Python operators are the special symbols that carry out arithmetic or logical operations between two or more operands. These operands can be values or variables. There are various types of operators available and based on their operations we can categorize them into the following classes:-
- Arithmetic Operators
- Relational or Comparision operators
- Logical Operators
- Bitwise Operators
- Assigment Operators
- Special Operators
Arithmetic Operators
Arithmetic Operators are used to performing basic arithmetic operations such as
- Addition ( + )
- Subtraction ( – )
- Multiplication ( * )
- Division ( / )
- Floor Division ( // )
- Modular Division ( % )
- Exponent Operator or Power operator ( ** )
Addition Operator ( + )
Useful for adding two operands and gives the result of the addition. The result will be an integer and if a float number is involved it returns a floating-point number.
add_1 = 3
add_2 = 5
add_3 = 5.0
print(add_1 + add_2)
print(add_1 + add_3)
# Output
7
8.0
Addition Operator can also be used to concatenate two strings, lists. This concatenation is allowed only between two strings or two lists.
str_1 = "pyt"
str_2 = "hon"
print(str_1 + str_2)
print(str_1 + 3)
# Output
'python'
#str_1 + 3
TypeError: can only concatenate str (not "int") to str
Subtraction Operator ( – )
Subtraction is an arithmetic operation that represents the operation of removing an object from a collection. The result of subtraction will be integer unless a float number is involved in subtraction it returns a floating-point number.
sub_1 = 5
sub_2 = 3
print(sub_1 - sub_2)
# Output
2
Multiplication Operator ( * )
Multiplication Operator performs mathematical multiplication between the operands. It is denoted by *
mul_1 = 7
mul_2 = 6
print(mul_1 * mul_2)
# Output
42
Multiplication operators can also be used to perform string multiplication. We cannot use String to multiply with a string rather we can print a string for a specific number of times.
print("Python" * 3)
# Output
"PythonPythonPython"
Division Operator ( / )
Division Operators perform arithmetic division between the numerator and denominator. Unlike the other operators, a division operator result is a floating-point number.
div_1 = 6
div_2 = 2
print(div_1 / div_2)
# Output
3.0
If we try to divide any number with zero, logically it’s infinite and throws an error.
print(5/0)
ZeroDivisionError: division by zero
Floor Division ( // )
Floor division returns the lower possible integer using the result of the division thus eliminates decimal points. Floor division can perform both integral and floating-point arithmetic.
Floor division returns an integer number if both numerator and denominator are integers and returns floating-point number if either of numerator or denominator is float number.
var_1 = 7
var_2 = 5.3
var_3 = 2
print(var_1 // var_3)
print(var_2 // var_3)
# Output
3
2.0
Modular division ( % )
Modular division returns remainder as a result obtained by performing division between two operands
print(5 % 2)
# Output
1
Exponent or Power Operator ( ** )
Used to multiply the number with itself for a specific number of times.
print(10 ** 3)
# Output
1000
Relational Operators
Relational operators define how the operands are related to each other using a set of symbols such as
- Greater than ( > )
- Greater than or equal to ( >= )
- Lesser than ( < )
- Lesser than or equal to (<= )
Usually, these relational operators are used to compare two numerical values and a boolean value True is returned if the statement is true. False is returned if the statement evaluates to false.
var_1 = 56
var_2 = 234
print(var_1 < var_2) -> True
print(var_1 <= var_2) -> True
print(var_1 > var_2) -> False
print(var_1 >= var_2) -> False
These relational operators are also useful to compare two strings. When we establish a relation operator between two strings, it is evaluated using the Unicode values(ASCII values) of the characters.
Python provides an inbuilt method for finding the Unicode value of a character. Similarly, there is an inbuilt method for knowing the character value of Unicode value.
# returns character value of a unicode value
chr(97)
'a'
# returns unicode value of a character
ord('a')
97
These Unicode values are case-sensitive for characters. For example, the Unicode value of 'A'
is 65 whereas the Unicode value of 'a'
is 97. To read more details regarding ASCII values please click the link ASCII Table
ord('a')
97
ord('A')
65
Thus when we compare two strings the Unicode value of the first character of these strings are checked internally by python and returns True or False based on the result.
If the first characters of the two strings are the same then the second characters of the string are evaluated.
var_1 = "hello"
var_2 = "python"
print(ord('h'), ord('p'))
104 112
# returns False since the unicode value of 'p' is less than 'h'
print(var_1 > var_2)
False
We can use these relational operators to perform a chain of evaluations in a series known as the chaining concept. It returns True as long as each and every relation is true. In case one statement evaluates to False then the whole statement is considered as False.
10.2>3>1.5>-6
True
10.2>3>1.5<-6
False
These relation operators are very important to evaluate if clause statements.
var_1 = 56
var_2 = 234
if var_1 > var_2:
print("var_1 is greater than var_2")
else:
print("var_1 is less than var_2")
# Output
var_1 is less than var_2
Equality and Not Equal Operators
In python Equality, operators are used to comparing two values on either side of the equality symbol. If both the values are equal then the comparison returns True, and if both are not equal then it returns False.
