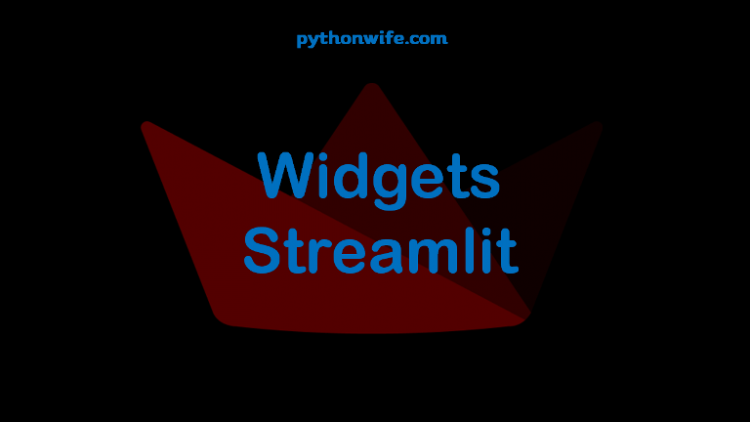
With widgets, you can make your project more appealing to the audience. Streamlit offers a range of widgets that you can include in your project like buttons, checkboxes, sliders, expanders, and selection boxes.
It is desirable to have such widgets in your ML app to make it more interactive and help view relevant information only.
Buttons
You can create a button using streamlit.button()
import streamlit as st
#Working with buttons
st.button("Submit")
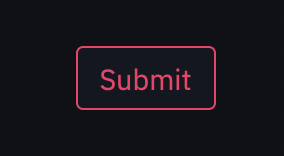
To show you a simple demonstration of how you can work with buttons, we take a variable called name ( John in the example ).
Now on clicking the Submit button it turns to uppercase. Similarly, on pressing the second submit button it turns to lowercase.
However, we need to specify a property key
to differentiate between the buttons having the same name. The key
property is useful in a workflow where you might be asking the user to click buttons with the same name more than once.
name = "John"
st.write(name)
if st.button ("Submit"):
st.write ("Name: {}".format(name.upper()))
if st.button ("Submit", key='new2'):
st.write ("Name: {}".format(name.lower()))
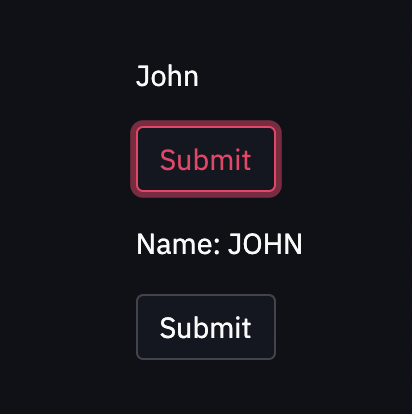
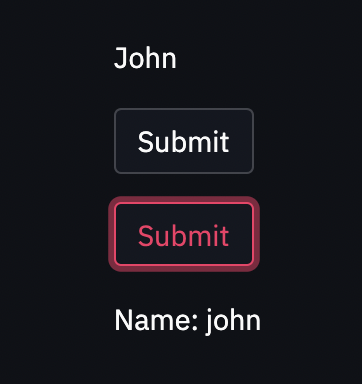
Radio Buttons
Radio buttons help select an option out of many to perform the desired function or give an output.
#Working with Radio Buttons
status = st.radio ("What is your status?", ("Active","Inactive"))
if status == 'Active':
st.success ("You are active")
elif status == 'Inactive':
st.warning ("You are inactive")
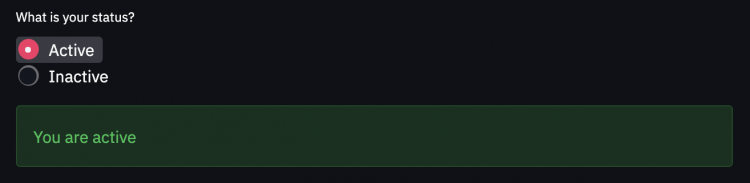
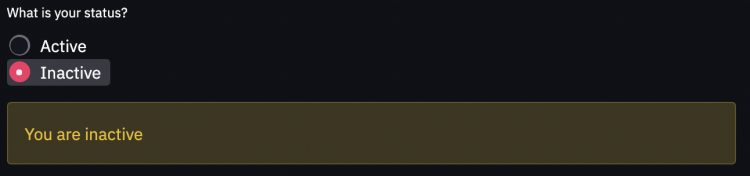
Checkboxes
Checkboxes are used to include or exclude certain aspects of our workflow.
#Working with checkbox
if st.checkbox ("Show/Hide"):
st.text("Show something")


