Question-1: Import NumPy, check NumPy version and configurations:
Solution:
import numpy as np
print(np.__version__)
np.show_config()
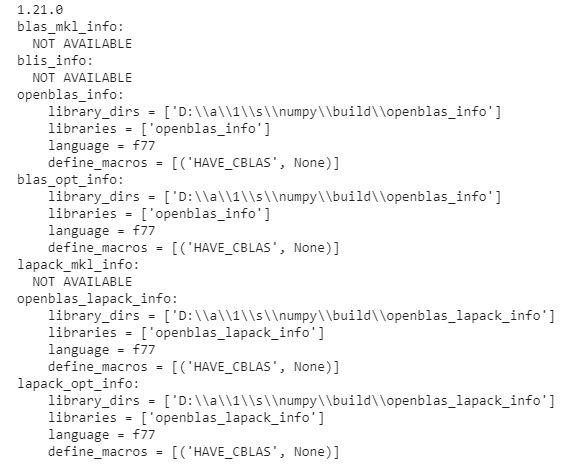
Question-2: Create a 1-D array of size 5, type float, and find the memory size(in bytes) of the array:
Solution:
array1d = np.arange(5, dtype = float)
print(f"{array1d.size * array1d.itemsize} bytes")
#Output:
40 bytes
Question-3: Print documentation of NumPy abs() function:
Solution:
print(np.abs.__doc__)
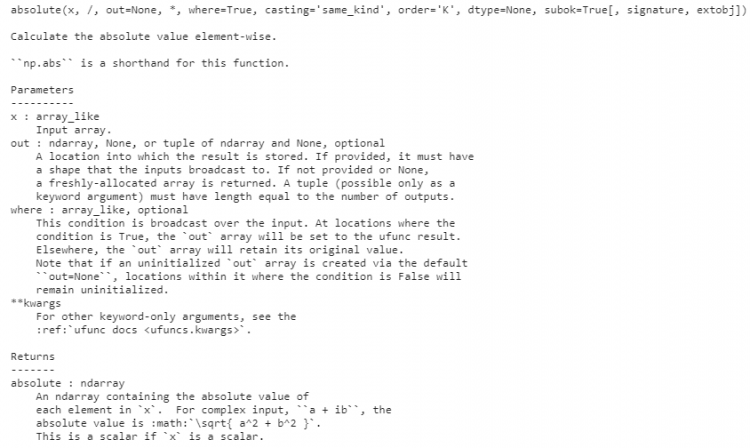
Question-4: Replace all even numbers in the array with NaN values:
Given:
#Input:
input_array = np.arange(1, 10, dtype = float)
#Expected Output:
[ 1. nan 3. nan 5. nan 7. nan 9.]
Solution:
input_array[input_array % 2 == 0] = np.nan
print(input_array)
#Output:
[ 1. nan 3. nan 5. nan 7. nan 9.]
Question-5: Create a 4 x 4 chessboard from a 2-d array filled with 0’s and 1’s:
#Input:
input_array = np.array([[0, 1], [1, 0]])
#Expected Output:
[[0 1 0 1]
[1 0 1 0]
[0 1 0 1]
[1 0 1 0]]
Solution:
print(np.tile(input_array, (2, 2)))
#Output:
[[0 1 0 1]
[1 0 1 0]
[0 1 0 1]
[1 0 1 0]]
Question-6: Generate a custom sequence pattern in NumPy without hardcoding:
#Input:
input_array = np.array([1, 2, 1])
#Expected Output:
[1 1 2 2 1 1 1 2 1 1 2 1]
Solution:
print(np.hstack((np.repeat(input_array, 2), np.tile(input_array, 2))))
#Output:
[1 1 2 2 1 1 1 2 1 1 2 1]
Question-7: Get the indices where elements of two arrays are the same:
#Input:
input_array1 = np.array([1, 2, 7, 2, 10, 4])
input_array2 = np.array([1, 2, 6, 5, 10, 3])
#Expected Output:
(array([0, 1, 4],)
Solution:
print(np.where(input_array1 == input_array2))
#Output:
(array([0, 1, 4], dtype=int64),)
Question-8: Reverse the values of the rows of a 2-D array:
#Input:
input_array = np.arange(2, 25, 2).reshape(4, 3)
#Expected Output:
[[ 6 4 2]
[12 10 8]
[18 16 14]
[24 22 20]]
Solution:
print(input_array[: , ::-1])
#Output:
[[ 6 4 2]
[12 10 8]
[18 16 14]
[24 22 20]]
Question-9: Find the maximum of two arrays using a scalar function get_max:
def get_max(a, b):
if (a > b):
return a
else:
return b
#Input:
input_array1 = np.array([2, 3, 4, 67, 15, 62, 46, 82])
input_array2 = np.array([0, 5, 12, 25, 36, 23, 39, 90])
arr_max(input_array1, input_array2)
#Expected Output:
array([ 2, 5, 12, 67, 36, 62, 46, 90])
Solution:
arr_max = np.vectorize(get_max)
arr_max(input_array1, input_array2)
#Output:
array([ 2, 5, 12, 67, 36, 62, 46, 90])
Question-10: Reverse an integer taken from input() function:
#Input:
Enter integer: -937
#Expected Output:
-739
Solution:
x = int(input("Enter integer:"))
if(x == 0):
print(0)
elif(x > 0):
x_array = np.array(list(str(x)))
print(int("".join(x_array[::-1])))
else:
x_array = np.array(list(str(abs(x))))
print(int("-" + "".join(x_array[::-1])))
#Output:
Enter integer: -937
-739
Question-11: Find the set exclusive-or of two arrays. Set exclusive-or operation will return the sorted, unique values that are in only one (not both) of the input arrays.
