All the programming languages execute code line by line, but in some cases, we might want to avoid executing few lines of code. This is where we use conditional statements. The conditional statements may ignore certain lines or execute certain lines again and again.
In this article we are going to discuss conditional statements, conditional statements include:-
- if
- nested if
- if – else
- nested if else
- elif
- Custom Switch
These if and else statements are also known as conditional statements because the flow control of the program is based on the evaluation of the conditions.
The if statement in python is followed by a condition that is a result of an expression or a value and an indentation.
If condition
If clause is used to evaluate a condition or expression whether it is True or False. When the if expression is True then the code present inside the if block gets executed otherwise control is passed to the next lines of code present after the if block.
# Syntax
if condition1:
statement1
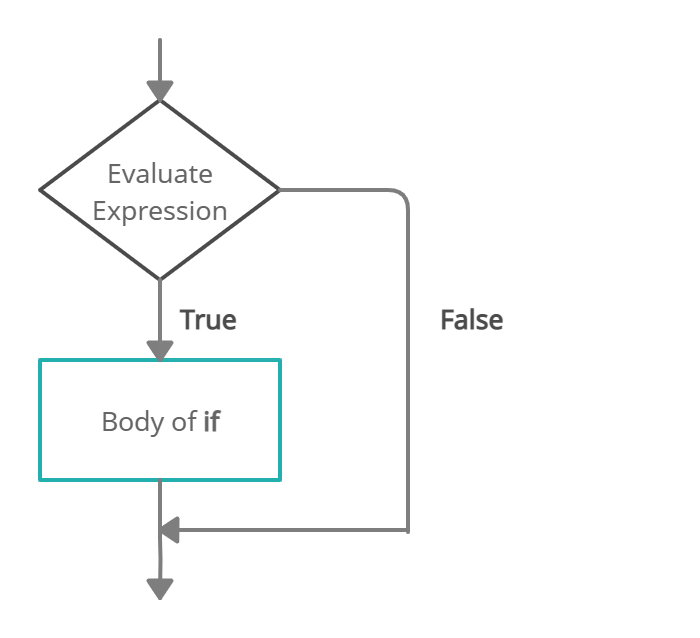
Here is the example of an if clause that gets executed since the condition is True. Any value that is other than zero is considered True, so the if clause gets executed.
if True:
print("inside")
# Output
inside
if 5:
if 2 < 5:
if 3 + 4 == 7:
The below are examples where the if clause is turned out to be False.
if False:
if 0:
if 2 > 5:
After we declare an if-clause with proper indentation, the code that is indented below the if is automatically detected as they are belonging to the if block.
value = 2 * 4
if value > 3:
print("Result is greater than 3")
# Output
Result is greater than 3
We can write multiple if blocks in the python program.
my_string = "Hello World"
if "i" in my_string:
print("statement 1 executed")
if "W" in my_string:
print("statement 2 executed")
# Output
statement 2 executed
Else Clause
The else clause will be executed when the expression evaluates to False. When we declare an if and else clause, only one among them is executed.
- when if is executed else is not executed
- when if not executed else is executed
Unlike if, we don’t have to declare a condition in else clause.
