A sequence of elements typically characters, inside single or double quotes is called string. Even a single character inside the quotes is also called a string.
Creating a String
We can create a sequence of characters inside a single or double quote to a variable. Thus a string is created.
This sequence of characters inside the quotes can be anything such as numeric, alphanumeric, symbols, etc., It will be considered as a string if it’s enclosed between these quotes
# Examples of strings
str_1 = "Python 3.8"
str_2 = '2547'
str_3 = "a"
str_4 = "^%*&%$"
We can also represent strings using triple quotes also. Multi-line strings are represented using triple quotes.
# Example of Multi-line strings
str_1 = '''hey, let us
start learning
python'''
When a string is declared using single quotes, all the characters between these single quotes are considered as one string.
# Example of single quote strings
str_1 = 'hi welcome'
str_2 = 'a'
When we use single quote strings and we would like to declare a single quote inside it, the interpreter throws an error as an invalid string literal.
# Invalid string literals
str_1 = 'it's raining'
str_2 = 'we have'nt started'
It’s better to use double quotes or triple quotes to create a string so that we can insert any kind of single quote inside it.
# valid string literals
str_1 = "it's raining"
str_2 = "we have'nt started"
If we like to use double quotes inside a string then the strings have to be declared using triple quotes.
str_1 = ''' she said: "what's up". '''
str_2 = ' she said: "what up". '
Accessing the string using index
To access the characters of the string we can use indexes. Each character is assigned a separate index in a string.
There are two types of indexing to access the characters of the string. They are +ve indexing and -ve indexing.
- If the string is accessed from left to right i.e, first element as the left most character and the last element as the right most character, it means string is accessed using +ve indexing
- By default, in the +ve indexing the index value starts from 0 and ends at value equal to (length of string – 1), which means the left most character index is 0 and the right most character index is equal to (length of string – 1)
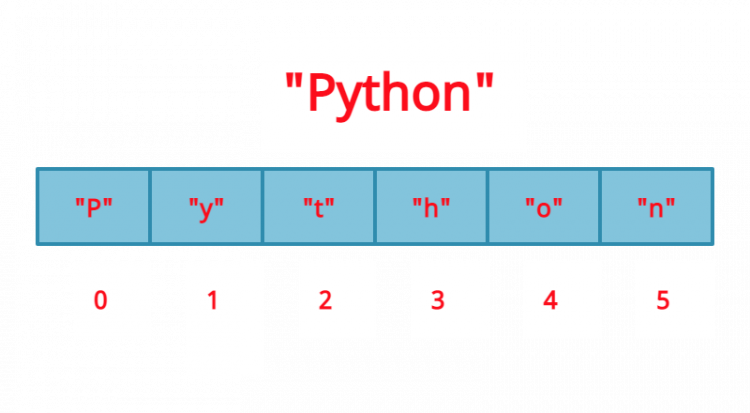
To access these characters using +ve index we can pass the index value into square brackets [] which return the character stored at that position.
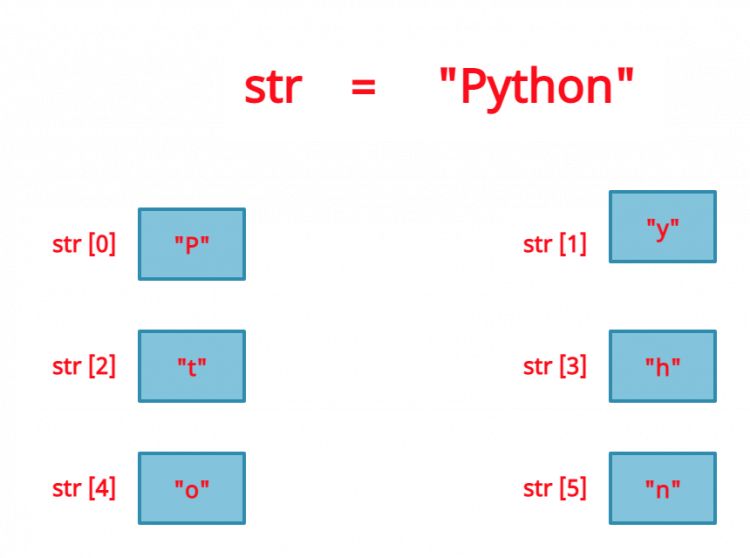
simple_str = "Python 3.8"
simple_str[5] -> "n"
simple_str[2] -> "t"
- If the string is accessed from right to left i.e, first element as the right most character and the last element as the left most character, it means string is accessed using -ve indexing
- By default, -ve indexing starts from -1 and ends with ( – length of string), it means the right most character index is -1 and the left character index is ( – length of string)
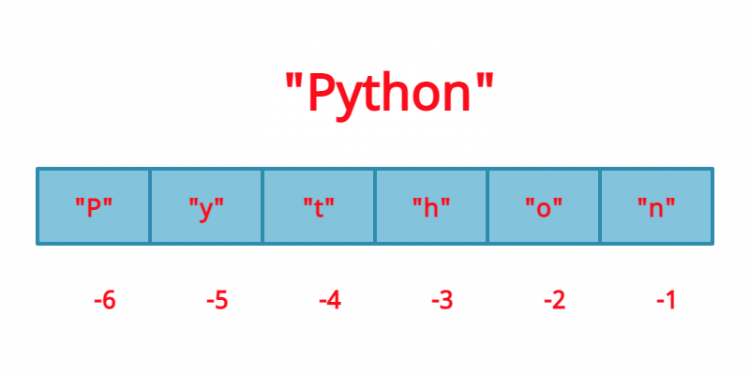
Using the negative index inside the square brackets returns the character at that position.