Expanders / Accordions
Expanders can be used to hide optional information that you might not always want to show to the user or overpopulate the screen with information.
Note: Expander can be created write
keyword.
#Working with Expander
with st.expander("Python"):
st.write("Hello python")


Dropdowns
Single Value Dropdown
You can create a dropdown box with st.selectbox()
along with a pre-defined list of options for the user to select. By default, the first element defined in the list or the tuple is selected.
# Select
flowers = ["Rose", "Lotus", "Lily", "Tulip"]
choice = st.selectbox("Flower", flowers)
st.write("You selected {}".format(choice))

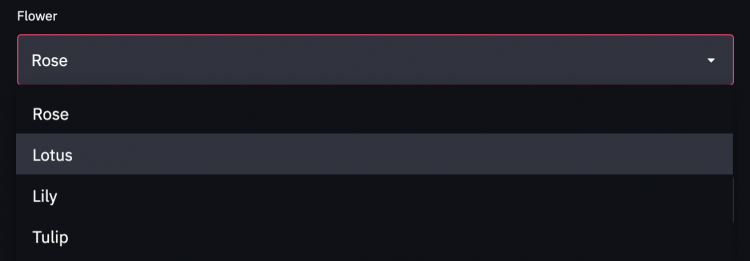

Multiple Value Dropdown
You can also create an option for the user to select multiple choices with st.multiselect()
. The default property selects already selects a choice by default.
# Select Multiple
languages = ("English", "Spanish", "Hindi", "French")
my_spoken_language = st.multiselect("Languages Spoken",languages, default="English")

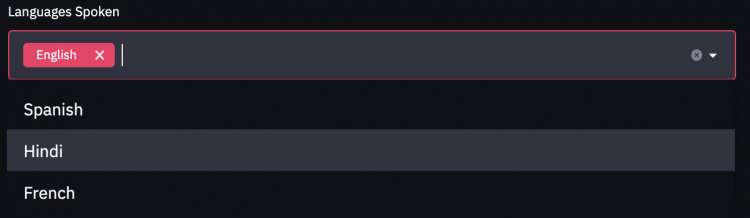

Sliders
Sliders provide an efficient and cleaner way to select an option compared to dropdown boxes or selection boxes if we have many choices. You can create a slider using st.slider()
.
When using integer or float values, by default, the difference between subsequent numbers is 1. You can change the difference accordingly by passing the 3rd parameter.
We can use a slider with integer or float values like below.
# Slider
yob = st.slider("Year Of Birth", 1940,2020)
st.write("You selected {}".format(yob))
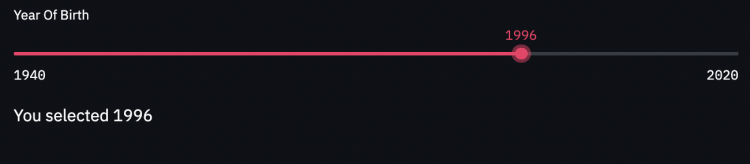
You may also create a slider with predefined options using st.select_slider()
color = st.select_slider("Choose color", options = ["yellow","red","green","black","white"])
