An Exception is an unwanted event that is caused during the execution of the program. This unwanted event that disturbs the normal flow of the program is called an exception.
Examples:- Zero Division Error, Type Error, ValueError, FileNotFoundError, etc.,
There are two types of errors, they are:-
- Syntax error
- Runtime error
Syntax error
The errors that are caused due to invalid syntax are known as syntax errors. The user is responsible for syntax errors and these errors need to be corrected to start the execution of the program.
# Colon missing in "if" clause caused syntax error
x_value = 45
if x_value < 20
print("value is low")
SyntaxError : invalid syntax
Runtime error
While executing the program if something goes wrong because of the programmer or program logic or memory problem, etc., runtime errors occur. These runtime errors are also called Exceptions.
print(2 + "hello")
TypeError: unsupported operand type(s) for +: 'int' and 'str'
Exception Handling
Since exceptions are raised due to runtime errors, it is highly recommended to handle these exceptions to avoid Non-Graceful Termination of the program.
Exception handling is not making changes to the program to avoid the exception, it is rather providing an alternate way for the program execution when an exception is raised such that graceful termination occurs.
The below are the few points that describe the characteristics of the exception.
- Every exception in python is an object. For every exception object to handle them there are corresponding classes
- When the exception occurs the python virtual machine will create the corresponding exception object and will check for handling code.
- If the handling code is not available then python interpreter terminates the program abnormally and prints corresponding exception information to console. The rest of the program wont be executed
- All exception classes are child classes of BaseExceptio. Every exception class extends BaseException either directly or indirectly. Hence BaseException acts as root for Python Exception Hierarchy
- Most of the time being a developer we have to concentrate Exception and its child classes
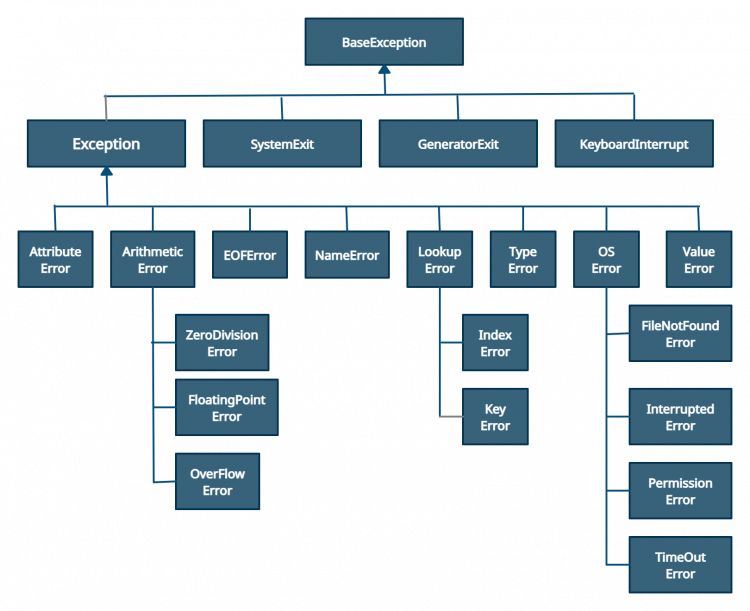
Customizing Exception Handling
We can customize the exceptions using try-except blocks. When there is a probability that a part of the code might interrupt the normal flow of the program or cause abnormal termination then it can be declared in the try block.
In the below example, if we divide any number by zero the interpreter throws an error. To handle such an error we can create an except block such that there is an alternative way for the program to get executed.
print(5/0)
# Output
ZeroDivisionError: division by zero
To handle the exception caused by the try block, we can use the except block to handle these errors.
try :
a = 5/0
except:
print("except block")
# Output
except block
We can customize the except block such that when a particular error is faced it can be handled by the corresponding exception block.
In the below example, when a ZeroDivisionError occurs the control is passed to the corresponding except block. Thus abnormal termination of the program is handled by the except block.
try :
a = 5/0
except ZeroDivisionError:
print("can't divide by Zero")
Control Flow of try-except block
Let’s see a few examples to check how the try and except blocks are getting executed.
- If a try block is declared it has to be followed by an except block. When there few statements between
try
andexcept
blocks, program throws invalid syntax error.
try :
a = 5/0
print("middle statement")
except:
print("except block")
# Output
Invalid syntax
- When the control enters the try block and if all the statements inside
try
are executed without any error, then execution of except block is skipped
try :
print("statement_1")
print("statement_2")
except Exceptions:
print("statement_3")
print("statement_4")
# Output
statement_1
statement_2
- If the exception is raised by executing a statement inside the
try
block then execution of remaining statements are skipped and control passed to except block
try :
print("statement_1")
a = 5/0
print("statement_2")
except ZeroDivisionError:
print("statement_3")
# Output
statement_1
statement_3
- If the exception raised in a
try
block is not handled by theexcept
block then it results in abnormal termination of the program
try :
print("statement_1")
a = 5/0
print("statement_2")
except FloatingPointError:
print("statement_3")
print("statement_4")
# Output
statement_1
ZeroDivisionError: division by zero
Note:- When the exception is raised in the except block itself then it results in abnormal termination of the program
Exception Information
Exception information tells us what kind of exception occurred and the class to which the exception belongs. To know the exception type we need to create a reference to the exception object.
We can reference the exception object using as
operator. This reference tells us the exception type that has occurred.
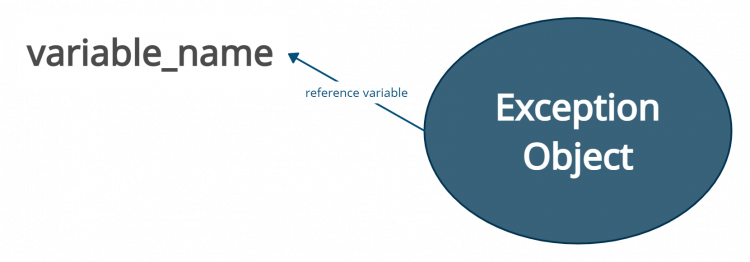
- variable_name returns what exception has raised
- variable_name.__class__ return the exception class that it belongs to
- variable_name.__class__.__name__ returns the type of exception
In the below example, we are performing a division operation of two numbers. If we try to perform zero division let’s see how the exception handles it.
try:
a = int(input())
b = int(input())
print(a/b)
except BaseException as exception:
print("the exception is", exception)
print("the exception type is :", exception.__class__.__name__)
print("the exception class is :", exception.__class__)
# Input
5
0
# Output
the exception is division by zero
the exception type is : ZeroDivisionError
the exception class is : <class 'ZeroDivisionError'>
But when we perform a division operation between an integer and string the exception type, exception classes are returned as follows.
try:
a = int(input())
b = int(input())
print(a/b)
except BaseException as exception:
print("the exception is", exception)
print("the exception type is :", exception.__class__.__name__)
print("the exception class is :", exception.__class__)
# Input
3
e
# Output
the exception is invalid literal for int() with base 10: 'e'
the exception type is : ValueError
the exception class is : <class 'ValueError'>
try with multiple except blocks
When there are multiple except blocks then the python interpreter executes the except block that can handle the exception.