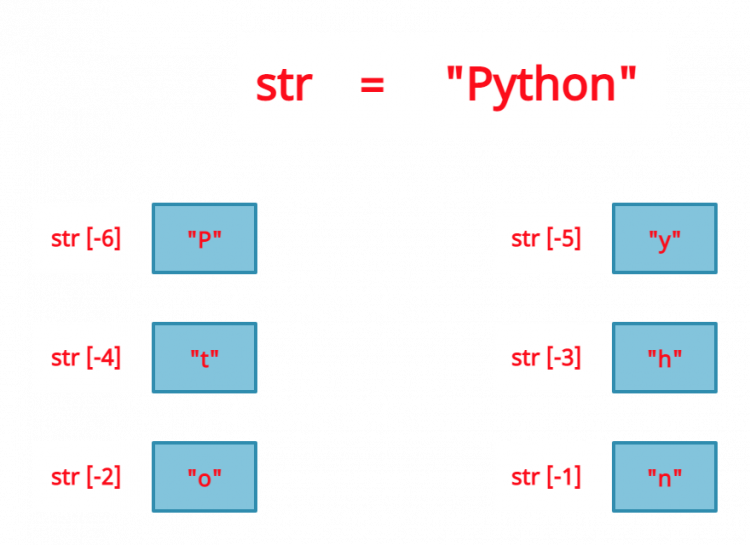
simple_str = "Python 3.8"
simple_str[-1] -> "8"
simple_str[-2] -> "."
simple_str[-5] -> "n"
Accessing the string using slice operator.
We can also access the strings using the slicing operator. The slicing operator returns the substring of the main string. The slicing operator is denoted by a colon enclosed inside square brackets. The general syntax is shown below:-
str[start : end]
By default, the start value is 0 and the end value is len(string) – 1. So if we pass only the colon into the string then the whole string is returned.
simple_str = "Python 3.8"
simple_str[:] -> "Python 3.8"
We can customize the value of start and end by using the indexes of the string. Thus customized start and end values are used to return a sub-string from the main string.
Values passed out of the index throws an error known as Index out of range.
- If we pass str[ x : y ] where x & y being +ve indexes of the string, direction of slicing is forward direction and the value returned is a substring from index “x” to “y-1”
- If we pass str[ x : y ] where x & y being -ve indexes of the string, direction of the slicing is backward direction and the value returned is a substring from index “x” to “y+1”
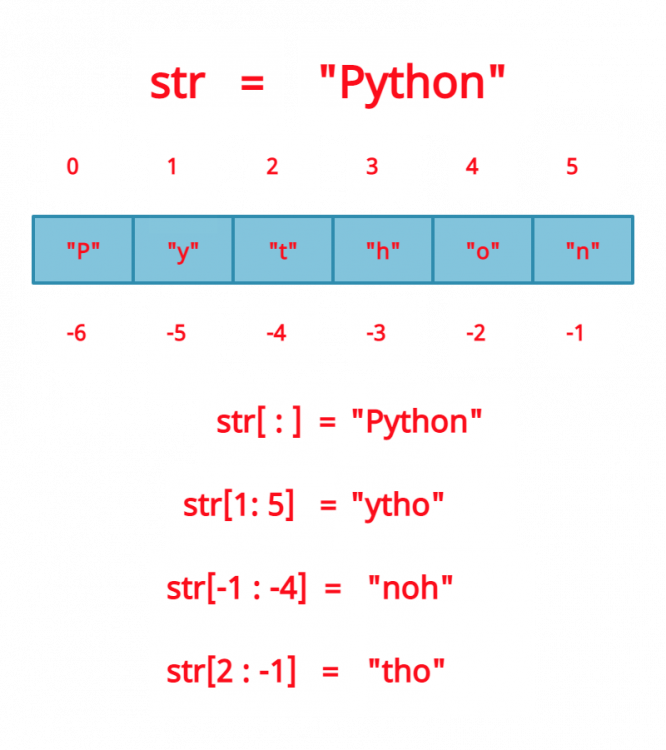
simple_str = "Python 3.8"
simple_str[2:4] -> "th"
Step value in slice operator
Apart from start and end values, there is one more value that can be used in the slice operator known as the step value. By default, the step value is +1 if we don’t declare it.
Implementing step returns every stepth value between start and end indexes.
s[start : end : step]
- The step value can be either +ve or -ve
- If the value is +ve then it is forward direction and we have to consider the sub-string from start to end-1
- If the value is -ve then it is backward direction and we have to consider the sub-string from start to end+1
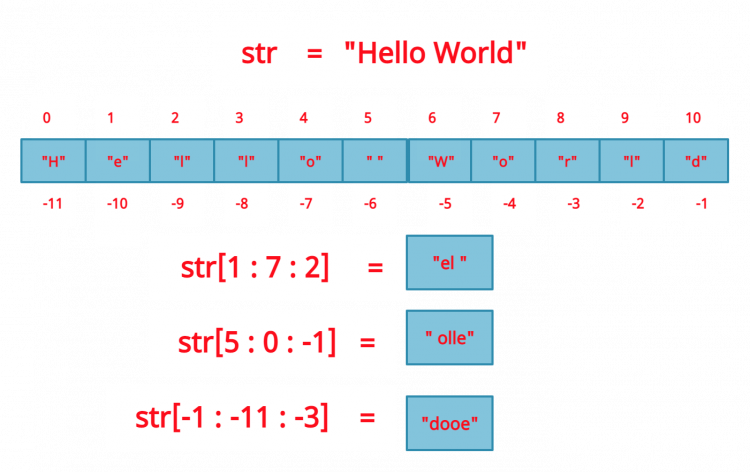
s = "Hello World"
s[-8 : -1 : 2] -> 'l ol'
s[7 : 1 : -2] -> 'o l'
s[9 : 1 : -3] -> 'lWl'
s[::-1] -> 'dlroW olleH'
s[::1] -> 'Hello World'
s[2 : 6 : 0] -> ValueError: slice step cannot be zero
# step operator never raise index out of range error
s[100: -11: -2] -> 'drWol'
Operators for strings
There are few operators which can perform Mathematical, Membership, Comparision operations between two strings.
Mathematical Operators for strings
The Two mathematical operators available for string operations are +
and *
. These two mathematical operators perform operations such as:-
- String Concatenation ( + )
- String Repetition ( * )
String Concatenation is used to combine two strings to form a single string. The operator used for this operation is the + operator.
"Hello" + "World" -> "HelloWorld"
"HII" + "5" -> "HII5"
For the concatenation to happen, both the operands have to string type only.
"str" + 67 -> Type error - can concatenate string to string
The Repetition Operator is used to repeat a string multiple times. The operator used for this purpose is * operator.
"hi" * 3 -> "hihihi"
"Python" * 2 -> "PythonPython"
For string repetition one operand has to be string type and other operand has to be integer type. If we use both repetition operator between two strings Type error is returned.