10 == 10 -> True
python" == "python" -> True
"python" == "Python" -> False
As in the name Not equal operators returns True if the values are not equal. Since the main operation performed by the not equal operator is to check for values not being equal, thus it returns True if they are not equal and return false if they are equal.
10 != 10 -> False
"python" != "Python" ->True
Chain of Equality operators returns True if all the equality operators return True, Even if one operator returns False then the whole statement is evaluated as False.
23 == 34 == 34 == 34 -> False
2 == 2 == 2 == 2 -> True
When we compare compatibility handling of the equality and not equal operators with other relational operators, the main difference is that when we compare an incompatible value such as a string with integer the equality operator evaluates False whereas other relational operators throw an error and stop execution.
10 == "10" -> False
10 >= "10"
TypeError: '>=' not supported between instances of 'int' and 'str'
Equality operator vs is Operator
Definitely equality and is operators are completely different, because the Equality operator compares the values ( content ) that are stored in the memory object, whereas the is operator check whether the reference of the memory object is equal or not.
In the below example the values of the variables var_1, var_2 are equal but the references of the variables are not the same thus the equality operator( == )
returns True
, and is
operator returns False
.
var_1 = [1, 2]
var_2 = [1, 2]
print(var_1 is var_2)
print(var_1 == var_2))
False
True
print(id(var_1), id(var_2))
(1940833584064, 1940833644096)
Logical Operators
A logical operator is a symbol or word used to connect two or more expressions such that the value of the compound expression produced depends only on that of the original expressions and on the meaning of the operator. Common logical operators include AND, OR, and NOT.
Boolean Values with AND
- AND returns True if both expressions are True
- AND returns False if either one or both expression are False
True and True -> True
True and False -> False
False and True -> False
False and False -> False
Boolean values with OR
- OR returns True if both expressions are either True or False
- OR returns False if one expression is False and other is True
True or True -> True
True or False -> True
False or True -> True
False or False -> False
Boolean values with not
- Not is complement which returns the opposite or an inverted value
- If the value is True it returns False, if the value if False it returns True
not True -> False
not False -> True
When we apply non-boolean values with logical operators the calculation is the same but the assumptions are different.
- A zero value is considered as False. Example:- “”, [], 0, (), etc.,
- A non-zero value is considered as True. Example:- “python”, [1, 2], 1, 42, (1, 2), etc.,
Thus we can Conclude few statements on logical AND, OR
- In AND if the first value evaluates to True then the result is the evaluation of the second expression, irrespective of the value of first expression
- In AND if the first expression evaluates to False then the result is first expression, irrespective of second expression.
- In OR if the first expression evaluates to True then the result is evaluation of first expression, irrespective of second expression.
- In OR if the first expression is False, then the result is the evaluation of second expression, irrespective of first expression
10 and 15 -> 15
10 or 15 -> 10
0 and 15 -> 0
0 or 15 -> 15
Bitwise Operators
Bitwise operators perform bitwise calculations by converting the given values into their respective binary values. These bitwise operators are applicable only between integer or boolean data types. If we try to perform bitwise calculations between an integer and float or string error will be raised.
Different types of bitwise operators available are:-
- Bitwise AND ( & )
- Bitwise OR ( | )
- Bitwise XOR ( ^ )
- Bitwise COMPLEMENT ( ~ )
- Bitwise LEFT-SHIFT ( << )
- Bitwise RIGHT-SHIFT ( >> )
Bitwise AND ( & )
Bitwise AND compares binary bit values between each operand and yields a bit value one ( 1 ) if both bits are 1 and returns zero( 0 ) if either of the bit is 0.
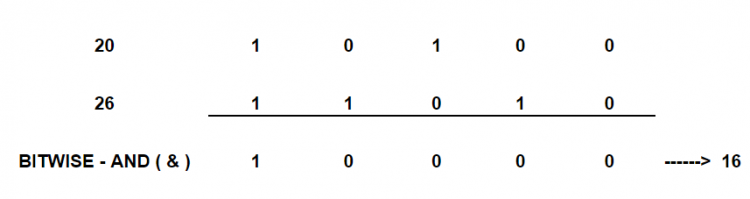
- 1 & 1 = 1
- 1 & 0 = 0
- 0 & 1 = 0
- 0 & 0 = 0
print(20 & 26)
16
Bitwise OR ( | )
Bitwise OR compares binary bit values between each operand and yields a bit value one( 1 ) if at least one bit is non-zero and returns zero( 0 ) if both the bits are zero.