# Syntax
if condition1:
statement1
else:
statement2
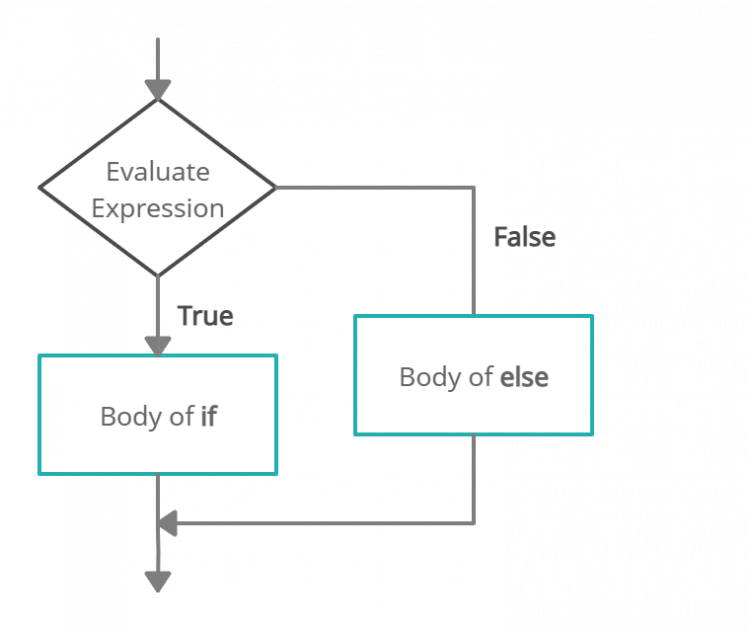
value = 2 * 4
if value < 10:
print("if block is executed")
else:
print("else block is executed")
# Output
else block is executed
While using multiple if or elif conditions the else block belongs to the condition that is declared just before it.
In the below example, the execution of the else block is dependent on whether the condition that is declared just before the else block. Hence it is independent of other if statements.
name = "python"
if name == "python":
print("welcome, python")
if name == "jack":
print("welcome, jack")
else:
print("unknown user")
# Output
welcome, python
unknown user
if-elif-else
elif is also known as else-if, and a condition is associated with an elif clause. When the if-clause fails to execute, the control is passed to the next elif clause. This process goes on as long as there are multiple numbers of elif clauses.
# Syntax
if condition1:
statement1
elif condition2:
statement2
elif condition3:
statement3
else:
statement4
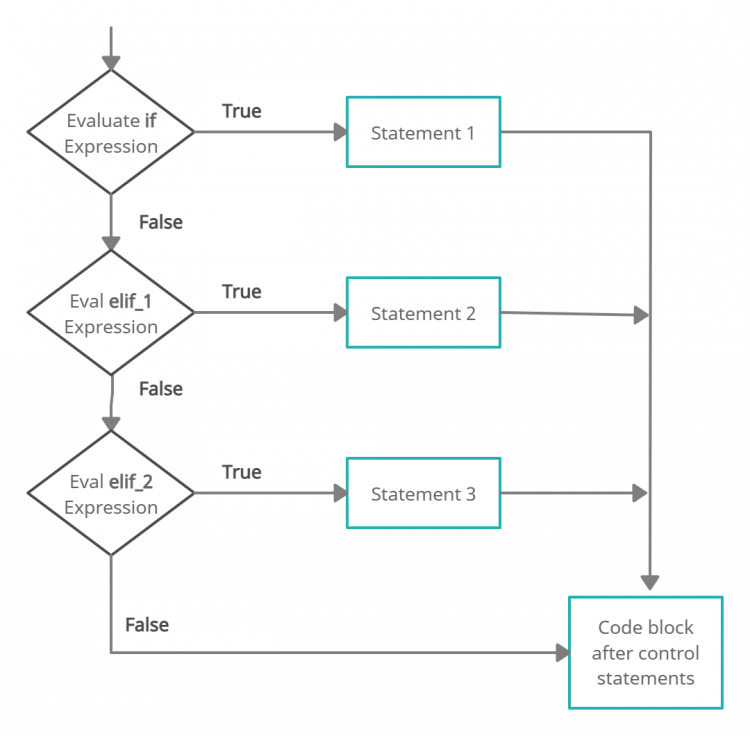
my_string = "Hello World"
if "i" in my_string:
print("if block is executed")
elif "o" in my_string:
print("elif is executed")
else:
print("else block is executed")
# Output
elif is executed
We can declare multiple elif statements just like declaring multiple if-clauses. But the execution of these clauses depends on which condition is first evaluated to True. When a statement is evaluated True, then the other conditions are skipped by the interpreter.