Given:
#Input:
input_arr1 = np.array([0, 12, 34, 53, 67])
input_arr2 = np.array([2, 12, 53, 45, 89])
#Expected Output:
[ 0 2 34 45 67 89]
Solution:
import numpy as np
input_arr1 = np.array([0, 12, 34, 53, 67])
input_arr2 = np.array([2, 12, 53, 45, 89])
print(np.setxor1d(input_arr1, input_arr2))
#Output:
[ 0 2 34 45 67 89]
Question-12: Calculate average values of two given NumPy arrays.
Given:
#Input:
input_arr1 = np.arange(4).reshape(2, 2)
input_arr2 = np.array(4, 8).reshape(2, 2)
#Expected Output:
[[2. 3.]
[4. 5.]]
Solution:
average_arr = (input_arr1 + input_arr2)/2
print(average_arr)
#Output:
[[2. 3.]
[4. 5.]]
Question-13: Calculate the sum of all columns of a 2D NumPy array.
Given:
#Input:
input_arr = np.arange(2, 10, 2).reshape(2, 2)
#Expected Output:
[ 8 12]
Solution:
print(input_arr.sum(axis = 0))
#Output:
[ 8 12]
Question-14: Extract first and last elements of the first and last rows from a given (4×4) array.
Given:
#Input:
input_arr = np.arange(1, 32, 2).reshape(4, 4)
#Expected Output:
[[ 1 7]
[25 31]]
Solution:
print(input_arr[::3, ::3])
#Output:
[[ 1 7]
[25 31]]
Question-15: Drop all missing values(nan) from a NumPy array.
Given:
#Input:
input_arr = np.array([np.nan, 11, 31, np.nan, 53, 67, 43, np.nan])
#Expected Output:
[11. 31. 53. 67. 43.]
Solution:
print(input_arr[~ np.isnan(input_arr)])
#Output:
[11. 31. 53. 67. 43.]
Question-16: Compute the row-wise counts of all possible values in an array
Given:
#Input:
input_arr = np.array([[1, 3, 4, 1],[1, 2, 3, 2],[0, 3, 3, 0],[4, 2, 3, 2]])
#Expected Output:
1 2 3 4
[[2 0 1 1]
[1 2 1 0]
[2 0 2 0]
[0 2 1 1]]
Solution:
count_arr = [np.unique(row, return_counts=True) for row in input_arr]
res = []
for a, b in count_arr:
for i in np.unique(input_arr):
if (i in a):
res.append(int(b[a==i]))
else:
res.append(0)
print(" ",1, 2, 3, 4)
print(np.array(res).reshape(4, 4))
#Output:
1 2 3 4
[[2 0 1 1]
[1 2 1 0]
[2 0 2 0]
[0 2 1 1]]
Question-17: Find the positions of the top 5 maximum values in a given array.
Given:
#Input:
input_arr = np.array([[11, 30, 24, 15],[19, 42, 35, 29],[70, 38, 33, 50],[49, 28, 63, 82]])
#Expected Output:
[49 50 63 70 82]
Solution:
print(np.sort(input_arr.flat)[-5:])
#Output:
[49 50 63 70 82]
Question-18: Sort a given array by the middle column.
Given:
#Input:
input_arr = np.array([[1, 3, 4, 1, 2],[9, 4, 3, 7, 9],[0, 8, 6, 3, 5]])
#Expected Output:
[[9 4 3 7 9]
[1 3 4 1 2]
[0 8 6 3 5]]
Solution:
print(input_arr[input_arr[:,2].argsort()])
#Output:
[[9 4 3 7 9]
[1 3 4 1 2]
[0 8 6 3 5]]
Question-19: Change the sign of a first array to that of the given second array, element-wise.
Given:
#Input:
input_arr1 = np.arange(-20, 10, 5).reshape(2, 3)
input_arr2 = np.arange(-10, 10, 3.5).reshape(2, 3)
#Expected Output:
[[-20. -15. -10.]
[ 5. 0. 5.]]
Solution:
print(np.copysign(input_arr1, input_arr2))
#Output:
[[-20. -15. -10.]
[ 5. 0. 5.]]
Question-20: Create the following pattern for an integer input.
Given:
#Input:
5
#Expected Output:
--------e--------
------e-d-e------
----e-d-c-d-e----
--e-d-c-b-c-d-e--
e-d-c-b-a-b-c-d-e
--e-d-c-b-c-d-e--
----e-d-c-d-e----
------e-d-e------
--------e--------
Solution:
n = int(input())
padding = (n-1)*4 + 1
char_arr = []
for i in range(n, 0, -1):
char_arr.append(chr(i+96))
n_arr = np.array(char_arr)
pstr = "-".join(n_arr)+"-"+"-".join(n_arr[-2::-1])
print(pstr.center(padding,'-'))
for i in range(n, 1, -1):
n_arr = np.delete(n_arr, -1)
pstr = "-".join(n_arr)+"-"+"-".join(n_arr[-2::-1])
print(pstr.center(padding,'-'))
#Output:
5
--------e--------
------e-d-e------
----e-d-c-d-e----
--e-d-c-b-c-d-e--
e-d-c-b-a-b-c-d-e
--e-d-c-b-c-d-e--
----e-d-c-d-e----
------e-d-e------
--------e--------