"HI" * "HI" -> TypeError: can't multiply sequence by non-int of type 'str'
Membership operators for strings
The two membership operators in and not in check whether a substring is a part of the main string or not.
in
operator returns True if the substring is a part of the main string, or else returns Falsenot in
operators returns True if the sub-string is not a part of the main string, if it is in main string then returns False
"p" in "python" -> True
"Hello" in "Hello World" -> True
"python" not in "Hello World" -> True
Comparision Operators for strings
When we compare two strings using comparison operators, it is evaluated using the Unicode values(ASCII values) of the characters.
These Unicode values are case-sensitive for characters. For example, the Unicode value of 'A'
is 65 whereas the Unicode value of 'a'
is 97. To read more details regarding ASCII values please click the link ASCII Table
"S" > "a" -> False
Thus when we compare two strings the Unicode value of the first character of these strings are checked internally by python and returns True or False based on the result.
If the first characters of the two strings are the same then the second characters of the string are evaluated.
var_1 = "hello"
var_2 = "python"
print(ord('h'), ord('p'))
104, 112
# returns False since the unicode value of 'p' is less than 'h'
print(var_1 > var_2)
False
In-built functions in Strings
There are many in-built functions for strings, with each having its own different purpose. They are:-
- capitalize
- casefold
- center
- count
- encode
- endswith
- expandtabs
- find
- format
- format_map
- index
- isalnum
- isalpha
- isascii
- isdecimal
- isdigit
- isidentifier
- islower
- isnumeric
- isprintable
- isspace
- istitle
- isupper
- join
- ljust
- lower
- lstrip
- maketrans
- partition
- replace
- rfind
- rindex
- rjust
- rpartition
- rsplit
- rstrip
- split
- splitlines
- startswith
- strip
- swapcase
- title
- translate
- upper
- zfill
split()
Returns a list of the words in the string, using sep
as the delimiter string.
# Syntax
split(sep, maxsplit)
The delimiter according to which to split the string. None (the default value) means split according to any whitespace, and discard empty strings from the result.
Max split is the maximum number of splits to do. -1 (the default value) means no limit.
S = "hiii, welcome world. lets work out wonders"
print(S.split("w", maxsplit = 1))
print(S.split("w", maxsplit = 2))
print(S.split("w", maxsplit = -1))
# Output
['hiii, ', 'elcome world. lets work out wonders']
['hiii, ', 'elcome ', 'orld. lets work out wonders']
['hiii, ', 'elcome ', 'orld. lets ', 'ork out ', 'onders']
rsplit()
Returns a list of the words in the string, using sep as the delimiter string. Splits are done starting at the end of the string and working to the front.
# Syntax
rsplit(sep, maxsplit)
- sep = The delimiter according which to split the string. None (the default value) means split according to any whitespace, and discard empty strings from the result.
- maxsplit = Maximum number of splits to do. -1 (the default value) means no limit.
S = "hiii, welcome world. lets work out wonders"
print(S.rsplit("w", maxsplit = 1))
print(S.rsplit("w", maxsplit = 2))
print(S.rsplit("w", maxsplit = -1))
# Output
['hiii, welcome world. lets work out ', 'onders']
['hiii, welcome world. lets ', 'ork out ', 'onders']
['hiii, ', 'elcome ', 'orld. lets ', 'ork out ', 'onders']
strip()
strip()
return a copy of the string with the leading whitespace removed. By default, if no parameter is passed into the function trailing white space on both ends of the string is removed.
If a parameter is passed characters matching the parameter are removed from the right end and left end of the string.
# Syntax is S.strip()
s = " HellO HAI "
s.strip() > 'HELLO HAI'
s = "@@HellO HAI@@"
s.strip("@") > "HellO HAI"
rstrip()
rstrip()
return a copy of the string with the leading whitespace removed. By default, if no parameter is passed into the function trailing white space on the right end of the string is removed.
If a parameter is passed characters matching the parameter are removed from the right end of the string.
# Syntax is S.rstrip()
s = " HellO HAI "
s.rstrip() > ' HELLO HAI'
s = "@@HellO HAI@@"
s.rstrip("@") > "@@HellO HAI"
lstrip()
lstrip()
return a copy of the string with the leading whitespace removed. By default, if no parameter is passed into the function trailing white space on the left end of the string is removed.
If a parameter is passed characters matching the parameter are removed from the left end of the string.
# Syntax is S.lstrip()
s = " HellO HAI "
s.lstrip() > 'HELLO HAI '
s = "@@HellO HAI@@"
s.lstrip("@") > "HellO HAI@@"
join()
"s".join()
Concatenate any number of strings. The string(s) whose method is called is inserted in between each given string. The result is returned as a new string.
In the below example the string(s) is passed to the join() function. This string(s) is inserted between each item of the sequence and is returned in the form of a string.
# Syntax is "string".join(sequence)
my_list = ["Hello", "Welcome"]
str_1 = "@ ".join(my_list)
print(str_1)
# Output
Hello@Welcome
find()
Returns the lowest possible index in S where substring that we have passed as parameter is found, such that substring is contained within S[start: end]. Optional arguments start and end are interpreted as in slice notation. Returns -1 on failure.
# Syntax S.find("sub", start, end)
s = "Hey world, welcome world, peaceful world"
s.find("world") -> 4
s.find("world", 6, 20) -> -1
s.find("world", 6, 30) -> 19
rfind()
Returns the highest index in S where substring that we have passed as parameter is found, such that substring is contained within S[start: end]. Optional arguments start and end are interpreted as in slice notation. Returns -1 on failure.
# Syntax S.rfind("sub", start, end)
s = "Hey world, welcome world, peaceful world"
s.rfind("world") -> 35
s.rfind("hey") -> -1
s.rfind("world", 5, 25) -> 19
replace()
Returns a copy with all occurrences of substring old replaced by new. The count is the value for a Maximum number of occurrences to replace. If the count is -1 (the default value) means to replace all occurrences.
If the optional argument count is given, only the first count occurrences are replaced.
# Syntax S.replace("old", "new", count)
s = "Hey world, welcome world, peaceful world"
s.replace("world", "python") -> 'Hey python, welcome python, peaceful python'
s = "Hey world, welcome world, peaceful world"
s.replace("world", "buddy", 2) -> 'Hey buddy, welcome buddy, peaceful world'
s = "Hey world, welcome world, peaceful world"
s.replace("world", "adam", -1) -> 'Hey adam, welcome adam, peaceful adam'
startswith()
Return True if S
starts with the specified prefix, False otherwise. With optional start, test S
beginning at that position. With optional end, stop comparing S
at that position. The prefix can also be a tuple of strings to try.