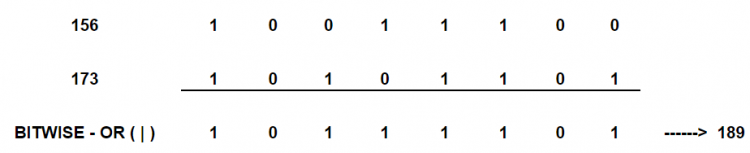
- 1 | 1 = 1
- 1 | 0 = 1
- 0 | 1 = 1
- 0 | 0 = 0
print(156 | 173)
189
Bitwise XOR ( ^ )
Bitwise XOR compares binary bit values between each operand and yields a bit value one( 1 ) if the bits are of different values and returns zero( 0 ) if both are equal.
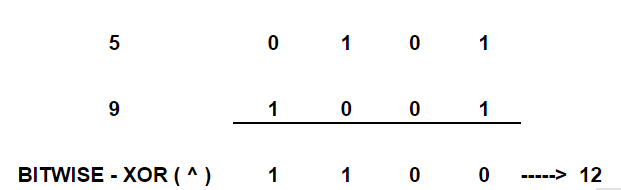
- 1 | 1 = 0
- 1 | 0 = 1
- 0 | 1 = 1
- 0 | 0 = 1
print(5 ^ 9)
12
Bitwise COMPLEMENT ( ~ )
Bitwise COMPLEMENT performs 2’s complement of the given number and returns the value. The Most Significant Bit is used to store the sign of the number whether it’s a +ve or -ve negative number. This MSB is at the left-most place.
- Most significant bit as 0 indicates number is a +ve
- Most significant bit as 1 indicates number is a -ve
The steps involved are as follows, Bitwise complement( ~ ) first performs a complement operation of the given bits. It means 1 becomes 0 and 0 becomes 1.
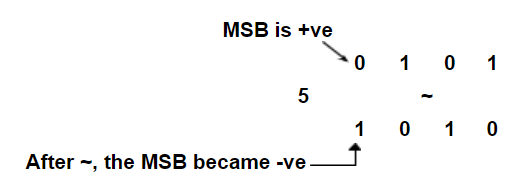
After Complementing all the bits the next step is to obtain a 2’s complement of the obtained complement bits. To perform 2’s complement we first convert the complemented bits into 1’s complement and then add a 1 bit to get 2’s complement.
1’s complement is just reversing the values of bits. If the bit value is 0 it is converted to 1 and if the value of the bit is 1 then it is converted to 0. while performing 1’s complement and 2’s complement the MSB is neglected and is added at the left-most position of the final result.
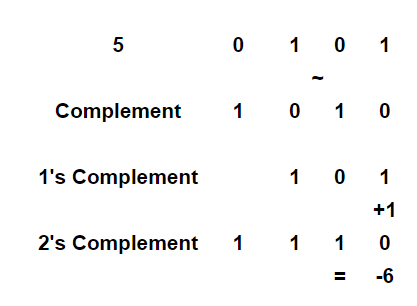
print(~5)
-6
print(~ -3)
2
Thus bitwise complement of 5 is -6. If we perform a bitwise complement of a negative number such as -3, -4, -12, etc., the complement of the 2’s complement of the bits gives the result.
Bitwise LEFT-SHIFT ( << )
The bitwise shift operators move the bit values of a binary object. The left operand specifies the value to be shifted. The right operand specifies the number of positions to be shifted.
Bitwise Left-shift removes a respective number of bits from the left-most side considering the right operand value and add’s exactly the same number of zero-bits to the right-hand side. Basically based on the bit-version the memory uses such as 32-bit, 64-bit, 128-bit, etc,
In memory by default if the usage is less the memory manager allocates 32-bit, thus the bits occupied by the number are represented in binary format and the rest of the bits are represented as zero’s.
In the below example we are left-shifting the binary bits of 10 by 2( 10 << 2 )
, which means the last two bits are removed from the left-hand side and two zero’s are added to the right-hand side.
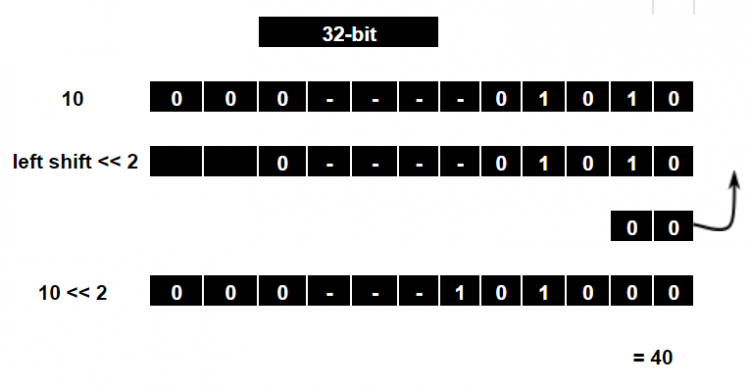
print(10<<2)
40
Bitwise RIGHT-SHIFT ( >> )
The bitwise shift operators move the bit values of a binary object. The left operand specifies the value to be shifted. The right operand specifies the number of positions that the bits in the value are to be shifted.