If the exception is not raised in the try block then the multiple except blocks are not executed. In the below example, the exception is not raised so the except blocks are not executed.
try :
a = 6/2
print(a)
except ZeroDivisionError:
print("statement_3")
except FloatingPointError:
print("statement_4")
# Output
3.0
try with multiple except blocks available then based on raised exception the corresponding except block will be executed.
try :
a = 6/0
print(a)
except FloatingPointError:
print("floating point error")
except ZeroDivisionError:
print("can't divide by zero")
# Output
can't divide by zero
When try with multiple except blocks are declared, if an exception raised in try
block can be handled by two or more except
blocks then the Python interpreter will always consider from top to bottom until matched except block identified.
In the below example, the Python interpreter considers the topmost except block that can handle the exception.
try :
a = 6/0
print(a)
except ArithmeticError:
print("Its a arithmetic error")
except ZeroDivisionError:
print("can't divide by zero")
# Output
Its a arithmetic error
try :
a = 6/0
print(a)
except ZeroDivisionError:
print("can't divide by zero")
except ArithmeticError:
print("Its a arithmetic error")
# Output
can't divide by zero
Single Except block handling Multiple Exceptions
If the handling code is the same, then instead of declaring multiple except blocks with the same code inside them we can declare all these exception objects in a single tuple and pass it to the except block.
In this way, we can reduce the number of lines of code. But we have to make sure, the handling code of all these exceptions has been the same.
In the below example, the exception blocks are the same for exception_1 and exception_2. Both of them are returning the name of the exception type using the reference variable of the exception object.
try :
a = int(input())
b = int(input())
print(a/b)
except ZeroDivisionError as msg:
print("It's a exception", msg.__class__.__name__)
except ValueError as msg:
print("It's a exception", msg.__class__.__name__)
Since the code block is the same for both the exceptions we can pass them as a tuple, such that based on the exception raised it will be handled by the exception object.
Note:- When we pass multiple exception objects in a single exception block then we need to send inside parenthesis as a tuple.
try :
a = int(input())
b = int(input())
print(a/b)
except (ZeroDivisionError, ValueError) as msg:
print("It's a exception", msg.__class__.__name__)
# Input # Output
5, 0 It's a exception ZeroDivisionError
4, 's' It's a exception ValueError
Default exception block
There might be a chance when the exception block might not be able to handle the exceptions that were raised. This might cause the abnormal termination of the program. So, in this case, we can declare a default exception block at the end of all the exceptions.
Thus when exceptions that aren’t handled by the exception blocks will be handled by the default exception block.
try :
----
code block
----
except ZeroDivisionError:
code block_1
except ValueError:
code block_2
except:
default exception block
- In the below example, we are performing division between two numbers. If a user enters values other than numbers then we cannot perform division which returns an exception known as ValueError.
- But our code block cannot handle the exception raised, so the control is passed to the default exception block that is declared at the end.
try :
a = int(input())
b = int(input())
print(a/b)
except ZeroDivisionError:
print("cannot divide by zero")
except:
print("enter valid input")
# Input # Output
5, i enter valid input
If the raised exception can be handled by the exception block, then the default exception block is not executed.
try :
a = int(input())
b = int(input())
print(a/b)
except ZeroDivisionError:
print("cannot divide by zero")
except:
print("enter valid input")
# Input # Output
5, 0 cannot divide by zero
We have to make sure the default exception block has to be declared at the end of the exceptions. If we write any except blocks after the default except block then the Syntax error is raised.
To avoid such syntax errors, the default except block has to be declared in the last.
# Syntax error is raised if we write any
# exception blocks after the default exception block
try :
a = int(input())
b = int(input())
print(a/b)
except:
print("enter valid input")
except ZeroDivisionError:
print("cannot divide by zero")
SyntaxError: default 'except': must be last
try :
a = int(input())
b = int(input())
print(a/b)
except ValueError:
print("enter int type")
except ZeroDivisionError:
print("cannot divide by zero")
except:
print("enter valid input")
finally block
In try block when there is an exception raised the statements placed after the line that is responsible for an exception are not executed. These statements might be clean up code such as Resource Deallocating code(close DB connection, close filehandle, etc.,).
If this cleanup code is not executed a resource will be always wasted handling a connection. In this case, we can use the finally block.
try:
statement_1
except:
statement_2
finally:
statement_3
When a few statements need to be executed for sure, then we need to place them in finally block.
- It is not recommended to have the clean up code inside except block, SInce the except block wont be executed if there is no exception. In this case cleanup code is not executed
- Hence we required some place to maintain clean up code which should be executed always irrespective of whether exception raised or not raised and whether exception handled or not handled.
- Such type of block that gets executed irrespective of exception raised or not is the
finally
block - Hence the main purpose of
finally
block is to maintain clean up code.
The below example, shows the finally block gets executed even though the exception block is not executed.
try:
print("it's a try block")
except:
print("it's a exception block")
finally:
print("it's a finally block")
# Output
it's a try block
it's a finally block
When there is an exception raised then control is passed to except block. If the exception is handled by the except block, even in this case also finally block
is executed.
try:
a = 5 / 0
print(a)
except ZeroDivisionError:
print("cannot divide by zero")
finally:
print("finally block")
# Output
cannot divide by zero
finally block
There might be a situation where the exception is raised by the try block and not handled by the except block, in such cases also finally block is executed. After the finally block execution then the program is terminated with an exception returned as an error.
try:
print("try block")
a = 5 / 0
print(a)
except ValueError:
print("value error")
finally:
print("finally block")
# Output
try block
finally block
ZeroDivisionError: division by zero
os._exit(0) vs finally block
There is only one situation where finally block is not executed when the program is terminated by some code. We can terminate the program using os._exit(0).
Whenever we are using os._exit(0)
then Python Virtual Machine gets itself shut down. In this case, finally block won’t be executed.
os._exit(0)
- value inside the os._exit() represents the status code
- If the status code is ‘0’ then the Python Virtual Machine it shutdown normally
- If the status code is non-zero value then Python Virtual Machine it shutdown abnormally
- Since this shutdown occurs internally in the Python Virtual Machine there isn’t any difference on the user side, the program is terminated
import os
try:
print("try block")
os._exit(0)
except:
print("Exception")
finally:
print("finally block")
# Output
try block
finally vs destructors
- finally is used to deallocate the resources that are created in try block
- Used for activities that are pending in the try block
- When a finally block is declared it’s execution is independent of the try-except blocks
- destructor is also used to deallocate the resources created for a object
- destructor delete an object references
- When a destructor is called whatever resources associated to a object are deallocated inside destructor
Control Flow in try-except-finally
No matter whether there is an exception raised or not, whether the program is terminated or not, finally
block will be executed for sure. Let’s check out a few examples of how the control flow is going to be among try-except-finally on different scenarios.
- When there is no exception raised in try-block, exception block is not executed and finally block gets executed and program results in normal termination.
try:
print("try block")
a = 5/2
print(a)
except:
print("Exception")
finally:
print("finally block")
# Output
try block
2.5
finally block
- When there is exception raised in try block and the exception is handled by the except block and finally block gets executed with normal termination of the program
try:
print("try block")
a = 5/0
print(a)
except ZeroDivisionError:
print("Exception: cannot divide by zero")
finally:
print("finally block")
# Output
try block
Exception: cannot divide by zero
finally block
- When exception raised in try block and the exception is not handled by the except block, finally block gets executed and then program ends with abnormal termination
try:
print("try block")
a = 5/0
print(a)
except ValueError:
print("value error")
finally:
print("finally block")
# Output
try block
finally block
ZeroDivisionError: division by zero
- If the exception raised in exception block then it always result in abnormal termination of the program by the finally block gets executed before the termination
- If an exception raises in finally block then it’s always a abnormal termination
else block with try-except-finally
We can also declare an else block with try-except-finally blocks. In such a case else block is executed if there is no exception raised in the try block.
