print(set(result))
# Output
{122, 46, 98}
We can also pass lambda functions into filter()
that can filter a sequence and return an even or odd number based on the requirement.
The expression that we need to pass as a condition into the lambda function is number % 2 == 0
to return even numbers and number % 2 != 0
to return odd numbers.
list_sequence = [24, 12, 56, 23, 34, 77, 49]
print(list(filter(lambda n: n%2 == 0, list_sequence)))
print(list(filter(lambda n: n%2 != 0, list_sequence)))
# Output
[24, 12, 56, 34]
[23, 77, 49]
map() in Python
map()
function is used to apply a function over all the items of the iterable sequence. It takes a function and an iterable sequence as parameters.
map()
returns a map object that contains values that are returned by passing the items of the sequence to the function.
map(function, iterable_sequence)
We can calculate the square of all the numbers in a list using the map() function. We pass a predefined function that returns the square of a number( n ) and a sequence of numbers in a list.
def sq_num(n):
return n*n
list_sequence = [1, 2, 3, 4, 5]
print(map(sq_num, list_sequence))
# Output
<map object at 0x0000019A8428E250>
We can type-cast the map object into a mutable list using the list()
function.
print(list(map(sq_num, list_sequence)))
# Output
[1, 4, 9, 16, 25]
If we would like to get an immutable sequence so that the items cannot be modified later then we need to pass the map object into a tuple()
.
print(tuple(map(sq_num, list_sequence)))
# Output
[1, 4, 9, 16, 25]
To remove the duplicate values that are present in the object we can type-cast the map object into a set.
list_sequence = [5, 1, 6, 5, 1, 7, 5]
print(set(map(sq_num, list_sequence)))
# Output
{25, 36, 1, 49}
We can also pass lambda functions into map()
that can apply the items of the sequence over an expression and return the values in a map object.
To calculate the square of a number we need to pass number( n ) as argument and number * number as expression.
list_sequence = [1, 2, 3, 4, 5]
print(list(map(lambda n: n*n, list_sequence)))
# Output
[1, 4, 9, 16, 25]
If we like to multiply the corresponding items of two lists then we can pass these two lists into the map object that can pick corresponding values of the two lists and multiply them.
lambda function can easily calculate the multiplication and return the result to store it in the map object.
If lists are of variable lengths then the number of values returned is equal to the length of the smaller list.
list_1 = [1, 2, 3, 4, 5]
list_2 = [5, 6, 7, 8, 9, 10, 12, 14]
print(list(map(lambda l1, l2: l1 * l2, list_1, list_2)))
# Output
[5, 12, 21, 32, 45]
reduce() in Python
reduce()
function reduces the sequence of items into a single item by applying a function on the sequence. It accepts a function and a sequence as parameters.
Unlike filter()
and map()
, the reduce()
function is not an in-built function so it has to be imported from functools
When we perform an addition operation then from the sequence every time two items are picked in an order to add them. The result is thus added to the addition result of the next two items and so on. the process is carried out till all the items are added.
from functools import *
list_1 = [1, 2, 3, 4, 5]
def addition(x, y):
return x + y
print(reduce(addition, list_1))
# Output
15
The same program can be coded using lambda functions in a single line. Since lambda functions take variables and an expression, we need to pass two variables as arguments because reduce function picks two values at a time.
Similarly, we can perform the multiplication of all the items in the sequence.
from functools import *
list_1 = [1, 2, 3, 4, 5]
print(reduce(lambda x, y : x + y, list_1))
print(reduce(lambda x, y : x * y, list_1))
# Output
15
120
FAQ’s in Functions
How to call a function in python?
The function can be called using the function name and a pair of parenthesis. The function can be called only after defining the function. If we try to call the function before defining it, the Python interpreter returns the name not defined error.
# Function Definition
def func1():
return "hi"
# Functon Call
func1()
How to define a function in python?
To define a function we use def
keyword and then followed by the function name. After the function name parenthesis[ () ] are used which are followed by a colon[ : ]. This colon is used to provide indentation for the function body.
# Function Definition example
def func_1():
<statement_1>
<statement_2>
----
What is a function in python?
Functions are a group of statements that can be executed when we call them. We need to define these statements inside a single block such that whenever there is a need for these statements we can call the block.