Unlike the left-shift, In Bitwise RIGHT-SHIFT( >> ) the specified number of right-most bits are removed and exactly the same number of signed bits are added on the left-most side. If the number is positive, zero’s are added. If the number is negative, ones are added.
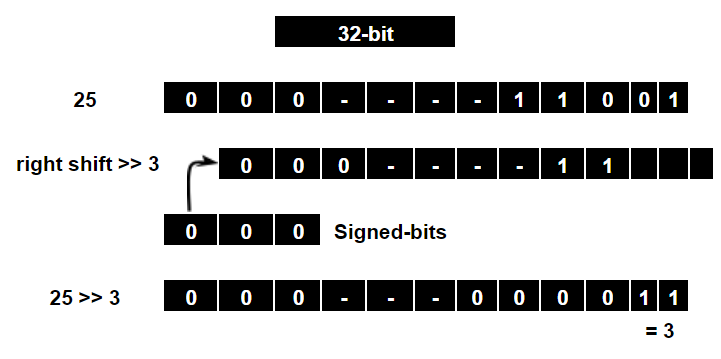
print( 25 >> 3 )
3
Assignment Operator
In Python, assignment operators are used to storing the values in the memory. The variable acts as a reference and the values are stored inside the memory block.
var = "hello world"
print(var)
"hello world"
Apart from assignment operators, there are compound assignment operators. They are:-
- +=
- -=
- *=
- /=
- %=
- //=
- **=
- &=
- |=
- ^=
- >>=
- <<=
These Compound operators perform the operation by applying the right operand value on the left operand using the operator that is specified. The resultant value is stored under the left operand as a reference.
var = 25
# For all the compound assignment operations "var" is 25
# Compound addition assignment operator
var += 20
print(var) -> 45
# Compound subtraction assignment operator
var -= 5
print(var) -> 20
# Compound multiplication assignment operator
var *= 2
print(var) -> 40
# Compound division assignment operator
var /= 5
print(var) -> 5
# Compound modulo assignment operator
var %= 5
print(var) -> 0
# Compound floor division assignment operator
var //= 4
print(var) -> 6
# Compound power assignment operator
var **= 2
print(var) -> 625
# Compound AND assignment operator
var &= 22
print(var) -> 16
# Compound OR assignment operator
var |= 22
print(var) -> 31
# Compound XOR assignment operator
var ^= 22
print(var) -> 15
# Compound right shift assignment operator
var >>= 3
print(var) -> 3
# Compound left shift assignment operator
var <<= 3
print(var) -> 200
In Python increment and decrement operators are not applicable, if we use them syntax error will be thrown by the interpreter. But the use of pre-increment operators is valid but the result is different than expected.
In python, the pre-increment and decrement operators instead of increasing or decreasing the value, act as a sign bit.
- +++x means + * + * + * x = +x
- -+x means – * + * x = – x
- -(-x) means – * – * x = +x
x = 30
++x
print(x) -> 30
-+x
print(x) -> -30
--x
print(x) -> +30
Ternary Operators
Basically, in any programming language, there are different types of operators. Such as unary, binary, ternary, etc.,
When a single operator handles a single operand, then it’s known as a unary operator.
~a, !a
If the single operator handles two operands then it’s known as a binary operator.
a&b, a ^ b, a > b, a = b
If an operator handles three operands then it’s known as a ternary operator. In python, ternary operators help to code in a simpler way with less syntax.
The ternary operator is a conditional expression that returns the result based on the evaluation of the condition. If the conditional expression evaluates to True then a value associated with it is returned or a value associated with False expression is returned.
There are different ways of implementing ternary operators in python. Basic implementation of the ternary operator can be seen using if-else conditional statements.
result = true_value [condition] false_value
var_1 = 25
var_2 = 22
max = var_1 if var_1 > var_2 else var_2
print(max)
# Output
25
Ternary Operators can be implemented just in a similar way we use list comprehension or lambda functions.
We can implement ternary operators in tuple and dictionaries also. The basic syntax for implementing a tuple with ternary operators is
(value_false, value_true)[Condition]
If the expression evaluates true then the variable in the place of condition_true is returned, or else condition_false is returned.
var_1 = 3
var_2 = 5
print("the largest value is ", ( var_2, var_1)[var_1 > var_2])
# Output
the largest value is 5
The same concept is used in dictionaries, the only difference will be True
and False
act as keys and their values associated are returned based on the conditional expression evaluation.
a, b = 4, 5
print({True : "a is greater", False : "b is greater"}[ a > b ])
# Output
b is greater
Special Operators
In python is, is not, in, not in
are considered are special operators. These special operators are categorized into two types based on their functionality.