If we declare else block in try-except-finally, then the order of writing the blocks is:-
- try -> except -> else -> finally
The finally block has to be the last block while using try-except blocks and if we use else block with try-except blocks then it has to be declared immediately after the except block.
Note:- If there is no exception raised in a try block or the exception block is not executed then only else block is executed
In the below code, there is no exception raised in try block thus statements associated with a try, else, finally, blocks are executed and result in normal termination of the program. The else block is executed because there is no exception raised in the try block.
try:
print("try block")
except:
print("except block")
else:
print("else block")
finally:
print("finally block")
# Output
try block
else block
finally block
If there is an exception raised in the try block then the exception is handled by the except block then else block is not executed but finally block is executed.
Here, finally block is executed since it is independent of the execution of the try-except blocks.
try:
print("try block")
div = 5/0
except ZeroDivisionError:
print("except block")
print("Division error")
else:
print("else block")
finally:
print("finally block")
# Output
try block
except block
Division error
finally block
If the exception is raised in a try block and is not handled by the except block then else block is not executed but finally block is executed.
Here, the else block is not executed since the exception is raised in a try block, and the control is passed to except block, even though it is not handled by the corresponding exception block, but it results in else block not getting executed.
try:
print("try block")
div = 5/0
except ValueError:
print("except block")
else:
print("else block")
finally:
print("finally block")
# Output
try block
finally block
div = 5/0
ZeroDivisionError: division by zero
Nested try-except-finally blocks
We can declare try-except-finally blocks inside a try or finally block. Thus nesting of try-except-finally is allowed.
If a nested try-except-finally is declared inside a try block, the risky code is placed inside an inner try block and it will be handled by the inner exception block such that all the statements inside the try block are executed.
In the below example, there might be a chance that an exception might be raised in statement_2
, thus we have placed it in the inner try block so that it will be handled by the inner exception block.
In this way the statement_4
is executed, thus all the statements of the try block will be executed and any exceptions raised in the outer try block will be handled in the try block itself.
try:
statement_1
try:
statement_2
except <exception>:
statement_3
statement_4
except <exception>:
statement_5
If the exception raised in the inner try block is not handled by the inner except block then the control is passed to the outer except block.
In the example below, all the statements of the outer-try block are getting executed thus no statement is being skipped. This is the use of nested try-except-finally blocks.
try:
print("outer-try")
try:
print("inner-try")
print(5/0)
except ArithmeticError:
print("inner-except")
finally:
print("finally block")
print("end of try block")
except BaseException:
print("outer-except block")
finally:
print("outer-finally")
# Output
outer-try
inner-try
inner-except
finally block
end of try block
outer-finally
Control flow in Nested try-except-finally
Based on the exception raised corresponding except block will be handling the exception. If any except block could not handle the exception then program execution results in abnormal termination.
In the below code, we have nested try-except-finally blocks. Let’s check the control flow based on different scenarios.
try:
statement_1
statement_2
try:
statement_3
except <exception>:
statement_4
finally:
statement_5
except <exception>:
statement_6
finally:
statement_7
- If no exception raised in try block then statements – 1, 2, 3, 5, 7 are returned with normal termination of the program
- If exception raised in
statement_3
and handled by correspondign except block then statements – 1, 2, 4, 5, 7 are returned with normal termination of the program - If exception raised in
statement_3
and not handled by the corresponding inner except block but handled by the outer except block then statements – 1, 2, 5, 6, 7 are returned with normal termination of program - If exception raised in
statement_3
and not handled by both inner and outer blocks then statements – 1, 2, 5, 7 are returned with abnormal termination of the program - If exception raised in
statement_2
and corresponding outer except block matched then statements – 1, 6, 7 are returned with normal termination of the program - If exception raised in
statement_2
and not handled by corresponding outer except block then statements – 1, 7 are returned and results in abnormal termination of the program - In the whole program if the control enteres any try block either outer or inner then their corresponding
finally
blocks are executed before termination of the program
Pre-defined and Customized Exceptions
There are two types of exceptions, they are:- Pre-defined Exceptions and Customized Exceptions
Pre-defined Exceptions
Pre-defined Exceptions are those that are in-built exceptions. These are known by the Python Virtual Machine and based on the kind of exception raised they are returned. The different kinds of pre-defined exceptions are ZeroDivisionError, ValueError, ArithmeticError, IndexError, KeyError, etc.,
Below are the use-cases of different pre-defined exceptions:-
- Whenever we try to perform division of any number with zero then automatically PVM returns
ZeroDivisioError
- When the user inputs value other than required value then PVM returns ValueError
- If we try to open a file that doesnot exist then PVM returns FIleNotFoundError
- If we try to access a string beyond its index then PVM returns IndexError
div = 5/0 -> ZeroDivisionError
# file is not found in the location
f = open("C:\Users\abc.txt", 'r') - FileNotFoundError
S = "abc"
S[5] -> IndexError
Customized Exceptions
The Customized Exceptions are defined by the user and are also known as Programmatic Exceptions.
When we have to define and raise exceptions explicitly to indicate that something goes wrong such types of exceptions are called Customized Exceptions.
Programmers have to raise these exceptions by defining them using the raise
keyword. But the user-defined exception has to inherit from the Exception class since python does not know whether it’s an exception or not.
In the below example, we raise an exception if the user enters a value less than 10. Then based on the condition evaluation we call the exception class that we have defined. We have to make sure the exception class that we have defined is inherited from the Exception class.
class customexception(Exception):
def __init__(self,arg):
self.arg=arg
x = int(input())
if x >= 10:
print(x)
elif x < 10:
raise customexception("x is very less")
# Input # Output
15 15
2 customexception: x is very less
FAQ’s in Exceptions – Python
How to Raise an Exception in Python
To raise an exception raise
keyword can be used. Raising an exception is also called throwing an exception.
x = int(input("enter a number"))
if x < 0:
raise Exception("Sorry,negative numbers are not accepted")
Output
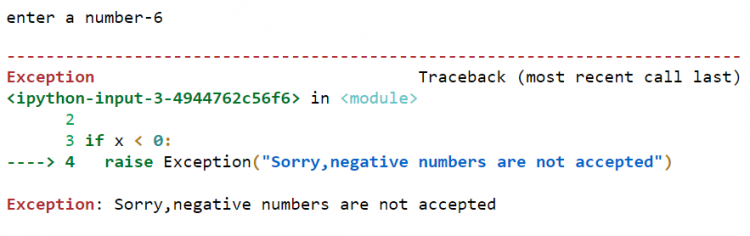
How to Catch an Exception in Python
The try and except class can be used to catch and handle exceptions in python.
try:
print(y)
except:
print("An exception occurred\nAs the variable is not declared!")
Output
An exception occurred
As the variable is not declared!
How to Print Exception in Python
The print()
function can be used in except block to print the exception.
try:
res=1/0
except:
print("divide by zero error")
Output
divide by zero error
How to Handle Exception in Python?