In the below example, statement 3 is True and is executed and even though statement 4 is True, it is skipped since it’s the basic nature elif clause.
num_1 = 5
num_2 = 6
if num_1 > 10:
print("statement 1 executed")
elif num_1 == 3:
print("statement 2 executed")
elif num_1 < num_2:
print("statement 3 executed")
elif num_2 == 6:
print("statement 4 executed")
else:
print("else block is executed")
# Output
statement 3 executed
Nested if
Nested if means declaring one or more if conditions inside an if clause. We can also declare multiple elif or if clauses inside an if condition.
# Syntax
if condition1:
if condition2:
if conditon 3:
---
---
if user_name == "python":
print("welcome")
if password == "1234":
print("logged in")
else:
print("invalid user")
# Output
welcome
logged in
Usage of expressions that have assignment operators while declaring if and elif statements is strictly prohibited since the control statements do not allow the usage of assigning values.
name = "python"
# throws error, since assignment operator is used
if name = "python":
print("welcome, python")
# Executed perfectly
if name == "python":
print("welcome, jack")
Nested if else
Nested if-else is a series of statements that are either declared inside the if clause or else clause.
if condition_1:
if condition_2:
---
---
else:
---
else:
if condition_3:
---
---
else:
---
Custom Switch
In python, we don’t have switch statements. But we can implement the concept of switch-case by customizing the if-elif clauses.
In the below example among all the statements, only one will be executed based on the condition being evaluated, which serves as a customized switch in python.
value_1, value_2 = 9, 3
input_operator = input("Enter an operator :")
if input_operator == "+":
print(f"the sum is {value_1 + value_2}")
elif input_operator == "-":
print(f"the subtractio is {value_1 - value_2}")
elif input_operator == "*":
print(f"the multiplication is {value_1 * value_2}")
elif input_operator == "/":
print(f"the division is {value_1 / value_2}")
elif input_operator == "%":
print(f"the remainder is {value_1 % value_2}")
else:
print("entered invalid operator")
# Input # output
+ the sum is 12
* the multiplication is 27
Ternary operators using if
A ternary operator is known to have three operands, it can be implemented using if and else statements. These ternary operators when integrated with the if-else clause they provide inline coding.
The basic syntax is as follows:-
result = true_value [condition] false_value
In the place of a condition block, we can insert an if-else statement.
var_1 = 25
var_2 = 22
max = var_1 if var_1 > var_2 else var_2
print(max)
# Output
25
if using comparision operators
We can declare an expression using comparison operators such as >, <, and, or, etc., As we know if
gets executed only when the evaluation of the condition is True, when the evaluation is False it does not get executed, the else
block is executed if we declare any.
Note:- Usage of assignment operators is strictly prohibited.
In the below example, if statement is evaluated to True only if both comparisons are evaluated to True, since and operator requires both operands as True.
a, b, c = 4, 2, 3
if a > b and a>c:
print("a is greater")
# Input
a is greater
When there is or
in the expression one True operand is enough, that means one expression has to be True, if both operands are False the whole statement is considered as False which results in skipping the if
clause.
a = 5
b = 6
c = 7
if a > b or c > b:
print("one comparision is True, and if clause is executed")
# Output
one comparision is True, and if clause is executed
We can implement chaining of operators in a single if condition.
a = 5
b = 6
c = 7
if c > b > a:
print("c is greater")
# Output
c is greater
if clause using Pass
When we declare and if clause there has to be some statement inside it since we are using indentation. But when there is a requirement of using the if clause in the future we can declare an empty clause using the pass keyword.
if condition:
pass
if __name__ == “__main__”
In Python, every program has its special variable defined. One among them is the __name__ variable. The main importance of the __name__ variable is its behavior.
When we print the __name__
variable in a program it returns __main__
.
# this is file_1
print(__name__)
# Output
__main__
But when we load file_1 into file_2 and run the file_2 the value returned by the print function of file_1 is different. Instead of returning __main__
value as it did while running file_1 it rather returns the name of the file.