- Identity operators ( is, is not )
- Membership operators ( in, not in )
Identity Operators
Identity operators are used to comparing the identity of the objects, and these objects are the variables that act as a reference to a memory object. If two identifiers point to the same memory object then they are equal in terms of reference and their reference id is the same.
is
-> returnsTrue
if the reference is sameis not
-> returnsTrue
if the reference is not same
a = "hi"
b = "hi"
print(a is b)
True
In the above example, the string object is the same and hence the references created to it are also the same, but if we create a list object with the same content then the result will be different because each time we create a new list, a new object will be created in the memory to store it, so they will not be same.
var_1 = [1, 2]
var_2 = [1, 2]
print(var_1 is var_2)
print(id(var_1), id(var_2))
False
(1940833584064, 1940833644096)
Membership Operators
We can use Membership operators to check whether the given object present in the
given collection. (It may be String, List, Set, Tuple OR Dict)
in
-> ReturnsTrue
if the given object present in the specified Collectionnot in
-> ReturnsTrue
if the given object not present in the specified Collection
a = [1, 2]
b = 2
print(b in a)
True
Operators Precedence
If an expression has multiple operators with multiple operands python follows the operator precedence to evaluate the expression. The following list describes the precedence order of the operators.
The top of the list is the most top priority operator and is executed first in the expression, and the bottom of the list is the lowest priority operator and is executed at the final stage of the calculation.
- () -> Parenthesis
- ** -> Exponential Operator
- ~, – -> Bitwise Complement Operator, Unary Minus Operator
- *, /, %, // -> Multiplication, Division, Modulo, Floor Division
- +, – -> Addition, Subtraction
- <<, > -> Left and Right Shift
- & -> Bitwise And
- ^ -> Bitwise X-OR
- | -> Bitwise OR
- >, >=, <, <=, ==, != -> Relational OR Comparison Operators
- =, +=, -=, *=… -> Assignment Operators
- is , is not -> Identity Operators
- in , not in -> Membership operators
- not -> Logical not
- and -> Logical and
- or -> Logical or
a, b, c, d, e, f = 1, 5, 2, 6, 2, 8
print((a + b)*c - d / e + f)
# 6*2 - 3 + 8
17.0
FAQ’s in Python Operators
Which mathematical operator is used to raising five to the second power in python?
The exponent operator( **
) is used for performing base to the power calculations. Operand on the left side of the operator is the base and operand on the right side is the power.
pow_operator = 6**2
print(pow_operator)
# Output
36
How to convert string to the operator in python?
In python operator module provides functionalities for performing operations that an operator would perform. And it takes the operand values inside a parenthesis ().
We can assign a dictionary with operators in string format as key and the associated operation of it using operator module as a value, thus acting as key-value pairs.
import operator
ops = {"+" : operator.add}
result = ops["+"](5,4)
print(result)
# output
9
What does can’t assign to operator mean in python?
This error might have been caused due to the inappropriate assignment of values to an operator. The basic rule of operators is left operand has to be a reference that stores the value and the right operand is meant to perform a calculation and return it to the left operand. Doing reverse might cause such an error.
4+5 = var_1
SyntaxError: cannot assign to operator
What is operator overloading in python
The operator overloading is the ability of a single operator to support multiple operations such as a multiplication operator( *
) can perform multiplication of two integers and also multiply an integer with a string.
var_1, var_2, var_3 = 3, "python", 2
print(var_1 * var_2)
print(var_1 * var_3)
# Output
pythonpythonpython
6
How to create a custom operator python?
In python, we cannot create new operators apart from the existing ones.
Which operator is ^ on python sets?
The set symmetric difference is represented using the symbol ( ^
). It returns all the values of the two sets but excluding the common values between the two sets.
set_1 = {1, 2, 3, 5}
set_2 = {4, 3, 5, 7, 8}
print(set_1 ^ set_2)
# Output
{1, 2, 4, 7, 8}
How to assign an operator to a variable in python?
We can pass an operator as a string using the input function and perform the calculations using the operator module in python.
import operator
ops = {
"+" : operator.add,
"-" : operator.sub,
"*" : operator.mul,
"/" : operator.truediv,
"%" : operator.mod
}
string_operator = input()
result = ops[string_operator](5,4)
print(result)
# input , # output
/ 1.25
+ 9
- 1
How to use and operator in python?
In AND it returns True if both the operands are True else it returns False if either of them or both are False. Any value apart from 0 and False is considered as True. and we can use expressions also.
5 and 6 -> returns 6
0 and 6 -> returns 0
if 8>9 and 2<3:
print("statement is True")
else:
print("Statement is False")
How to count the number of occurrences, operator in python?
In python operator.countOf()
returns the number of occurrences of an item in a sequence.
from operator import *
list_1 = [1, 2, 3, 2, 2, 5]
print(countOf(list_1, 2))
# Output
3
What is the operator for string in python?