The try, except, and finally block can be used to handle exceptions in python.
try:
k = 5//0
print(k)
except ZeroDivisionError:
print("Can't divide by zero")
finally:
print('Finally block is always executed')
Output
Can't divide by zero
Finally block is always executed
What is an Exception in Python
An exception is an event that occurs during program execution and disrupts the normal flow of the program’s instructions. When a Python script encounters a situation that it cannot handle, it throws an exception. A Python object that represents an error is known as an exception.
How to get Exception Details in Python
The module logging.exception()
can be used to get details about exceptions.
import logging
try:
1/0
except BaseException:
logging.exception("An exception was thrown!")
Output
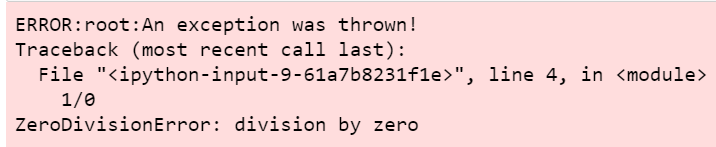
How to Trace an Exception in Python
The print_exc()
function from traceback library can be used to trace an exception.
import traceback
A = [1, 2, 3, 4]
try:
value = A[5]
except:
traceback.print_exc()
print("end of program")
Output

How to Restart Python Program After Exception
By calling the main()
inside a try block and using the continue keyword in the except block it can be used to restart the program after exception.
import numpy as np
def main():
np.load('File.csv')
for i in range(1, 3):
try:
main()
except Exception as e:
print(e)
print('Restarting the program!')
continue
else:
break
Output
[Errno 2] No such file or directory: 'File.csv'
Restarting the program!
[Errno 2] No such file or directory: 'File.csv'
Restarting the program!
How to Throw Index Error Exception in Python
The index error is thrown, when we try to access an index that is not present.
sample = [1,2,3]
try:
sample[4]
except Exception as e:
print(e)
Output
list index out of range
Which of the following Except Classes will Catch a built-in Python Exception Type
The built-in python exception type can be raised from the try block and handled in the except block.
sample = [1,2,3]
try:
sample[4]
except IndexError:
print("an index error!")
Output
an index error!
How to Store Exception in a Variable using Python
The use of as
keyword in except block can be used to store exception body in a variable.
try:
raise Exception("Storing Exception in a Variable..!")
except Exception as x:
print(x)
Output
Storing Exception in a Variable..!
What is Used for Exception Handling in Python
Exceptions in Python can be handled with a try statement. The try clause contains the critical operation that can cause an exception. The except clause contains the code that handles exceptions.
import sys
random = ['a', 0, 2]
for entry in random:
try:
print("The entry is", entry)
r = 1/int(entry)
break
except:
print("Oops!", sys.exc_info()[0], "occurred.")
print("Next entry.")
print()
print("The reciprocal of", entry, "is", r)
Output
The entry is a
Oops! <class 'ValueError'> occurred.
Next entry.
The entry is 0
Oops! <class 'ZeroDivisionError'> occurred.
Next entry.
The entry is 2
The reciprocal of 2 is 0.5
Why Python Need Try and Exception
The try block allows you to test a section of code that may cause errors. The except block allows you to handle errors. The finally block lets you execute code, regardless of the result of the try and except blocks.
try:
print(z)
except NameError:
print("Variable z is not defined")
finally:
print("finally will always be executed!")
Output
Variable z is not defined
finally will always be executed!
Does Python Load Any Package through Exception
Yes, the python loads the packages through try and except block. If the package is not found, the corresponding exception is raised and handled.
from importlib import import_module
libnames = ['numpy', 'scpy', 'operator']
for libname in libnames:
try:
lib = import_module(libname)
except:
print(libname,"module is not found")
else:
globals()[libname] = lib
Output
scpy module is not found
How to Continue Loop after Exception in Python
The use of the continue
keyword in the try block, within the loop, can be used to continue the loop after exception.
alphabet = ['a' , 'b' , 'z' , 'b' ]
for letter in alphabet:
try:
if letter == 'b' :
print(letter)
continue
else:
raise ValueError
except(ValueError):
print("error")
Output
error
b
error
b
How to Prevent Too Broad Exception in Python
The basicConfig()
function from logging module can be used to prevent the too broad exception.
import logging
logging.basicConfig()
# noinspection PyBroadException
try:
raise RuntimeError('Bad stuff happened.')
except Exception:
logging.error('Failed.', exc_info=True)
Output

How to Raise Value Error Exception in Python
Value error happens when we try to convert non-compatible data types. To throw a ValueError exception with the error message text, use the syntax raise exception with exception as ValueError(text)
.
try:
num = int("string")
except ValueError:
raise ValueError("ValueError exception thrown")
Output
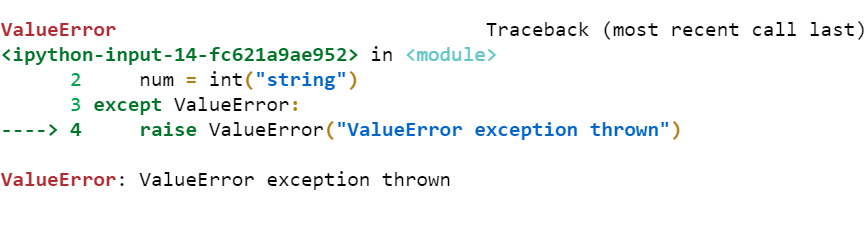
How to Write Exception Class in Python
The user-defined exception class that derives from the Exception class, has been generated. This new exception, like existing exceptions, can be raised with an optional error message using the raise command.
class Error(Exception):
pass
class ValueSmallError(Error):
pass
class ValueLargeError(Error):
pass
number = 10
while True:
try:
i_num = int(input("Enter a number: "))
if i_num < number:
raise ValueSmallError
elif i_num > number:
raise ValueLargeError
break
except ValueSmallError:
print("This value is too small, try again!")
print()
except ValueLargeError:
print("This value is too large, try again!")
print()
print("Congratulations! You guessed it correctly.")
Output
Enter a number: 90
This value is too large, try again!
Enter a number: 2
This value is too small, try again!
Enter a number: 10
Congratulations! You guessed it correctly.
How to Check Exception in Python
Using the function assertRaises(exception, function, *args, **keywords)
, just pass the exception, the callable function, and the parameters of the callable function as keyword arguments that will elicit the exception.
