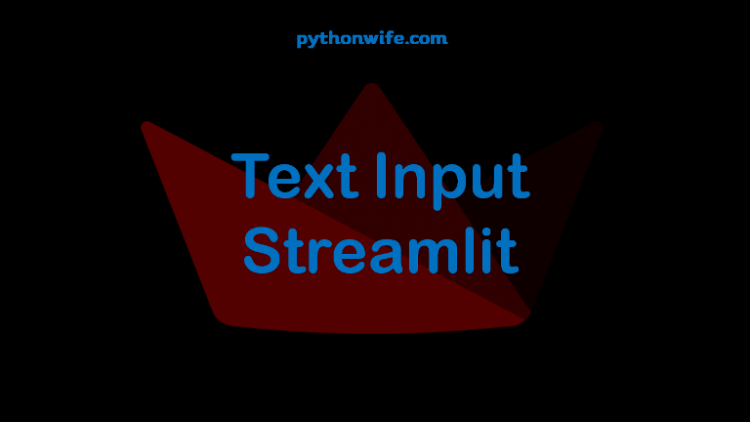
When creating a data app it’s desirable the app is dynamic in nature and the results are shown as per the user demands. For this, we need to take input from the user which can be in the form of text, number, date or even a colour.
There are various ways you can input text in your Data/ML app using streamlit. These include a text box, text area, number input, date and time input, colour picker.
Text Box
You can generate a simple text box using st.text_input()
import streamlit as st
your_name = st.text_input("Enter your name")
st.title(your_name)
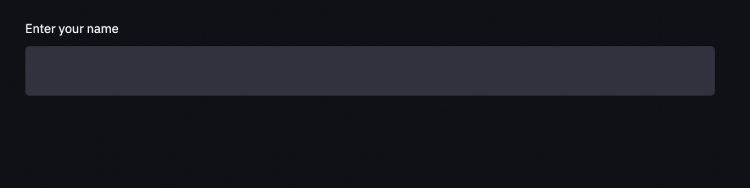
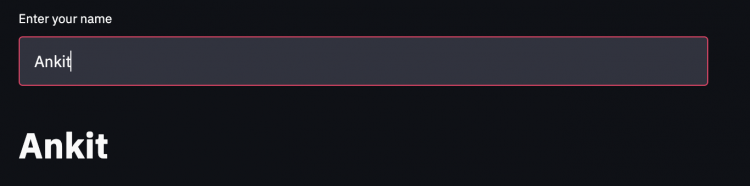
If you want to set a limit to the number of characters that the text box takes you can use max_chars
property
your_name = st.text_input("Enter your name", max_chars=10)
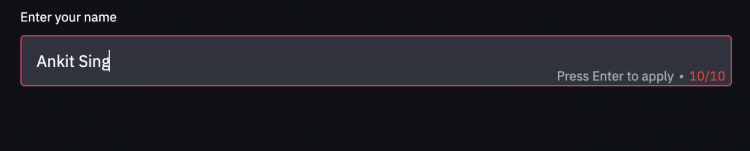
You can also set a property type = 'password'
so that the characters are hidden as it is usually in the password input.
password = st.text_input("Enter Password", type='password')
st.title(password)

Text Area
You can set a text area as well using st.text_area()
and control its height as well using the property height
message = st.text_area("Message", height=100)
st.title(message)
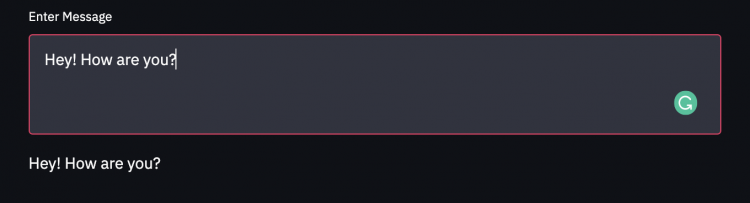
Number Input
A number input can box can be created using number_input()
function.
st.number_input("label", start, end, default_value, step_size)
- label – dispayed to the user over the input field
- start, end – minimum, maximum values that cab set
- default_value – this value is set as default value
- step_size – when you click + the new value will be value+step_size
In the example below, 0 and 25 are the minimum and maximum values of the range; 6 is the default value and 2 is the step size
number = st.number_input("Enter number", 0, 25, 6, 2)
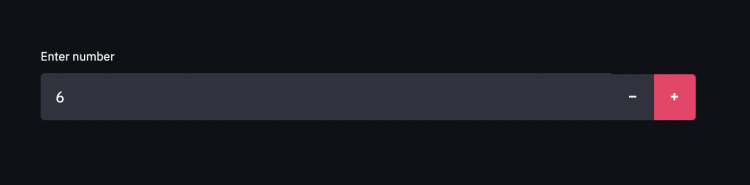
Date Input
You can create a date input using date_input()
By default, the date is set to today’s date and you can view all the dates in the calendar 10 years before and after the present date.
