Histograms are the graphical representation of the pixel intensities distribution in the form of a digital image. Histograms provide an easy understanding of the features of the pixels in an image such as contrast, brightness, intensity distribution, etc.,
- X-axis represents the range of values a variable can take, and is divided into several series of intervals knows as bins
- Y-axis represents number of pixels that have particular intensity
- We can draw histograms both for colored images and gray images
cv2.calcHist()
can be used to draw the histogram and takes parameters such as source image, color channels, number of bins, range of the x.
- Image sent in the form a list
- Index of color channels in the image
- mask ( to represent the full image pass none )
- Histogram size ( represents number of bins provided as a list )
- Range ( represents range of intensity values along x-axis )
import cv2
# cv2.Hist( [image], [channel], mask, [bins], [Range] )
hst = cv2.calcHist([img], [2], None, [256], [0, 256])
Using matplotlib we can represent the retrieved histograms in the form of a digital image.
#for plotting the histogram
plt.plot(hist)
#labelling the x-axis
plt.xlabel(' x label ')
#labelling the y-axis
plt.ylabel(' number of pixels ')
#represents x-liimits of the current axis#for plotting the histogram
plt.xlim([0, 256])
#used for giving title to the histogram graph
plt.title(' Histograms ')
Histogram of gray image
import cv2
import matplotlib.pyplot as plt
import numpy as np
#opening image in gray scale
image = cv2.imread('C:\images\person.jpg', 0)
# In number of channels we pass 0 since its a gray image
hst = cv2.calcHist([image], [0], None, [256], [0, 256])
plt.figure()
plt.title("histogram representation")
plt.xlabel("bins")
plt.ylabel("number of pixels")
plt.plot(hst)
plt.xlim([0, 256])
plt.show()
Output:-
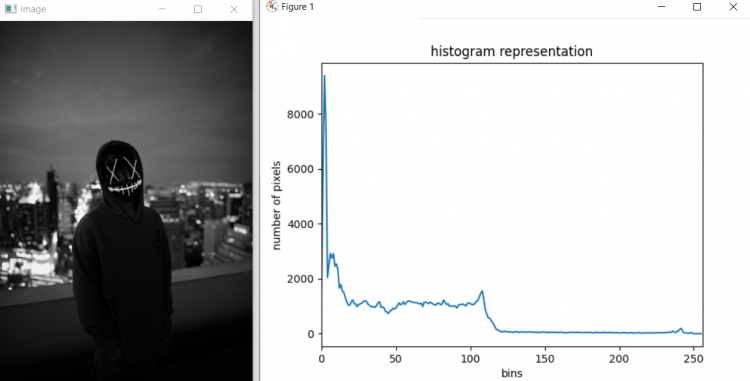
Histogram of colored image
Drawing histograms for a colored image follows the same process but cv2.calcHist()
takes the color channels ( B, G, R ) separately and plots them. For this process, we can create a for loop and loop over the blur, green, red channels as a sequence.
We create a sequence using enumerate in python since it provides a counter for the iterator.
for i, col in enumerate(['b', 'g', 'r']):
#here index of the channel is the iterator 'i'
hst = cv2.calcHist([img], [i], None, [256], [0, 256])
plt.plot(hst, color = col)
plt.xlim([0, 256])
import cv2
import matplotlib.pyplot as plt
image = cv2.imread('C:\images\person.jpg')
plt.figure()
for i, col in enumerate(['b', 'g', 'r']):
hst = cv2.calcHist([img], [i], None, [256], [0, 256])
plt.plot(hst, color = col)
plt.xlim([0, 256])
plt.title("histogram of a colored image")
plt.xlabel("bins")
plt.ylabel("number of pixels")
cv2.imshow('image', img)
plt.show()
Output:
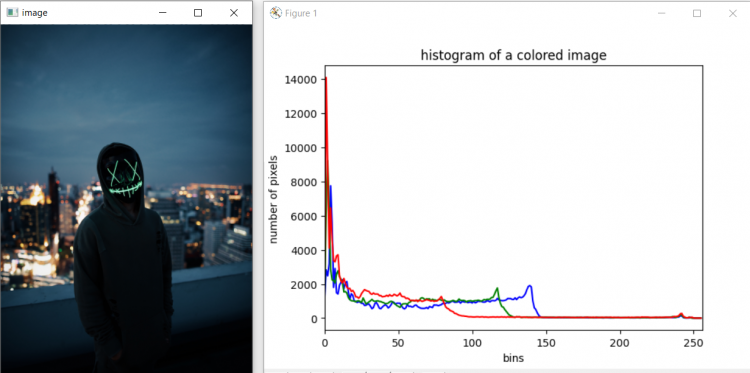