# Syntax S.startswith(sub, start, end)
S = "hii, welcome world. lets work out wonders"
S.startswith("hii") -> True
S.startswith("welcome", 5, 20) -> True
endswith()
endwith()
function return True if the string ends with the substring(suffix) passed. Optionally we can pass start and end indexes also.
# Syntax is S.endswith(suffix, start, end)
S = "Hello World"
S.endswith("World") -> True
S.endswith("llo", 0, 6) ->False
index()
index()
function returns the lowest index in S where substring(sub) is found, such that sub is contained within S[start: end]. Optional arguments start and end are interpreted as in slice notation.
Raises ValueError when the substring is not found.
# Syntax is S.index(sub-string, start, end)
S = "Hello World"
S.index("H") -> 0
S.index("l") -> 2
rindex()
Returns the highest index in S
where the substring sub
is found, such that sub
is contained within S[start:end]. Optional arguments start and end are interpreted as in slice notation. Raises ValueError when the substring is not found.
# Syntax S.rindex(sub, [start, end]]
S = "hiii, welcome world. lets work out wonders"
print(S.rindex("wo"))
print(S.rindex("wo", 15 , 35))
# Output
35
26
capitalize()
This function is used to return a capitalized version of the string. More specifically, it makes the first character have upper case and the rest of the string into lowercase.
S = "aehrcD"
S.capitalize()
# Output
'Aehrcd'
casefold()
This function converts strings to case folded strings for caseless matching. If there are different combinations of upper and lower characters all of them are converted into the lower case so that they can be used to make comparisons with other strings.
S = "aEHrcD"
S.casefold()
# Output
'aehrcd'
swapcase
swapcase()
converts upper case letters into lower case and lower case letters into upper case in the string.
string_1 = "Hii Helo"
print(string_1.swapcase())
# Output
hII hELO
upper()
upper()
returns a copy of the string converted to uppercase.
# Syntax is S.upper()
s = "HellO HAI"
s.upper() > 'HELLO HAI'
lower()
lower()
returns a copy of the string converted to lowercase.
# Syntax is S.lower()
s = "HellO HAI"
s.lower() > 'hello hai'
isupper()
isupper()
returns True if the string is an uppercase string, False otherwise. A string is uppercase if all cased characters in the string are uppercase and there is at least one cased character in the string.
# Syntax is S.isupper()
s = "HELLO"
s.isupper() -> True
s = "Hello"
s.isupper() -> False
islower()
islower()
returns True if the string is a lowercase string, False otherwise. A string is lowercase if all cased characters in the string are lowercase and there is at least one cased character in the string.
# Syntax is S.islower()
str = "abcDef"
str.islower() -> False
str = "abcdef"
str.islower() -> True
str = "abc123"
str.islower() -> True
isalnum()
The isalnum()
function returns True if the string is an alpha-numeric string, False otherwise. A string is alpha-numeric if all characters in the string are alphanumeric and there is at least one character in the string.
# Syntax is S.isalnum()
S = "Hello3.8"
S.isalnum() -> True
isalpha()
isalpha()
function returns True if the string is an alphabetic string, False otherwise. A string is alphabetic if all characters in the string are alphabetic and there is at least one character in the string.
# Syntax is S.isalpha()
S_1 = "Hello3.8"
S_1.isalpha() -> False
S_2 = "Hello"
S_2.isalpha() -> True
isascii()
isascii()
returns True if all characters in the string are ASCII, False otherwise. An empty string is ASCII too.
# Syntax is S.isascii()
my_string = "ABCD"
my_string.isascii() -> True
my_string = "123"
my_string.isascii() -> True
isdecimal()
isdecimal()
returns True if the string is a decimal string, False otherwise. A string is a decimal string if all characters in the string are decimal and there is at least one character in the string.
# Syntax is S.isdecimal()
str_1 = "123.23"
str_1.isdecimal() -> False
str_2 = "1234"
str_2.isdecimal() -> True
isdigit()
isdigit()
returns True if the string is a digit string, False otherwise. A string is a digit string if all characters in the string are digits and there is at least one character in the string.
# Syntax is S.isdigit()
str_1 = "123ab"
str_1.isdigit() -> False
str_2 = "1"
str_2.isdigit() -> True
isidentifier()
isidentifier()
method returns True if the string is a valid Python identifier, False otherwise. An identifier is valid if it is started with an underscore or an alphabet and does not have any special character symbols in it.
We can use keyword.iskeyword()
to test whether string s is a reserved identifier, such as “def” or “class”.
# Syntax is S.isidentifier()
str = "ello@"
str.isidentifier() -> False
str = "_variable1"
>>> str.isidentifier() -> True
isnumeric()
isnumeric()
returns True if the string is a numeric string, False otherwise. A string is numeric if all characters in the string are numeric and there is at least one character in the string.
# Syntax is S.isnumeric()
s = "1abc"
s.isnumeric() -> False
s = "2"
s.isnumeric() -> True
s = "13465"
s.isnumeric() -> True
isprintable()
isprintable()
returns True if the string is printable, False otherwise. A string is printable if all of its characters are considered printable or if it is empty.
# Syntax is S.isprintable()
s = "1abc"
s.isprintable() -> True
s = " "
s.isprintable() -> True
s = "hello \n Welcome"
s.isprintable() -> False
isspace()
isspace()
returns True if the string is a whitespace string, False otherwise. A string is whitespace if all characters in the string are whitespace and there is at least one character in the string.
# Syntax is S.isspace()
s = " "
s.isspace() -> True
s = " ab "
s.isspace() -> False
istitle()
istitle()
function returns True if all the words in a string start with an upper case letter and the rest of the letters in the word as a lower case letter, else it returns False.
In a title-cased string, upper- and title-case characters may only follow uncased characters and lowercase characters only cased ones.
# Syntax is S.istitle()
s = " "
s.istitle() -> False
s = "Hello@, World Welcome"
s.istitle() -> True
s = "welcome To Python"
s.istitle() -> False
ljust()
ljust()
function returns a left-justified string of a certain length. Two parameters are passed into it., they are length and fillchar.
The length parameter returns a left-justified string equal to the length that we pass. The remaining length of the string is filled with fillchar parameter. If the fill char parameter is not passed by default python fills the remaining string with empty spaces.
