Python is an easy-to-learn, powerful programming language. It has efficient high-level data structures and a simple but effective approach to object-oriented programming. Python’s elegant syntax and dynamic typing, together with its interpreted nature, make it an ideal language for scripting and rapid application development in many areas on most platforms.
The Python interpreter is easily extended with new functions and data types implemented in C or C++ (or other languages callable from C). Python is also suitable as an extension language for customizable applications.
Features of Python
- Simple and Easy to Learn – The syntax of python is very simple to learn and code. The number of lines of code python takes to create an algorithm or a program is very less compared to other languages.
- Open Source Programming Language – Python is free to use and you can download and install it on your local machine. The Benefit of open source is that we can create anything using python in your machine. For example, you can create a new language that can be built on python.
- High-Level Programming Language – Python is a human-understandable language and is written in human language.
- Platform Independent and Portability – Python provides flexibility to run the same program on multiple platforms, known as WORA ( write once run anywhere ). Once the program is created we dont have to change the program to run it on Windows, Linux, macOS, etc.,
- Dynamically Typed Language – While creating a variable we dont have to declare the data type of the variable. Since Python is a dynamically typed programming language, we dont have to explicitly declare variable type.
# variable data type need not be mentioned
a = 5
print(a)
>>> 5
- Object-Oriented and Procedure Oriented – Python is both a procedure-oriented and object-oriented language. Everything in python is an object to make it re-usable and it uses the loops concept to implement iterations and Procedure oriented programming.
- Interpreted Language – Python is an interpreted language unlike java or C we dont have to compile it before running the program. The python interpreter runs in the backend and throws errors if there are any in the syntax
- Extensible Programming Language – Python provides the ability to run a program that is coded in a different language other than python. Python provides Native Support.
- Embedded Support – Similar to using other languages in python, we can use python in other languages too known as embedded support.
- Huge library Support – There are many pre-built libraries in python that make the number of lines of code to be very minimal when compared to other languages. This makes developing machine learning and data science models quickly and focussing only on performance tuning rather than reducing the lines of code.
- Speed of Python – Compared with Other Languages such as C or Java, the time is taken for execution of python code is more. Even the speed of python might be slow, it provides the speed of developing a code in less amount of time compared to other languages, this helps reduce the development cost. Python is slow because code is interpreted at runtime instead of being compiled to native code at compile time. To overcome this situation we can use the embedded features to run the code in other languages.
Applications of Python
- Desktop Application – We can create simple desktop applications that do not require the internet such as a calculator.
- Web Application – Python provides frameworks for developing both simple and complex web apps. For building a simple web app we can use Flask, and for building complex enterprise applications we can use the Django framework
- Database-related applications – For processing the data from a database and perform CRUD operations on a database python provides a connector for this purpose
- Networking – For accessing a remote machine or server using python, there are different libraries that can serve this purpose such as socket programming, Netmiko can be used
- Games – Python provides support for developing games. The most used libraries for this purpose are kivy, pygame.
- Data Analytics/ Data Science – There are numerous data handling libraries in python to clean and represent data. Libraries such as NumPy, pandas, Scipy can be used in data science
- Machine Learning – Python can be used for building artificial intelligence and machine learning models.
- IoT applications – For handling and building IoT applications python provides a library known as Raspberrypi
Installing Python
# variable data type need not be mentioned
a = 5
print(a)
>>> 5
- Desktop Application – We can create simple desktop applications that do not require the internet such as a calculator.
- Web Application – Python provides frameworks for developing both simple and complex web apps. For building a simple web app we can use Flask, and for building complex enterprise applications we can use the Django framework
- Database-related applications – For processing the data from a database and perform CRUD operations on a database python provides a connector for this purpose
- Networking – For accessing a remote machine or server using python, there are different libraries that can serve this purpose such as socket programming, Netmiko can be used
- Games – Python provides support for developing games. The most used libraries for this purpose are kivy, pygame.
- Data Analytics/ Data Science – There are numerous data handling libraries in python to clean and represent data. Libraries such as NumPy, pandas, Scipy can be used in data science
- Machine Learning – Python can be used for building artificial intelligence and machine learning models.
- IoT applications – For handling and building IoT applications python provides a library known as Raspberrypi
Installing Python
Python does not come as pre-packaged with the operating system. So the users might have to download an appropriate version and install it. Since the initial release of Python in 1991, it has been constantly been updating its versions and fixing the bugs.
For installation purposes, you can choose python – 3.6 to python – 3.8 in order to get the latest features. In this article, we will be learning how to install python, and other IDE’s such as Pycharm, Visual Studio code, Spyder.
Download python
To download the python you can navigate to https://www.python.org/downloads/ and choose the operating system, version, and 32-bit or 64-bit installer based on the configuration you are having.
Python Installation
After you run the installer you can select ADD python to path option this enable python to be accessed from the default directory when we open the command prompt and then proceed to click Customize installation.
While customization you can unselect the documentation, and click next to proceed
After proceeding to the next window select “Install for all users”, and click install.
Once done with the installation you can check whether Python is installed properly or not by checking the python version using the command python --version
Download and Install Pycharm
PyCharm is an integrated development environment used in computer programming, specifically for the Python language. PyCharm provides smart code completion, code inspections, on-the-fly error highlighting, and quick fixes, along with automated code refactorings and rich navigation capabilities.