How to call a function within a function python?
Function within a function is called nested function and the inner function can be called from the body of the outer function only. If we try to call the inner function from outside of the outer function then the interpreter returns an error.
def outer_func():
print("outer 1")
def inner_func():
print("inner 1")
inner_func()
outer_func()
# Output
outer 1
inner 1
How to exit a function in python?
We can exit a function in several ways. Such as we can use a return
statement that ends the execution of the function and passes the control back to the statements that are outside of the function.
Apart from the return statement, there are a few more methods that can exit the function.
exit()
,quit()
,sys.exit()
,os._exit()
def func():
print("hii")
exit()
print("bye")
func()
# Output
hii
def func():
print("hii")
quit()
print("bye")
func()
# Output
hii
import sys
def func():
print("hii")
sys.exit("exit")
print("bye")
func()
# Output
hii
SystemExit: exit
How to pass a function as a parameter in python?
To pass a function as a parameter we have in-built methods in python that accepts a function and a sequence as parameters. The items of the sequence are passed to the function that we have passed. Based on requirements we can use filter
, map
, reduce
, etc.,
# Using filter()
filter(func_1, seq_1)
# Using map()
map(func_2, seq_2)
Using reduce()
from functools import *
reduce(func_3, seq_3)
How to pass a list to a function in python?
We can pass a list using the map()
function that accepts a function and an iterable sequence as parameters and maps all the items of the list to the function.
# returns square of all the values of the list
def sq_func(n):
return n * n
my_list = [1, 2, 3, 4, 5]
print(list(map(sq_func, my_list)))
# Output
[1, 4, 9, 16, 25]
How to import a function in python?
To import a function we need to use the file name followed by which function needs to be imported from that file.
from file_1 import func1, func2
---
---
How to use the strip function in python?
.strip()
function in python is used to remove the trailing spaces that are at the start and end of the string.
We can also customize it by passing a sub-string into the strip function. If there are any sub-string in the start and end of the string then they are removed.
my_string = "@@ hello world @@"
print(my_string.strip("@"))
# Output
hello world
How to use split function in python?
.split()
function returns a list of the words in the string, using sep
as the delimiter string. The delimiter that we pass is used to split the string.
Once the string is split using the delimiter all the sub-strings that are split are stored in a list.
my_string = "hiii, welcome world, lets work out, wonders"
print(my_string.split(","))
# Output
['hiii', ' welcome world', ' lets work out', ' wonders']
How to use the round function in python?
round()
function is used to round a number to a given precision in decimal digits.
decimal_number = 453.973
print(round(decimal))
# Output
454
How to call a variable from another function python?
Variables that are defined in a function are locally bound to that particular function only. If we want to use the same variable in another function then the variable has to be declared as a global variable.
A variable can be declared as a global variable using a global
keyword.
def func1():
global test_var
test_var = 'hi'
func1()
def func2():
print(test_var)
func2()
# Output
hi
What is a lambda function in python?
Lambda Function also referred to as ‘Anonymous function’ is the same as a regular python function but can be defined without a name. In python, normal functions are defined using the def
keyword, whereas the anonymous functions are defined using the lambda
keyword.
new_func = lambda x, y : x * y
print(new_func(4, 5))
# Output
20
How to use the input function in python?
input()
function in python allows the user to provide input to the program. Basically whatever the user enters will be in string format only, but we can type-cast the input based on the requirement.
user_input = input()
print(user_input, type(user_input))
# Input # Output
345 345 <class 'str'>
hii hii <class 'str'>
What does the range function do in python?
The range is an in-built function that takes integers as parameters and returns a sequence of integers.
