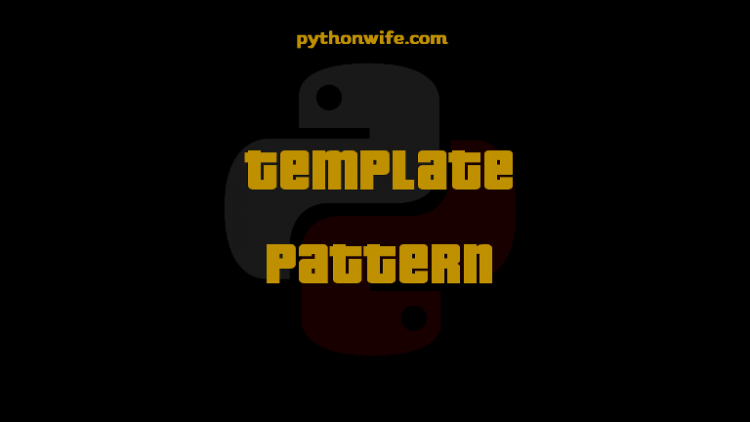
The template method design pattern allows us to define a base class that serves as the “Skeleton” of how a certain algorithm could be performed, with concrete implementation defined in child classes.
- Skeleton: Set of steps involved in an alogrithm.
Template Design Pattern is useful in situations where part(some steps) of the algorithm could have multiple implementations.
Template Design Pattern is quite similar to Strategy Design Pattern, in Strategy Pattern selection of complete algorithm is done at a run-time whereas in Template Design Pattern part of the algorithm is selected in run-time.
Members of Template Pattern
- Abstract class: Class consisting of template method and primitive methods–methods that subclasses will implement.
- Template method: Method of Abstract class defining the skeleton of an algorithm.
A Template Method defines an algorithm in a base class using abstract operations that sub-classes override to provide concrete(i.e detailed) behaviour and calls primitive operations defined in subclasses as well as operations defined in AbstractClass or those of other objects.
class AbstractClass():
def template_method(self):
self._primitive_operation_1()
self._primitive_operation_2()
@abstractmethod
def _primitive_operation_1(self):
pass
@abstractmethod
def _primitive_operation_2(self):
pass
- Concrete subclass: Class that implements the primitive methods.
class ConcreteClass(AbstractClass):
""" Implement the primitive operations """
def _primitive_operation_1(self):
pass
def _primitive_operation_2(self):
pass
Example
Here is an example of the Template Design Pattern.
- The
Meal
is an abstract class with a template method calleddoMeal()
that defines the steps involved in preparing a meal. - The
eat()
method is implemented in base class itself although subclasses can override the implementation.
"""Abstract Class"""
class Meal():
# template method
def do_meal(self):
self.get_ingredients()
self.cook()
self.eat()
self.clean_up()
def eat(self):
print(f"Mmm, {type(self).__name__} is good.")
@abstractmethod
def get_ingredients(self):
pass
@abstractmethod
def cook(self):
pass
@abstractmethod
def clean_up(self):
pass
- The
prepareIngredients()
,cook()
, andcleanUp()
methods are abstract method, implemented in seperate sub-classes.
class Pizza(Meal):
def get_ingredients(self):
print("Getting pizza base, pizza sauce, and vegetables.")
def cook(self):
print("Baking pizza in an oven.")
def clean_up(self):
print("Throwing away paper plates.")
class Tea(Meal):
def get_ingredients(self):
print("Getting tea leaves, milk and water.")
def cook(self):
print("Boliing water, putting tea leaves, adding milk.")
def clean_up(self):
print("Doing the dishes.")
- The driver code
if __name__ == '__main__':
meal1 = Pizza()
meal1.do_meal()
print("-----"*5)
meal2 = Tea()
meal2.do_meal()
print("-----"*5)
Output
Getting pizza base, pizza sauce, and vegetables.
Baking pizza in an oven.
Mmm, Pizza is good.
Throwing away paper plates.
-------------------------
Getting tea leaves, milk and water.
Boliing water, putting tea leaves, adding milk.
Mmm, Tea is good.
Doing the dishes.
The template method is an effective way to distribute tasks between classes, redefine processes, and reduce code.
Applying Template Design Pattern to an algorithm or solution can help in avoiding redundant methods and streamlining the longer execution processes.