# Syntax is S.ljust(length, fillchar)
s = "Hello"
s.ljust(8) -> 'Hello '
s = "Hello"
s.ljust(10, "*") -> 'Hello*****'
rjust()
rjust()
function returns a right-justified string of a certain length. Two parameters are passed into it., they are length and fillchar.
The length parameter returns a right-justified string equal to the length that we pass. The remaining length of the string is filled with the fillchar parameter. If the fill char parameter is not passed by default python fills the remaining string with empty spaces.
# Syntax is S.rjust(length, fillchar)
s = "Welcome"
s.rjust(10) -> ' Welcome'
s = "Hello"
s.rjust(10, "*") -> '***Welcome'
partition()
partition()
function returns a tuple by splitting the string into three parts based on the parameter we pass into it. All these split strings are stored in a tuple.
- The first element of tuple is the part of the string before the parameter
- The second element of the tuple is the parameter itself
- The third element of the tuple is part of the string after the parameter.
# Syntax is S.partition(parameter)
s = "Hello World, Welcome"
s.partition("World") -> ('Hello ', 'World', ', Welcome')
If the passed parameter is not present in the string then the partition()
function stores the first element of the tuple as a complete string and the remaining two parts being empty strings.
s = "Hello World, Welcome"
s.partition("Python") -> ('Hello World, Welcome', '', '')
rpartition()
rpartition()
function returns a tuple by splitting the string into three parts based on the parameter we pass into it. All these split strings are stored in a tuple.
If the parameter passed is repeated 2 or more times in the string then the right-most parameter of the string is considered for partition.
# Syntax is S.rpartition(parameter)
s = "ABC ABC ABC"
s.rpartition("B") -> ('ABC ABC A', 'B', 'C')
s = "ABCD"
s.rpartition("B") -> ('A', 'B', 'CD')
If the passed parameter is not present in the string then the r
partition()
function stores the first element of the tuple as a complete string and the remaining two parts being empty strings.
s = "ABCDE"
s.rpartition("M") -> ('ABCDE', '', '')
expandtabs()
Returns a copy where all tab characters( \t ) are expanded using spaces. If tab size is not given, by default a tab size of 8 characters is assumed.
s = "hello\tworld\twelcome"
print(s.expandtabs())
print(s.expandtabs(2))
print(s.expandtabs(4))
print(s.expandtabs(6))
# Output
hello world welcome
hello world welcome
hello world welcome
hello world welcome
format
Using .format()
returns a formatted string and we can customize the string with other strings or variables inside a pre-defined text.
The string format is done using a pair of curly braces inside a text. This text is then called using the .format()
method in which we can pass the variable or strings into it in an order in which curly braces are defined.
print("Hello, i am using python {}".format(3.7))
# Output
Hello, i am using python 3.7
We can format a string in a different way also.
version = 3.7
print(f"I am using python {version}")
# Output
I am using python 3.7
format_map()
.format_map()
is used the same as .format()
and is used to format a string except that we can pass a dictionary into the .format_map()
method.
we can define a string with dictionary keys inside curly braces and then format the string using .format_map()
in which we pass the dictionary.
The values associated with the dictionary keys are print in the string.
dictionary = {"key_1" : "python", "key_2" : "project"}
print("I am, working on a {key_1} {key_2}".format_map(dictionary))
# Output
I am, working on a python project
center()
center()
returns a centered string of specific length. It accepts an integer(n) as a parameter to return a string of length equal to n with string(s) as the center.
s = "abc"
s.center(5)
# Output
" abc "
count()
count()
function accepts a substring as a parameter and checks how many times the sub-string has non-overlapping occurrences in main string S[start: end]. Optional arguments start and end are interpreted as in slice notation.
S = "abcdabcdabcd"
S.count("ab") -> 3
S.count("ab", 0, 7) -> 2
encode()
encode()
function encodes the string using the codec registered for encoding. Parameters passed are encoding, errors.
If no parameter is passed, by default it returns the UTF-8 encoded format of the string.
S = "abcdabcdabcd"
S.encode(encoding = "utf-8") -> b'abcdabcdabcde'
maketrans()
Returns a translation table usable for str.translate()
. It makes one to one mapping of a character to its translation/replacement.
- If there is only one argument, it must be a dictionary mapping Unicode. ordinals (integers) or characters to Unicode ordinals, strings or None. Character keys will be then converted to ordinals
dict = {"x": "34", "y": "63", "z": "548"}
string = "abc"
print(string.maketrans(dict))
# Output
{120: '34', 121: '63', 122: '548'}
- If there are two arguments, they must be strings of equal length, each character will be mapped to the character at the same position
string_1 = "Hii Helo"
my_translate = string_1.maketrans("Helo", "byee")
print(string_1.translate(my_translate))
# Output
bii byee
zfill
zfill()
pad a numeric string with zeros on the left, to fill a field of the given width. The string is never truncated.
S = "7862"
S.zfill(6) -> 007862
S.zfill(5) -> 07862
S.zfill(2) -> 7862
splitlines
Returns a list of the lines in the string, breaking at line breaks( “\n” ). Line breaks are not included in the resulting list unless keep ends are given and true.
S = "hiii, \n welcome \n world"
print(S.splitlines())
# Output
['hiii, ', ' welcome ', ' world']
FAQ’S in strings
what is a string in python?
A sequence of elements typically characters, inside single or double quotes is called string. Even a single character inside the quotes is also called a string.
# Examples of strings
"Hello", "what's up", 'D'
How to create a string in python?
We can create a sequence of characters inside a single or double quote to a variable. Thus a string is created.
This sequence of characters inside the quotes can be anything such as numeric, alphanumeric, symbols, etc., It will be considered as a string if it’s enclosed between these quotes
# Examples of creating strings
str_1 = "Python 3.8"
str_2 = '2547'
str_3 = "a"
str_4 = "^%*&%$"
How to reverse a string in python?
There are several techniques for reversing a string. We can use for loop to iterate through the string in reverse and store the iterated values in a new string.
my_str = "hello world"
rev_str = ""
my_str_length = len(my_str) - 1
for i in range(my_str_length, -1, -1):
rev_str = rev_str + my_str[i]
print(rev_str)
# Output
dlrow olleh
How to convert a string to a list in python?
We can split a string using .split()
function which returns a list of strings being split using the parameter we pass into the function.
If no parameter is passed into the function then by default string is split using spaces.