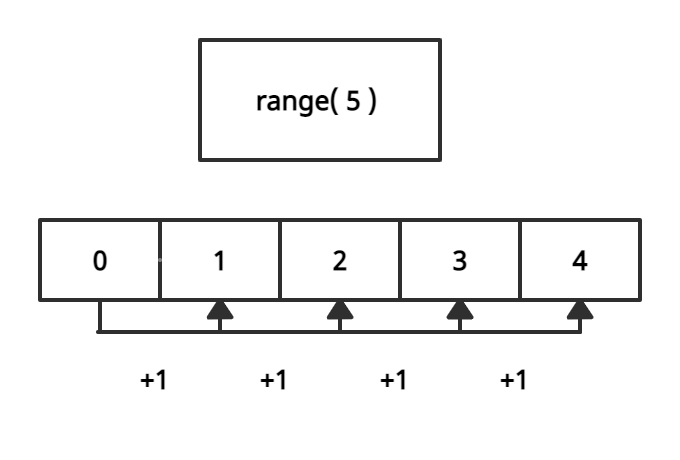
my_list = list(range(0, 6))
print(my_list)
# Output
[0, 1, 2, 3, 4, 5]
How to use the max function in python?
max()
function in python returns the maximum value that is present in a sequence. If the sequence is a list the highest value is returned and so on.
In the case of strings, their ASCII values are compared to find the maximum value among them.
my_list = list(range(0, 6))
my_string = "ABCdef"
print(max(my_list))
print(max(my_string))
# Output
5
g
What is the len function in python?
len()
function is used to return the count of the items that are in a sequence. The sequence can be a string, list, set, or tuple.
my_list = [0, 1, 2, 3, 4, 5]
print(len(my_list))
# Output
6
How to make a square root function in python?
We can pass a number whose square root has to be calculated to a function that calculates the square root using a math module.
import math
def sq_root(n):
return math.sqrt(n)
print(sq_root(5))
# Output
5.0
How to use the sum function in python?
sum()
function returns the result that is obtained by adding all the values that are present in an iterable.
my_list = [1, 24, 12, 677]
sum(my_list)
# Output
714
What does the map function do in python?
map()
function is used to apply a function over all the items of the iterable sequence. It takes a function and an iterable sequence as parameters.
map()
returns a map object that contains values that are returned by passing the items of the sequence to the function.
[1, 2, 3, 4, 5]
print(list(map(lambda n: n*n, list_sequence)))
# Output
[1, 4, 9, 16, 25]
What does zip function do in python
zip()
is a python in-built function to iterate over two iterables simultaneously. It stores the items at equal positions as a tuple. Thus forms a set of tuples as a sequence to be iterated.
zip_obj1 = [1, 2, 3]
zip_obj2 = [1, 2, 5]
print(list(zip(zip_obj1, zip_obj2)))
# Output
[(1, 1), (2, 2), (3, 5)]
How many parameters can a function have in python?
A function can have parameters that are equal to a number of formal parameters that are declared in the function header. We can also use *args
so that we can pass a variable number of arguments.
What is a recursive function in python?
A Recursive function is a function that calls itself during its execution. The process may repeat several times such that the result is returned at the end of each iteration.
def recursion(n):
if n == 0:
result = 1
else:
result = n * recursion(n-1)
return result
print(recursion(6))
# Output
720
How to keep count in a recursive function python?
We can create a court variable that keeps on incrementing when the function is executed. For each execution of the function, the value of count is incremented by 1.
The count variable has to be made global
or else the value will remain local and will not reflect while printing count value at the end of the program.
count = 0
def recursion(n):
global count
if n == 0:
result = 1
else:
result = n * recursion(n-1)
count = count + 1
return result
recursion(6)
print(count)
# Output
7
In python, which function would capitalize the first character of each word for a string?
.title()
function in python capitalizes the first letter of each word in a string.
string = "hello world welcome to python"
print(string.title())
# Output
Hello World Welcome To Python
What does the def function do in python?
To define a function we use def
keyword and then followed by the function name. Whenever a Python interpreter encounters a def keyword it considers it as a function.
def func(arg1, arg2):
<statement_1>
<statement_2>
---
How to use format function in python?
Using .format()
returns a formatted string and we can customize the string with other strings or variables inside a pre-defined text.
The string format is done using a pair of curly braces inside a text. This text is then called using the .format()
method in which we can pass the variable or strings into it in an order in which curly braces are defined.
print("Hello, i am using python {}".format(3.7))
# Output
Hello, i am using python 3.7
How to use the count function in python?
count()
function accepts a substring as a parameter and checks how many times the sub-string has non-overlapping occurrences in the main string. Optional arguments start and end are interpreted as in slice notation.
