Resizing Images & Videos
Resizing Images
Generally, we perform resizing or rescaling on images to reduce the computational load on a computer while analyzing the visual content. When we work on huge data sets resizing is quite often practice we do to reduce the computation time and increase the performance of the model.
Reducing the image size will reduce the number of pixels available in the image and decreases the dimensions of the image. We can perform operations such as changing the height and width by changing the dimensions of the image.
In the below example, we redefine the dimensions of the image by changing the width, height and then using cv2.resize()
to apply new dimensions to the frame.
cv2.resize() takes 3 parameters
- Image
- Dimensions
- Height & Width
- Interpolation
- There are different types of Interpolation, for resampling the pixels of the image we apply cv2.INTER_AREA
import cv2
img = cv2.imread('C:\images\personmask.jpg')
def reduce_framesize(frame, scale=0.50):
width = int(frame.shape[1]*scale)
height = int(frame.shape[0]*scale)
dimensions = ( width, height)
return cv2.resize(frame, dimensions, interpolation = cv2.INTER_AREA)
cv.imshow("profile", reduce_framesize(img))
cv.waitKey(1)
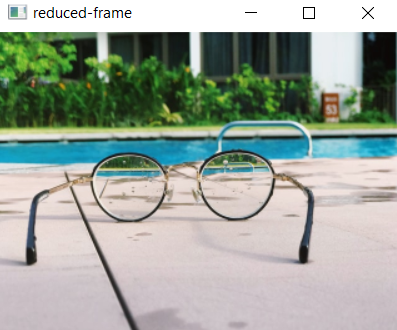
Resizing Videos
For resizing the video, the Procedure remains the same as resizing the image. We apply new dimensions to the frame and then apply it over the video file using cv2.resize().
import cv2
def reduce_framesize(frame, scale = 0.50):
width = int(frame.shape[1]*scale)
height = int(frame.shape[0]*scale)
dimensions = (width, height)
return cv2.resize(frame, dimensions, interpolation = cv2.INTER_AREA)
capture = cv2.VideoCapture(0)
while capture.isOpened():
ret, frame = capture.read()
resized_frame = reduce_framesize(frame)
if ret==True:
cv2.imshow('vide',resized_frame)
if cv2.waitKey(10) & 0xFF==ord('q'):
break
capture.release()
cv2.destroyAllWindows()
Drawing on Images using OpenCV
Drawings are necessary while handling object detection. OpenCV provides pre-defined methods to draw different shapes on images.
We can automate the process of object detection by comparing the frames in a video and the change in pixel colors thus drawing an outline on each frame where there is a significant change in pixel color.
Drawing Rectangle
Using cv2.rectangle()