import unittest
class MyTestCase(unittest.TestCase):
def test_1(self):
with self.assertRaises(Exception):
1 + '1'
if __name__ == '__main__':
unittest.main()
Output
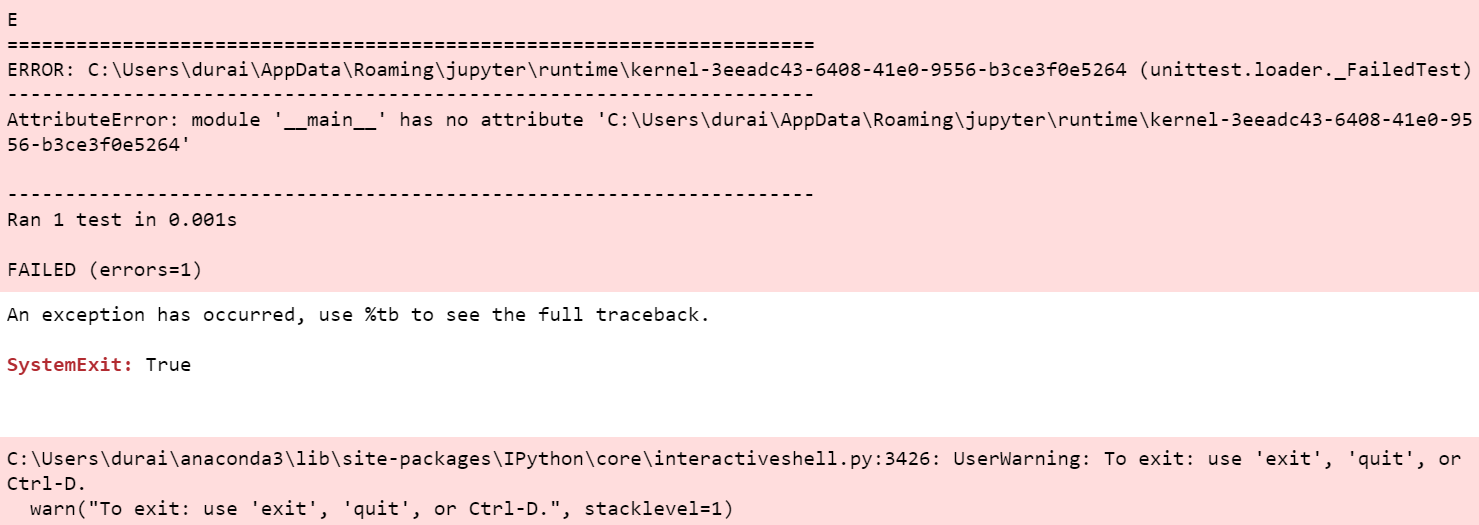
How to Raise a User-Defined Exception in Python
We can raise a user-defined exception by defining a user-defined exception class derived directly or indirectly from the main exception class.
class MyError(Exception):
def __init__(self, value):
self.value = value
def __str__(self):
return(repr(self.value))
try:
raise(MyError(3*2))
except MyError as error:
print('A New Exception occured - Exception number: ',error.value)
Output
A New Exception occured - Exception number: 6
Why should we Use Exception Handling in Python
An exception is an occurrence during the execution of a program that causes the normal flow of the program’s instructions to be disrupted. When a Python script comes across a circumstance that it can’t handle, it raises an exception. A Python object that describes an error is called an exception.
When a Python script throws an exception, it has two options: handle the exception right away or terminate and quit.
If there is any questionable code that could cause an exception, it can be protected by putting it in a try block. Include an except statement after the try block, followed by a block of code that handles the situation as much as possible.
How to Capture an Exception in Python
The use of as
keyword in except block can be used to capture exception body in a variable.
try:
raise Exception("Storing Exception in a Variable..!")
except Exception as x:
print(x)
Output
Storing Exception in a Variable..!
How to handle Exception when Working with File in Python
The IOError exception class can be used to handle an exception that arises when working with the file.
try:
f = open('random.txt', 'r')
print(f.read())
f.close()
except IOError:
print('file not found')
Output
file not found
What Exceptions can Socket Close Raise in Python
The normal exceptions for invalid argument types and out-of-memory conditions can be raised. The errors related to socket or address semantics raise OSError
or one of its subclasses from socket.error.
import socket
s = socket.socket()
s.settimeout(5)
try:
s.connect(('123.123.123.123', 12345))
except(socket.error):
print("Caught exception socket.error ")
Output
Caught exception socket.error
How to Append Information to Exception in Python
The attribute message
can be used to append information to the exception.
def bar(arg1):
try:
raise IOError('Stuff')
except Exception as e:
raise type(e)(e.message + ' happens at %s' % arg1)
bar('arg1')
Output
Traceback (most recent call last):
File "test.py", line 13, in <module>
bar('arg1')
File "test.py", line 11, in bar
raise type(e)(e.message + ' happens at %s' % arg1)
IOError: Stuff happens at arg1
How to Raise an Exception on User Input in Python
The ValueError can be raised to handle the exceptions on user input.
status =input('What is your status?')
try:
if(status == "citizen" or status == "legal" or status == "illegal"):
print("Success")
else:
raise ValueError
except(ValueError):
print("enter only the correct option")
Output
What is your status?legal
Success
What is your status?hello
enter only the correct option
What is too many redirects exception in Python-requests
The error of too many redirects indicates that the website is being transferred between several locations in an inefficient manner. This is frequently caused by competing redirects, such as one attempting to impose HTTPS (SSL) and the other diverting back to HTTP (non-SSL), or between the www and non-www forms of the URL.
How to stop running a program when an exception raises in Python
The sys.exit()
can be used in except block to stop running the program when an exception is raised.
import sys
try:
x = 1/0
except Exception as e:
sys.exit()
Output
This will exit the program.
Which is not a Standard Exception in Python among NameError, IOError, ValueError, and Assignment Error
NameError, IOError, and ValueError are standard exceptions in Python whereas Assignment error is not a standard exception in Python.
How to Print Exception Name in Python
The format except Exception as
can be used to print the exception name.
try:
a = 6/0
except Exception as e:
print(e)
Output
division by zero
How to Handle Exception within an Exception in Python
The nested try and exception blocks cab be used to handle the exception within an exception.
x = 10
y = 0
try:
print("outer try block")
try:
print("nested try block")
print(x / y)
except TypeError as te:
print("nested except block")
print(te)
except ZeroDivisionError as ze:
print("outer except block")
print(ze)
Output
outer try block
nested try block
outer except block
division by zero
How to Print the Exception in Python
The print_exc()
function from the traceback library can be used in except block to print the exception.
import traceback
try:
1/0
except Exception:
traceback.print_exc()
Output
ZeroDivisionError: division by zero
How to Exit Program Execution when a Thread gives Exception in Python
The PyThreadState_SetAsyncExc()
method allows us to raise an exception and exit the program if the thread raises an exception.
import threading
import ctypes
import time
class thread_with_exception(threading.Thread):
def __init__(self, name):
threading.Thread.__init__(self)
self.name = name
def run(self):
try:
while True:
print('running ' + self.name)
finally:
print('ended')
def get_id(self):
if hasattr(self, '_thread_id'):
return self._thread_id
for id, thread in threading._active.items():
if thread is self:
return id
def raise_exception(self):
thread_id = self.get_id()
res = ctypes.pythonapi.PyThreadState_SetAsyncExc(thread_id,
ctypes.py_object(SystemExit))
if res > 1:
ctypes.pythonapi.PyThreadState_SetAsyncExc(thread_id, 0)
print('Exception raise failure')
t1 = thread_with_exception('Thread')
t1.start()
time.sleep(2)
t1.raise_exception()
t1.join()
Output
running Thread
running Thread
running Thread
running Thread
running Thread
ended
How to Catch Missing Argument Exception in Python
The TypeError is raised when there is no missing argument. The except block with TypeError is used to catch and handle the missing argument exception.
def add(a,b):
res=a+b
return res
try:
add(10)
except(TypeError):
print("Function requires 2 arguments")
Output
Function requires 2 arguments
How to Catch Integer Exception in Python?
The ValueError is used to catch the integer exceptions.
n = int(input("Please enter a number: "))
while True:
try:
n = input("Please enter an integer: ")
n = int(n)
break
except ValueError:
print("No valid integer! Please try again ...")
print("Great, you successfully entered an integer!")
Output
Please enter a number: 1
Please enter an integer: 3.4
No valid integer! Please try again ...
Please enter an integer: a
No valid integer! Please try again ...