S = "abcdabcdabcd"
S.count("ab") -> 3
S.count("ab", 0, 7) -> 2
How to use type function in python?
type()
function in python returns the datatype of the parameter that we pass into it.
my_list = [1, 2, 3]
print(type(my_list))
# Output
<class 'list'>
What does the append function do in python?
.append()
function appends the item into the list. It does not return a new list it updates the existing list with the item that we pass into it. This operation is similar to that of the concatenation operation.
my_list = [1, 2, 3]
my_list.append(5)
print(my_list)
# Output
[1, 2, 3, 5]
In python, how to change global variable in function?
We can change a variable into global using the global
keyword. This global variable can then be used in any function.
def func():
global new_variable
How to pass arguments in python function?
We can pass the arguments into the function using a function call. If the arguments are positional then they are mapped to the respective parameters in the function. If the arguments are keyword arguments then they will be directly used in the function.
The order has to be positional arguments followed by keyword arguments.
def func(company_name, employee_name, employee_id):
print(f"{company_name}: {employee_name}, {employee_id}")
func("comp1", employee_name = "mike", employee_id = "j9834")
# Output
comp1: mike, j9834
What does the float function do in python?
float()
is an in-built function in python and accepts a number as a parameter. It converts the number into a floating-point data type.
num = 23
print(float(num))
# Output
23.0
What is str function in python?
str()
is an in-built function in python and returns data converted into string type. It accepts a variable or a value as a parameter.
num = 112
print(str(num))
# Output
'112'
How to write lambda function in python?
Lambda functions are defined using lambda
keyword and the function evaluates an expression using the arguments that we pass into it.
The arguments and the expression are separated using a colon( :
). To the left of the colon, arguments are declared and to the right of the colon, expression is declared.
lambda value_1, value_2 : value_1 + value_2
What is the int function in python?
int()
is an in-built function in python that converts a numerical value into an integer. If a number is in string format then using the int()
function we can convert it into integer data type.
If a numerical value is in floating-point we can convert it into the integer type. Thus different data types such as boolean, bytes can be converted into integers.
int("234") -> 234
int(22.65) -> 22
What is a pop function python?
pop()
function removes the last element from a list or set. It also returns the value before removing it from the sequence. In the case of a set randomly one item will be returned and removed since the set is an unordered data type.
my_list = [1, 2, 3]
my_set = {1,2, 3, 4}
print(my_list.pop(), my_set.pop())
# Output
3, 1
How to apply a function to elements in a list in python?
If we like to apply a function over a list of elements we can use the map()
function that maps each element to the function and stores the result in a map object. We can type-caste it into a list to view the result.
In the below example, we are using the inbuilt function int()
to convert values into the integer type.
my_list = ["1", "2", "3"]
print(list(map(int, my_list)))
# Output
[1, 2, 3]
How to pass the function as argument in python?
We can pass a function as an argument to another function and call them in the body of the function. This can be done in several ways such as a high-order function where a function accepts other functions as parameters.
Also, there are wrapper functions that take other functions as parameters. These wrapper functions are also called Decorators.
How to rename a function in python?
We can rename the function into another name and is called function aliasing. The Function renaming is different from the function call.
If a function is renamed then it can be called using a new name and also with an old name.
def Sum(x, y):
return x + y
addition = Sum
print(addition(2, 3))
# Output
5
What is the return type of function ‘id’ in python?
id()
is a built-in function and returns the identity of an object. It returns the memory address of the variable that we pass into it. The return type of the value it returns is in integer format.
value_num = 341
result = id(value_num)
print(type(result))
# Output
<class 'int'>
What do function by default return in python?
By default, functions in python return None. Unless we provide a return statement in the function it always returns None.
def addition(x, y):
result = x + y
print(addition(2, 3))
# Output
None
What is the help function in python?
help()
function takes any module or keyword or topic as a parameter and returns documentation that has all the details. Each module also comes with a one-line summary of what it does.
help("str")
help("dict")
What is an __init__ function python?
__init__()
is a reserved method in python classes. It is also called the constructor of the class. The constructors are used to initialize the state of the object. The task of constructors is to initialize the data members of the class when an object of the class is created.
What is the purpose of type function in python?
type()
is an in-built function in python and returns the data type of the parameter that we pass into it. Generally, type()
function is mostly used for debugging purposes. When we don’t know the type of the object and if we try to associate the objects with a few methods it might throw an error.