S = "Hello Welcome to my house"
S.split() -> ['Hello', 'Welcome', 'to', 'my', 'house']
S.split("m") -> ['Hello Welco', 'e to ', 'y house']
How to turn a list into a string python?
.join()
function in python creates a string using the items of the list. We can pass the list as a parameter into the function.
my_list = ["h", "e", "l", "l", "o"]
"".join(my_list) -> hello
"*".join(my_list) -> h*e*l*l*o
How to remove characters from a string python?
To remove certain characters from the string we can use replace()
function which takes the characters/substring that needs to be removed and we can replace them with an empty string.
string = "Hello world welcome"
print(string.replace("o", ""))
# replaces only one character
print(string.replace("o", "", 1))
# Output
Hell wrld welcme
Hell world welcome
How to remove punctuation from a string python?
Punctuations are basically the symbols such as !()-[]{};:'”\,<>./?@#$%^&*_~. We can remove them using a for loop and replace them with empty string when the loop variable is punctuation.
string = "Hello world! welcome. password is :- @#DOLLar@#"
punctuation = '''!()-[]{};:'"\,<>./?@#$%^&*_~'''
for i in string:
if i in punctuation:
string = string.replace(i, "")
print(string)
# Output
Hello world welcome password is DOLLar
# Using regex
import re
string = "Hello world! welcome. password is :- @#DOLLar@#"
new_string = re.sub(r'[^\w\s]', '', string)
print(new_stirng)
# Output
Hello world welcome password is DOLLar
How to turn a string into an int python?
We can turn a string into an integer using the type-casting method. It is possible only if all the characters of the string are digits.
string = "89673"
if string.isdigit():
string = int(string)
print(string)
# Output
89673
How to slice a string in python?
To slice a string in python we can use the slice operator “[ : ]” and pass the start, end values for slicing the string.
string = "Hello world, welcome"
string[2 : 10] -> 'llo worl'
How to add to a string in python?
We can use concatenation operator( + ) to add character to a string.
string_1 = "Hello"
string_1 + "world" -> "Hello world"
How to check if a string is in a list python?
The membership operator in
can be used to check whether a string is in a list or not. It returns True if the string is in list or else returns False.
my_list = ["h", "e", "l", "o"]
"e" in my_list -> True
"w" in my_list -> False
How to check if a string contains a substring python?
To check if a substring is in a string or not we can use the membership operator.
my_string = "hello world"
"ello" in my_string -> True
How to print each character of a string in python?
To print each character of the string we can iterate over it using the for loop.
my_string = "helo"
for i in my_string:
print(i)
# Output
"h"
"e"
"l"
"o"
How to make a string lowercase in python?
To make all the characters of the string into the lower case we can use the inbuilt function .lower()
which converts all the uppers case letters into lower case. If a character is a lower case one then it remains the same.
string = "Hello worlD, welCOme"
string.lower() -> 'hello world, welcome'
How to capitalize a string in python?
To capitalize a string we can use .upper
function which returns the string with all the characters converted into upper case.
string = "Hello worlD, welCOme"
string.upper() -> 'HELLO WORLD, WELCOME'
How to get the length of a string in python?
Inbuilt function len()
returns the length of the sequence that we pass into it. We can pass the string as a sequence to know the length of the string.
string = "Hello"
len(string) -> 5
How to capitalize the first letter of a string in python?
.capitalize()
is used to return a capitalized version of the string. More specifically, it makes the first character have upper case and the rest of the string into lowercase.
S = "aehrcD"
S.capitalize()
# Output
'Aehrcd'
How to count the number of words in a string python?
To count the number of words in the string we can split the string using spaces and store the words in a list. Then using len()
function we can know the number of words present in the string.
string = "Hello worlD, welCOme"
my_list = string.split()
len(my_list) -> 3
How to sort a string in python?
To sort a string we can use an in-built method sorted()
that arranges the characters of the string based on the ASCII values and stores each character in the form of a list.
We can iterate through the list of characters and add the iterating values to form a new string that is sorted.
S = "Hello World"
print(S)
sort_s = sorted(S)
print(sort_s)
# Output
Hello World
HWdellloor
How to convert bytes to string in python?
Generally, bytes are stored in an encoded format, and to convert them into a string format we need to use .decode()
function.
# String in byte-encoded format
S = b'abcde'
# decode bytes to string
b"abcde".decode("utf-8") -> "abcde"
How to convert string to bytes in python?
Basically, everything in programming is stored by the memory manager in the form of bytes by default. If we want to convert a string into bytes then we need to use .encode()
function which stores the string in the form of bytes.
string_1 = "hello world"
string_1.encode() -> b'hello world'
How to compare strings in python?
To compare strings we need to use a comparison operator. This operator checks the ASCII values of the first character of the strings and compares them to return a result.
We can also check using is an operator that checks for the identity of the operands
"Hello" == "hello" -> False
"hi" is "hi" -> True
How to see if the string is equal to string python?
Two strings can be compared using comparison operators. To check whether the string is equal to other strings or not we need to use the equality operator( == ). If all the characters of the string are equal to other string then True is returned or else False is returned.
We can also use identity operator is
to evaluate equality between two strings.
string_1 = "hii"
string_2 = "hii"
if string_1 == string_2:
print("equal")
else:
print("not equal")
# Output
equal
In python, count how many times a word appears in a string?
In python, count()
function returns the number of times a word or a substring is present in the string.
s = "hi, welcome. hi how are you. hi hi"
s.count("hi") -> 4
How to add spaces in python string?
.join()
function can be used to add spaces in a string. But a sequence has to be passed as a parameter into the join function.
For this purpose, we use .split()
function which splits the strings into a list sequence.
S = "hii,How,are,you"
" ".join(S.split(","))
# Output
'hii How are you'
How to remove the first character of a string in python?
To remove the first character we can use .replace
function and replace it with an empty character. Since we need to replace only the first character we can pass “1”, into the function so that only once the replacement operation is performed.
S = "hi, welcome. hi how are you. hi hi"
print(S.replace(S[0], "", 1))
# Output
'i, welcome. hi how are you. hi hi'
How to insert variable into string python?
Using the string .format()
function we can customize the string with other strings or variables inside a pre-defined text.
The string format is done using a pair of curly braces inside a text. This text is then called using the .format()
method in which we can pass the variable or strings into it in an order in which curly braces are defined.
version = 3.7
print(f"I am using python {version}")
# Output
I am using python 3.7
How to check if a string is a palindrome in python?