#Date Input
my_date = st.date_input("Select date")
st.write(my_date)
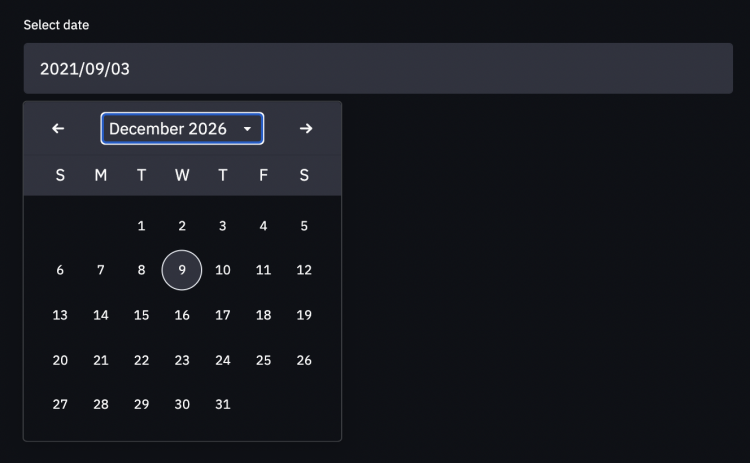
You can also specify a range of dates along with the default date.
value
, min_value
, max_value
each takes a tuple defining a date using the datetime
library as shown below. value
specifies the fault date while min_value
and max_value
define the range
import datetime
#Date Input
my_date = st.date_input("Select date", value = datetime.date(1995, 6, 15),
min_value = datetime.date(1990, 1, 1),
max_value = datetime.date(2000, 12, 31))
st.write(my_date)
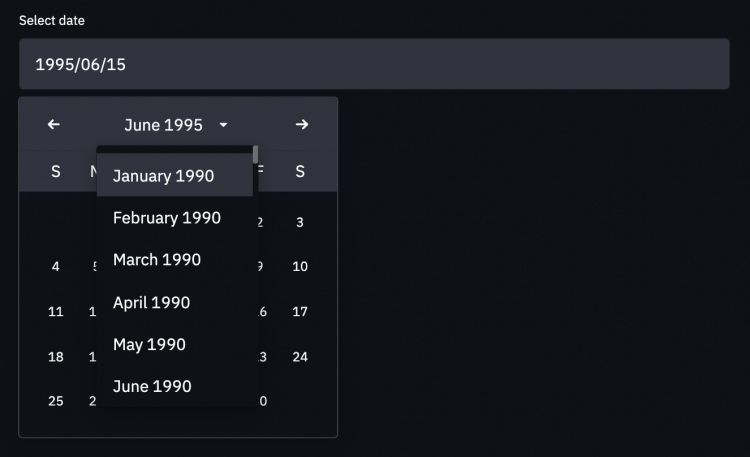
Time Input
You can use function time_input
to create a time input box
my_time = st.time_input("Select time")
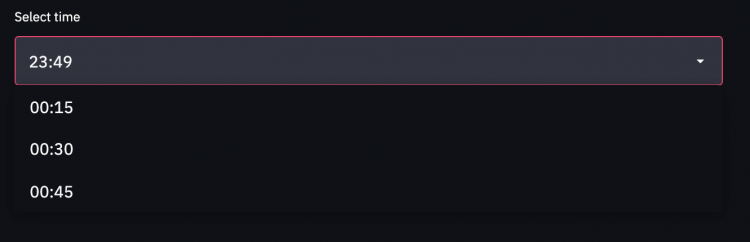
Colour Selector
You can select a colour of your choice for input as well using st.color_picker()
color = st.color_picker("Select Colour")
st.title(color)
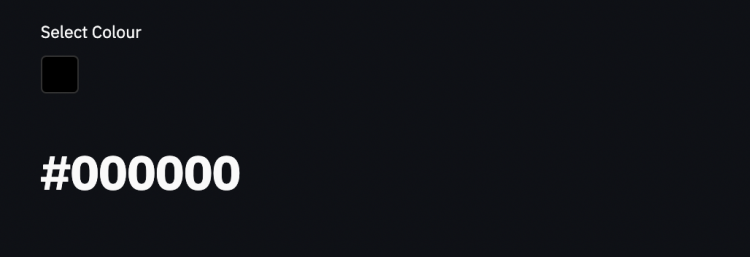
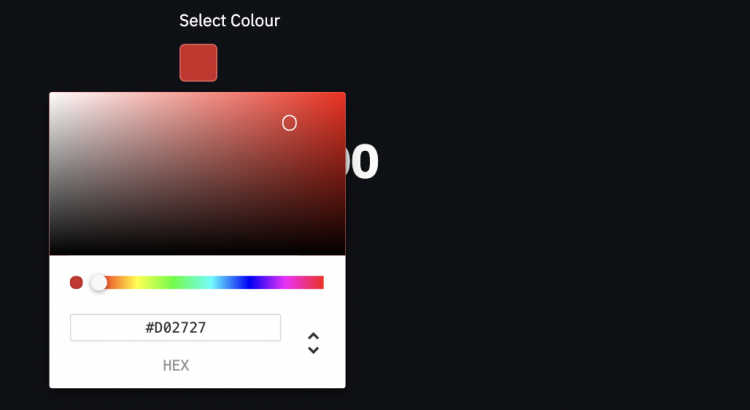
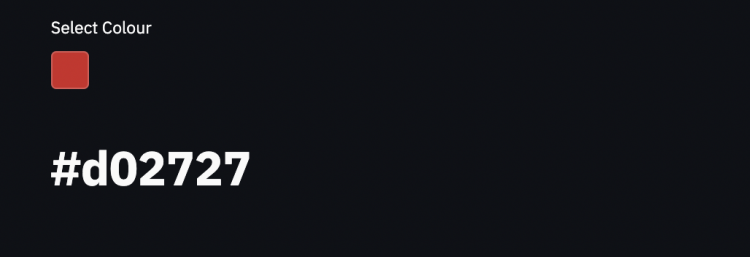