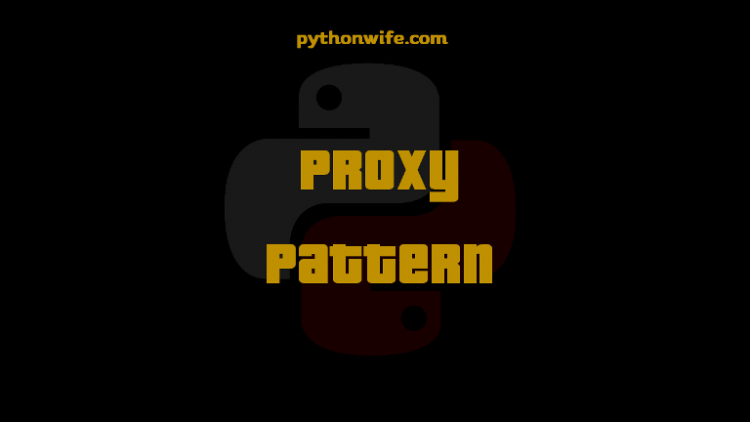
Proxy Pattern provides a placeholder for an object in order to control the access of that object. Proxy Pattern is classified into structural patterns category.
In Proxy Pattern, we create an object (proxy) for the original object (real) to interface its functionality to the outer world. The Proxy object will be the instance of a Proxy Class.
The proxy class will be functioning as an interface to a particular resource, such as a network connection, a large object in memory, a file, or some other resource that is expensive or impossible to duplicate.
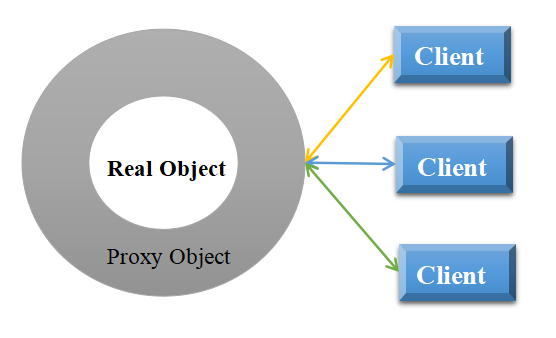
A Proxy interface object is a wrapper object that is being called by the client to access the real serving object. For the client, usage of a proxy object is similar to using the real object, because both implement the same interface.
Real-life analogy:
A real-world example can be a credit card. A credit card is a proxy for what is in our bank account. It can be used in place of cash and provides a means of accessing the cash.
Elements of Proxy Pattern:
- Subject: Interface class that defines operations and tasks
- Real Subject: Concrete class that do real operations and tasks
- Proxy Subject: Proxy class that performs the real operations and tasks on behalf of a Real Subject.
Example:
Let’s say there is a hospital that has an application for managing the information of the patients.
class PatientDataManager:
""" Real Subject"""
def __init__(self):
self.__patients = {}
def _add_patient(self, patient_id, data):
self.__patients[patient_id] = data
def _get_patient(self, patient_id):
Problem, Date = self.__patients[patient_id]
return f"Name: {patient_id}, Problem: {Problem}, Date: {Date}"
Considering the privacy of the patient, the hospital prohibits spreading the information further than necessary for the hospital to provide its services.
Here, the hospital can introduce a Proxy Class(AcessPatientData
) that controls which users can or cannot interact with certain features of the PatientDataManager
. The AccessPatientData
is a proxy class and the user communicates with the underlying class through it.
class AccessPatientData(PatientDataManager):
""" Proxy Subject"""
def __init__(self, fm):
self.fm = fm
def add_patient(self, patient_id, data, password):
if password == '1234':
self.fm._add_patient(patient_id, data)
else:
print("Wrong password.")
def get_patient(self, patient_id, password):
if password == '1234':
return self.fm._get_patient(patient_id)
else:
print("Unauthorized Access.")
We’ve got a couple of checks in Proxy Subject. If the password provided is right, the AccessPatientData
instance can add or retrieve patient info, else – it can’t.
Now, let’s instantiate an AccessPatientData
and add a patient:
obj = AccessPatientData(PatientDataManager())
obj.add_patient('Vinayak', ['pneumonia', '2021-11-09'], '1234')
print(obj.get_patient('Vinayak', '1234'))
Output:
Name: Vinayak, Problem: pneumonia, Date: 2021-11-09
It’s important to note here that Python doesn’t have true private variables – the underscores are just an indication to other programmers not to alter existing member variables or methods.
Types of Proxy:
- Virtual Proxy: Virtual Proxy is mostly used with Databases. For example if any heavy resource is consuming data from the database and we need that resource frequently. Here, we can use the proxy pattern which would create multiple proxies and point to the common real object.
- Protective Proxy: Protective Proxy is used to create a protective layer over the application. For example in case of Schools where only a few websites are allowed to open with there WiFi.
- Remote Proxy: Remote Proxy is particularly used when the service(real) object is located on a remote server. In such cases, the proxy passes the client request over the network handling all the details.
- Smart proxy: Smart Proxy is used to provide the additional security to the application by introducing specific actions, like asking Passwords, whenever the object would be accessed.
Pros and Cons of Proxy Design Pattern
Pros
- Proxy objects work even when real objects are not available.
- Proxy objects increase the performance of the application by avoiding the instantiation of duplicate objects.
Cons
- Proxy Pattern introduces another layer of abstraction in the application.
- The complexity of code may increase with the introduction of new proxy classes.