In such cases type() is used to know the data type of the object.
value_1, value_2 = 4, "hi"
print(type(value_1), type(value_2))
# Output
<class 'int'>, <class 'str'>
In python .find function which finds the second appearence in a string?
Basically .find()
function in python returns the index of the first appearance of a substring in a string. If an index is provided then the find() function starts a search of the sub-string from the index that we passed.
Thus first, we find the first occurrence of the sub-string and pass the index returned into find()
again, such that the second appearance of the sub-string is returned.
string = "Hello world, Welcome"
index_1 = string.find("el")
index_2 = string.find("el", index_1+1)
print(index_2)
# Output
14
What does the *args do in a python function and how might you use it?
In Python, we can pass any number of values to the function using variable-length arguments. We can declare a variable-length argument using the symbol( * ).
These variable-length arguments are represented using *variable_name
in the function definition. We can iterate through all the values passed using a for loop.
def addition(*var_args):
if len(var_args) == 0:
print("zero arguments passed")
else:
result = 0
for i in var_args:
result = result + i
print(result)
addition(2, 3, 6)
# Output
11
What does the ** function do in python?
**kwargs
is used to send variable-length keyword arguments. In the **kwargs
values are sent in the form of key-value pairs. These key-value pairs are stored in a dictionary.
def func(**kwargs):
print(kwargs, type(kwargs))
func(company = "com1", emplyee_name = "john")
# Output
{'company': 'com1', 'emplyee_name': 'john'} <class 'dict'>
Why does python say return is outside function?
When we declare the return
statement outside of the function then SyntaxError is raised with “return” outside function error. To solve this error, we need to declare the return statement inside the body of the function.
def addition(x, y):
result = x + y
return result
print(addition(2, 3))
# SyntaxError
"return" outside function
What is the staticmethod function python?
A static method is a method that knows nothing about the class or the instance it was called on. It just gets the arguments that were passed, no implicit first argument. Instead of static methods, we can also use module functions.
How to call function of class in python?
To call a function of a class we need to use the instance object of the class. Also, the first parameter of the class function has to be self.
class MathOperations:
def __init__(self, x, y):
self.x = x
self.y = y
def Addition (self):
return self.x + self.y
class_obj = MathOperations(2, 3)
print(class_obj.Addition())
# Output
5
Which statement in python is optional when writing a function?
The return statement is optional but if the user wants to return the value to the store and use it somewhere we can use it. It’s completely based on the user requirement only.
The return statement does not print the value it rather can be used to return the result to the caller.
How many returns are allowed in a python function ?
In function, only one returned is allowed. In case if we declare multiple return statements then the first occurring return statement is considered and the rest of them are ignored by the interpreter.
def addition(x, y):
return x * y
return x + y
print(addition(2, 3))
# Output
6
How does end= in python actually function?
The end parameter is used to append any string at the end of the output of the print statement in python. By default, the print method ends with a newline.
for i in range(3):
print(i, end = ",")
# Output
0,1,2,
What is the difference between function and method in python?
- Methods definition are always present inside the class
- Methods are dependent on class they belong to
- Python method is called upon a object
- A Method can alter the state of the object
- To define a function we dont need a class
- Functions are independent entities in a program
- Functions are called quite generically, they are not called on any object
- Function operates on data that we pass and returns the result
min() function to identify which of the two is small python?
min()
is an in-built function in python that returns the smallest item that is in a sequence. It takes a sequence of items as a parameter and returns the smallest value.
min(3, 4, 5) --> 3
In python, name not defined when calling function?
When a function is created it has to be called only after defining the function. If we try to call the function before its definition then the name not defined error is returned.
func()
def func():
print("hi")
# Output
NameError: name 'func' is not defined
In python, what is the return data type of an input function?
The return type of input()
function is always in string format. This can be type-casted based on the requirement.
user_input = input()
print(type(user_input))
# Input # Output
hii <class 'str'>
3325 <class 'str'>
How to use the “in” function in python?
in
is a keyword that belongs to membership operators. It helps us check the contents of an object and returns True if a value is present in the object or else returns False.