This happens because we are loading the file_1 into file_2. __name__
returns __main__
only when we run it in our program, if we load it into another file it returns the name of the file.
# this is file_2
import file_1
print("This is file_2")
# Output
file_1
This is file_2
So we use if
condition to evaluate whether the program is being imported or in its file.
FAQ’s in Python if, else
How to do if statements in python?
We can declare an if
statement using the if keyword and then declare a condition followed with indentation. The statements that we write inside the indentation are considered that they belong to the if clause.
if condtion:
statement 1
statement 2
---
How to end an if statement python?
We can simply remove the spaces provided by the indent in the if statement and start coding from the new line.
if condtion:
---
---
print("if block is ended")
How to check if a number is prime in python?
A number is known to be a prime number it doesn’t have factors other than 1 and itself. In simple terms If a number has only 2 factors then it is known as a prime number.
So when we divide a prime number with any other number, it is not completely divided, rather it leaves a remainder. Using this concept we find out whether a number is prime or not.
num = int(input())
for i in range(2, num):
if num % i == 0:
print("not a prime number")
break
else:
print("prime")
# Input # Output
6 not a prime number
11 prime
How to check if list is empty python?
We can check the length of a list using len()
method which returns the number of items that are present in the list. if the value returned by the len()
is 0 then the list is empty. We can implement all these conditions in a simple if statement.
list_1 = []
list_2 = [1, 2, 3]
if len(list_1) == 0:
print("list_1 is empty")
else:
print("list_1 is not empty")
if len(list_2) == 0:
print("list_2 is empty")
else:
print("list_2 is not empty")
# Output
list_1 is empty
list_2 is not empty
How to check if key exists in dictionary python?
We can pass membership operator( in
) for the dictionary as a condition into the if clause.
dictionary = {"hello" : "world", "python" : 2}
if "hello" in dictionary:
print("yes")
else:
print("no")
# output
yes
How to check if a string is in a list python?
To check a string is present in a list or not we use in
operator to check the membership of the string. All these conditions can be passed into a if
clause for evaluation.
my_list = ["hello", "world", "python"]
if "hello" in my_list:
print("yes")
else:
print("no")
# output
yes
How to check if a number is even in python?
Every even number is divisible completely by 2 and does not leave any remainder, so we can pass a condition with these specifications into an if-clause
.
num = 24
if num % 2 == 0:
print(f"{num} is an even number")
else:
print(f"{num} is an odd number")
# output
24 is an even number
How to check if a number is an integer in python?
The type() method in python returns the data type of a value if we can pass the value and compare its data type using type()
method we can find out the value is an integer or not.
num_1 = 23.6
num_2 = 125
for i in num_1, num_2:
if type(i) == int:
print(f"{i} is an integer")
else:
print(f"{i} is not integer")
# Output
23.6 is not integer
125 is an integer
How to check if a string is a number python?
In python, isdigit()
method returns True, whether all the characters in a string are digit are not. This evaluation could be passed into the if clause.
my_string = "1234"
if my_string.isdigit():
print("my_string is a number")
else:
print("my_string is not a number")
# Output
my_string is a number
How to check if a dictionary is empty in python?
dictionary = {}
if len(dictionary) == 0:
print("dictinary is empty")
else:
print("dictionary is not empty")
# Output
dictinary is empty
How to check if a letter is uppercase in python?
string = "HaL"
for i in string:
if i.isupper():
print(f"{i} is an uppercase")
else:
print(f"{i} is lowercase")
# Output
H is an uppercase
a is lowercase
L is an uppercase
How to check if two lists are equal in python?
To check any two values using the if clause we need to use the comparison operator. For checking equality between two lists we can pass ==
operator.
a = [1, 2]
b = [3, 4]
if a == b:
print("a & b are equal")
# Output
a & b are equal
How to check if a variable is a list in python?
a = [1, 2]
if type(a) == list:
print("it's a list")
else:
print("not a valid list")
# Output
it's a list
How to write else if in python?