it’s a piece of cake to draw a rectangle of required dimensions at particular coordinates on an Image.
cv2.rectangle()
takes the following parameters
- Image
- starting coordinates of the rectangle
- ending coordinates of the rectangle
- color of the line in BGR
- the thickness of the line
import cv2
image = cv2.imread('C:\images\chairs.jpg')
cv2.rectangle(image, (180,250), (470,500), (0,0,255), thickness=2)
cv2.imshow('frame', image)
cv2.waitKey(0)
cv2.destroyAllWindows()
Output:
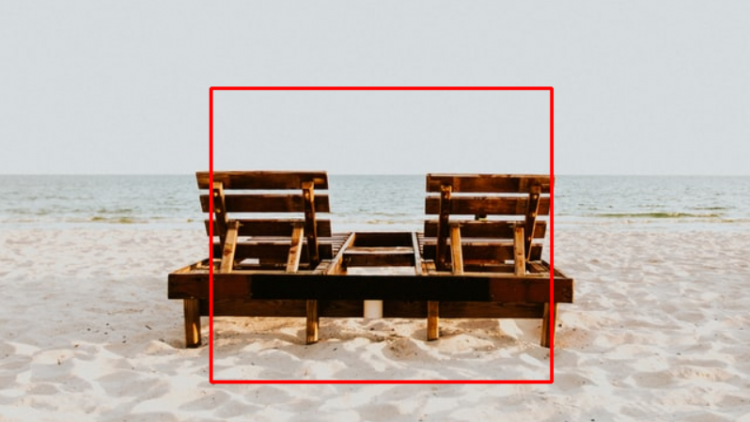
Drawing Circle
Using cv2.circle()
we can draw a circle of specific radius and color. The following are the parameters we should pass
- Image
- Coordinates of the center of the circle
- the radius of the circle
- color scheme
- thickness
import cv2
image = cv2.imread('C:\\images\chairs.jpg')
cv2.circle(image, (320,400), 90, (0,0,255), thickness = 2)
cv2.imshow('frame', image)
cv2.waitKey(0)
cv2.destroyAllWindows()
Output:
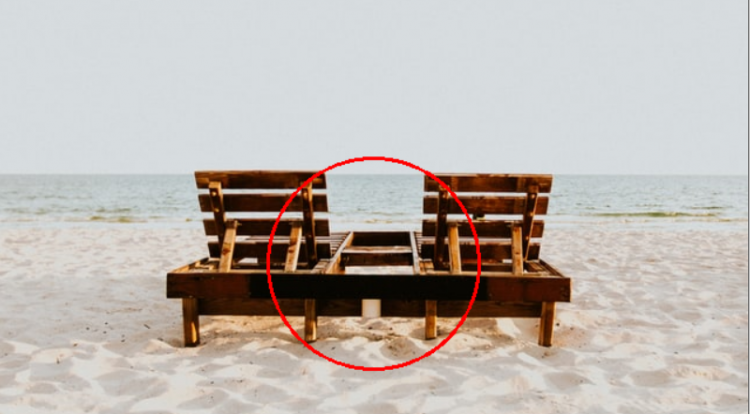
Drawing Ellipse
cv2.ellipse()
takes several parameters :-
- Center of the ellipse (x, y)
- Axis Length (major and minor axis)
- Angle (angle of horizontal axis of ellipse with the actual axis)
- Start angle (the start angle of the ellipse with respect to horizontal axis of ellipse)
- End angle(the end angle of the ellipse with respect to horizontal axis of ellipse)
- color
- thickness
import cv2
image = cv2.imread('C:\images\chairs.jpg')
cv2.ellipse(image, (300, 300), (150,100), 0, 0, 360, (255, 255, 255), 4)
cv2.imshow('frame', image)
cv2.waitKey(0)
cv2.destroyAllWindows()
Output:
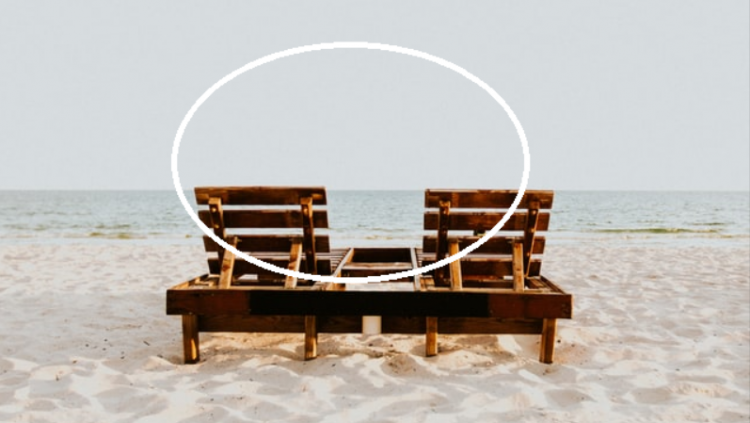
Adding Text to Images
using cv.putText()
we can add text with added font and color
parameters are Frame, Text, coordinates, font, font scale, color, thickness, inline-type
import cv2
image = cv2.imread('C:\images\chairs.jpg')
font = cv2.FONT_HERSHEY_SIMPLEX
cv2.putText(image, 'Hii',(3, 500), font, 4, (0, 0, 0), 2, cv2.LINE_AA)
cv2.imshow('frame', image)
Output :-
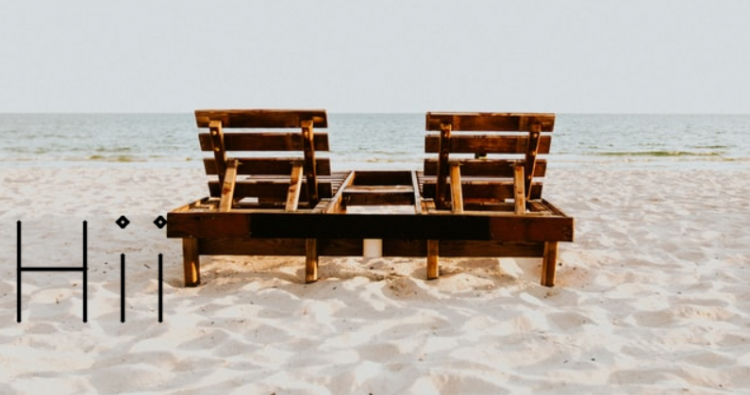
Drawing a line:-
We can draw a line between two points on any image using cv2.line()
method.
Parameters passed are image, start coordinates, end coordinates, color of the line, thickness of the line.
import cv2
image = cv2.imread('C:\images\desk.jpg')
cv2.line(image, (100, 150), (500,550), (0, 0, 255), 3)
cv2.imshow('line', image)
Output:-
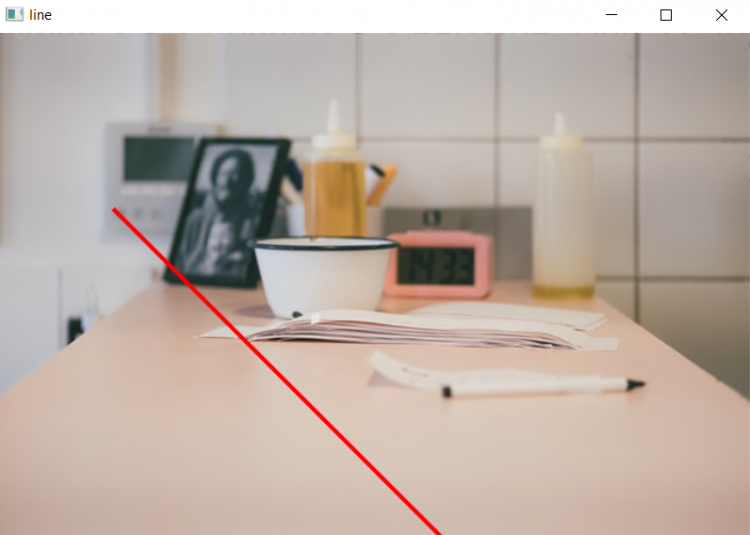