Please enter an integer: 2
Great, you successfully entered an integer!
How to Catch a Socket Timeout Exception in Python
The socket.timeout
is raised when a timeout occurs on a socket.
import socket
s = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
s.settimeout(0.0000001)
try:
s.connect(("www.pythonwife.com", 80))
except socket.timeout:
print("Timeout raised and caught.")
Output
Timeout raised and caught.
How to Implement Illegal Argument Exception in Python
The ValueError()
can be used to raise the exception for illegal argument exception.
def left_or_right(sequence, index, right = False, left = False):
if not right and not left:
raise ValueError("Both cannot be False.")
elif right and left:
raise ValueError("Both cannot be True.")
elif right:
return sequence[index+1]
elif left:
return sequence[index-1]
case_1 = left_or_right([1, 2, 3], 1, right = False, left = False)
Output
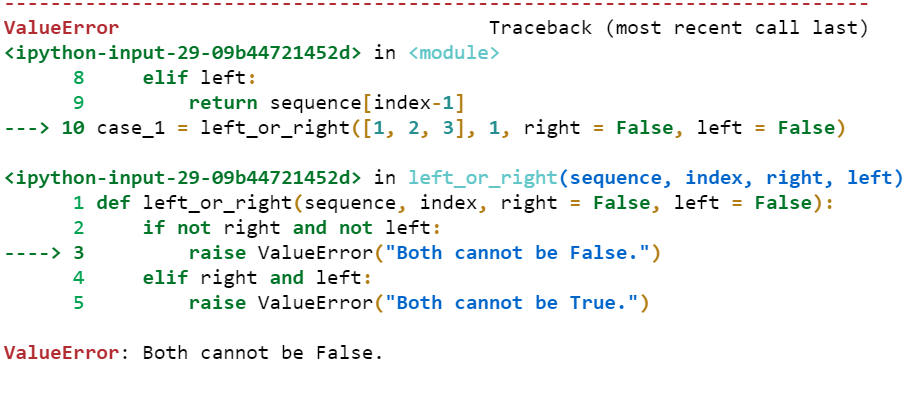
How to Capture Python Exception Value
The traceback.print_exc()
can be used to print the current exception to standard error, just like it would be printed if it remained uncaught, or traceback.format_exc()
to get the same output as a string.
import traceback
try:
1/0
except Exception:
traceback.print_exc()
Output

How to Throw Exception from a Python Function
The raise keyword can be used within the function definition to throw exceptions from a python function.
def sample():
x = -1
if x < 0:
raise Exception("Sorry, no numbers below zero")
sample()
Output

Which of the following Exceptions Occurs, when an Integer object is added to a String object in Python
The TypeError Exception arises when we try to add a string object to the integer.
print(1+"abc")
Output

How to Add Exception Handling in Python
A try statement can be used to manage exceptions. The try clause contains the critical operation that can cause an exception. The except clause contains the code that handles exceptions. As a result, once we’ve caught the exception, we may choose which operations to conduct.
import sys
randomList = ['a', 0, 2]
for entry in randomList:
try:
print("The entry is", entry)
r = 1/int(entry)
break
except:
print("Oops!", sys.exc_info()[0], "occurred.")
print("Next entry.")
print()
print("The reciprocal of", entry, "is", r)
Output
The entry is a
Oops! <class 'ValueError'> occurred.
Next entry.
The entry is 0
Oops! <class 'ZeroDivisionError'> occurred.
Next entry.
The entry is 2
The reciprocal of 2 is 0.5
How to Print Exception Error Message in Python
The except Exception as
can be used to catch an exception and print()
method in except clause can be used to print an exception error message.
try:
a = 1/0
except Exception as e:
print(e)
Output
division by zero
How to Intentionally Raise Exception in Python
The raise
keyword can be used to intentionally raise the exception.
x = int(input("Enter a number "))
if x < 0:
raise Exception("Sorry, no numbers below zero")
Output
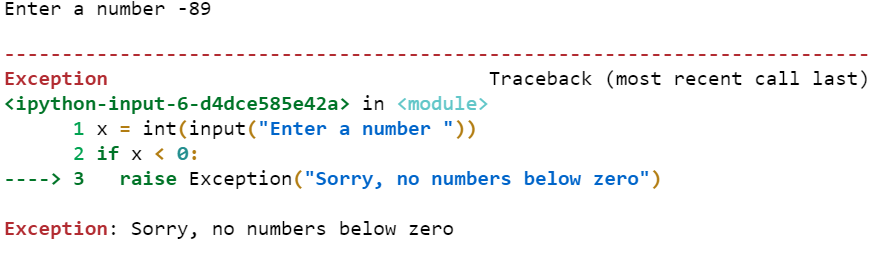
How to Print a Caught Exception in Python
Using the format except (exception name) as
we can print the name of the caught exception.
try:
l = [1, 2, 3]
l[4]
except IndexError as e:
print(e)
Output
list index out of range
What is Uncaught Exception in Python
When an exception is raised and uncaught, the interpreter calls sys.excepthook
with three arguments, the exception class, exception instance, and a traceback object.
import sys
import logging
import traceback
def log_except_hook(*exc_info):
text = "".join(traceback.format_exception(*exc_info))
logging.error("Unhandled exception: %s", text)
sys.excepthook = log_except_hook
None()
Output
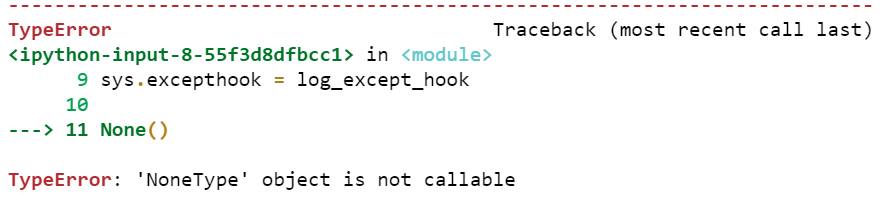
How to Show the Cause for Exception in Python
The print_exc()
function from the traceback library can be used to show the cause for the exception.
import traceback
try:
x = 5
y = 0
print(x/y)
except ZeroDivisionError:
print("Error Dividing %d/%d" % (x,y))
traceback.print_exc()
except:
print("A non-ZeroDivisionError occurred")
Output

What does the Else Block in the Exception Handling do in Python?
The code enters the else block only if the try clause does not raise an exception. That is, the Else block will execute only when no exception occurs.
def divide(x, y):
try:
result = x // y
except ZeroDivisionError:
print("You are dividing by zero ")
else:
print("Your answer is :", result)
divide(60, 6)
divide(30, 0)
Output
Your answer is : 10
You are dividing by zero
What happens to the Finally Block when there is no Exception in Python
A finally block is always get executed whether the exception has occurred or not. finally block is always executed after leaving the try statement.
try:
k = 50//5
print(k)
except ZeroDivisionError:
print("Can't divide by zero")
finally:
print('finally block is always executed')
Output
10
finally block is always executed
How to Return to Try Block after Exception in Python
The optional else clause is executed if and when control flows off the end of the try clause.
def divide(x, y):
print('entering divide')
try:
return x/y
except:
print('error')
else:
print('no error')
finally:
print('exit')
print(divide(1, 1))
print(divide(1, 0))
Output
entering divide
exit
1.0
entering divide
error
exit
None
How to get the Source of Exception in Python
The function format_exc()
from traceback library can be used to get the source of the exception.
import traceback
try:
print(4/0)
except ZeroDivisionError:
print(traceback.format_exc())
Output
Traceback (most recent call last):
File "<ipython-input-20-c49f2cfc1a2a>", line 4, in <module>
print(4/0)
ZeroDivisionError: division by zero
What is the Difference Between Raising an Exception and Handling an Exception in Python?