To download the Pycharm use the link provided PyCharm and choose the operating system of your choice ( Windows, Linux, or macOS ). The community version of pycharm downloads the free version of pycharm. If you require extra features from pycharm then you can go for a professional version.
Once downloaded go to file location and double click to proceed for installation. Click next to proceed.
Choose a folder for installing the application and its files, and then click Next. Pycharm required around 1.0 GB of disk space on your local machine.
Click the checkboxes of your requirements basically, you can select the create shortcut option and Update context before proceeding further.
After clicking next it will take few moments to install the pycharm. After successful installation, you can open pycharm using the desktop shortcut we have created in the previous step.
Running a sample script in pycharm.
To run the program click the run option that you can see on the menu bar. If the runtime has not been configured you can name the run time and proceed to execute the script.
hi python
Process finished with exit code 0
Download and install VS Code
Visual Studio Code is a source-code editor made by Microsoft for Windows, Linux, and macOS. Features include support for debugging, syntax highlighting, intelligent code completion, snippets, code refactoring, and embedded Git.
Download VS Code from https://code.visualstudio.com/download.
After downloading you can open the downloaded folder and double click to run the vscode.exe file to install. And click accept to proceed to the further installation process.
Choose the destination folder for installing the visual studio files. Make sure there is disk space of 350 MB available to install.
You can customize the installation by selecting VScode to add to the path or not. Also, it’s better if you add the desktop icon shortcut for opening the IDE.
You can summarize the requirements during the installation in the next phase and continue to install. Once the installation is completed you can open it by double-clicking the desktop icon.
Since the visual studio code can run multiple language programs but there is a requirement for extension that can support the execution of the programs. We require a python executor and can search for it in the extensions search box that is available on the bottom left of the sidebar.
Run a sample .py file in VS Code
Since we have installed a .py extension in VS Code, when we open any file with .py extension will be executed in the python environment. To run the python script click the run button on the top right of the menu bar.
Install Spyder using Anaconda
Anaconda is a distribution of Python and R programming languages that aims to simplify package management, deployment and is focused on data-driven projects. Anaconda provides scientific computing for data science, machine learning, predictive analysis, and long-scale data processing.
You can download the anaconda over the Anaconda link which serves the purpose of individuals and is completely free to download and use.
After you run the installer you can NEXT option and click, I Agree.
You need to choose the directory and folder for anaconda installation with a disk space of 2.9GB approximately.
Choose whether or not to add Anaconda to your path, it’s not recommended since it may interfere with the other software of your system so instead open the anaconda prompt from the start menu.
After a successful installation, you will see the “Thanks for Installing Anaconda” dialog box.
Now anaconda is installed it provides several IDE’s to launch through a single application. Once we open the Anaconda File from the start menu select the Anaconda navigator.
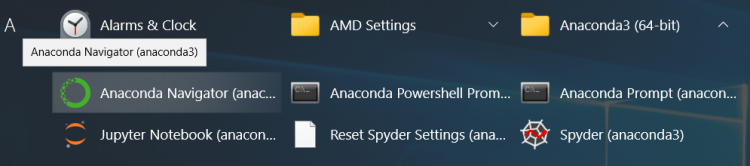
Once we click the icon it will open Anaconda navigator as shown in the below figure, as we can see the spyder shell we can click launch to open Spyder IDE.
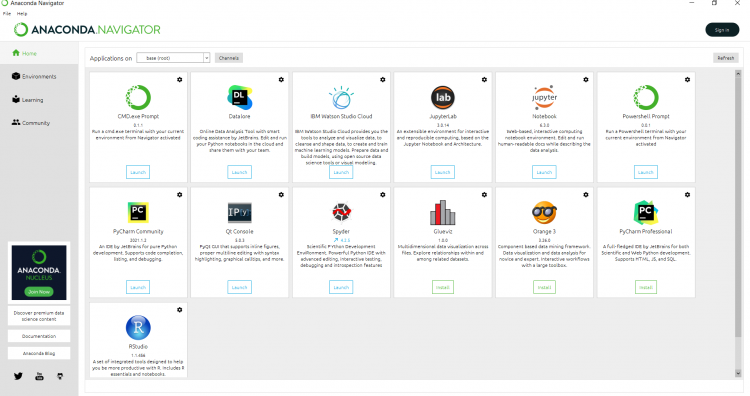
Running a basic Hello script on Spyder
To run the script we can click the run button on the menu bar to execute the program and returns the output as shown in the below figure.
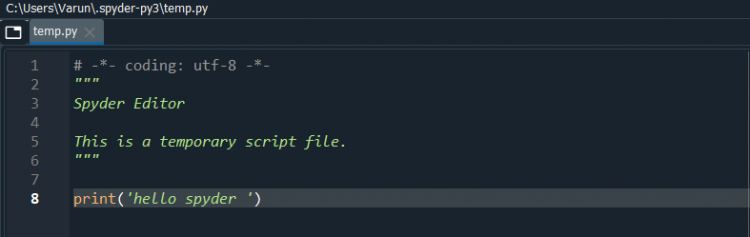
