Image thresholding is a concept of changing the colors of pixels and manipulating the pixels in an image into only two colors, mostly black and white. This concept is mostly used to display the objects of the image clearly.
With the use of Image thresholding, we can compare the pixel color with min and max values of thresholding, and based on the threshold type we can apply the thresholding effect.
Different threshold types are
- BINARY THRESHOLDING ( THRESH_BINARY )
- INVERSE BINARY THRESHOLDING ( THRESH_BINARY_INV )
- TRUNCATE THRESHOLDING ( THRESH_TRUNC )
- THRESHOLD to ZERO ( THRESH_TOZERO )
- INVERTED THRESHOLD to ZERO ( THRESH_TOZERO_INV )
BINARY THRESHOLDING
Binary threshold looks for pixels with intensity less than the thresh1 we pass and, converts them to black color by manipulating their color code to zero. Pixels with a color value more than thresh1 are converted into thresh2.
Thus it is easy to distinguish the shapes or hidden features of the image.
import cv2
image = cv2.imread('C:\images\people.jpg')
imgray = cv2.cvtColor(image, cv2.COLOR_BGR2GRAY)
#cv2.threshold(src, thresh1, thresh2, threshold_type)
ret, thresh = cv2.threshold(imgray, 127, 255, cv2.THRESH_BINARY)
cv2.imshow('original', image)
cv2.imshow('binary_thresh', thresh)
Output:- As we can see cv2.THRESH_BINARY performs binary operations and converts the pixel colors according to threshold values
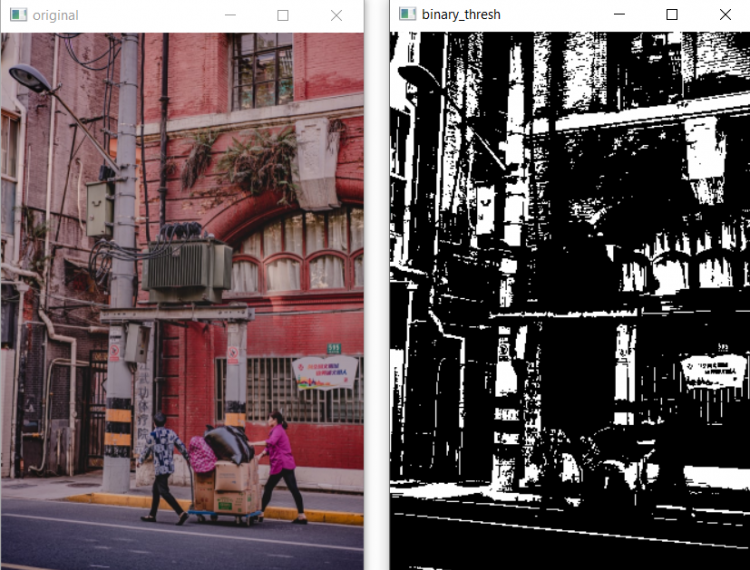
INVERSE BINARY THRESHOLD
The inverse binary thresholding concept is the same as the binary threshold but the manipulation of pixel colors is exactly the opposite. As we pass the threshold values( thresh1, thresh2 ) cv2.THRESH_BINARY_INV
looks for pixels that are above the thresh1 and applies them ‘0’ intensity and pixels below thresh1 are applied to color thresh2.
In the example below pixels with intensity less than thresh1( 127 ) are converted into thresh2( 255 ), and pixels with intensity more than thresh1 are converted to zero intensity.
import cv2
image = cv2.imread('C:\images\people.jpg')
imgray = cv2.cvtColor(image, cv2.COLOR_BGR2GRAY)
#cv2.threshold(src, thresh1, thresh2, threshold_type)
ret, thresh = cv2.threshold(imgray, 127, 255, cv2.THRESH_BINARY_INV)
cv2.imshow('original', image)
cv2.imshow('Inv_thresh', thresh)
Output:-
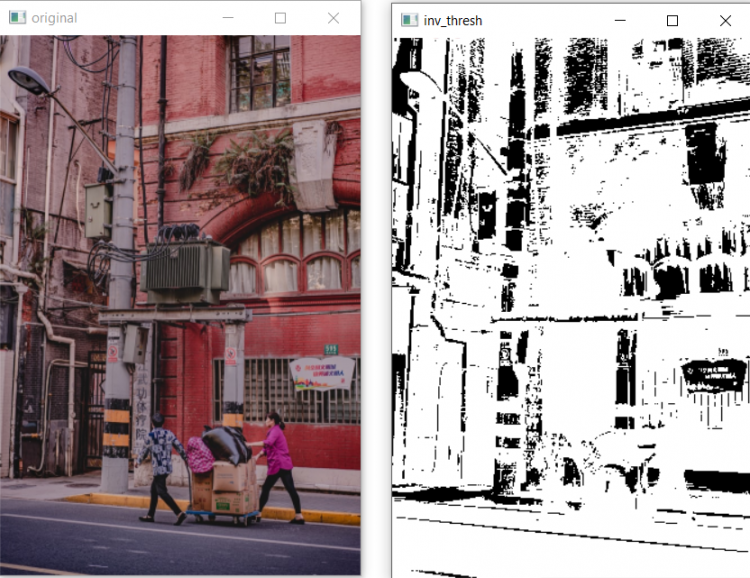
TRUNCATE THRESHOLDING
Truncate thresholding (cv2.THRESH_TRUNC
) is a technique for detecting the pixel intensities that are greater than thresh1 and apply them to thresh1 and the pixels that are less than thresh1 remain unchanged, the Thresh2 value is ignored.
