In this tutorial we will be learning how to perform basic operations such as read/write on images & videos
Reading an Image
import cv2
.- We need to pass the path of the image location to the
cv2.imread()
function and then store it in a variable. The cv2.imread() function returns image as a matrix of pixels. - Using
cv2.imshow()
we can display the image. cv2.imshow()
takes two parameters.- The name of the frame we use to display the image
- Variable that has the image file.
import cv2
img = cv2.imread('C:\images\personmask.jpg')
cv2.imshow("image", img)
cv2.waitKey(0)
output:
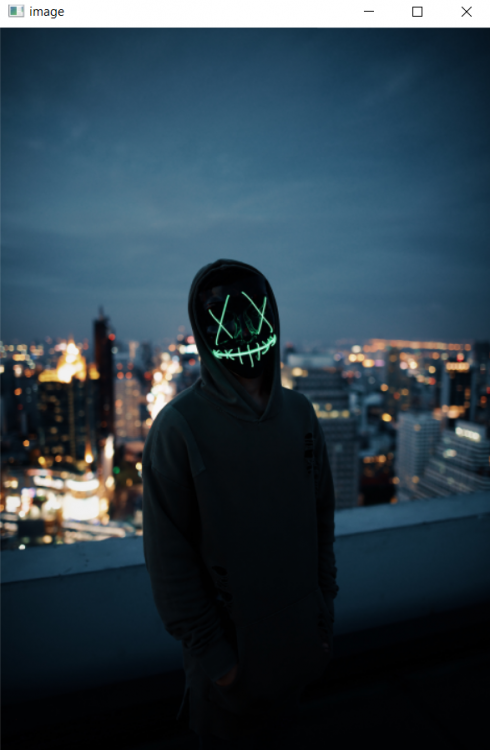
Write or store an Image
We can store or write an image using cv2.imwrite('folder', image)
cv2.write()
takes two parameters the destination folder to save the image and the image object that we have opened using cv2.imread()
import cv2
img = cv2.imread('C:\images\personmask.jpg')
cv2.imwrite('C:\images\dupimage.jpg', img)
Reading a Video file
Video is a combination of images passing at a frequency known as fps(frames per second).
- We can use
cv2.VideoCapture
() for using any camera source and retrieve video or can directly provide a path to the video source and store it in a variable. - In this example, we are storing the captured video in the “capture” variable
- Using
capture.read()
, we can capture the video.read()
function returns whether any frame is present as boolean value and the frame. In the below code we are storing the boolean value isTrue and we store the frame object in the frame. - Once the Frame is capture, we can display it by
cv.imshow()
. - We can quit the window by assigning the keyboard button click value equal to ‘q’.
0xFF==ord('q')
- Release the memory used for video capture using
capture.release()
and destroy all the windows using cv2.destroyAllWindows().
import cv2
#to open a default camera of the device we need to pass '0'
capture = cv2.VideoCapture(0)
while True:
isTrue, frame = capture.read()
cv.imshow('video', frame)
if cv2.waitKey(15) & 0xFF==ord('q'):
break
capture.release()
cv2.destroyAllWindows()
Writing a Video file using cv2.VideoWriter()
Firstly cv2.VideoWriter takes 4 parameters
- File name
- cv2.VideoWriter_fourcc()
- We pass the codec we would like to compress our video file
- There are many codecs available such as XVID, MPEG, HEVC etc.,
- But make sure your video format can open the compressed video
- In our example we will be using XVID and open it in mp4 format
- Frames per second
- Video Resolution (in our example we use ‘640×480’)
Once we create an object for cv2.VideoWriter we follow certain steps to avoid blank video’s or camera failures
- We set a while loop with the condition set to
capture.isopened()
- This determines Camera is opened
- Before Capturing the frames we check whether frames are being returned or not
if ret==True:
- Thus by setting the conditions as the Camera is opened and the frames are received we can write the frames using the object of cv2.VideoCapture.
Go through the following code snippet it has the steps we have discussed.
import cv2
capture = cv2.VideoCapture(0)
fourcc = cv.VideoWriter_fourcc(*'XVID')
cap = cv2.VideoWriter('C:\videos\person.mp4', fourcc, 10.0, (640,480))
while capture.isOpened():
isTrue, frame = capture.read()
if ret==True:
cap.write(frame)
cv2.imshow('video', frame)
if cv2.waitKey(15) & 0xFF==ord('q'):
break
capture.release()
cv2.destroyAllWindows()
Read video from web-cam
To read the live feed from the webcam, we need to pass ‘0’ to cv2.VideoCapture(), opens the default camera of the device which is a web-cam.
import cv2
capture = cv2.VideoCapture(0)
while True:
isTrue, frame = capture.read()
cv.imshow('video', frame)
if cv2.waitKey(15) & 0xFF==ord('q'):
break
capture.release()
cv2.destroyAllWindows()
Read video from url
You can read video from a url we need to pass the url into cv2.VideoCapture()
Since we are passing a short video url once the video ends and no frame is retrieved in the statement if ret==True:
, then else block gets executed and breaks the loop.
import cv2
capture = cv2.VideoCapture('https://chercher.tech/files/video-from-website.mkv')
while capture.isOpened():
ret, frame = capture.read()
if ret==True:
cv2.imshow('video', frame)
if cv2.waitKey(15) & 0xFF==ord('q'):
break
else:
break
capture.release()
cv2.destroyAllWindows()