There are various operators for a string in python such as +, *, [], [:], in, not in, etc.,
str_1 = "hello"
str_2 = "python"
print(str_1 + str_2) -> "hello python"
print(str_1*2) -> "hellohello"
print(str_1[3]) -> l
print(str_2[0:2]) -> "py"
What is modulo operator python?
The modulo operator( %
) calculates and returns the remainder that is obtained by dividing two values. The syntax of the modulo operator is number_1 % number_2.
5 % 2 -> 1
6 % 3 -> 0
In python when is an operator bound to an associativity?
The associativity is the order in which Python evaluates an expression containing multiple operators of the same precedence. Almost all operators except the exponent (**)
support
the left-to-right associativity.
4 * 7 % 3 -> 1 (left-right associativity)
3 ** 2 ** 2 -> 81 (right-left associativity)
How to use or operator in python?
In OR it returns True if either of the two operands is True or both of them are True. It returns False if both are False.
True or True -> True
True or Flase -> True
False or True -> True
False or Flase-> False
What are the operator symbols in python?
- Addition ( + )
- Subtraction ( – )
- Multiplication ( * )
- Division ( / )
- Modular dIvision ( % )
- Floor division ( // )
- Exponent ( ** )
- Greater than ( > )
- Less then ( < )
- Greater than or equal to( >= )
- Less than or equal to( <= )
- Equality operator ( == )
- Not Equal operator ( != )
- Logical AND ( and )
- Logical OR ( or )
- Logical NOT ( not )
- Bitwise AND ( & )
- Bitwise OR ( | )
- Bitwise XOR ( ^ )
- Bitwise Compliment ( ~ )
- Bitwise left shift ( << )
- Bitwise right shift ( >> )
- Assignment operator ( = )
What is power operator?
Power operator is also known as exponent operator multiples a number to itself for particular number times by using the exponent value.
4 ** 2 -> 16
3 ** 3 -> 27
How to write a python program to add two positive integers without using the ‘+’ operator?
def add(a, b):
while b != 0:
data = a & b
a = a ^ b
b = data << 1
return a
print(add(2, 10))
# Output
12
When to use ! operator in python?
In python !
operator cannot be used alone and is associated with the equality operator. It is used to represent the not equality operator ( !=
)
print(2 != 3)
# Output
True
What symbol is used for a format operator after a string in python?
The %
symbol is used as a placeholder in strings.
a = "hello"
print("%s world" %(a))
hello world
What is python string repetition operator?
In python *
operator is used for string repetition. We can repeat the string any number of times by associating the string with an integer using the * operator.
"Python"*3 -> "PythonPythonPython"
How to use index operator in python?
Index operator is represented using the square brackets [] and we can pass the index to return the item at that location in a sequence.
string = "HELLO PYTHON"
string[3]
"L"
What is an augmented assignment operator?
An augmented assignment is generally used to replace a statement where an operator takes a variable as one of its arguments and then assigns the result back to the same variable.
x = 5
x += 3 -> x = x + 3
What is a slicing operator in python?
Slicing fetches a sequence with items over a particular range. In python, the slicing operator is represented using a colon inside the square brackets and the range can be specified.
string = "HELLO PYTHON"
string[1 : 5]
"ELLO"
Why doesn’t python support ++ operator?
Because in Python integers are immutable. When it comes to the ++
operator there is a chance of pre-increment and post-increment. Clearly, the post-increment operator is not valid and throws a syntax error.
pre-increment operator just acts as a sign bit operator.
+5 -> +5
+-5 -> -5
--5 -> +5
What operator compares the states of two objects in python?
In python ==
operator is used for checking if the two states of the object are the same to not. It returns True or False based on the evaluation.
a = c = 5
a == c -> True
How the dot operator works with class instance?
In python, everything is an object and each object has its own method and attributes. Since an object is an instance of the class we can use the dot operator ( . )
to access the member of the class.
class ABC:
a = 5
obj = ABC()
obj.a
5
What is the tild operator python?
Python’s Tilde ~ operator is the bitwise negation operator. It takes the number n as a binary number and “flips” all bits 0 to 1 and 1 to 0 to obtain the complement binary number.
~8 -> -9
~4 -> -5
Logical operator evaluates to what data type python?
The Logical operator in python is used to evaluate whether a statement is true or not. Thus when evaluated it returns a boolean data type either True or False.
a, b = 4, 5
type(a>b)
<class 'bool'>
Where does modulus operator come in order of preference in python?
Clearly, the modulus operator precedence is below the operators such as (), **, ~, -, *, / and it’s above all the other operators such as +, -, <<, +=, etc.,
How to add a string to a list in python using the + operator?
The + operator in python allows only the same data types to perform addition. Thus to add a string into a list we need to caste the string object into a list and then add it to the list.
string = "234"
list = [1,2, 3, 6]
list+=[string]
print(list)
# Output
[1, 2, 3, 6, "234"]
What is subscript operator in python?