- Raising an Exception is used to throw or raise the exception using the
raise
keyword. - Handling an Exception is catching all the thrown exceptions in the except block.
try:
raise ValueError('ValueError')
except Exception as error:
print('Caught this error: ', error)
Output
Caught this error: ValueError
How to write a Try and Except block in Python Without throwing Exception?
Without using the raise
keyword in try block exception can still be handled, as in the below code using some in-built exceptions.
try:
k = 50//0
print(k)
except ZeroDivisionError:
print("Can't divide by zero")
Output
Can't divide by zero
How to Get Information From the Python Exception
Using the format.exc()
function from the traceback library we can get information from the exception.
import traceback
try:
print(4/0)
except ZeroDivisionError:
print(traceback.format_exc())
Output
Traceback (most recent call last):
File "<ipython-input-20-c49f2cfc1a2a>", line 4, in <module>
print(4/0)
ZeroDivisionError: division by zero
How to Raise an Exception in Python When asking a Yes or No Input from User
The if-else
statement can be used inside the try block to raise an exception for yes or no input from the user.
status =input('Are you above 18?')
try:
if(status == "Yes"):
print("Congratulations you are eligible to vote..!")
else:
raise ValueError
except(ValueError):
print("Sorry you are not eligible to vote..!")
Output
Are you above 18?Yes
Congratulations you are eligible to vote..!
Are you above 18?No
Sorry you are not eligible to vote..!
How to Catch Specific Exception in Python
A try
block can have any number of except
blocks to handle different exceptions, however, only the specific except block will be executed in case an exception occurs.
try:
x=9//0
except ValueError:
print("Value error arised")
except ZeroDivisionError:
print("divide by zero error occured")
except TypeError:
print("Type error occured")
except:
print("An error occured")
Output
divide by zero error occured
How to Print the Contents of an Exception in Python?
We can use traceback.print_exc()
to print the current exception to standard error, it would be printed even if it remained uncaught, or traceback.format_exc()
to get the same output as a string
import traceback
try:
print(4/0)
except ZeroDivisionError:
print(traceback.print_exc())
Output

What Causes an Exception in Python
Bad data, faulty network connectivity, corrupted databases, memory pressures, and unexpected user inputs can all cause an Exception. An exception is thrown when such an event occurs and when the software is unable to continue its normal flow.
How to Handle Index Out of Bound Exception in Python?
The in-built IndexError
can be used to handle index out of bound exceptions.
try:
sample=['a', 'b' ,'c']
print(sample[6])
except IndexError:
print("list index out of range")
Output
list index out of range
How to Throw an Invalid Input Exception in Python?
The user-defined exception class InvalidInputError
is defined and handled in except block.
class InvalidInputError(Exception):
pass
try:
name = input("Enter your Last Name:")
if not name.isalpha():
raise InvalidInputError
except(InvalidInputError):
print("Enter only alpha values.")
Output
Enter your Last Name:alan87
Enter only alpha values.
How to Add Message to the Name Error Exception in Python?
The attribute message
can be used to append a message to the exception.
def bar(arg1):
try:
raise IOError('Stuff')
except NameError as e:
raise type(e)(e.message + ' happens at %s' % arg1)
bar('arg1')
Output
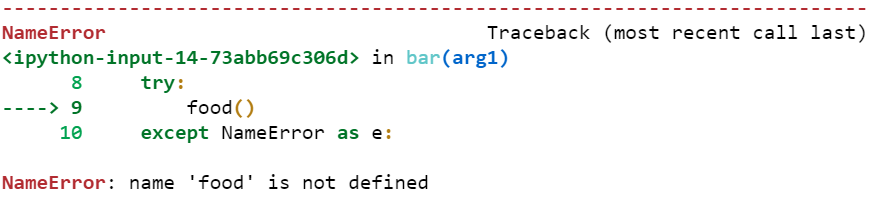
What Exception can be Thrown to check if a variable equals to a value in python
The ValueError can be used to check if the variable equals an expected value or not.
status =input('Are you above 18?')
try:
if(status == "Yes" or status == "yes"):
print("Congratulations you are eligible to vote..!")
else:
raise ValueError
except(ValueError):
print("Sorry you are not eligible to vote..!")
Output
Are you above 18?yes
Congratulations you are eligible to vote..!
What Exception is Raised when Python Attempts to Convert a String to a Number?
The ValueError exception arises when we try to convert a string to a number.
string = input("Enter a word ")
try:
stringtoint = int(string)
print(stringtoint)
except ValueError:
print('Oops!Cannot convert a word to a number')
Output
Enter a word sample
Oops!Cannot convert a word to a number
Enter a word 666
666
How to print the Line number in which exception occurred?
The exc_tb.tb_lineno
can be used to print the line number in which an exception occurred.
import sys, os
try:
raise NotImplementedError("No error")
except Exception as e:
exc_type, exc_obj, exc_tb = sys.exc_info()
print("Line number", exc_tb.tb_lineno)
Output
Line number 4
How to Log an Exception and Preserve the Stack Trace in Python?
Using the logging library the exception can be logged and stack trace can be preserved.
import logging
try:
90/0
except ZeroDivisionError:
logging.exception("message")
Output

How to Raise an Exception if Two Lists are Not of the Same Length?
The ValueError can be raised if two lists are not of the same length.
a=[1,2,3,4]
b=[2,3,6]
if len(a) != len(b):
raise ValueError("list a and list b must have the same length")
Output
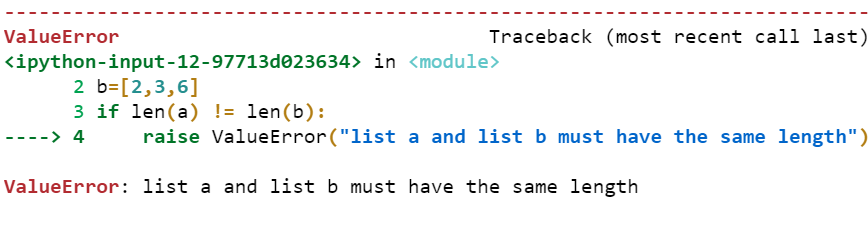
How to Create Exception with While Loops in Python?
try:
i=-3
while(i<0):
raise
i=i+1
except:
print("Negative number")
Output
Negative number
How to Assert an Exception in Python?
AssertionError is inherited from the Exception class when this exception occurs and raises AssertionError. It can be handled using the default exception handler.
try:
x = 1
y = 0
assert y != 0, "Cannot perform this Operation"
print(x / y)
except AssertionError as msg:
print(msg)
Output
Cannot perform this Operation
How to Handle Multiple Exceptions in a Single Line using Python?
We can have multiple except
blocks to handle different exceptions. But these handlers will only deal with exceptions that occurred in the corresponding try
block.
try:
x=int("sample")
y=9//0
except (ZeroDivisionError, ValueError, TypeError)as e:
print(e)
#method two
try:
x=int("sample")
y=9//0
except:
pass
Output
invalid literal for int() with base 10: 'sample'
Which Exception is Thrown When Parsing Float from String in Python?