A string is said to be a palindrome if the string parsed from left to right is equal to the string parsed from right to left.
We can just reverse the string using the slice operator and compare it with the original string to check whether the given string is a palindrome or not.
S = "abcba"
if S == S[::-1]:
print(f"{S} is a palindrome")
# Output
abcba is a palindrome
We can also iterate through the length of the string till the middle character and for every iteration check the corresponding character from another end. If both are equal for all the iterations then the string is a palindrome.
string = "abcdcba"
string_length = int(len(string)/2)
rev = -1
for i in range(string_length):
if string[i] == string[rev]:
rev = rev - 1
else:
break
if i == string_length - 1:
print("string is palindrome")
# Output
string is palindrome
How to remove vowels from a string in python?
Vowels are a, e, i, o, u. We can parse through the string to check whether a string has these characters or not.
If a string has these characters in it we can replace them with an empty character, so that all the vowels present in it will be removed.
S = "hi, welcome. hi how are you. hi hi"
vowels = "aeiou"
for i in S[:]:
if i in vowels:
S = S.replace(i, "", 1)
print(S)
# Output
h, wlcm. h hw r y. h h
How to get the last character of string in python?
To access any character in a string we need to use the index operator of the string. We can also get the last character of the string if we pass -1 into the index operator.
S = "abcbaabc"
S[-1] -> "c"
How to get the first character of a string in python?
To access any character in a string we need to use the index operator of the string. We can also get the first character of the string if we pass 0 into the index operator.
S = "abcbaabc"
S[0] -> "a"
How to generate a random string in python?
To generate a random string we can use the random module to generate random literals. We can keep repeating the generation of random value “n” number of times using a for loop.
We can use list comprehension to generate a sequence that can be combined together into a single string using .join()
function.
For more information about the string.ascii_letter
please read the proper documentation by clicking the link
import random
import string
random_string = "".join((random.choice(string.ascii_letters)) for i in range(5))
print(random_string)
# Output
"dFPUG"
How to check if the input is a string python?
By default, input()
function returns input provided by the user in the form of a string.
We can check the data type of any value using the type()
function which returns the class or data type of the variable we pass into it.
user_input = input()
print(type(user_input))
# Output
<class 'str'>
How to print a string multiple times in python?
To print a string multiple times we can use the print statement inside a for loop which iterates a certain number of times. On each iteration, the string is printed.
S = "Python"
for i in range(3):
print(S)
# Output
"Python"
"Python"
"Python"
How to find the index of a character in a string in python?
The index of a character in a string can be known using the .index()
function. It returns the lowest possible index of the character in the string.
Alternatively, we can also rindex()
which return the highest possible index of a character in the string.
S = "Python is Good"
S.index("o") -> 4
S.rindex("o") -> 12
S.index("t") -> 2
In python, how to remove newline from string?
To remove a new line from a string we can use replace()
function.
for i in "Python\n", "is \n Good":
if "\n" in i:
i = i.replace("\n", "")
print(i)
# Output
Python
is Good
How to extract numbers from a string in python?
To extract numbers from a string we need to iterate through the entire string by each character.
When iterating if we encounter any number then it has to be extracted. To check the value is a number or not, we need to associate a condition statement with .isnumeric()
inside the for loop.
string_1 = "hello 23, while 45"
for i in string_1:
if i.isnumeric():
print(i, end =" & ")
# Output
23 & 45
How to convert string to a dictionary in python?
To convert a string into a dictionary we need to assign a key for each character of the string. It can be done if we provide a flag value that keeps on increasing on every iteration of the string.
flag = 1
my_dict = {}
for i in "Hello":
my_dict[flag] = i
flag = flag + 1
print(my_dict)
# Output
{1: 'H', 2: 'e', 3: 'l', 4: 'l', 5: 'o'}
How to remove spaces in a string python?
To remove spaces we need to use replace() function that replaces all the whitespace with empty values.
my_string = "Hello World Welcome"
print(my_string.replace(" ", ""))
# Output
HelloWorldWelcome
How to strip a string in python?
.strip()
function strips the string and removes the trailing character from the string based on the substring that we pass as a parameter.
If we do not pass any character then by default trailing empty spaces will be removed from the string.
S = "Hello Welcome"
S.strip("e")
'Hello Welcom'
How to check for empty strings in python?
Empty strings do not have any characters in them. Thus the length of the string is “Zero”. So when a string length is returned as zero it means the string is empty.
String_1 = ""
if len(string_1) == 0:
print("empty string")
else:
print(strin_1)
# Output
empty string
How to concatenate int and string in python?
To concatenate any value to a string it has to be in string format only. So to concatenate an int value to a string it has to be type-casted into a string and then concatenated.
value_1 = "Python"
int_value = 3.7
value_1 + str(int_value) -> "Python3.7"
value_1 + int_value -> TypeError: can only concatenate str to str
How to find the longest string in a list python?
To check for the longest string then we need to track the lengths of these strings so that we can evaluate the string with the highest length will be the longest string.
str_1 = "ab"
str_2 = "a"
str_3 = "evrf"
str_4 = "abc"
long_list = [str_1, str_2, str_3, str_4]
highest = ""
for i in long_list:
if len(i) > len(highest):
highest = i
print(highest)
# Output
"evrf"
In python, how to count vowels in a string?
To count the number of vowels in a string we can create a COUNTER that keeps on incrementing when a vowel is encountered while iterating through the string.
S = "hi, welcome. hi how are you. hi hi"
vowels = "aeiou"
count = 0
for i in S:
if i in vowels:
count = count + 1
print(count)
# OUTPUT
12
How to format a string python?
Using .format()
we can customize the string with other strings or variables inside a pre-defined text.
print("Hello, i am using python {}".format(3.7))
# Output
Hello, i am using python 3.7
How to remove commas from a string in python?
To remove commas from a string we can use .replace()
function.
my_string = "Hello, world, welcome"
print(my_string.replace(",", ""))
# Output
Hello world welcome
What symbol is used to mark the beginning and end of a string in python?
Strings are enclosed between single, double, triple quotes. A pair of these quotes make the beginning and end of the string.
# Strings
'abc', "abc", '''abc'''
Why do you say .lower() instead of lower(string) python?
.lower()
is a method in the String class used to convert upper case letters into lower case. Since the methods inside a class can be invoked only using the dot operator( .
) we use .lower() instead of lower(string).