#cv2.threshold(src, thresh1, thresh2, threshold_type)
ret, thresh = cv2.threshold(imgray, 127, 255, cv2.THRESH_TRUNC)
cv2.imshow('original', image)
cv2.imshow('thresh_trunc', thresh)
Output:-
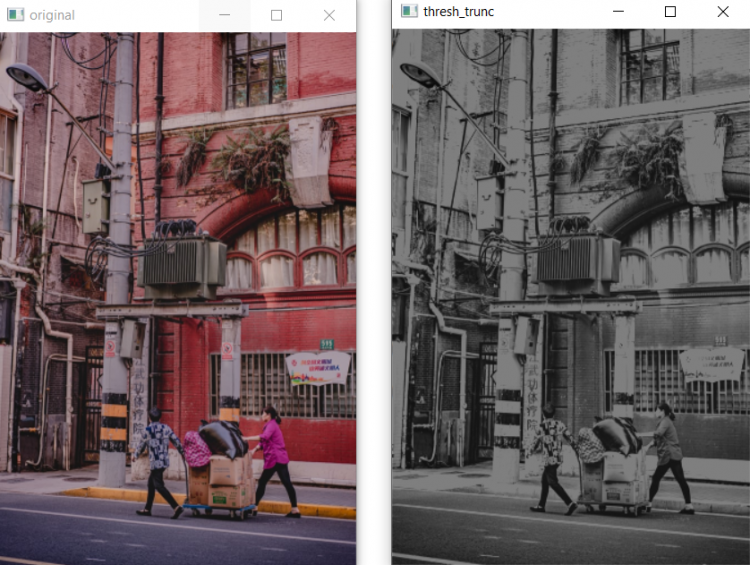
THRESHOLD to ZERO
When you apply cv2.THRESH_TOZERO
this does not affect the pixels that are having intensity more than thresh1, but the pixels that have intensity less than thresh1 are rounded to zero. thresh2 does not affect anything.
To notice the changes in the images we can pass a colored image instead of passing a gray image.
#cv2.threshold(src, thresh1, thresh2, threshold_type)
ret, thresh = cv2.threshold(image, 120, 255, cv2.THRESH_TOZERO)
cv2.imshow('original', image)
cv2.imshow('thresh', thresh)
Output:- In the output, the pixels less than 120 are applied zero intensity, and the pixels greater than 120 remain unchanged.
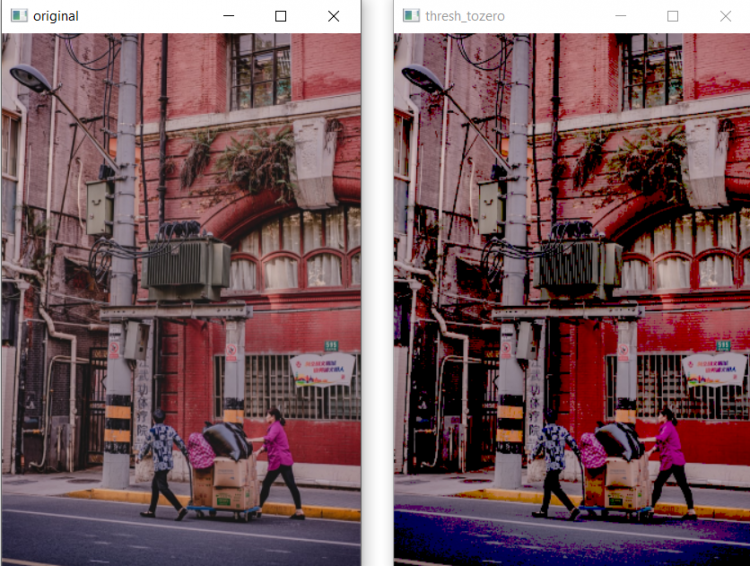
INVERTED THRESHOLD to ZERO
cv2.THRESH_TOZERO_INV
is exactly opposite to cv2.THRESH_TOZERO
and convert pixels with intensities more than thresh1 to zero and the ones that are less than thresh1 remain unchanged, ( thresh2 value is ignored ).
#cv2.threshold(src, thresh1, thresh2, threshold_type)
ret, thresh = cv2.threshold(image, 120, 255, cv2.THRESH_TOZERO_INV)
cv2.imshow('original', image)
cv2.imshow('thresh', thresh)
Output:-
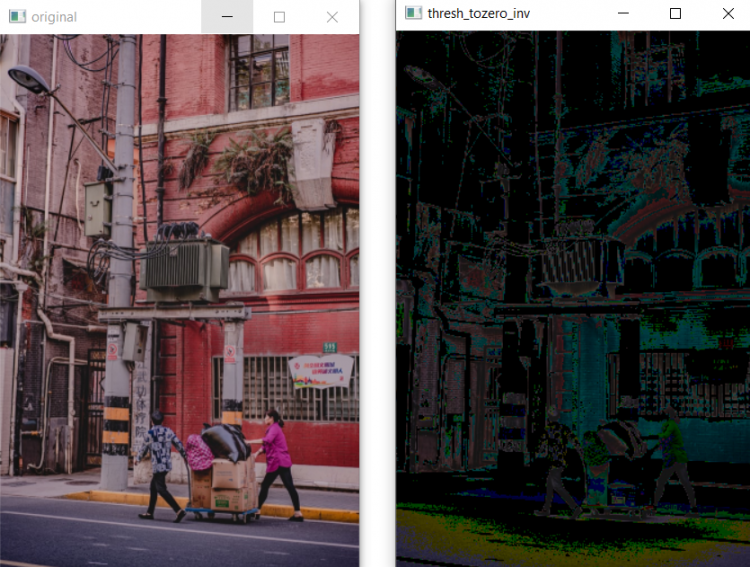