The ValueError is thrown when parsing a string to float if that string is not the form of a number.
sample="hello"
float_value=float(sample)
print(float_value)
Output
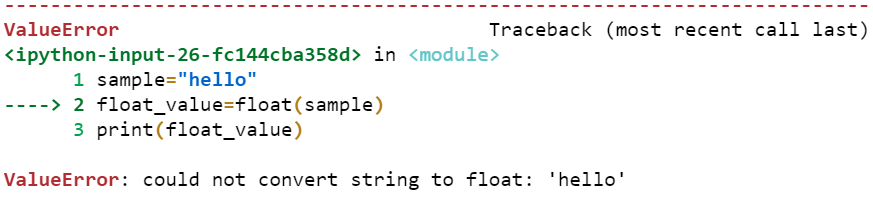
What is the Argument of an Exception in Python?
An argument to an exception is a description that provides more information about the situation. The argument’s content varies by exception.
my_string = "Python Wife"
try:
b = float(my_string / 20)
except Exception as Argument:
print( 'This is the Argument\n', Argument)
Output
This is the Argument
unsupported operand type(s) for /: 'str' and 'int'
How to Handle TypeError Exception in Python?
The TypeError is raised whenever an operation is performed on an incorrect or unsupported object type. The TypeError is handled in the except block as except(TypeError)
.
try:
word = "Pythonwife"
num = 4
print(word + num + word)
except(TypeError):
print("Cannot add a string and integer")
Output
Cannot add a string and integer
How to Handle String Index Out of Bound Exception in Python?
The except(IndexError)
can be used to handle string out of bound exception.
try:
sample="hello"
print(sample[9])
except(IndexError):
print("Couldn't access the index\nThe maximum length of the string is ",len(sample))
Output
Couldn't access the index
The maximum length of the string is 5
How to handle List Out of Range Exception when Adding Values to the List Using Loop in Python?
The IndexError that arises when iterating through the list can be handled using except(IndexError)
.
try:
list = [1, 3, 5, 7, 9]
for i in range(0,10):
print(list[i])
except(IndexError):
print("list index out of range")
Output
1
3
5
7
9
list index out of range
What would Happen When we Try to Rename a File that Doesn’t Exist in Python?
The NameError arises when we try to rename a file that doesn’t exist.
import os
os.rename(food.txt,sample.txt)
Output
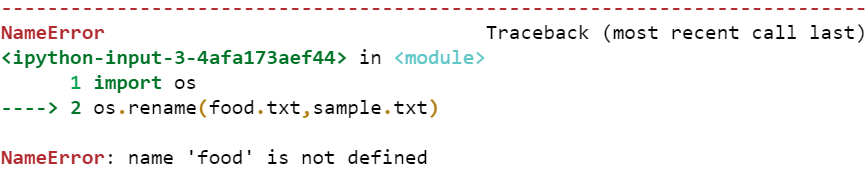
What Happens if you Try to Access the Key with no Value in Dictionary using Python?
The KeyError arises when we try to access the key with no value in the dictionary. It can be handled using get(key,default value)
. If the key is present, the value associated with the key is printed, else the default value passed in arguments is returned.
name_score = {'John' : '91',
'Alen' : '85',
'Nikhel' : '97'}
print(name_score.get('Andrew', 'Not Found'))
print(name_score.get('Nikhel', 'Not Found'))
Output
Not Found
97
How many Except Statements can a Try-Except Block have in Python?
The try block must have at least one except block and can have any number of except blocks.
try:
x=int("hello")
except ValueError:
print("ValueError occured")
except NameError:
print("NameError Occured")
except IndexError:
print("IndexError occured")
Output
ValueError occured
How to Return to Try Block after Exception in Python?
How many Try and Excepts can One have in Python?
There can be any number of try and expect blocks in python. The following code is the syntax for having multiple try and except blocks.
try:
code a
except ExplicitException:
pass
try:
code b
except ExplicitException:
try:
code c
except ExplicitException:
try:
code d
except ExplicitException:
pass
How to Break Loop in Try and Except in Python?
The break
keyword can be used to break the loop in try and except block.
try:
y=9
while(y>0):
print("breaking the loop")
break
except:
print("exception caught")
Output
breaking the loop
Can we use Try Except instead of If Else in Python?
No, We cannot use try and except block instead of if-else. As try and except is used to throw and handle the errors, while if-else acts as conditional statements.
How does Python Handle Nested Try Statements?
x = 10
y = 0
try:
print("outer try block")
try:
print("nested try block")
print(x / y)
except TypeError as te:
print("nested except block")
print(te)
except ZeroDivisionError as ze:
print("outer except block")
print(ze)
Output
outer try block
nested try block
outer except block
division by zero
How to Use For Loop in Try and Except block in Python?
try:
for i in range(1,6):
if(i>4):
raise
else:
print(i)
except:
print("An Exception Occured")
Output
1
2
3
4
An Exception Occured
How to Open Two Files with Try and Except in Python?
The method open()
can be called inside try block and in case the file is not found it can be handled using the exception block.
try:
with open("random") as f1, open("sample") as f2:
line_file1 = f1.readline()
line_file2 = f2.readline()
print(line_file1, line_file2)
except:
print("File cannot be opened")
Output
Hello world
How can we use a Try Block Without having to do Anything in the Except Block in Python?
The pass keyword can be used in the except block so that we can have the try block without having to do anything in the except block. The pass statement does nothing, so there will be no output.
try:
x=1000/0
print(x)
except:
pass
Output
What Does def and try Keyword Mean in Python?
- The def keyword is used to define a function in python.
- The try keyword is used to check for some errors in the code.
def sum(a,b):
return a+b
try:
res=sum(10,20)
print(res)
except:
print("Error in code")
Output
30
How to Use Try Else in Python?
The try block can be used to raise the error and else block will be executed if no error were raised.
def divide(x, y):
try:
result = x // y
except ZeroDivisionError:
print("Sorry ! You are dividing by zero ")
else:
print("Yeah ! Your answer is :", result)
divide(3, 2)
divide(3, 0)
Output
Yeah ! Your answer is : 1
Sorry ! You are dividing by zero
How to Loop a Try-Except block in Python?
The while loop can be used to loop through the try-except block.
while True:
try:
i = int(input('Select a number from 1 to 4: '))
if i in range(5):
break
except:
pass
print('\nIncorrect input, try again')
Output
Select a number from 1 to 4: 6
Incorrect input, try again
Select a number from 1 to 4: 3
How to use the Try and Except block to check if entered Input is a String or not in Python?
The isalpha()
method can be used within the try block to check if entered input is a string or not.
try:
sample=input("enter a string")
if(sample.isalpha()):
print("The entered string is ",sample)
else:
raise
except:
print("oops!u have entered a integer")
Output
enter a string hello
The entered string is hello
enter a string 123
oops!u have entered a integer
How to Ignore an Exception in python?
The pass keyword can be used to ignore an exception.
try:
print(x)
except Exception:
pass
print("Exception ignored")
Output
Exception ignored
How to suppress known Exceptions without using Try and Except block?
The suppress()
function from the contextlib library can be used to suppress the known exceptions without try and except block.
from contextlib import suppress
nums = [3, -1, -2, 1, 0,]
result = 0
for num in nums:
with suppress(ZeroDivisionError):
result += 1/num
result
Output
-0.16666666666666674