In python, else-if is referred to as elif and is defined by declaring a condition in it.
if 2 > 3:
print("if block")
elif 2 < 3:
print("elif block")
# Output
elif block
How to check if a string is a palindrome in python?
We can reverse a string using the slicing operator and compare it with the original string. If the result is True then the string is a palindrome, else it’s not.
s = "abcdcba"
rev_s = s[::-1]
if s == rev_s:
print("string is palindrome")
else:
print("not a palindrome")
# Output
string is palindrome
How to check if a number is negative in python?
num = -3
if num < 0 :
print("num is negative")
# Output
num is negative
How to do nothing in an if statement python?
When there is nothing to do with an if statement we can write pass
into it. This means it tells the Python interpreter that the statement is not empty so that error is not thrown.
if condition :
pass
How to check if a number is a whole number in python?
Whole numbers are numbers that are greater than or equal to zero. These numbers are completely positive integers which exclude decimal, fractions, negative numbers.
num = 3
if num >= 0 and type(num) == int :
print("Whole number")
# Output
Whole number
how to check if a character is alphanumeric in python?
Alphanumeric means string having both alphabets and numbers in it. Python provides an in-built method that returns True if the string is alphanumeric, or returns False when the string is not alphanumeric.
s = "as1234"
if s.isalnum() :
print("Yes")
# Output
Yes
How to check if a number is a perfect square in python?
In python, the math module has an in-built method that returns the square root of a number. We can multiply the square root by itself and compare it with the number.
import math
num = 225
sqrt = math.sqrt(num)
if sqrt*sqrt == num:
print("perfect square")
else:
print("not a perfect square")
# Output
perfect square
How to use if __name__ == ‘__main__’ in python?
__name__ returns __main__ when run in its program. So when a program is loaded into another program __name__ returns the file name instead of main.
Thus we can make comparisons and add code inside an if block and run them when the program is executed in its file and not execute when it’s being imported into another file.
How to break out of if statement python?
We can break out of an if statement either starting to write a new line by removing the space provided by the indent of the if block.
if condition:
# statements
print("outside of if block")
How to check if all elements in a list are equal python?
list_1 = [1, 1, 1, 1]
list_2 = []
for i in list_1:
if i in list_2:
continue
else:
list_2 += [i]
if len(list_2) == 1:
print("all items equal")
else:
print("items not equal")
# Output
all items equal
How to check if a number is binary in python?
When the number belongs to binary class it is considered a binary number, we can check this using type()
method.
if type(num) == bool:
# statements
print("outside of if block")
How to check if a value is null in python?
In python, there is no null in python but we have None. We can compare a variable with None to check whether it’s a None or not.
val = None
if val == None:
print("val is None")
How to check if list has duplicates python?
my_list = [1, 2, 3, 1, 2]
emp_list = []
for i in my_list:
if i not in emp_list:
emp_list += [i]
else:
print(f"{i} is a duplicate item")
# Output
1 is a duplicate item
2 is a duplicate item
In python how to reassign variable after an if statement?
val = None
if val == None:
val = "hello"
print(val)
# Output
hello
When to use try except rather than if else python?
try-except has to be used when an error is raised while performing an operation, to handle these errors we can use except block. Whereas the if-else is a conditional statement that evaluates based on the condition we pass.
How to check if two strings have common characters python?
string_1 = "Hello World"
string_2 = "Welcome"
# Empty list for removing duplicate comparisions
my_list = []
for i in string_1:
# checks for duplicate items and skips iteration if any
if i not in my_list:
my_list += [i]
if i in string_2:
print(f"{i} is common")
# Output
e is common
l is common
o is common
W is common
After if statement how many commands can you have for python?
After an, if statement we can declare as many commands as we need. We can implement any functionality or also implement nested operations.
How to make nested if elif statements in python?
To make a nested if elif statement we need to declare follow proper indentation.
if condition:
if condition:
# statements
elif condition:
# statement