The subscript operator is defined as square brackets []. It is used to access the elements of string, list tuple, and so on.
list = [1, 2, 3, 4, 5]
string = "hello"
list[2] -> 3
string[0] -> "h"
Which python operator assigns the value 44 to the variable age?
In python to assign a value to a variable we use assignment operator ( = )
.
age = 44
What python library has operator itemgetter?
The operator is a built-in module providing a set of convenient operators. Itemgetter belongs to the operator module that constructs a callable that assumes an iterable object.
import operator
getter = operator.itemgetter(0)
print getter([1, 2, 3])
# Output -> 1
How to get a round operator python?
round()
is an inbuilt function in python and returns a rounded or approximated number of the specified number.
round(67.343) -> 67
round(67.343, 2) -> 67.3
What is Pipe operator python
Python pipe operator is known as Bitwise OR operator( |
). It performs a bitwise operation between the operand and returns the result of the addition.
4 | 6 -> 6
345|3 -> 347
In Python, what does the unpacking operator do in python?
In python, the unpacking operator is denoted by * and **. Basically, we pass any number of values to a variable using these unpacking operators.
- * can be used for unpacking any iterable
- ** can be used on python dictionaries
Which function overloads the >> operator python?
In python, there are several magic methods for every operator overloading. For overloading >>
operator __rshift__(self, other)
is used.
How to divide in python without using the division operator?
num1, num2 = 250, 25
result = 0
div = num1 + num2
while(div > num2):
div = div - num2
result += 1
print(result)
# Output
10
What is operator chaining in python?
Operator chaining provides declaring multiple operands in a single line. In comparison to operators and assignment operators, the chaining concept can be used.
4 < 6 < 7 < 8 -> True
a = b = c = d = 5
What does the ‘//’ operator python?
The floor division operator is often represented by the symbol //
. Floor division returns the lower possible integer using the result of the division thus eliminates decimal points.
4 // 5 -> 0
6 // 2 -> 3
How to use a list for in operator python?
The in
operator is a membership operator and can check for the presence of values in a sequence or a string and return True or False based on the evaluation. Since a list is a sequence we can check for items present in it.
list = ["python", 1, 35, "string"]
1 in list -> True
2 in list -> False
Why use the bitwise operator & in python?
In Python, bitwise operators are used to performing bitwise calculations on integers. The bitwise addition is performed on binary bits of the integers and addition between 1 and 1 results in 1 or else it is 0
12 & 56 -> 8
1 & 4 -> 0
In python, what does the “%” operator do in the expression 6 % 4?
Basically % operator returns the remainder as a result of performing division. Thus it returns 2 as result.
6 % 4 -> 2
Which operator is used for division in complex numbers in python?
We can use the division operator( / ) to divide two complex numbers in python.
12 + 4j / 6 +2j -> (12+2.6666666666666665j)
What type of operator is |<=| operator in python?
The <=
is a comparison operator used for comparing two values or operands of the same type. It returns True or False based on the evaluation result.
5 <= 6 -> True
6 <= 6 -> True
How to use “not in” operator in python?
The not in
is also a membership operator same as an operator but the functionality is quite opposite. If an element is not present in the given iterable then it returns True else returns False.
string = "Hello Python"
"z" not in string -> True
"Hello" not in string -> False
How to classify operator and number in python?
An operator is a special symbol and is always used to perform operations between one or more values. Whereas the number is a value that is used to store and retrieve from the memory to perform operations on it.
In simple terms, operators are used for performing operations on numbers.
How to chain to if statments with or operator python?
Operator Chaining is used to perform two or more comparisons or evaluations. We can chain an if statement with multiple or statements, it will be just a sequence of or
statements, and evaluation of at least a single or statement is considered as True.
if 5>6 or 6<7 or 25>4 or var_1>var_2
What does python floor operator mean?
In python floor()
is a member of the math module and is used to perform mathematical approximations on a floating-point number, and return the largest integer not greater than the float number.
import math
math.floor(4.8)
# Output
4
In Python, what does the minus operator do for lists?
We cannot directly subtract a list from another list using the minus operator( – ), since it throws an error.
To overcome this situation we can convert the list into the set and then perform a minus operation and convert it back to the list.
a = [1,2,3,4,5,7]
b = [2,3]
c = list(set(a) - set(b))
print(c)
# Output
[1, 4, 5, 7]
How fast is the in operator in python?
The python in operator is very slow when it is operating on Lists. because it traverses the whole list. When the in operator is used on set and dict it’s comparatively fast
- Time Complexity of
in
with Lists is O(n) - Time Complexity of
in
with set and dicts is O(1) as average and O(n) as worst case
How to evaluate a logic in python if the operator is a variable?
To evaluate a logic if the python operator is stored in a variable we need to use the operator’s module and use a dictionary to assign the particular operation of the operator as a value and assign the variable as a key.
In python what is a format operator?