How to put aaa bbb ccc and soo on into a string list in python?
To generate alpabets from a-z we pass the ascii values of character a
and z
as range into for loop.
.append()
function appends any item into the list. To put a string into a list we need to pass the string into the .append
function.
my_list = []
for i in range(97, 123):
my_list.append(chr(i)*3)
print(my_list)
# Output
['aaa', 'bbb', 'ccc', 'ddd', 'eee', 'fff',
'ggg', 'hhh', 'iii', 'jjj', 'kkk', 'lll',
'mmm', 'nnn', 'ooo', 'ppp', 'qqq', 'rrr',
'sss', 'ttt', 'uuu', 'vvv', 'www', 'xxx',
'yyy', 'zzz']
How to count the number of uppercase letters in a string in python?
.isupper()
function returns True if the character is an uppercase and returns false if it’s a lower case.
We can iterate over a string and check the iterating item is an upper case or not by associating the .isupper()
with an if condition.
my_string = "HellO, WorlD, WelcOme"
count = 0
for i in my_string:
if i.isupper():
count = count + 1
print(count)
# Output
6
How to represent a tab in a string python?
‘\t’ is used to represent a tab space. We can insert a \t inside a string to represent a tab space.
my_string = "HellO,\t WorlD, \t WelcOme"
print(my_string)
# Output
"HellO, WorlD, WelcOme"
How to include addition and subtraction sign as a string input() in python?
input()
function in python accepts the user input in string format. We can input +
or -
and evaluate an if condition to perform addition when the user enters +, and – to perform subtraction.
input_string = input()
a, b = 5, 4
if input_string == "+":
print(a + b)
if input_string == "-":
print(a - b)
# Input # Output
+ 9
- 1
In python, how to split string by a list of delimiter?
If there is a list of delimiters we need to pass these as a regular expression using re.split()
function. This list of delimiters is separated using “|”.
import re
my_str = "Hey, Pyhton is a: good language; I like that language"
print(re.split('; |, |:', str))
# Output
['Hey', 'Pyhton is a', ' good language', 'I like that language']
How to turn string seven into 7 python?
In python, the word2number
module converts word numbers into digits. we can import the module using the command from word2number import w2n
.
from word2number import w2n
normal_string = "four five two seven"
result_string = w2n.word_to_num(normal_string)
print(result_string)
# Output
4527
How to count how many times each word occurs in a string in python?
To count the number of times each word occurs in a string we need to iterate through the words in the string and create a counter that gets incremented whenever the loop variable value is equal to the word that needs to be counted.
At the end we can assign the word as key and the number of time it occurred as a value.
dictionary = {}
word_string = "hi john hi john hi danny hi walden"
word_list = word_string.split()
for i in word_list:
count = 0
for j in word_list:
if j == i:
count = count + 1
dictionary[i] = count
print(dictionary)
# Output
{'hi': 4, 'john': 2, 'danny': 1, 'walden': 1}
How to break a string onto 2 lines in python?
Once a string is created we cannot modify it since strings are immutable. To break a string into 2 lines we can insert a new line(” \n “) using the concatenation operator and re-assign the value to the string.
my_string = "Hey, Pyhton is a good language; I like that language"
new_string = my_string[:31] + "\n" + my_string[32:]
print(new_string)
# Output
Hey, Pyhton is a good language;
I like that language
Which function converts an integer to hexadecimal string in python?
hex()
function in python converts an integer into a hexadecimal string. The integer number has to be passed as a parameter into hex()
.
num_int = 2425
num_hex = hex(num_int)
print(num_hex, type(num_hex))
# Output
0x979 <class 'str'>
How to return the most used character in a string python?
.count()
function returns the most used character in the string. We need to create a list of all the characters without duplicates and then iterate through them to get a count of each character which can be used to evaluate the most used character in the string.
my_string = "heello world, welcomee"
char_list = []
for i in my_string:
if i not in char_list:
char_list.append(i)
count = 0
for i in char_list:
char_count = my_string.count(i)
if char_count > count:
count = char_count
max_char = i
print(max_char, "->", count)
# outpt
e -> 5
How to skip over a character iteration in a string python?
To skip over a character during iteration we need to use the continue
keyword such that when the loop has encountered a value equal to the character that needs to be skipped we can use the continue keyword at that point.
string_iterate = "Hii, Welcome"
for i in string_iterate:
if i == "e":
continue
In python, how to combine two non-string values?
To combine two non-string values we need to type-caste them using the str()
function which converts any value into string type and then combine them using a concatenation operator. Thus we can combine any number of values.
item_1 = 34
item_2 = 56
str(item_1) + str(item_2) -> '3456'
In python, how to check if a number exist in a string?
First, the number has to be converted into a string type using the str()
function and then to check the value to be present in a string or not we can use the membership operator( in
). It returns True if the value is a member of the string else it returns False.
my_string = "hii john34, welcome to area 51"
num = 5
str(num) in my_string -> True
How to access the second to last index of a string in python?
To access any substring from the string we can use the slice operator. Since we need to access the second to the last index we need to pass start index = -2.
my_string = "hii john, welcome to house"
my_string[-2] -> "s"
How to remove multiple spaces from string in python
To remove multiple spaces from a string we need to use .replace()
and pass space as the first parameter and empty string as the second parameter. Thus empty spaces are removed and replaced with an empty string.
my_string = "hii welcome to python"
my_string = my_string.replace(" ", "")
print(my_string)
# Output
hiiwelcometopython
How to find the first vowel in a string python?
To find out the first vowel in a string we need to iterate through the string and stop iteration when the iterating value is a vowel. To stop the iteration we use the break statement.
my_string = "hii welcome to python"
for i in my_string:
if i in "aeiou":
print("the first vowel in the string is: ",i)
break
# Output
the first vowel in the string is: i
How to print letters in a string on different lines python?
To print each letter in a different line we can iterate over the string using for loop and print each iteration value.
my_string = "hii"
for i in my_string:
print(i)
# Output
h
i
i
How to read dictionary string data python?
Dictionary strings are basically in json format. To read the json data we need to convert the string into readable format of a dictionary. We can use json.loads()
and pass the json string into a python object.
import json
my_string = '{1 : "world", 2 : 3, "hi" : "welcome"}'
my_dict = json.loads(my_string)
print(my_dict)
# Output
{1 : "world", 2 : 3, "hi" : "welcome"}