A Gantt chart is a type of bar chart that illustrates a project schedule. This chart lists the tasks to be performed on the vertical axis, and time intervals on the horizontal axis. The width of the horizontal bars in the graph shows the duration of each activity.
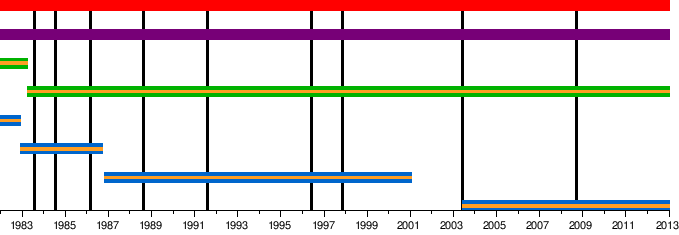
Gantt Charts and Timelines with plotly.express
Plotly Express is the easy-to-use, high-level interface to Plotly, which operates on a variety of types of data and produces easy-to-style figures. With px.timeline
each data point is represented as a horizontal bar with a start and endpoint specified as dates.
The px.timeline
function by default sets the X-axis to be of – type=date
, so it can be configured like any time-series chart.
Implementation
import plotly.express as px
import pandas as pd
- Data frame
df = pd.DataFrame([
dict(Task="Job A", Start='2021-09-01', Finish='2021-11-28'),
dict(Task="Job B", Start='2021-09-05', Finish='2021-11-15'),
dict(Task="Job C", Start='2021-09-20', Finish='2021-11-30')
])
- Creating the timeline
fig = px.timeline(
df,
x_start="Start",
x_end="Finish",
y="Task"
)
fig.show()
Output
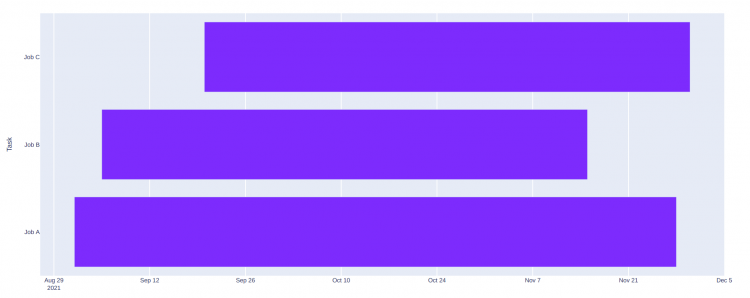
Customizing the colors of the Bars
px.timeline
supports discrete colour as well as continuous colour.
Discrete and Continuous Colour in Plotly Express
The Discrete colour scheme simply means each element of the plot will have a distinct colour. While Continuous colour scheme simply means elements of the plot may have different shades of the same colour.
Most Plotly Express functions accept a color
– argument which automatically assigns data values to discrete colours if the data is non-numeric. If the data is numeric, the colour will automatically be considered continuous.
By default, Plotly Express will use the colour sequence from the active template’s layout.
View available themes
To see information about the available themes and the current default theme, display the plotly.io.templates
configuration object like this.
import plotly.io as pio
pio.templates
Templates configuration
-----------------------
Default template: 'plotly'
Available templates:
['ggplot2', 'seaborn', 'simple_white', 'plotly',
'plotly_white', 'plotly_dark', 'presentation', 'xgridoff',
'ygridoff', 'gridon', 'none']
Coloured Gantt Chart
import plotly.express as px
import pandas as pd
df = pd.DataFrame([
dict(Task="Job A", Start='2021-09-01', Finish='2021-11-28', Resource = "Alice"),
dict(Task="Job B", Start='2021-09-05', Finish='2021-11-15', Resource = "Ron"),
dict(Task="Job C", Start='2021-09-20', Finish='2021-11-30', Resource = "Alice")
])
fig = px.timeline(
df,
x_start="Start",
x_end="Finish",
y="Task",
color = "Resource", #Color attribute
template = "gridon", #Template attribute
)
fig.show()
Output
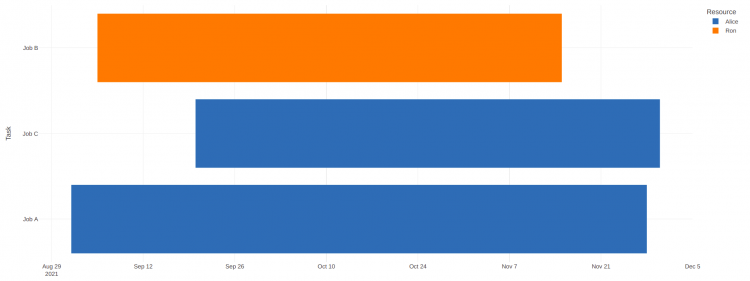
Gantt Chart with Figure Factory
Plotly also has one additional submodule Figure Factory other than plotly_express for various charts/plots. Let’s plot a Gantt chart using the figure factory.
Similar to ploltly_express.timeline
, figure factory has create_gantt()
– method for the Gantt chart.
Implementation
import plotly.figure_factory as ff
df = pd.DataFrame([
dict(Task="Job A", Start='2021-09-01', Finish='2021-11-28', Resource = "Alice"),
dict(Task="Job B", Start='2021-09-05', Finish='2021-11-15', Resource = "Ron"),
dict(Task="Job C", Start='2021-09-20', Finish='2021-11-30', Resource = "Alice")
])
fig = ff.create_gantt(df)
fig.show()
Output
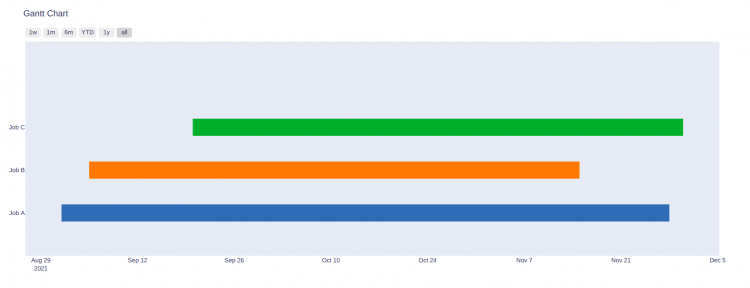