We can use in
to check whether the given object present in the given collection. (It may be String, List, Set, Tuple OR Dict).
a = [1, 2]
b = 2
print(b in a)
True
How to call trig function using math module python?
Trigonometric functions can be loaded into a program using a math module. There are several in-built trigonometric functions in the math module such as sin()
, cos()
, tan()
, etc.,
All these functions can be called using the math module as shown below.
import math
print(math.sin(4))
print(math.tan(-1))
# Output
-0.7568024953079282
-1.5574077246549023
What type of python function has a yield statement?
In python, the yield statement is used in generators. Generators are iterative functions, that return the iterated value when they are called. We can use multiple yield statements in a generator function.
def func():
---
---
yield <statement_1>
---
---
yield <statement_2>
---
In python, how to make function wait 2 seconds
We can make a function wait for 2 seconds or more using the time module. The time module has a method known as sleep() which takes a number of seconds for programs have to sleep.
If we declare this statement inside a function then the function sleeps for the particular amount of time that we pass into it.
import time
def func():
time.sleep(2)
return "function sleep"
print(func())
How to restart function python?
We can call the function from inside of the function itself which makes the function restart the execution. But the call inside the function has to be associated with a condition such that only in the particular case it gets restarted.
def func(a):
if a>5:
func(3)
exit()
print(a*a)
func(10)
# Output
9
How to use upper function in python?
upper()
is an in-built string function that returns a copy of the string converted to uppercase.
s = "HellO HAI"
print(s.upper())
# Output
HELLO HAI
In python, track how many time the function get called?
We can create a court variable that keeps on incrementing when the function is executed.
The count variable has to be made global
or else the value will remain local and will not reflect while printing count value at the end of the program.
count = 0
def func(n):
global count
result = n*n
count = count + 1
return result
func(6)
func(5)
func(2)
func(8)
print("number of times function called = ", count)
# Output
number of times function called = 4
What does enumerate function do in python?
enumerate()
in python takes a sequence as a parameter and provides a counter to each element of the sequence. Each element is stored along with its counter as an index in a tuple.
list_seq = ["a", "b", "c", "d"]
for i in enumerate(list_seq):
print(i)
# Output
(0, 'a')
(1, 'b')
(2, 'c')
(3, 'd')
What is ord function in python?
ord()
is an in-built function in python that converts a character into its Unicode. Any character of length 1 returns the Unicode value(ASCII value) of the character.
ord("a") -> 97
ord("d") -> 100
ord("K") -> 75
How do you use the ‘exp’ function in python?
exp()
is a method in the math module that returns the value obtained by calculating the e
raised to the power of the parameter that we pass into it. It returns a floating-point number.
import math
print(math.exp(6))
print(math.exp(12))
print(math.exp(-5))
# Output
403.4287934927351
162754.79141900392
0.006737946999085467
How to create a factorial function in python?
We can create a factorial function by using Function recursion, where the function calls itself by having a condition and returns the factorial of a number
def recursion(n):
if n == 0:
result = 1
else:
result = n * recursion(n-1)
return result
print(recursion(6))
# Output
720
How to write a nested function in python?
A function inside another function is called a nested function. We can declare an outer function and in the body of the outer function, we can define a nested function.
We can directly call the outer function and since the inner function is local to the outer function it cannot be called from outside of the outer function and has to be called from the body of the outer function.
def outer():
print("outer 1")
def inner():
print("inner 1")
print("outer 2")
inner()
outer()
# Output
outer 1
outer 2
inner 1
How to get list of available function in python
dir()
command is used to get all the available functions in a module. It takes a module name as a parameter and returns the list of all available functions.
dir("str")
['__add__', '__class__', '__contains__', '__delattr__',
----
---
'__doc__', '__eq__', '__format__', '__ge__',
-----
'title', 'translate', 'upper', 'zfill']
How to time a function in python?
We can use time()
module and calculate the start time of function execution and remove it from the end time of function execution which returns the time taken to complete the execution of the function.
from datetime import datetime
def func(a, b):
print(a + b)
print(a - b)
print(a * b)
end_time = datetime.now()
return (end_time - start_time)
start_time = datetime.now()
print("time taken = ",func(4, 5))
# Output
9
-1
20
time taken = 0:00:00.036308