The format operator, % allows us to construct strings, replacing parts of the strings with the data stored in variables. When applied to integers, % is the modulus operator. But when the first operand is a string, % is the format operator.
print(f"the list is {[1,2]}") -> the list is [1, 2]
What is the difference between the // operator and the / operator python?
The main difference between the Floor division( // ) and division operator( / ) is the values that they return.
- Floor division always return integer data type
- division operator always return a floating-point data type
3//4 -> 0
3/4 -> 0.75
In Python which operator returns float?
Irrespective of the values the division operator always returns float values.
What is an example of a comparison operator in python
There are various comparison operators available and they always return a boolean value based on the evaluation.
- Greater than ( > )
- Less then ( < )
- Greater than or equal to( >= )
- Less than or equal to( <= )
- Equality operator ( == )
- Not Equal operator ( != )
How to add two numbers using bitwise operator python
Bitwise operators convert the numbers into their binary bits and perform the operations based on the operator such as &, |, ^, ~, etc., Python performs these operations in the background and returns the values back into a normal number.
12 & 3 -> 0
25 | 5 -> 29
25 ^ 6 -> 31
What list operator in python will remove a specific element?
In Python, we can use either del or remove to delete a specific element from the list. del removes elements based on their index position and remove directly removes the elements that we pass.
a = [1, 2, 3, 4, 5, 6]
del a[3] -> accepts index
print(a)
a.remove(3) -> accepts values
print(a)
[1, 2, 3, 5, 6]
[1, 2, 5, 6]
What is ternary operator in python?
If an operator handles three operands then it’s known as a ternary operator. In python, ternary operators help to code in a simpler way with less syntax.
Which method or operator can be used to concatenate lists python?
To concatenate two lists we can use either extend()
function or use concatenation symbol( +
).
list_1 = [1, 2]
list_2 = [4, 5]
list_1 + list_2
[1, 2, 4, 5]
list_1.extend(list_2)
print(list_1)
[1, 2, 4, 5]
What is the concatenation operator in python?
The python concatenation operator is used to add two iterable into a single object. the result data type is the same as the operands used.
[1, 4, 6] + [2, 2] -> [1, 4, 6, 2, 2 ]
"hello" + "python" -> "helloython"
What happens if you have two values with no operator between them in python?
If there is no operator between two operands python throws a syntax error.
What does the delete operator do in python
The delete operator deletes the reference of an object completely. And the value stored by the reference will remain the same if there is any other reference for it, else the python managers detect it and delete it automatically.
Which python operator goes from right to left?
In python, there are several operators with a left to right associativity but the operator with the right to left associativity is the power operator( ** )
4 ** 2 ** 2 -> 256
In Python, which operator can be overloaded by using __invert__() ?
Using magic functions we can overload the operators in python. Thus to overload the tild operator( ~ ) we can use __invert__()
.
What is the operator library in python?
The operator module supplies functions that are equivalent to Python’s operators. These functions are handy in cases where callables must be stored, passed as arguments, or returned as function results.
The functions in the operator module have the same names as the corresponding special methods.
What is a relational operator in python?
Relational operators are used for comparing two values or operands of the same type. It returns True or False based on the evaluation result. These relation operators are also known as comparison operators.
Is break a keyword or an operator or what python
The break
is a keyword, not an operator. The functionality of break is to break a loop when the condition requirement is satisfied.
var = "python"
list = []
for i in var:
if len(list)>=3:
break
list+=[i]
print(list)
# Output
['p', 'y', 't']
In python, what is a boolean operator?
Python Boolean operator is a logical operator that performs logical operations between the operands and returns True or False based on the evaluation.
4 and 5 -> 5
45 and 3 -> 3
34 or 5 -> 34
not 34 -> False
In Python 3, what is slash followed by equality operator
In python plus followed by a slash is a compound or augmented division operator and is represented as /=
.
a = 5
a /= 2
print(a)
# Output
2.5
How to have a seperator as an operator python
We can use the separator as an operator in the print statement. We can pass the different symbols or an empty space while printing the values.
a = 4
b = 5
print(a, b, sep = "")
print(a, b, sep = " ")
print(a, b, sep = " * ")
45
4 5
4 * 5
How to find double and square of a number in python by using * operator?
- Multiply the number with itself for finding the square of the number
- Multiple with 2 for finding the double of the number
4 * 4 -> 16
4 * 2 -> 8
How many elements can u pass an in operator in python?
We can pass only one element to check its membership. If we pass more than one element also, the operator checks for the element that is just above it.
How to write if statement with and operator in python?
If
clause is executed only if the condition is True. We can pass multiple and statements as required but statements inside if clause get executed only if all are True.
if 8>5 and 5<6 and True and True
Which operator has the lowest precedence?
Logical or
has the lowest precedence in python.
Why we can’t use slicing operator [] in python data type set ?
In python sets, there is no indexing attached to any element. Thus accessing the elements using indexing is prohibited, so slicing is also not provided.